Abseil C++ is a collection of open-source C++ libraries developed by Google that provides common libraries that complement the C++ standard library, enabling developers to create more efficient and maintainable code.
Here's a simple code snippet demonstrating the usage of Abseil's `absl::StrCat` for string concatenation:
#include "absl/strings/str_cat.h"
#include <iostream>
int main() {
std::string name = "World";
std::string greeting = absl::StrCat("Hello, ", name, "!");
std::cout << greeting << std::endl; // Outputs: Hello, World!
return 0;
}
What is Abseil?
Definition of Abseil
Abseil is a collection of C++ libraries designed to augment the C++ standard library with new functionalities that make programming easier and more efficient. Developed primarily for internal use at Google, Abseil aims to provide primitives that support the development of high-performance, complex systems. Even though it originated at Google, it is now available as an open-source library, enabling broader use across various projects and platforms.
Key Features
Abseil provides a range of features that distinguish it from the standard C++ libraries. Here are some** key highlights**:
-
Cross-platform compatibility: Abseil works seamlessly across multiple platforms, making it suitable for diverse development environments.
-
Component-based design: The libraries are designed to be modular, meaning developers can include only the components they need without unnecessary overhead.
-
Compatibility with existing Standard C++ Libraries: Abseil is crafted to integrate smoothly with existing C++ code bases, ensuring that developers can leverage their existing knowledge and libraries.
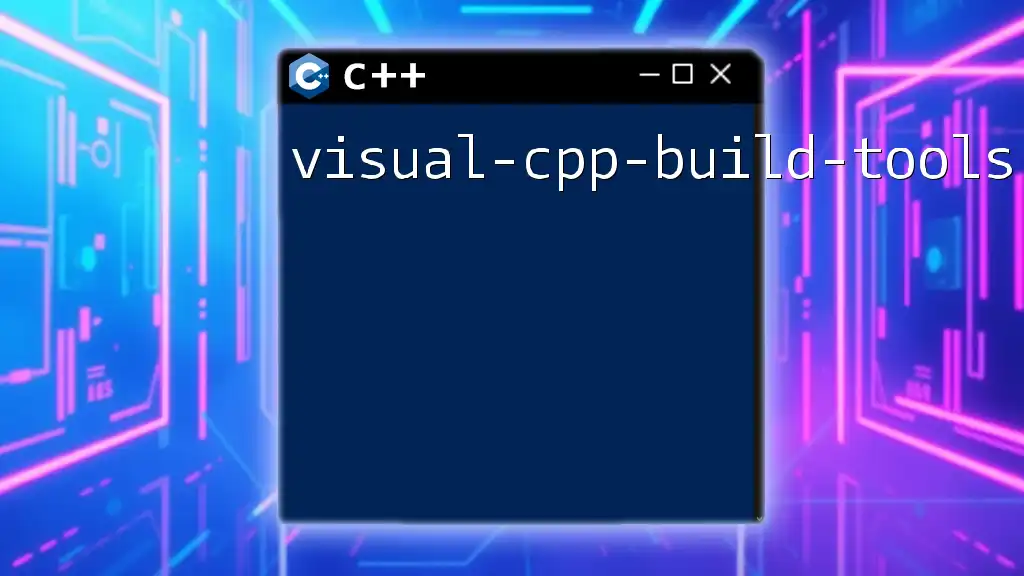
Getting Started with Abseil
Installation
To get started with Abseil, the first step is installing the library. The most common method is via CMake.
Using CMake
To add Abseil as a dependency in your CMake project, you can specify the following in your `CMakeLists.txt`:
cmake_minimum_required(VERSION 3.12)
project(MyProject)
# Add Abseil as a subdirectory
add_subdirectory(path/to/abseil)
# Link against Abseil libraries
target_link_libraries(MyProject absl::strings absl::time absl::flat_hash_map)
Basic Setup
Once installed, ensure that you've properly configured your project to include Abseil. A good way to verify the setup is by compiling a simple program.
#include "absl/strings/string_view.h"
#include <iostream>
int main() {
absl::string_view str = "Hello, Abseil!";
std::cout << str << std::endl;
return 0;
}
This code snippet simply verifies that you can include Abseil components in your program successfully.
