"LLM C++ refers to the use of C++ commands within a Large Language Model (LLM) environment to enhance natural language processing capabilities for tasks such as text generation and comprehension."
Here’s a simple code snippet demonstrating a basic LLM interaction using C++:
#include <iostream>
#include <string>
int main() {
std::string prompt = "What is the future of AI?";
std::cout << "Prompting LLM: " << prompt << std::endl;
// Call to LLM API would follow here
return 0;
}
Understanding the Basics of CPP
What is CPP?
C++ is a powerful general-purpose programming language that was developed by Bjarne Stroustrup at Bell Labs in the early 1980s. It is an extension of the C programming language and is widely used for system/software development, game programming, and applications requiring high performance. C++ offers a mix of both low-level and high-level programming capabilities, making it versatile for a broad range of applications.
Key Features of C++
C++ stands out due to several key features that enhance its functionality and versatility:
- Object-oriented programming (OOP): C++ supports OOP principles such as inheritance, encapsulation, and polymorphism, allowing developers to create modular and reusable code.
- Direct memory management: Programmers have the ability to manage memory manually through pointers, which can lead to efficient memory usage if handled correctly.
- Standard Template Library (STL): STL provides a vast collection of algorithms and data structures, enabling developers to handle complex tasks with ease.
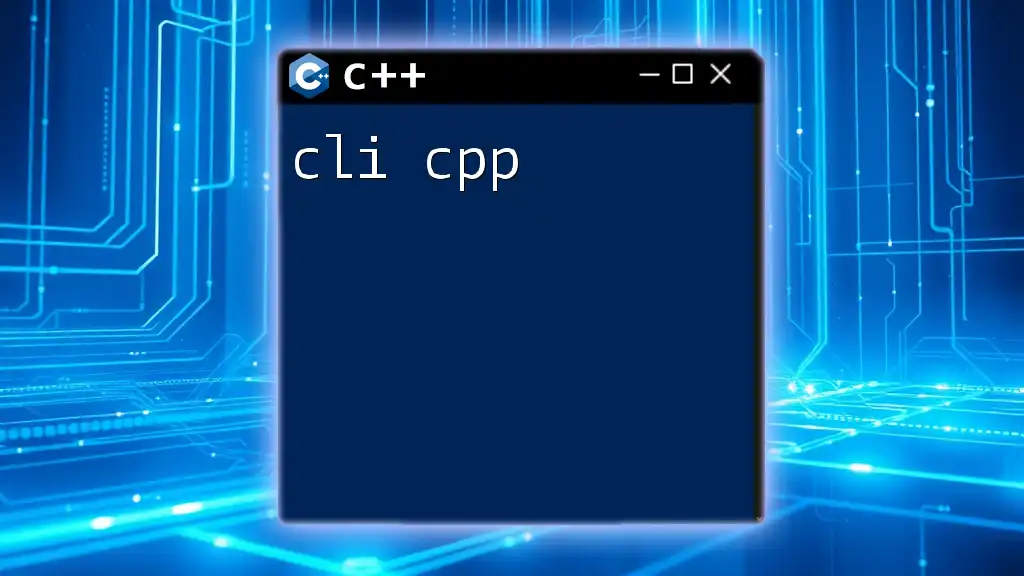
The Intersection of LLM and CPP
What is LLM CPP?
LLM CPP refers to the integration of Large Language Models (LLMs) with C++ programming. LLMs such as OpenAI's GPT and Hugging Face's Transformers can significantly enhance C++ applications by automating coding tasks, generating code snippets, and providing advanced natural language processing capabilities.
Benefits of Using LLM with C++
The combination of LLMs and C++ offers several advantages:
- Enhanced productivity: Developers can save time by utilizing LLMs to generate code or perform complex tasks that would otherwise require extensive manual coding.
- Improved debugging capabilities: LLMs can assist in detecting and correcting code errors by analyzing code structure and syntax, making debugging easier for programmers.
- Simplified complex algorithm implementations: LLMs can generate complex algorithms based on high-level descriptions, simplifying the programming process for developers.
