"CLI CPP" refers to the use of C++ commands and scripts executed through the command line interface to enhance programming efficiency and automation.
Here's a simple example of using a C++ program compiled via the command line:
#include <iostream>
int main() {
std::cout << "Hello, CLI CPP!" << std::endl;
return 0;
}
To compile and run this code, you can use the following commands in your terminal:
g++ -o hello hello.cpp
./hello
What is C++ CLI?
C++ CLI is a powerful extension to the C++ programming language that allows developers to utilize .NET features while still maintaining the strengths of C++. It acts as a bridge between native C++ and the .NET framework, making it possible to write code that runs under the common language runtime (CLR). This means that you can leverage the vast libraries available in .NET, offering a rich set of functionalities and features not typically found in standard C++ development.
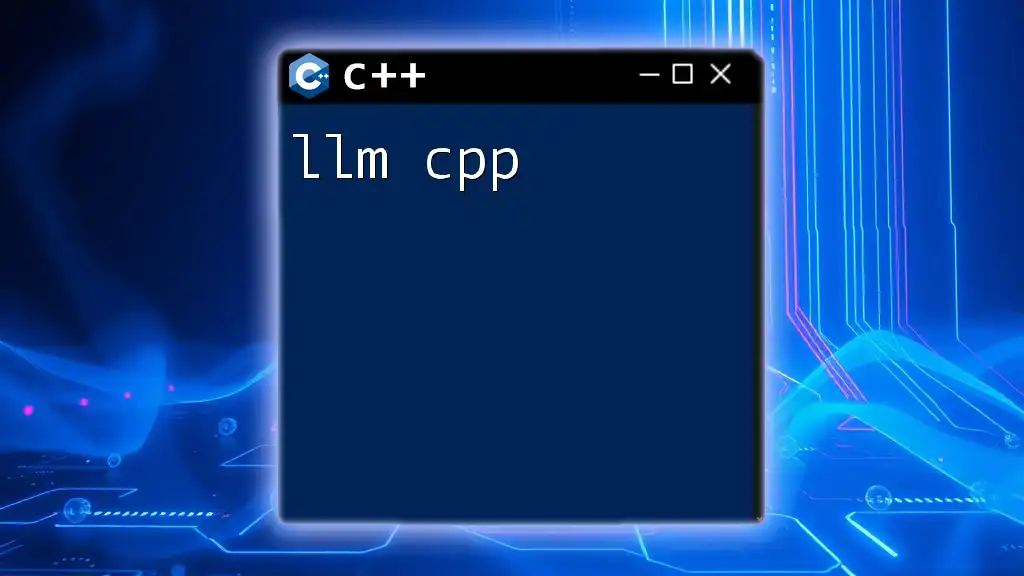
Why Use C++ CLI?
Using C++ CLI comes with several advantages:
- Interoperability: You can seamlessly integrate .NET libraries and features into your existing C++ code, making it easy to leverage a rich ecosystem of components.
- Memory Management: C++ CLI introduces garbage collection, which simplifies memory management, reducing the chances of memory leaks that are common in standard C++ development.
- Performance: By utilizing both the speed of native code and the high-level features of .NET, C++ CLI can achieve impressive performance metrics.
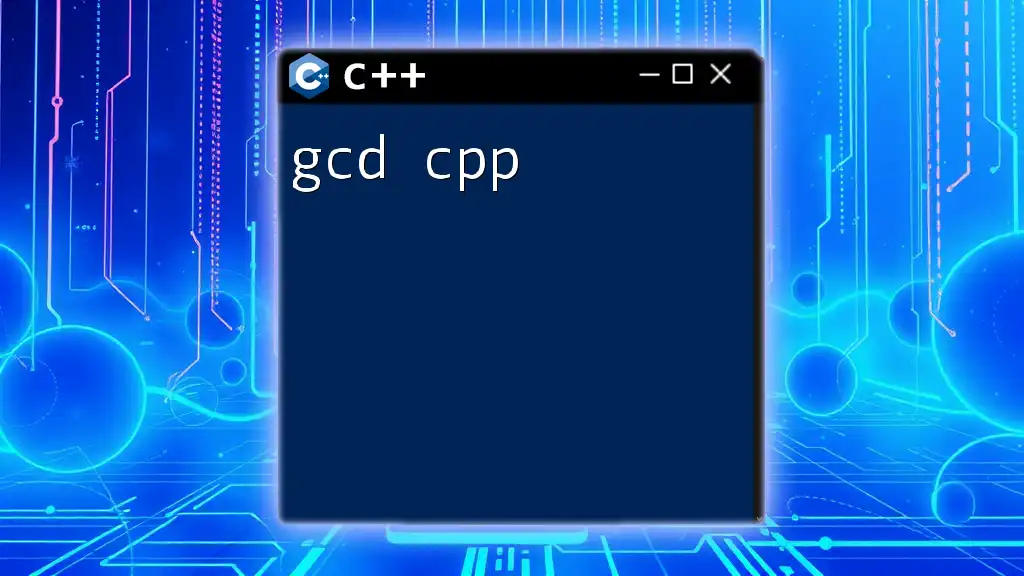
Installing Visual Studio for C++ CLI
To get started, you need to install Visual Studio, which is the most efficient development environment for C++ CLI. Follow these steps:
- Download the Visual Studio installer from the official Microsoft website.
- During the installation, make sure to select the Desktop development with C++ workload, which includes support for C++ CLI, along with other essential tools.
- After installation, launch Visual Studio to begin setting up a new project.