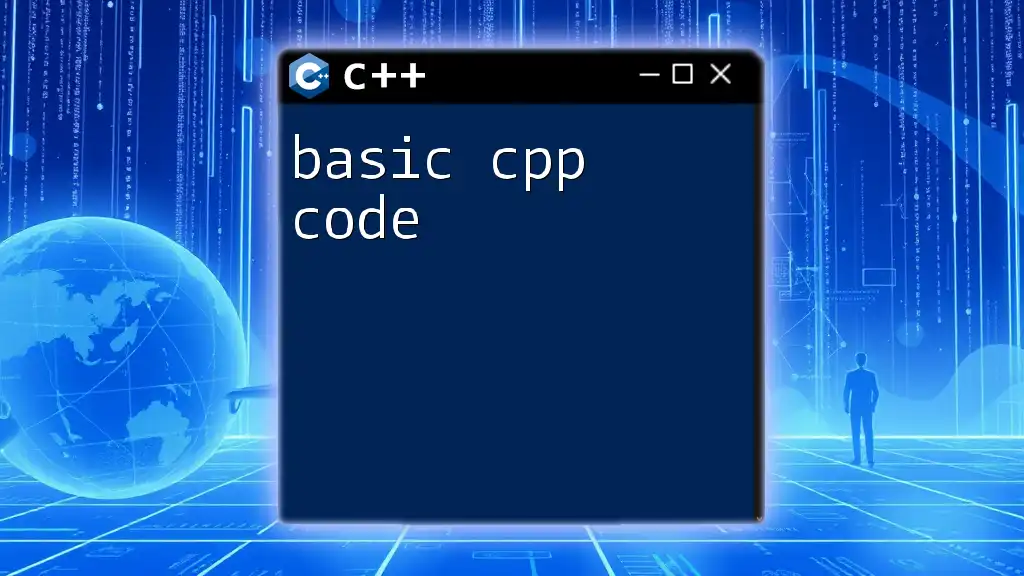
Core Components of Abseil
Abseil Strings
Introduction to Abseil Strings
One of the most fundamental abstractions in Abseil is its string handling utilities. Abseil strings offer improved performance and flexibility compared to Standard C++ strings. Concepts like `absl::string_view` allow for more efficient string manipulation without unnecessary memory allocations.
Key Functions and Usage
The primary string utility is `AbslStringRef`, which offers read-only access to character data, significantly reducing overhead in situations where modifications are not required.
Example:
#include "absl/strings/string_view.h"
#include <iostream>
void PrintString(absl::string_view str) {
std::cout << str << std::endl;
}
int main() {
absl::string_view greeting = "Hello, Abseil!";
PrintString(greeting);
return 0;
}
Abseil Time
Understanding Abseil Time Types
Time management is critical in any application, and Abseil offers `absl::Time` and `absl::Duration` to effectively manage and represent time intervals and points in time.
Key Functions and Examples
Use `absl::Now()` to get the current time, and measure elapsed time with ease:
Example:
#include "absl/time/clock.h"
#include "absl/time/time.h"
#include <iostream>
int main() {
absl::Time start = absl::Now();
// ... your code here ...
absl::Duration elapsed = absl::Now() - start;
std::cout << "Elapsed time: " << absl::ToInt64Milliseconds(elapsed) << " ms" << std::endl;
return 0;
}
Abseil Status and Errors
Error Handling with Abseil
Error handling can be a cumbersome task in C++. Abseil streamlines this process using `absl::Status`, which enables you to manage error states explicitly, helping to reduce bugs and improve code reliability.
Key Functions and Examples
The `absl::Status` class comes with built-in error types and statuses, which can facilitate clearer error reporting.