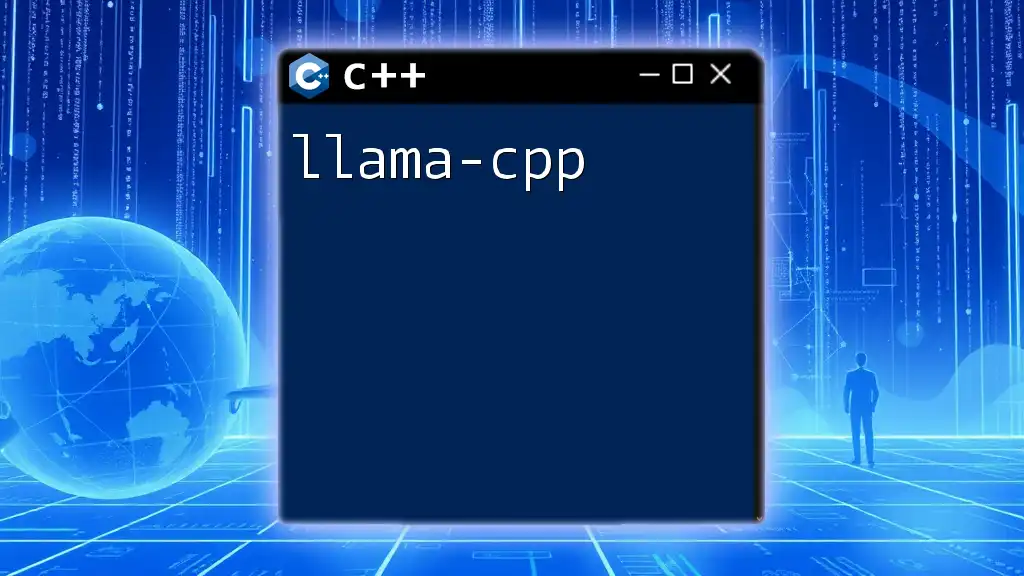
Setting Up Your Environment for LLM CPP
Required Tools and Software
To get started with LLM CPP, the following tools and software are essential:
- C++ Compiler: You need a C++ compiler such as GCC or Clang to compile and run your C++ code.
- Integrated Development Environment (IDE): Popular IDEs like Visual Studio, CLion, or Code::Blocks offer excellent support for C++ development.
- LLM APIs: Integrating LLM APIs such as OpenAI's GPT or Hugging Face's Transformers will enable you to access advanced language model functionalities.
Configuration Steps
Once you have the required tools, configuring them is essential:
- Install a C++ compiler suitable for your operating system.
- Set up your chosen IDE, ensuring it recognizes the C++ compiler and configure any necessary libraries.
- Integrate LLM APIs according to their documentation, ensuring you have valid API keys and required libraries installed.
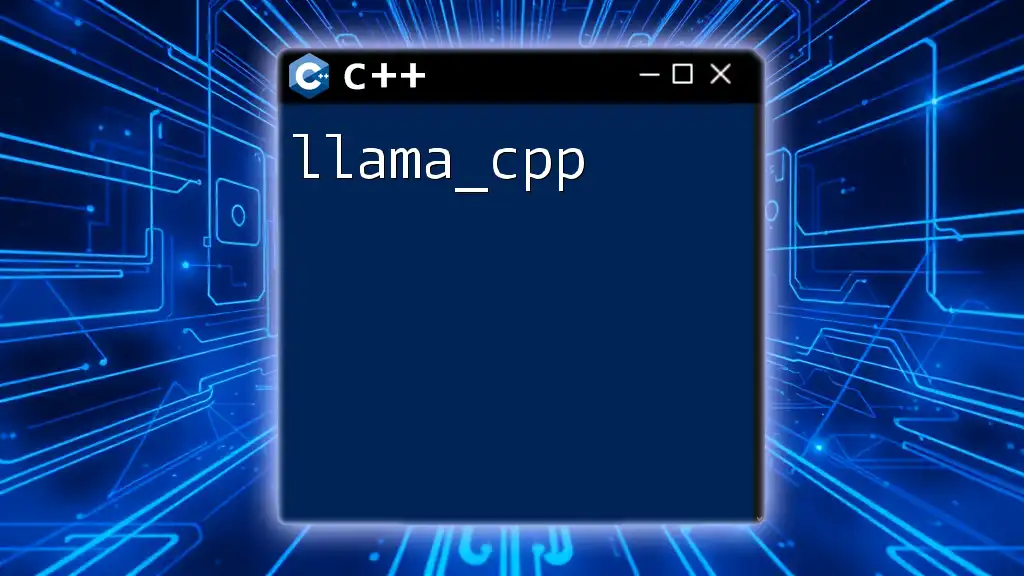
Working with LLM in C++
Basic Commands and Syntax
Interacting with an LLM in C++ requires basic commands and syntax. Here’s an example of how to initialize an LLM model in C++:
#include <llm_api.h>
int main() {
LLMModel model("gpt-3-performer");
model.load(); // Load the model
model.generate("Provide an explanation for the CPP basics"); // Generate a response
return 0;
}
This snippet shows how to include the LLM API, create a model instance, load the model, and generate text based on input.