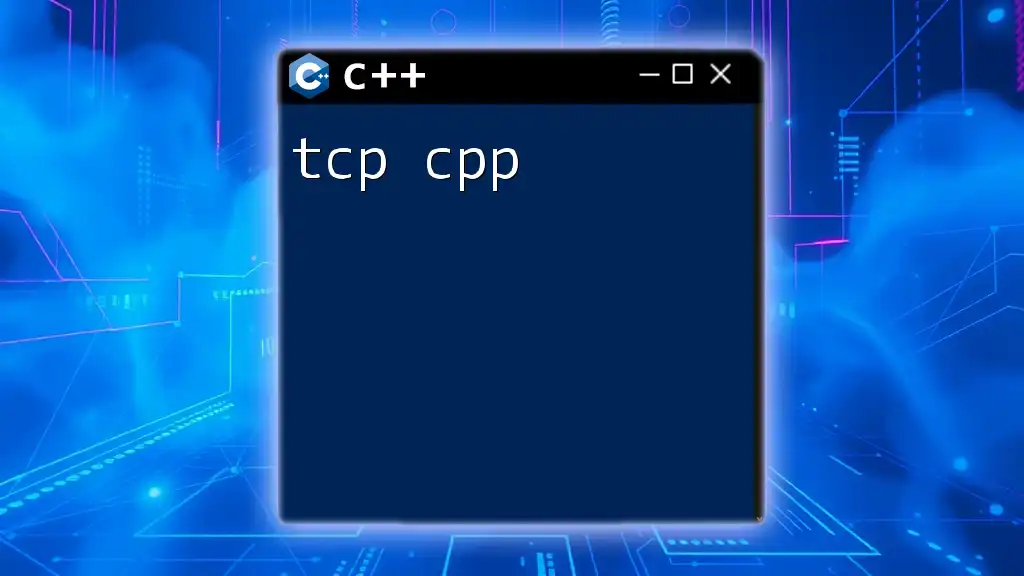
Creating Your First C++ CLI Project
Once Visual Studio is set up, you can create your first C++ CLI project:
- Open Visual Studio and select `Create a new project`.
- Choose the CLR Empty Project template under C++.
- Name your project and specify the location, then click `Create`.
This initializes a project configured for C++ CLI development where you can start writing managed code alongside native C++.
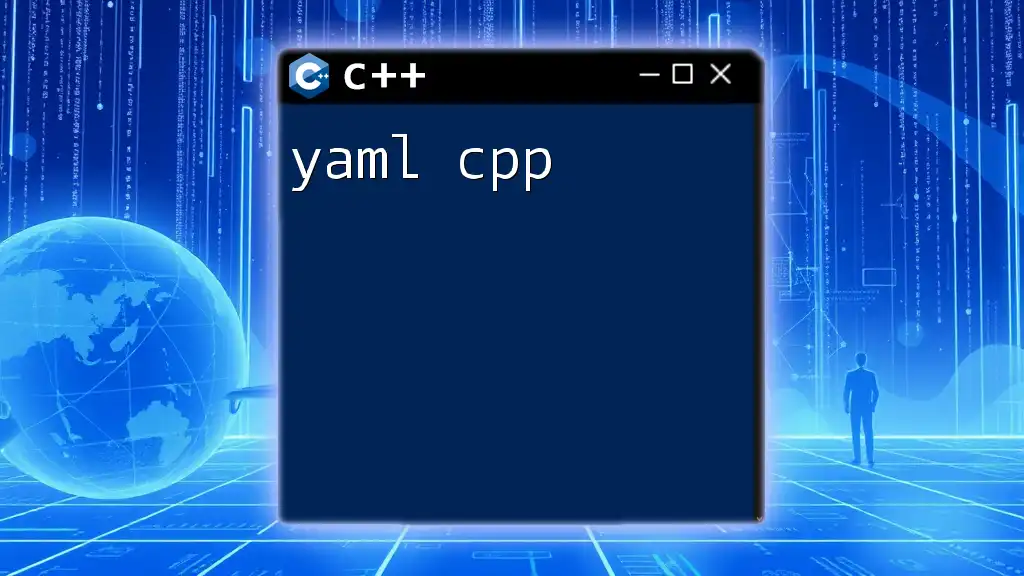
Managed vs. Unmanaged Code
The distinction between managed and unmanaged code is fundamental in C++ CLI development.
- Managed Code is executed by the CLR. It provides features like garbage collection, safety checks, and easy integration with other .NET languages.
- Unmanaged Code, on the other hand, is executed directly by the operating system. It gives developers more control over hardware resources but comes with risks like memory leaks and pointer issues.
Understanding the balance between these two types of code is crucial in C++ CLI development. Utilizing managed code for most high-level operations while falling back on unmanaged code for performance-critical tasks creates robust applications.