Example:
#include "absl/status/status.h"
#include <iostream>
absl::Status StatusExample() {
return absl::NotFoundError("Item not found");
}
int main() {
absl::Status status = StatusExample();
if (!status.ok()) {
std::cout << "Error: " << status.message() << std::endl;
}
return 0;
}
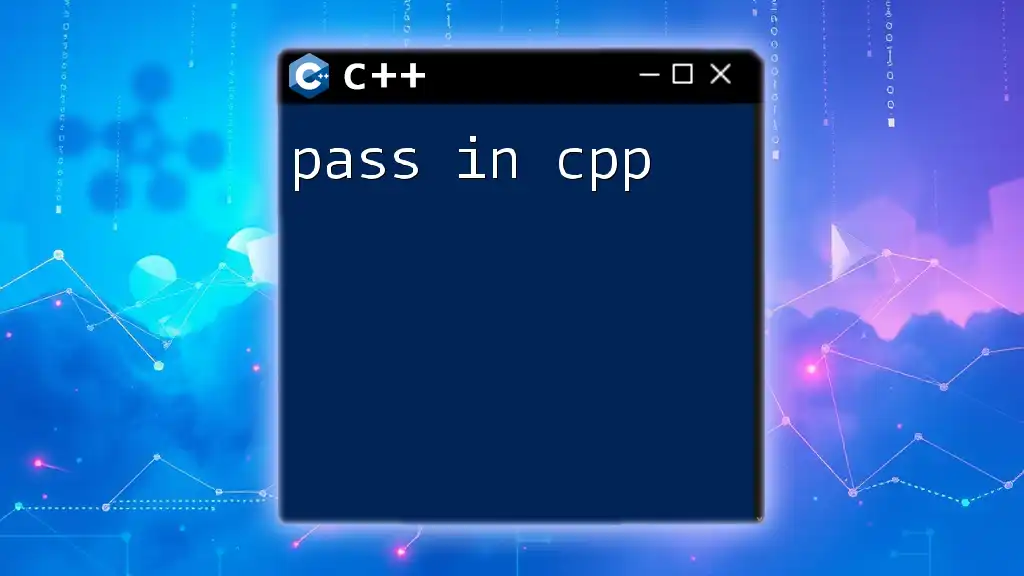
Advanced Features in Abseil
Abseil Containers
Overview of Container Classes
Abseil also offers improved container classes, such as `absl::flat_hash_map` and `absl::flat_hash_set`, providing alternatives that often outperform standard STL containers due to their optimizations.
Usage Examples
Here’s how you can utilize `absl::flat_hash_map`:
Example:
#include "absl/container/flat_hash_map.h"
#include <iostream>
int main() {
absl::flat_hash_map<int, std::string> map;
map[1] = "One";
map[2] = "Two";
for (const auto& pair : map) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Abseil Flags
Introduction to Command-Line Flags
Command-line flags simplify configuration management across different execution environments. Abseil provides a robust system for defining and using these flags.
Key Functions and Usage
You can define your flags using `DEFINE_*` macros and retrieve them easily during runtime.
Example:
#include "absl/flags/flag.h"
#include "absl/flags/parse.h"
#include <iostream>
DEFINE_string(name, "default_value", "Description of valid flag");
int main(int argc, char* argv[]) {
absl::ParseCommandLine(argc, argv);
std::cout << "Flag value: " << FLAGS_name << std::endl;
return 0;
}
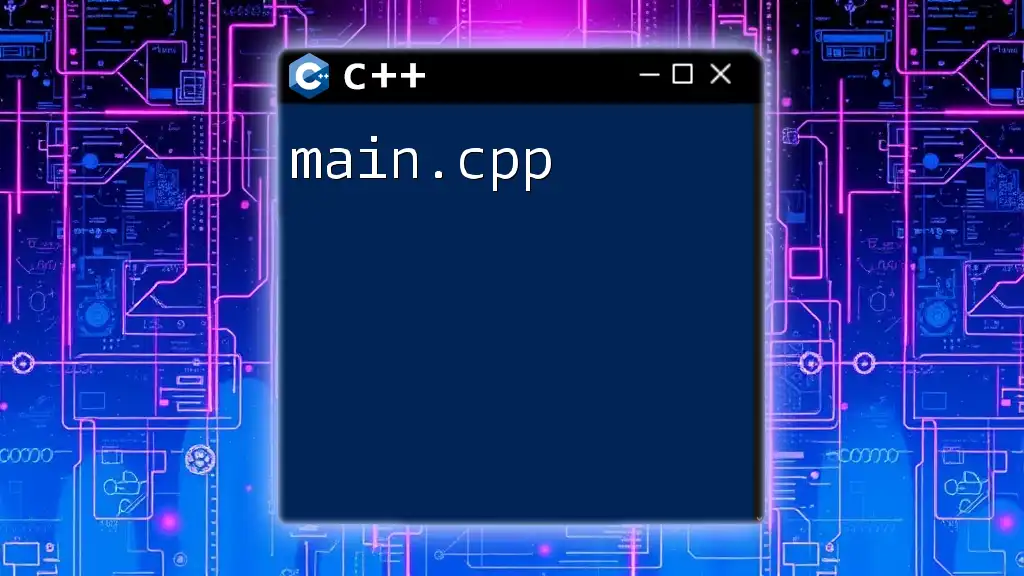
Best Practices for Using Abseil
When using Abseil, adhere to the following best practices:
-
Use Abseil components wisely: Integrate only the libraries necessary for your project to minimize bloat.