Common Use Cases
LLM CPP can be applied in various contexts, including:
- Text generation: Automating the creation of written content such as documentation or articles.
- Code generation and completion: Generating boilerplate code or completing code snippets based on context.
- Natural language processing (NLP) tasks: Performing tasks like sentiment analysis or language translation directly within C++.
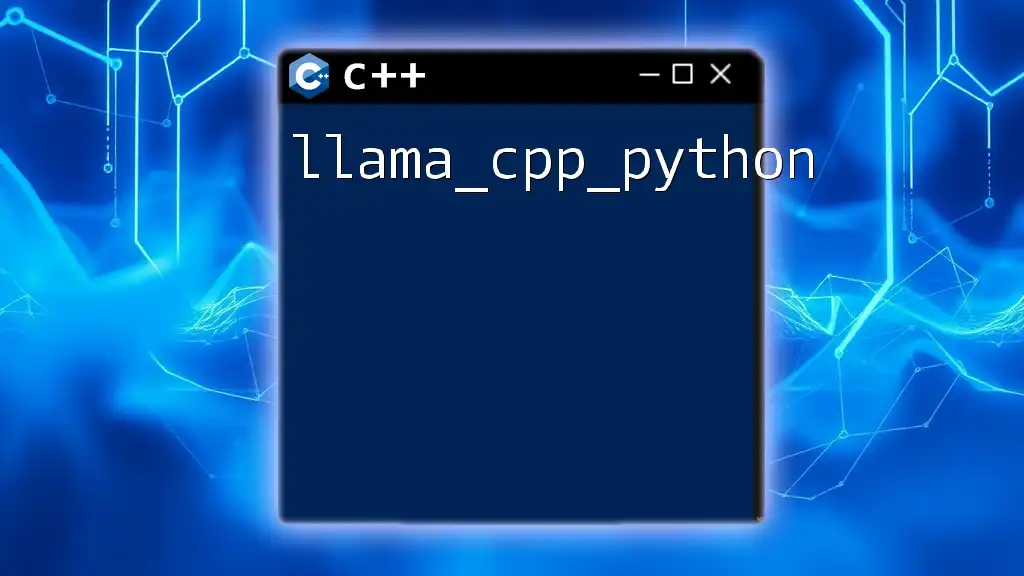
Advanced Techniques with LLM CPP
Fine-tuning LLM Models in C++
Fine-tuning an LLM model can drastically improve its performance for specific tasks. Fine-tuning involves adjusting model parameters to better suit your needs. Here's how to set fine-tuning parameters:
model.setLearningRate(0.001); // Set the learning rate for training
model.setEpochs(5); // Specify the number of training epochs
By customizing these parameters, developers can create models that are more efficient and better aligned with their project’s requirements.
Integrating LLM Output into CPP Applications
Once you have obtained output from an LLM, leveraging it in your C++ applications is vital. Here’s an example of capturing and utilizing LLM responses:
std::string output = model.generate("Simplify the example above");
std::cout << "LLM Response: " << output << std::endl;
In this snippet, we store the LLM's generated output in a string and then print it to the console. This integration is crucial for utilizing the model's intelligence effectively within applications.