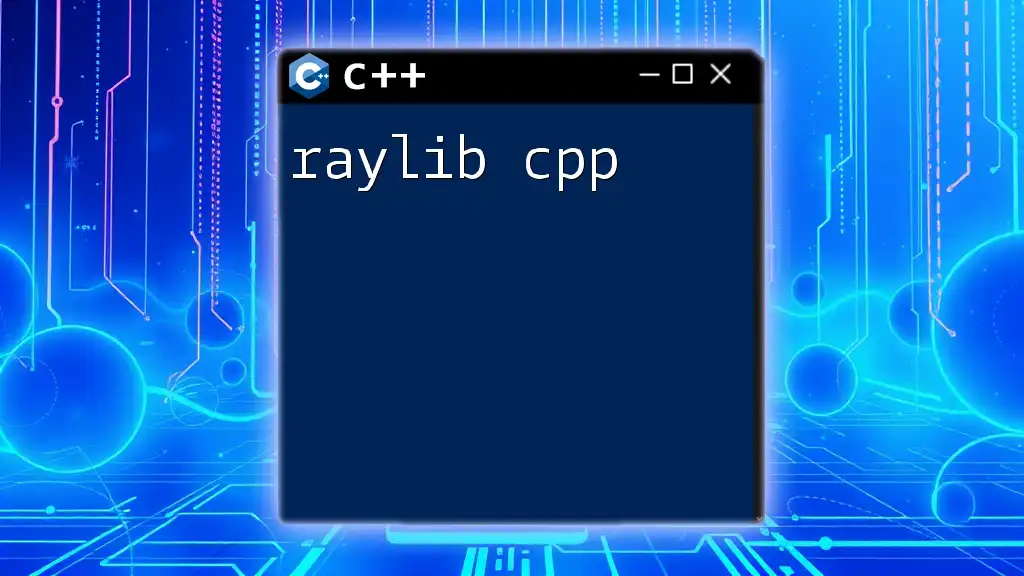
Using the CLI Syntax
C++ CLI introduces new syntax to accommodate managed code. For example, in C++ CLI, you always need to specify the ^ character when declaring a handle to a managed object, as shown below:
Traditional C++:
class MyClass {
public:
void MyMethod() {}
};
MyClass obj;
obj.MyMethod();
C++ CLI:
public ref class MyClass {
public:
void MyMethod() {}
};
MyClass^ obj = gcnew MyClass();
obj->MyMethod();
In this example, `MyClass^` indicates a handle to the managed object, while `gcnew` is used to allocate an instance of `MyClass` on the managed heap.
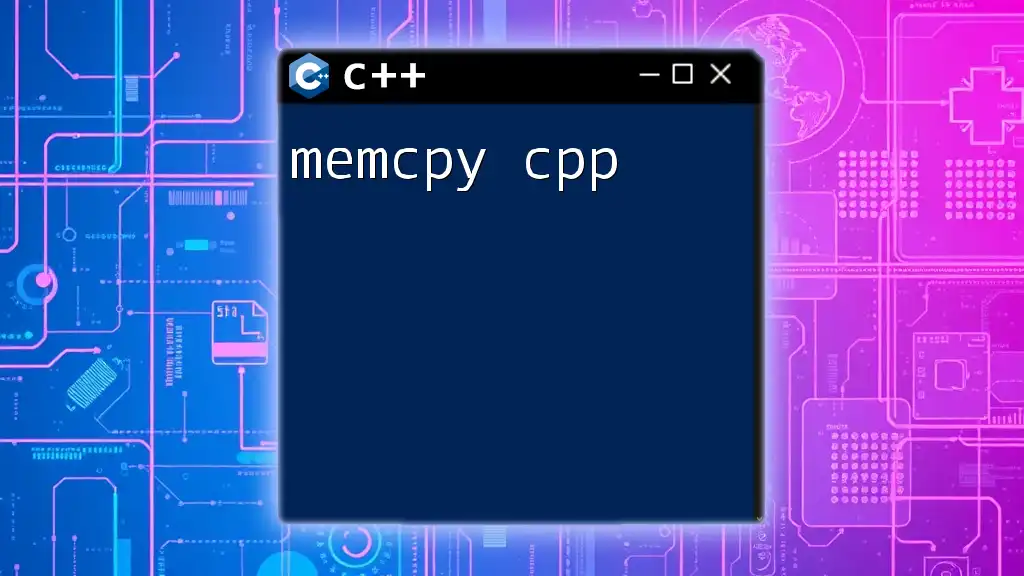
Classes and Objects in C++ CLI
Defining and using classes in C++ CLI follows a specific approach. To create a simple managed class in C++ CLI, you could do the following:
public ref class Person {
private:
String^ name;
int age;
public:
Person(String^ n, int a) : name(n), age(a) {}
void ShowInfo() {
Console::WriteLine("Name: {0}, Age: {1}", name, age);
}
};
In this code snippet, we have a class `Person` with private fields and a constructor. The `ShowInfo()` method demonstrates how to work with C++ CLI's `String^` type.
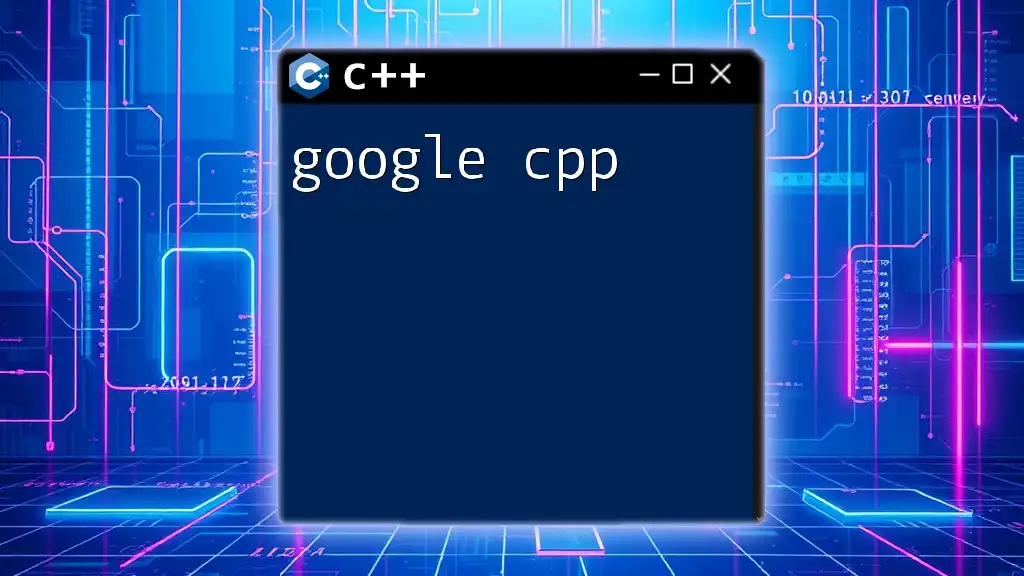
Reference Types vs. Value Types
C++ CLI introduces two main type categories: Reference Types and Value Types.
