In C++, templates are a powerful feature that allows you to create generic and reusable functions or classes that can operate with any data type.
Here's a simple example of a function template that returns the maximum of two values:
template <typename T>
T getMax(T a, T b) {
return (a > b) ? a : b;
}
What are C++ Templates?
C++ templates are a powerful feature of the C++ programming language that enables developers to write generic and reusable code. They allow you to define functions and classes with placeholders for data types, making it possible to create algorithms that can work with any data type without sacrificing type safety.
The key benefits of using templates include:
- Code Reusability: Write a function or class once and use it for different data types.
- Type Safety: Compiler checks ensure that the correct types are used during the instantiation of templates, reducing runtime errors.
- Flexibility: Easily adapt your code to work with various data types without rewriting it.
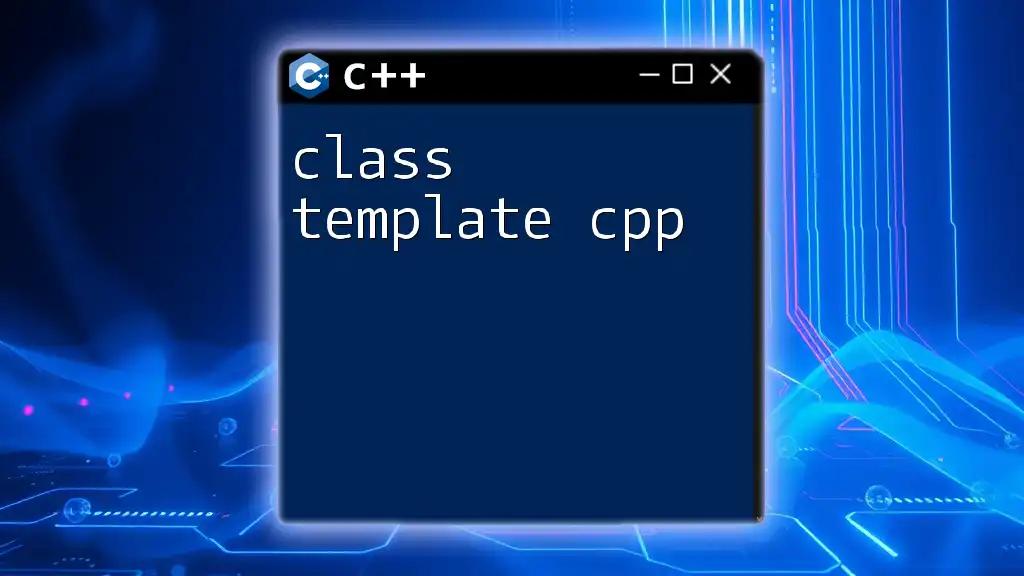
History and Evolution of Templates in C++
Templates were introduced in C++ as a way to achieve generic programming, which encourages code reuse and abstraction. Since their introduction, templates have evolved significantly with improvements in modern C++ standards such as C++11, C++14, C++17, and C++20. This evolution has included enhancements like variadic templates, better template specialization, and improved compile-time computations.