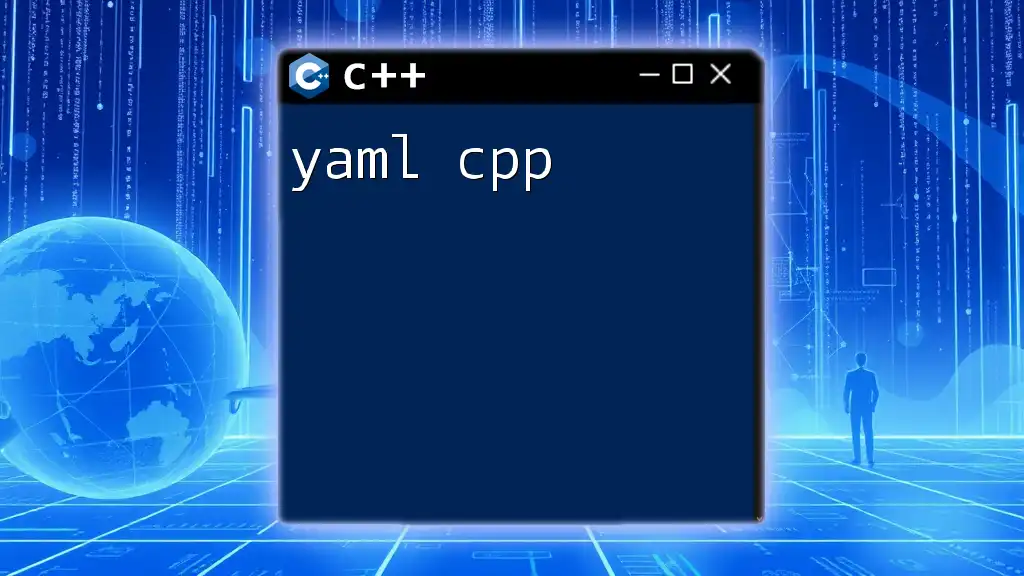
Challenges and Best Practices
Common Challenges with LLM CPP
While integrating LLMs with C++, you may encounter several challenges:
- Performance issues: Running complex models can slow down applications unless optimized properly.
- Handling model biases: LLMs can reflect biases present in training data, so it’s essential to be critical of their outputs.
- Errors and debugging strategies: Debugging LLM output can be complex, requiring a solid understanding of both the model and C++ code.
Best Practices for Efficient LLM Usage
To maximize the effectiveness of LLM CPP, consider these best practices:
- Optimizing model parameters: Experiment with different settings to find the optimal configuration for your application.
- Code organization and management: Maintain organized code structures to facilitate easier debugging and collaboration.
- Leveraging community resources: Engage with forums and discussions to share experiences and learn from others facing similar challenges.
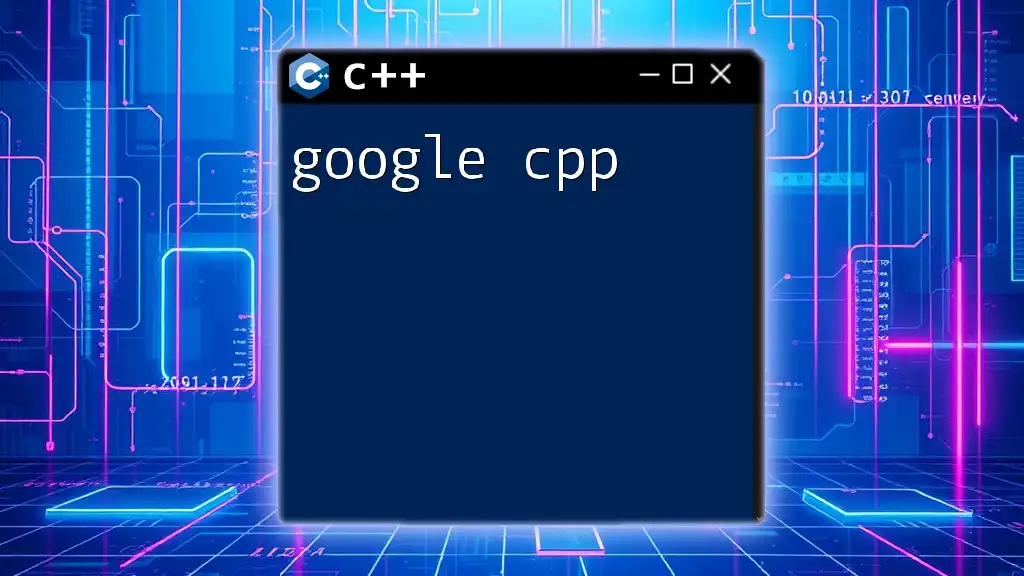
Real-World Applications of LLM CPP
Industry Applications
The applications of LLM CPP are vast, with several industries harnessing its potential:
- Finance: Used for developing algorithmic trading systems that can process market data and make quick, informed decisions.
- Healthcare: Automates tasks like patient document summaries or generates alerts for physicians based on available data.
- Game development: Assists in procedural content generation, enhancing gaming experiences by creating dynamic game scenarios.
Case Studies
Several organizations have successfully implemented LLM CPP, showcasing positive outcomes:
- A tech startup in the finance sector utilized LLM CPP to automate risk assessment, leading to quicker report generation and improved decision-making.
- A healthcare provider adopted LLM capabilities to streamline patient information processing, resulting in faster diagnosis times and improved patient care quality.