- Reference Types (e.g., classes) are allocated on the managed heap, and variables hold references to the actual data.
- Value Types (e.g., struct) are allocated on the stack, and variables hold the actual data.
Reference types are more flexible as they support inheritance and polymorphism, while value types are stored by value and are generally more performant. Properly choosing between these types is essential based on your application needs.
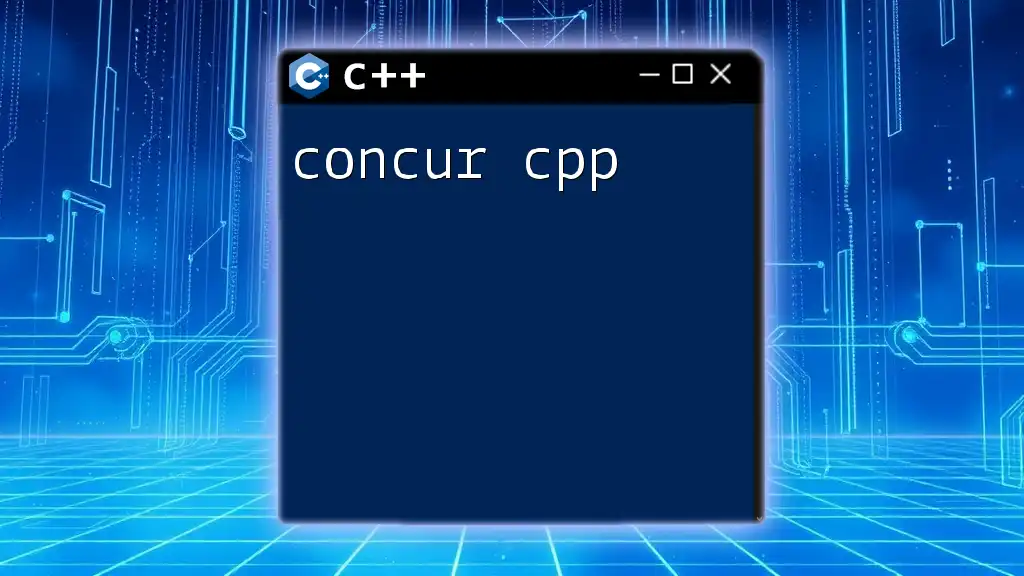
Garbage Collection in C++ CLI
One of the biggest boons of using C++ CLI is automatic memory management through garbage collection. Here's a simple example illustrating a situation where garbage collection would free up memory:
void CreateObject() {
Person^ p = gcnew Person("Alice", 30);
// No need to delete p; it will be collected automatically
}
In the above code, the object `p` is created to be collected automatically when it goes out of scope. This greatly reduces memory management overhead compared to standard C++, where developers are responsible for deallocation.
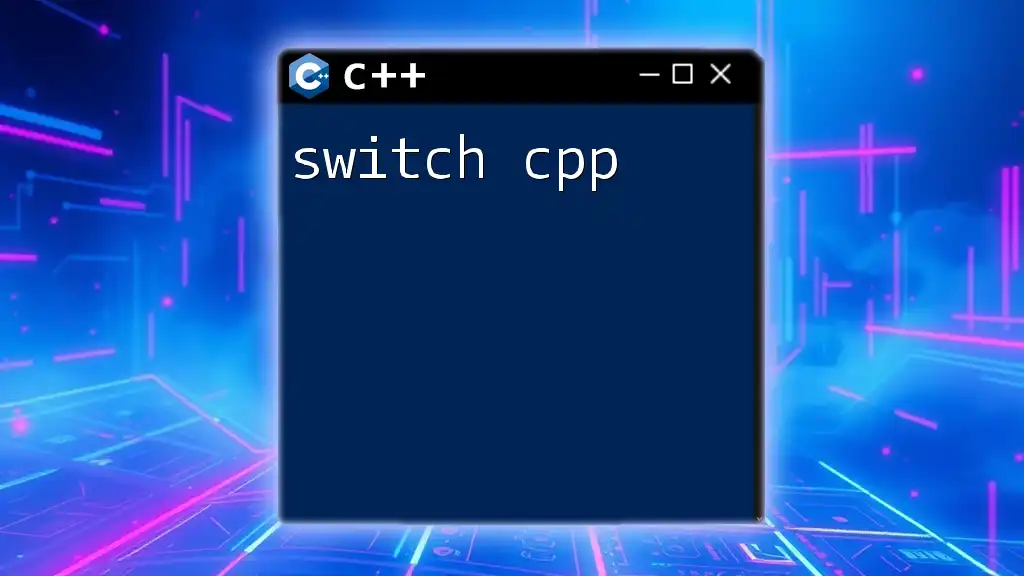
Interoperability with .NET Libraries
C++ CLI provides powerful interoperability features that allow you to call any .NET library directly. Here’s how you can import a .NET library:
- You can include the required namespace using `using namespace`.