-
Test for compatibility: While Abseil strives to be backward compatible with Standard C++, always test your project when integrating new dependencies.
-
Stay updated: Abseil continues to evolve, so ensure that you keep your libraries up-to-date to leverage the latest optimizations and features.
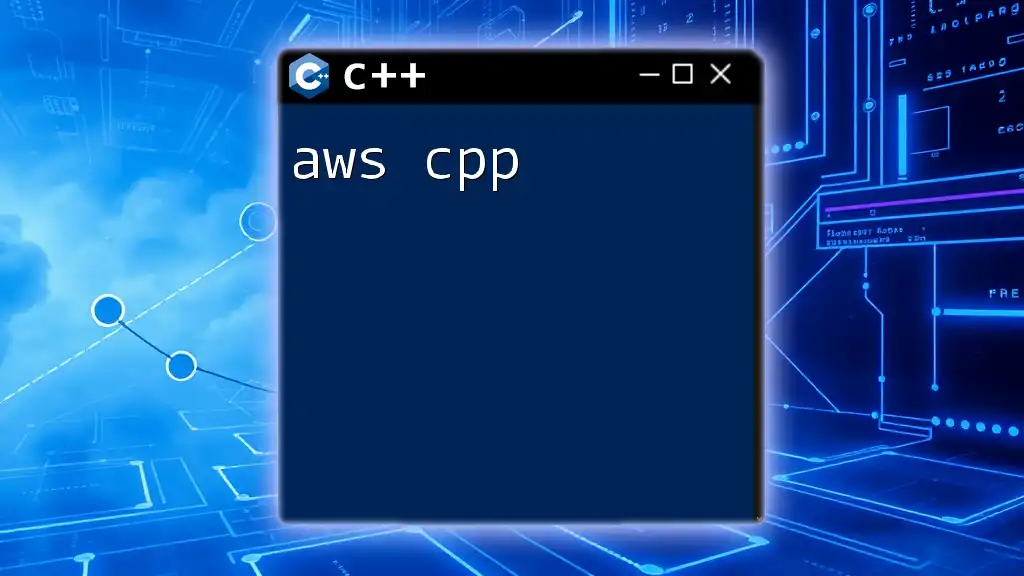
Use Cases and Applications
Abseil is utilized in various real-world applications, particularly in software systems that require efficient performance and robust error handling. Whether you're building a web server, databases, or large-scale data processing applications, Abseil's array of tools can significantly speed up your development process and enhance the final product.
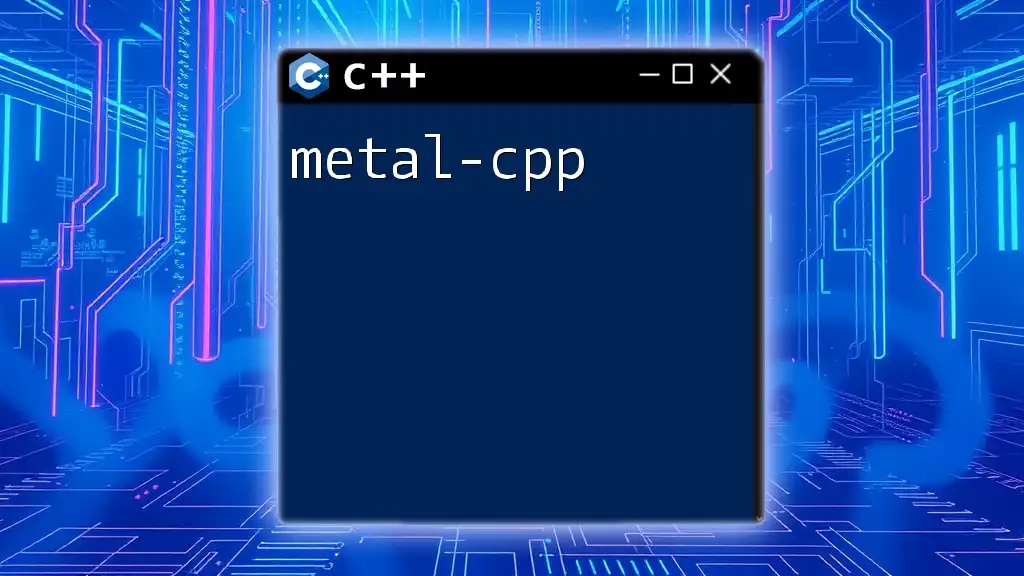
Conclusion
Incorporating Abseil C++ into your tooling can be a game-changer for your development workflow. With its rich set of libraries designed for performance and ease of use, Abseil serves as a valuable asset for developers looking to enhance their productivity and code quality.