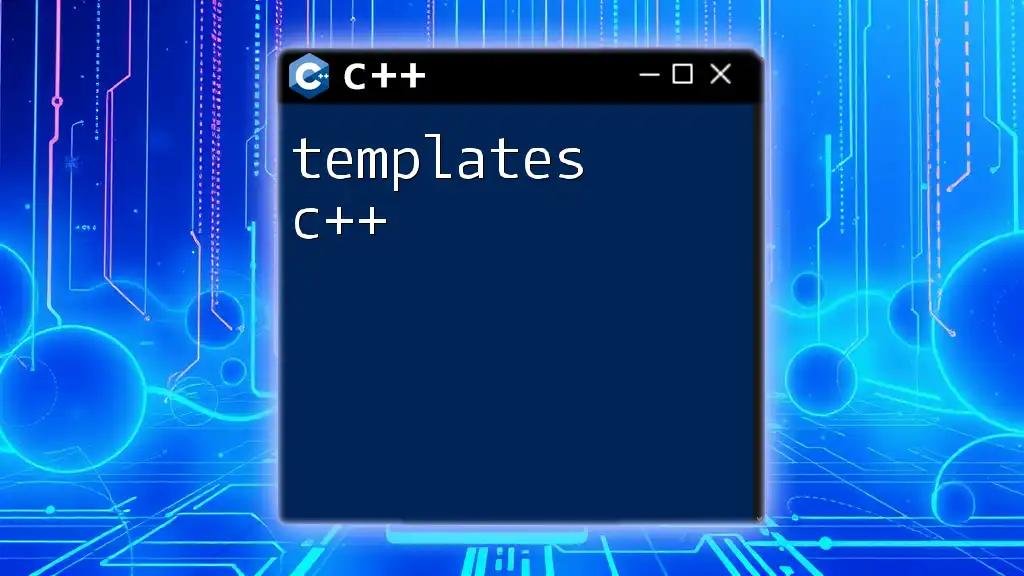
Types of C++ Templates
Function Templates
Definition and Use Cases
Function templates allow you to create functions that can operate on any data type. This is particularly useful for writing generic algorithms that can perform operations on multiple data types without needing multiple versions of the same function.
Code Example
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example, `add` is a function template that takes two parameters of type `T` and returns their sum. This function works for any data type that supports the `+` operator, such as integers, floats, or custom types. For example:
int intSum = add(5, 10); // Works with integers
double doubleSum = add(5.5, 10.5); // Works with doubles
Class Templates
What are Class Templates?
Class templates allow you to define a class that can handle any data type, providing a way to create generic data structures or algorithms that are type-independent.
Code Example
template <typename T>
class Box {
private:
T value;
public:
Box(T value) : value(value) {}
T getValue() { return value; }
};
In this example, `Box` is a class template that can store a value of any type `T`. This enables you to create instances such as `Box<int>`, `Box<double>`, or even `Box<std::string>`. This reduces code duplication and makes maintenance easier.
Alias Templates
When and Why to Use Alias Templates
Alias templates simplify complex data type definitions by providing an alternate name. This can be particularly helpful in cases where the type is verbose or nested.
Code Example
template <typename T>
using Vec = std::vector<T>;
In this snippet, `Vec` is defined as an alias for `std::vector<T>`. This simplifies the declaration of vector types throughout your code, enhancing readability and maintainability. For example, instead of writing `std::vector<int>`, you can simply write `Vec<int>`.
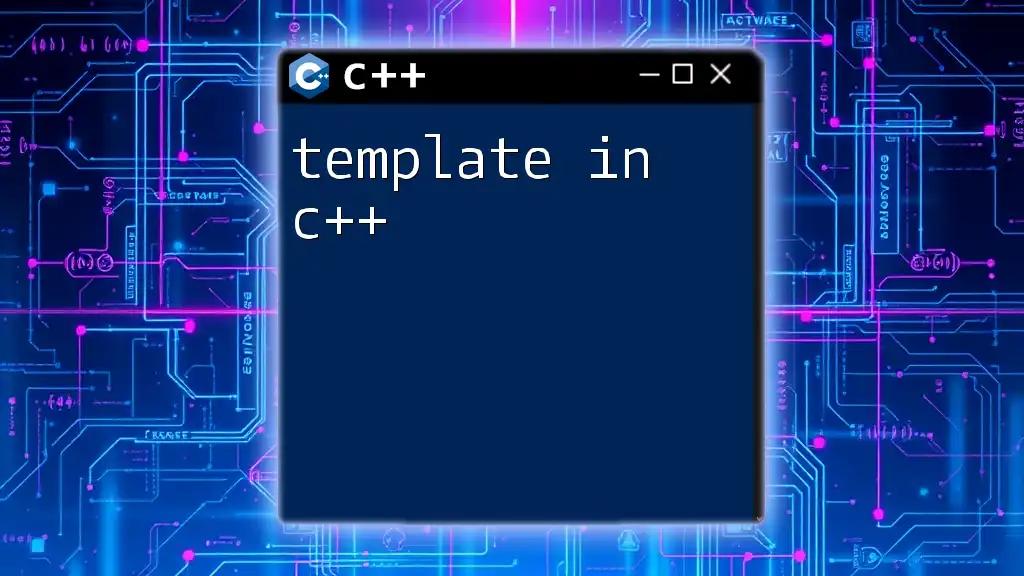
Template Specialization
What is Template Specialization?