- Call classes and methods as needed.
Here's a simple example:
using namespace System;
using namespace System::Collections::Generic;
void UseList() {
List<int>^ myList = gcnew List<int>();
myList->Add(10);
myList->Add(20);
Console::WriteLine("First item: {0}", myList[0]);
}
In this snippet, we create a `List<int>` from the .NET Collections library, add items to it, and access its elements seamlessly within C++ CLI.
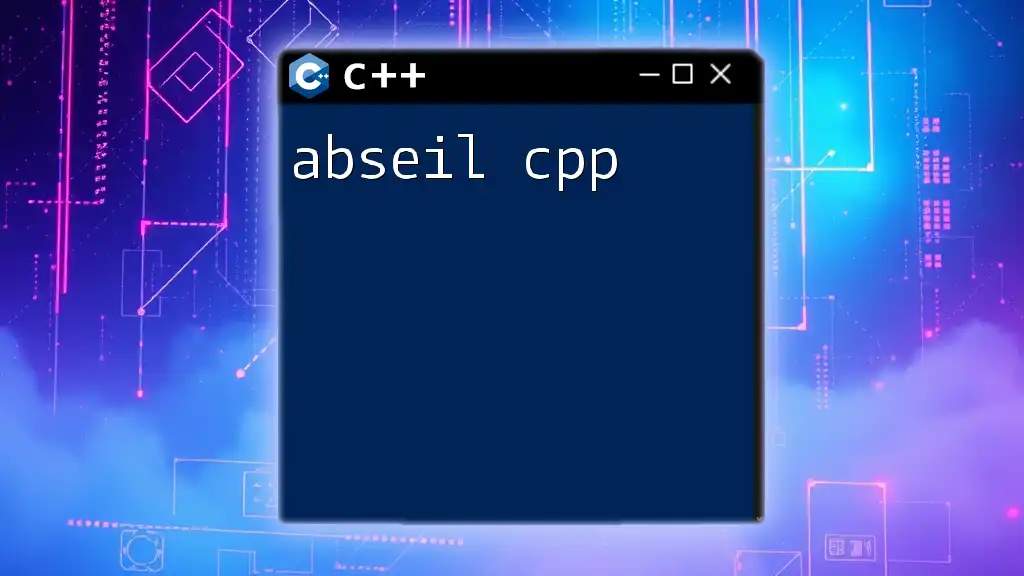
Using Attributes and Properties
Attributes in C++ CLI allow developers to add metadata to classes and methods, enhancing interoperability and functionality. You can create properties easily, as exemplified below:
public ref class Car {
private:
String^ model;
public:
property String^ Model {
String^ get() { return model; }
void set(String^ value) { model = value; }
}
};
In this code, we define the `Model` property, demonstrating how C++ CLI enables easy encapsulation to manage access to class members.
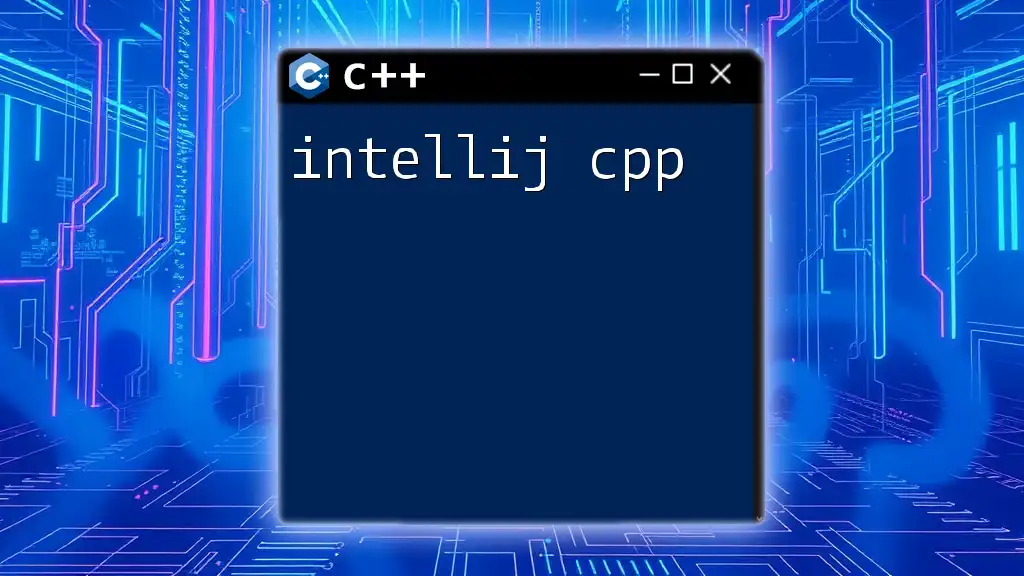
Exception Handling in C++ CLI
Handling exceptions in C++ CLI is similar to .NET's robust exception handling model. Here’s how you can elegantly handle exceptions:
try {
throw gcnew Exception("An error occurred");
} catch (Exception^ ex) {
Console::WriteLine(ex->Message);
}
With this approach, developers can gracefully manage errors while ensuring that resources are properly released as needed.