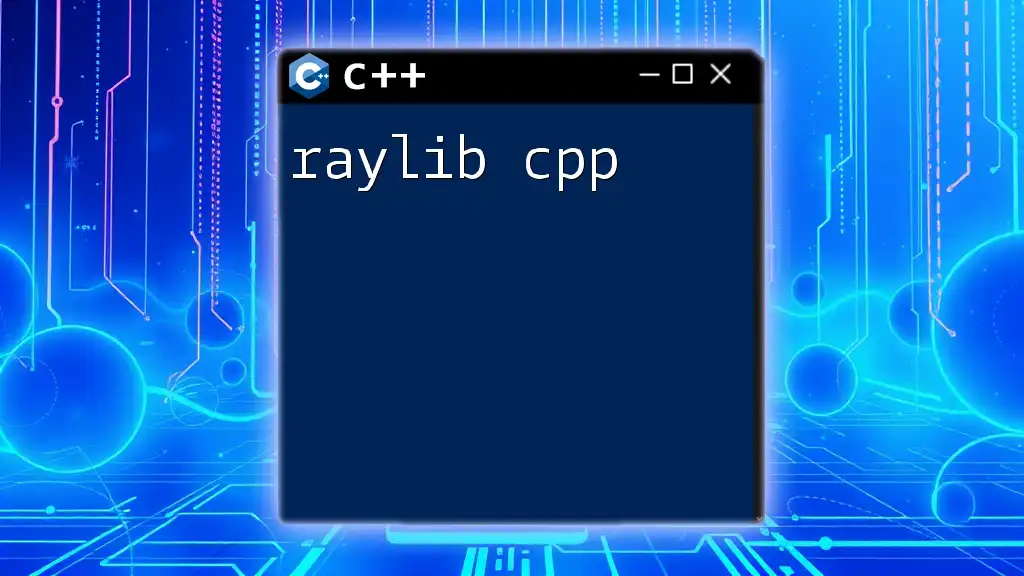
Conclusion
The integration of LLMs with C++ programming is not just an innovative approach; it is a transformative methodology that enhances productivity, simplifies complex tasks, and broadens the scope of what developers can achieve. As technology evolves, the future looks bright for LLM CPP, offering countless opportunities for businesses and developers alike to explore and experiment.
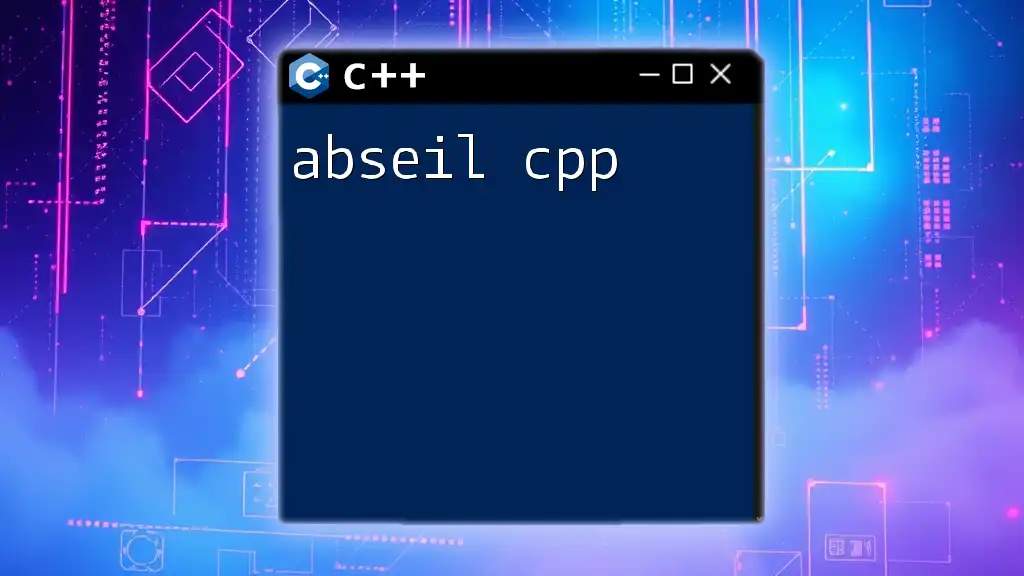
Call to Action
Join our C++ training program to delve deeper into the integration of LLMs with C++. Explore available resources for practice and engage with community forums to sharpen your skills and knowledge. Don’t miss out on the chance to harness the power of LLM CPP for your projects!