Template specialization allows you to define a specific implementation of a template for a particular type. This is useful when you need to handle specific data types differently from the generic implementation.
Full Specialization vs. Partial Specialization
- Full Specialization: This involves defining a completely customized version of a template for a specific type.
- Partial Specialization: This allows you to customize a template for a subset of its parameters.
Code Example of Full Specialization
template <>
class Box<int> {
private:
int value;
public:
Box(int value) : value(value) {}
int getValue() { return value; }
};
This code shows full specialization of the `Box` class template for the type `int`. It allows you to customize the implementation for integer values.
Code Example of Partial Specialization
template <typename T>
class Box<T*> {
private:
T* value;
public:
Box(T* value) : value(value) {}
T* getValue() { return value; }
};
In this example, partial specialization is demonstrated by defining a specific implementation for pointer types. This distinguishes it from the general template and enables unique handling for pointers.
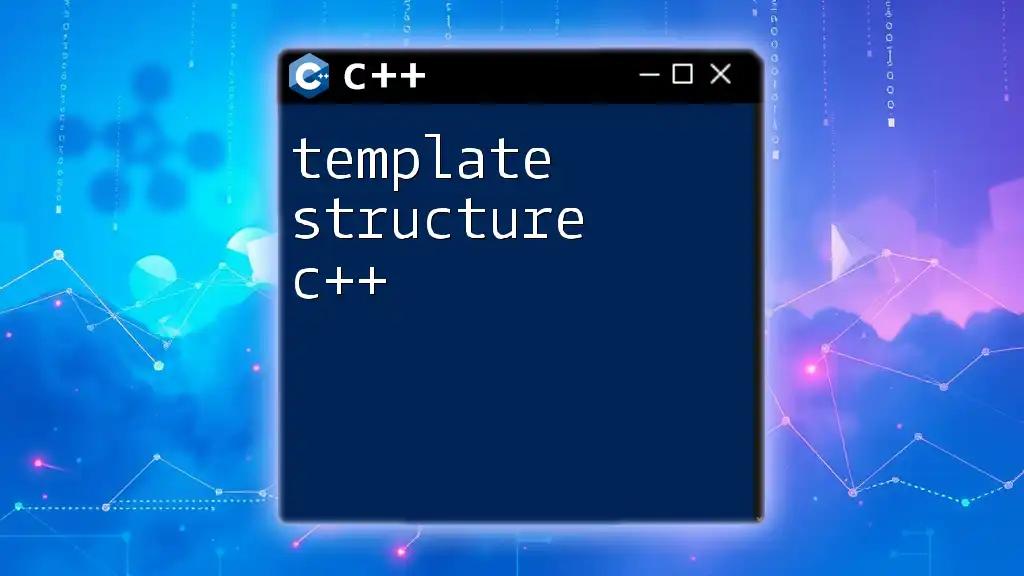
Variadic Templates
Understanding Variadic Templates
Variadic templates are a feature introduced in C++11 that allows a template to accept an arbitrary number of template arguments. They enhance the flexibility of templates, making it feasible to write functions and classes that can operate on multiple arguments.
Code Example
template<typename... Args>
void print(Args... args) {
(std::cout << ... << args) << '\n';
}
Here, the `print` function uses variadic templates to accept any number of arguments. The ellipsis (`...`) syntax allows for unpacking and applying the `<<` operator to all provided arguments, demonstrating a concise implementation for printing multiple values.