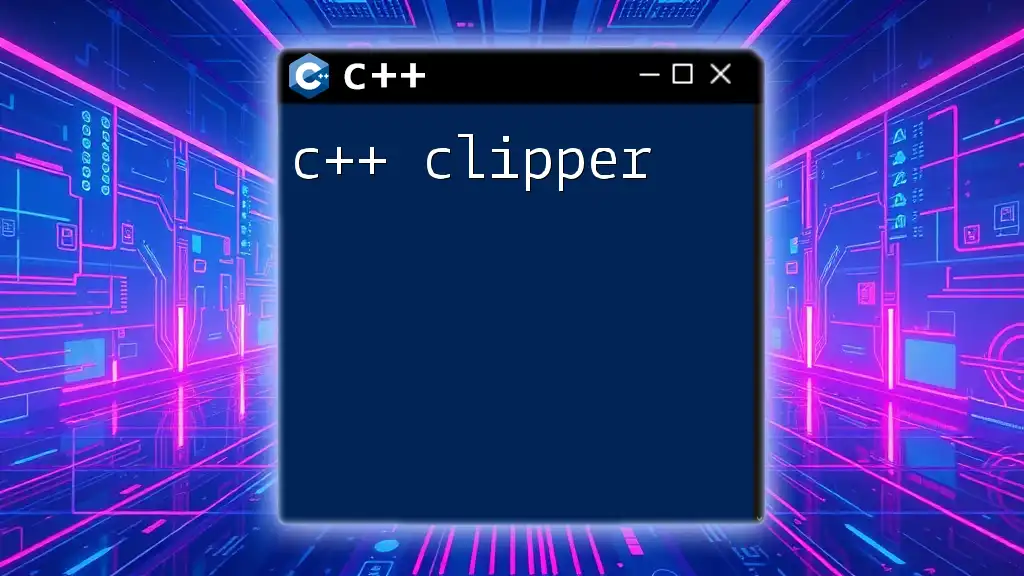
Multithreading and Asynchronous Programming
C++ CLI supports multithreading, allowing developers to write efficient applications that can handle multiple tasks simultaneously. Below is a basic example of asynchronous programming:
void MyAsyncMethod() {
Task::Run(gcnew Action(&SomeLongRunningMethod));
}
void SomeLongRunningMethod() {
// Simulating work...
Thread::Sleep(1000);
Console::WriteLine("Task completed!");
}
This code sets up a task that runs `SomeLongRunningMethod` asynchronously, allowing the main thread to remain responsive.
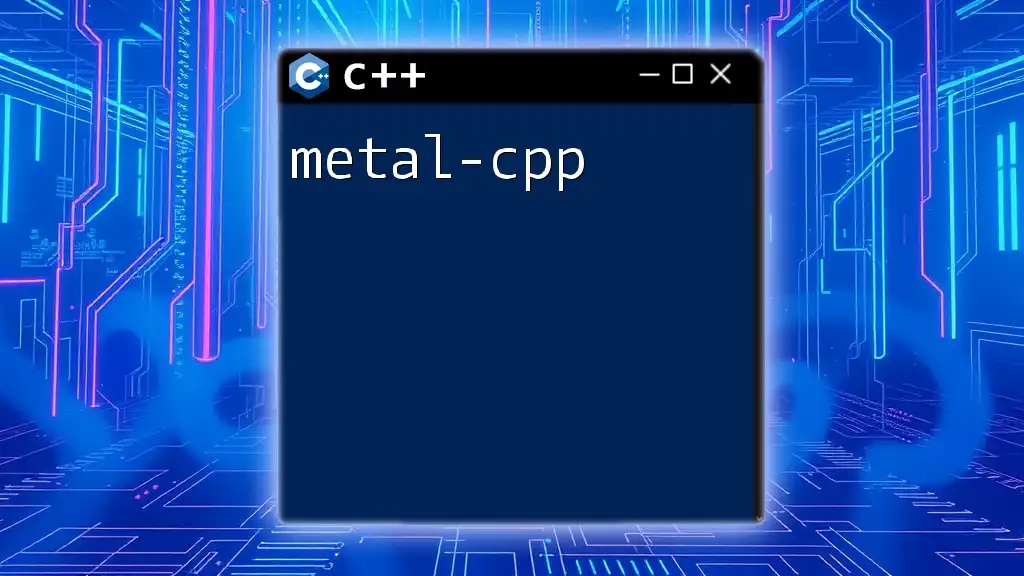
Using LINQ with C++ CLI
C++ CLI also offers the ability to use LINQ (Language Integrated Query), which makes querying collections more intuitive. Here’s an example:
array<int>^ numbers = gcnew array<int>{ 1, 2, 3, 4, 5 };
auto evenNumbers = from n in numbers
where n % 2 == 0
select n;
for each (int n in evenNumbers) {
Console::WriteLine(n);
}
This demonstrates how easily C++ CLI developers can leverage LINQ to efficiently filter collections.