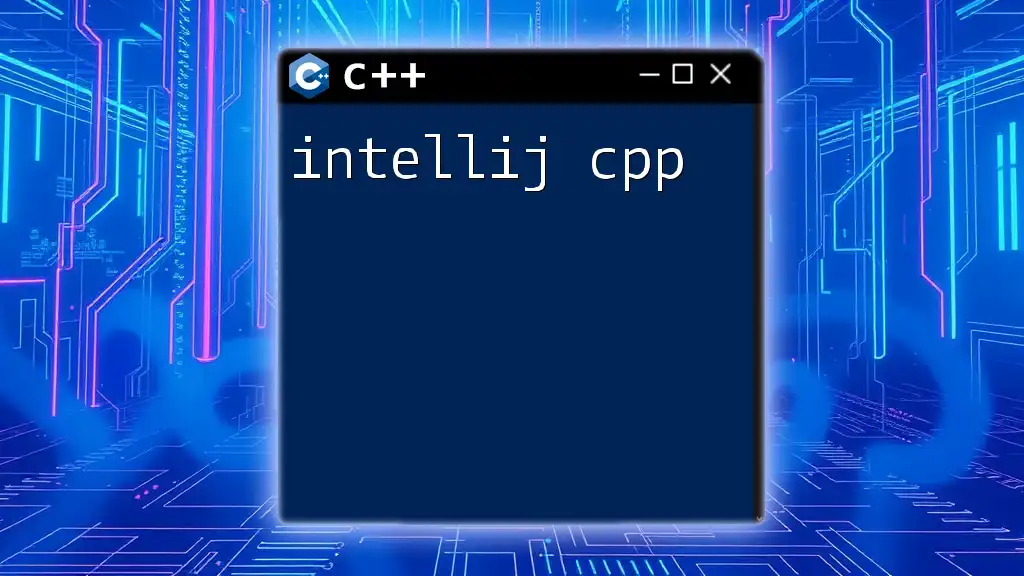
Additional Resources
Recommended Books and Online Courses
Discover some of the best books and online courses that can enhance your understanding of both C++ and LLMs.
Useful Libraries and Toolkits
Explore libraries that can facilitate your LLM CPP development journey.
Community and Support Forums
Participate in discussions and seek help from fellow developers and C++ experts who are navigating similar paths.
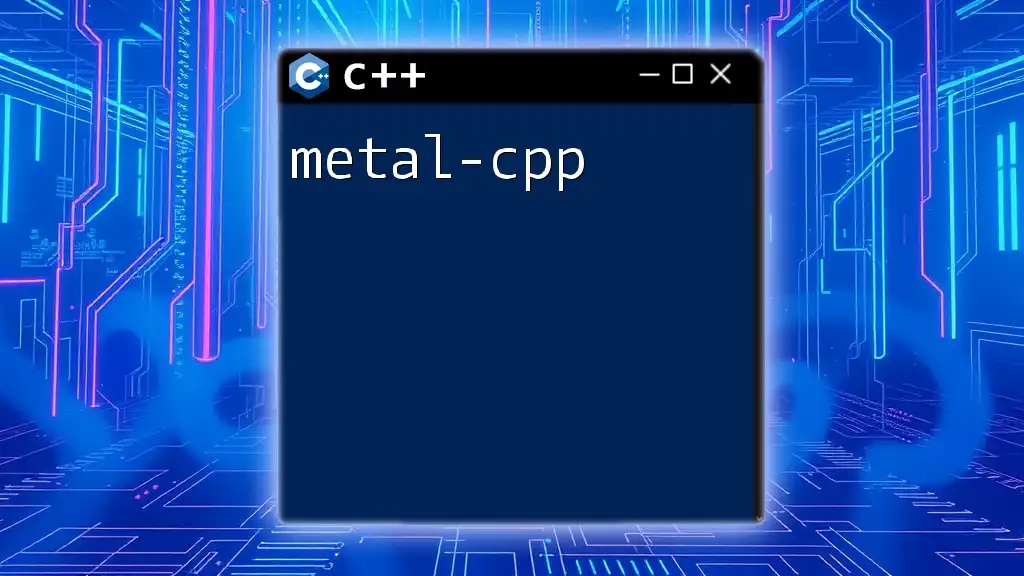
FAQs about LLM CPP
The integration of LLM CPP raises several questions. Addressing commonly asked inquiries can dispel misconceptions and guide newcomers on their journey. Clarifying these points allows for a better understanding of how LLMs can effectively augment C++ programming capabilities.