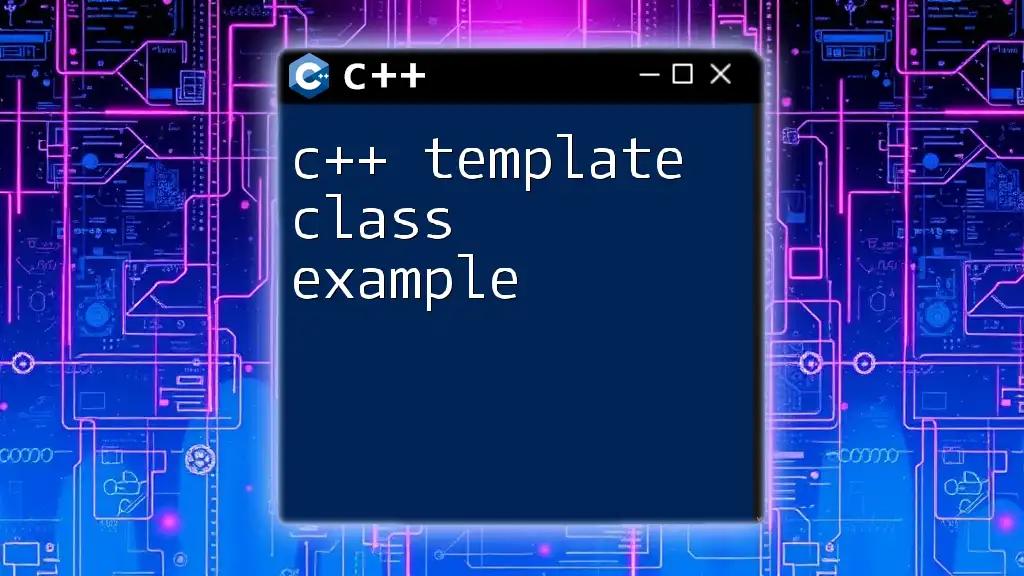
Template Metaprogramming
Introduction to Template Metaprogramming
Template metaprogramming is a technique that leverages templates to perform computations at compile time. This technique can improve performance by eliminating certain run-time calculations and can lead to more optimized code.
Use Cases
Template metaprogramming is beneficial for tasks that require type traits, static assertions, or compile-time calculations, enabling powerful abstractions.
Code Example
template<int N>
struct Factorial {
static const int value = N * Factorial<N - 1>::value;
};
template<>
struct Factorial<0> {
static const int value = 1;
};
This example shows how to compute the factorial of a number at compile time using template metaprogramming. The recursive structure calculates the factorial by defining a base case for `0`.
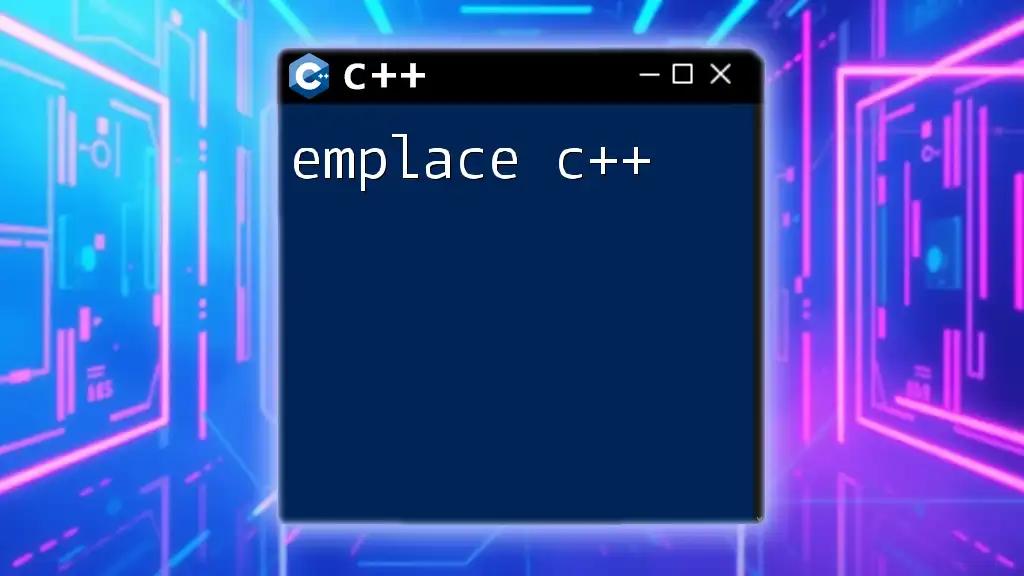
Best Practices for Using C++ Templates
Keep Templates Simple
When designing templates, aim for simplicity. This improves readability and maintainability. Avoid overly complex template constructs that can confuse others (or yourself).