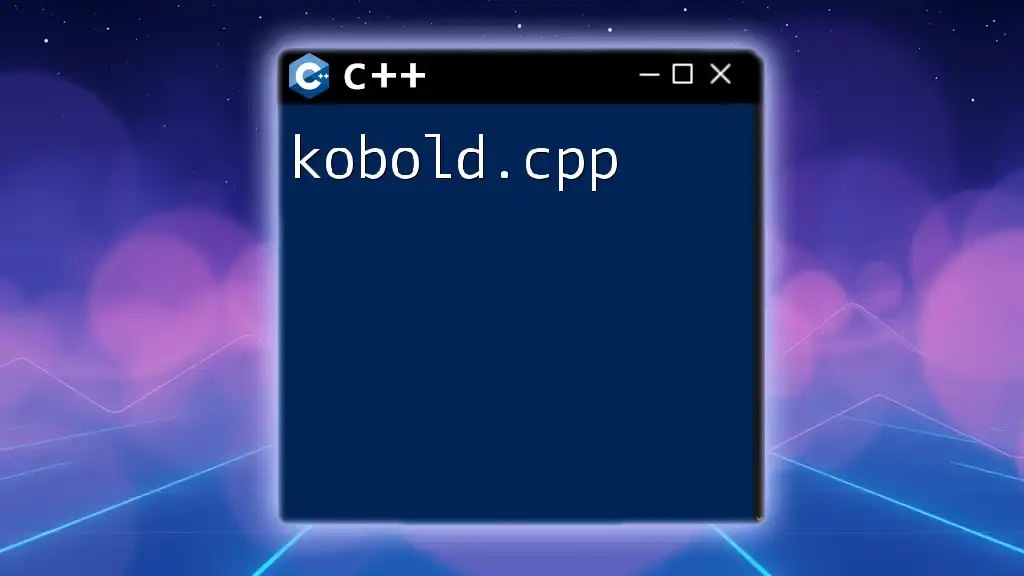
Writing Clean and Maintainable Code
To ensure that your C++ CLI code remains readable and maintainable, consider the following best practices:
- Use meaningful names for classes, methods, and variables.
- Consistently comment your code, explaining the purpose of complex segments.
- Follow standard naming conventions to enhance readability, such as using PascalCase for public classes and methods.
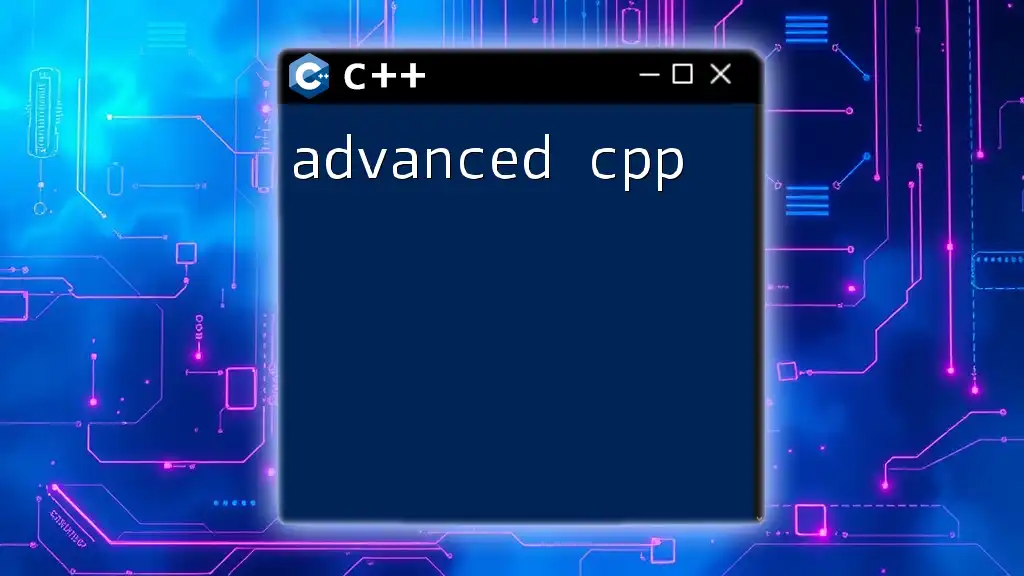
Debugging and Testing Your C++ CLI Applications
Visual Studio provides excellent tools for debugging C++ CLI applications, including breakpoints, watch windows, and step-through debugging. Utilizing these tools can drastically improve code quality. Additionally, implementing unit tests is crucial for verifying functionality:
[TestMethod]
void TestPersonCreation() {
Person^ person = gcnew Person("Bob", 25);
Assert::AreEqual(person->Model, "Bob");
}
In this example, we use a testing framework to validate that our `Person` class's constructor operates correctly.
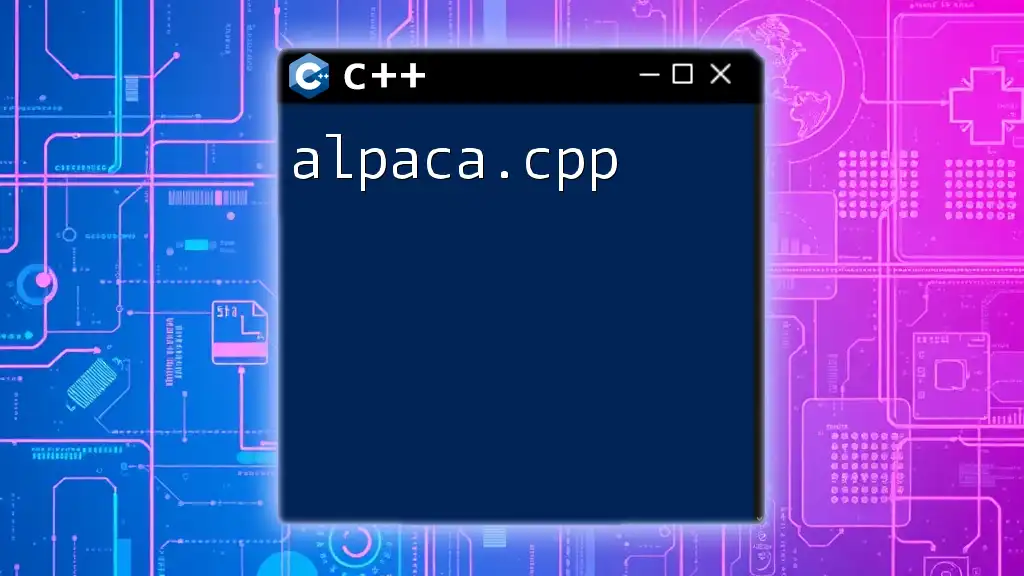
The Future of C++ CLI
As technology continues to evolve, C++ CLI remains relevant due to its unique capabilities in combining the strengths of C++ with the features of the .NET framework. It opens avenues for new applications that can utilize robust libraries while maintaining optimal performance. Aspiring developers are encouraged to dive deeper into C++ CLI, engage with the community, and explore further resources to enhance their skills.