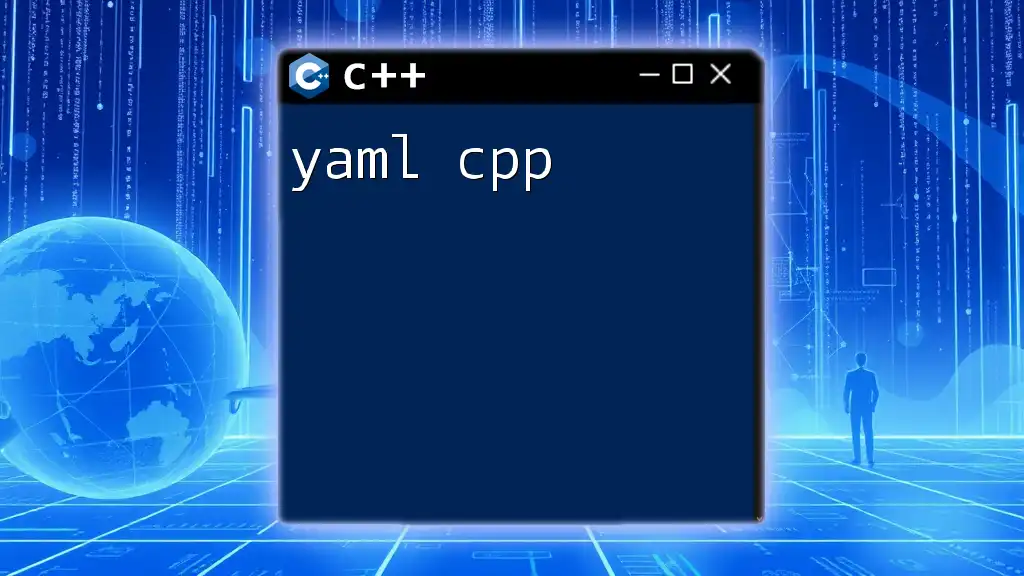
Additional Resources
To further explore Abseil and its functionalities, check out the [official Abseil documentation](https://abseil.io/docs/cpp). Community forums and GitHub repositories also provide platforms for discussions, examples, and additional learning materials. For those committed to mastering modern C++ practices with Abseil, consider diving into specialized books and courses on the subject.
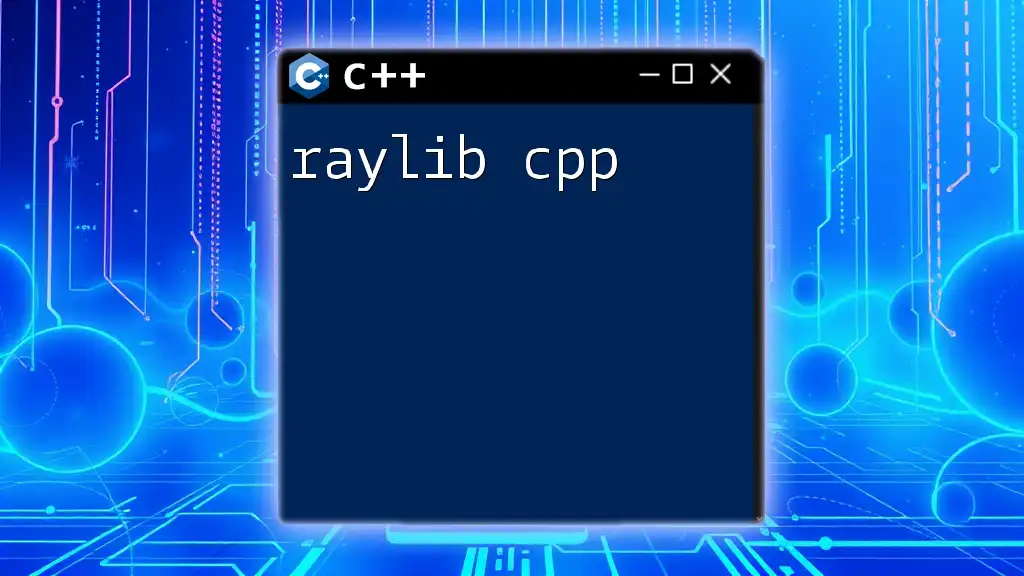
Call to Action
Start leveraging Abseil C++ in your projects today! If you have experiences or questions, feel free to share your thoughts in the comments.