Avoid Code Bloat
While templates offer flexibility, excessive use can lead to code bloat due to multiple instantiations. Be judicious with the use of templates, especially in performance-sensitive areas.
Provide Documentation and Examples
Because templates can be complex and non-intuitive, ensure to document their usage thoroughly. Providing clear examples can help other developers understand how to implement your templates effectively.
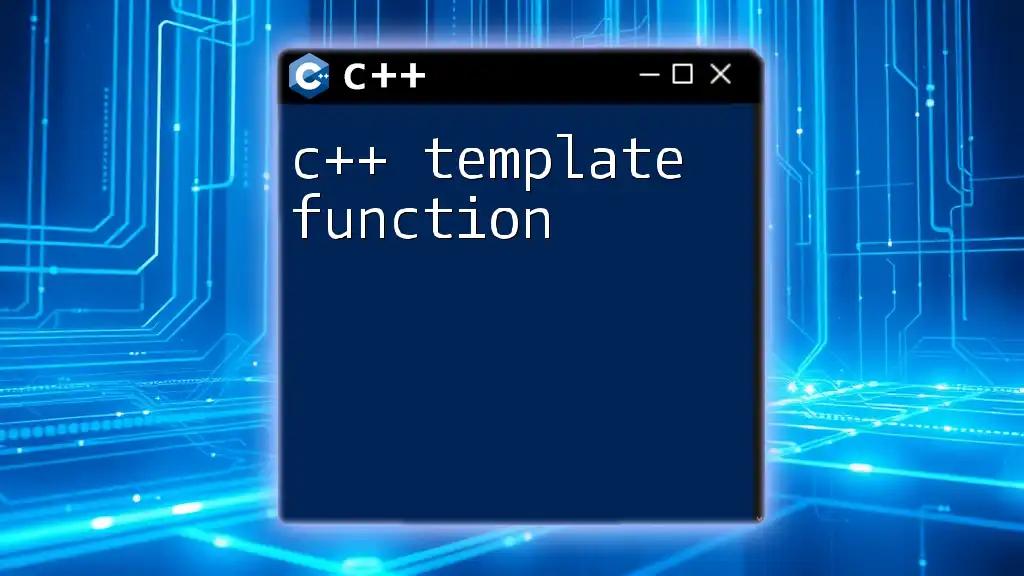
Recap of C++ Templates
In summary, C++ templates are incredibly useful for creating flexible, reusable code. They are essential tools for achieving generic programming while ensuring type safety and optimizing code performance.
Encourage exploration of templates in your own projects and enhance your programming skills by utilizing this powerful feature in C++. The world of template cpp holds many opportunities for cleaner and more efficient coding practices.