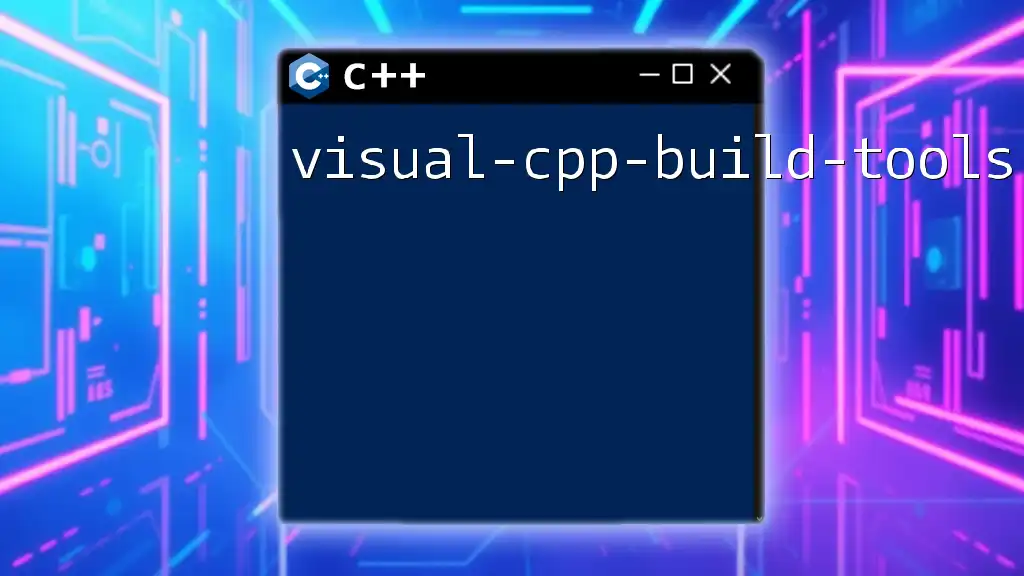
Learning Resources for C++ CLI
Various resources are available to help you deepen your understanding of C++ CLI, including:
- Books: Explore authoritative texts on C++ CLI programming.
- Online Courses: Check platforms like Udemy or Coursera for dedicated C++ CLI courses.
- Tutorials: Many blogs and websites offer step-by-step tutorials for hands-on learning.
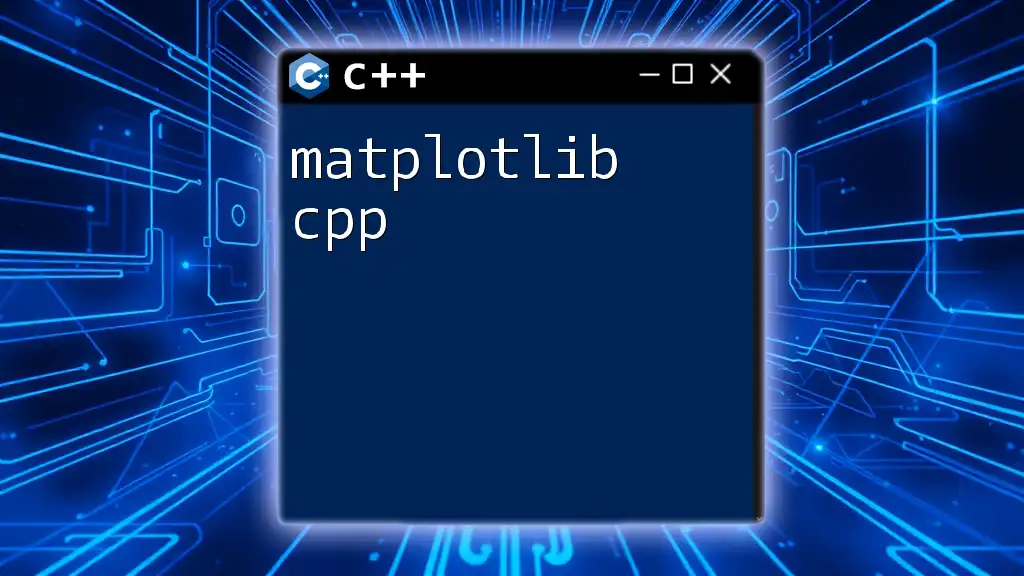
Community and Support
Joining communities such as Stack Overflow, GitHub, or specific C++ forums can offer you support, advice, and resources to overcome challenges in C++ CLI development. Engaging with others who share your interests will enhance your learning experience and provide insights into best practices and modern trends.