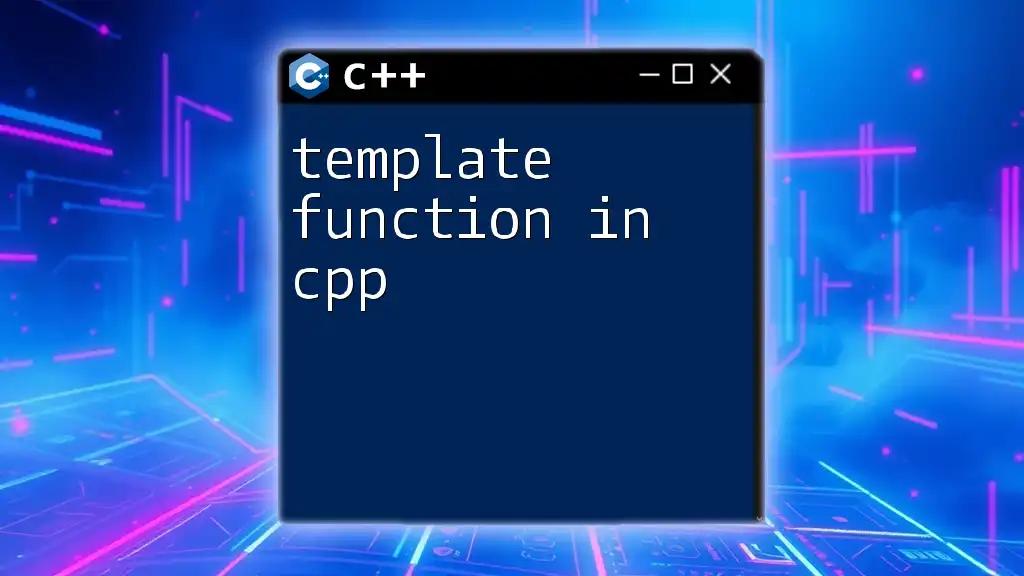
Recommended Books and Online Courses
For further learning on template programming, consider exploring resources that dive deeper into C++ features. Books like "C++ Templates: The Complete Guide" and various online courses can provide additional insights.
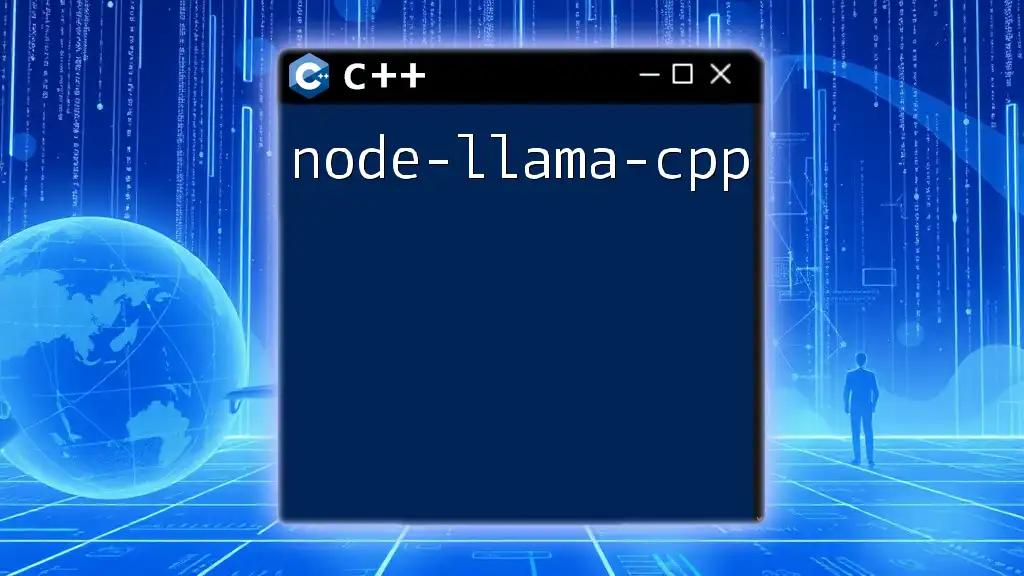
Community and Support
Engagement with online communities such as Stack Overflow and dedicated C++ forums can enhance your learning experience. Don't hesitate to share knowledge and ask for assistance when needed; the C++ community is vast and supportive.