In C++, the `replace` function is used to substitute specified characters or substrings within a string with new ones, as demonstrated in the following code snippet:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str.replace(6, 5, "C++");
std::cout << str << std::endl; // Output: Hello C++!
return 0;
}
Understanding C++ Strings
C++ Strings are a fundamental aspect of the language that allows developers to work with text efficiently. In C++, strings are typically represented using the `std::string` class, which is part of the C++ Standard Library. This class offers a variety of functionalities, including dynamic memory management and built-in functions that simplify string manipulation.
Unlike C-style strings, which are arrays of characters ending with a null character (`'\0'`), `std::string` provides a higher level of abstraction that helps manage strings with ease.
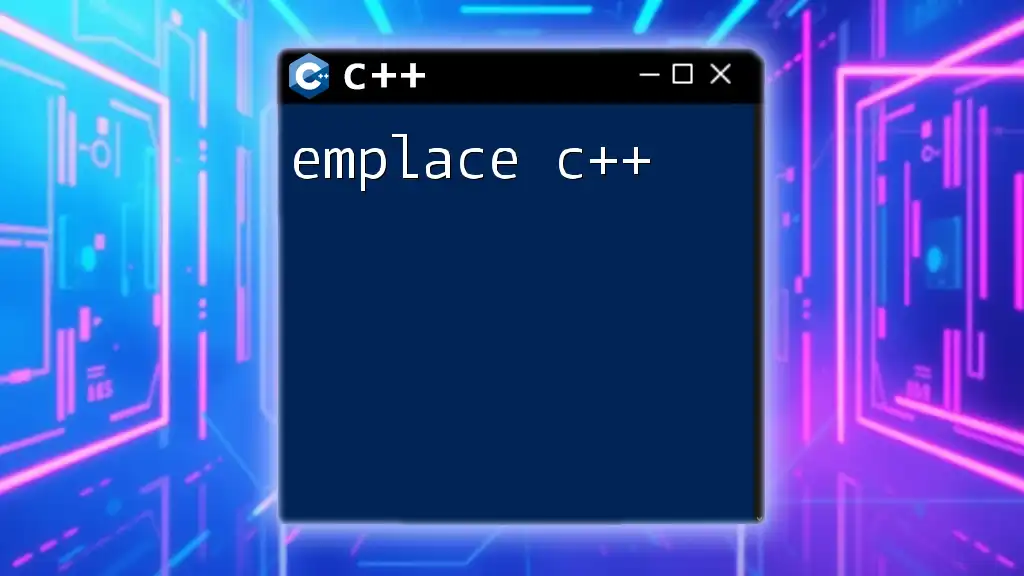
Methods to Replace Strings in C++
One of the primary operations when working with strings is the ability to replace portions of the string content. Here are the main methods for replacing strings in C++.
Using the `std::string::replace()` Method
The `std::string::replace()` method is a straightforward way to replace parts of a string. This method requires three parameters: the starting position of the substring to replace, the length of the substring, and the new substring to insert.
Code Example: Simple String Replacement
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str.replace(6, 5, "C++");
std::cout << str; // Outputs: Hello C++!
return 0;
}
In this example, the call to `str.replace(6, 5, "C++")` begins at index 6, replaces 5 characters (from "World") with "C++", resulting in "Hello C++!". It’s crucial to understand how the indices work to avoid runtime errors.
Using `std::string::find()` and `std::string::erase()` for Advanced Replacement
For more complex scenarios where you may want to replace a substring found within a string, a combination of `std::string::find()`, `std::string::erase()`, and `std::string::insert()` can be very effective.
The `find()` method searches for a substring and returns its starting index, while `erase()` removes the substring, and `insert()` adds the new substring.
Code Example: Finding and Erasing Old Substring
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
std::string to_replace = "World";
std::string replacement = "C++";
size_t position = str.find(to_replace);
if (position != std::string::npos) {
str.erase(position, to_replace.length());
str.insert(position, replacement);
}
std::cout << str; // Outputs: Hello C++!
return 0;
}
In this example, `find()` searches for "World" and, if found, `erase()` removes it while `insert()` adds "C++" in its place. Handling such replacements with care ensures that the right content is modified without altering the surrounding text.
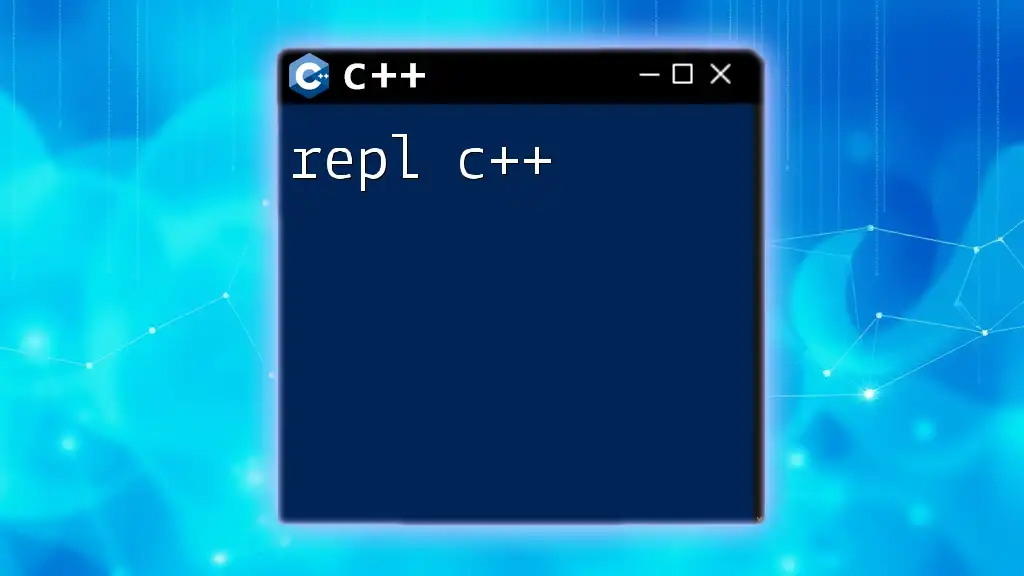
The Power of Regular Expressions
For intricate patterns and multiple occurrences of substrings, utilizing Regular Expressions (regex) can streamline the replacement process significantly. C++ provides the `<regex>` library, which enables pattern matching and replacement capabilities.
Code Example: Regex-based String Replacement
#include <iostream>
#include <string>
#include <regex>
int main() {
std::string str = "Hello 123, Hello 456";
std::regex pattern("Hello \\d+");
std::string replacement = "Hi there";
std::string result = std::regex_replace(str, pattern, replacement);
std::cout << result; // Outputs: Hi there, Hi there
return 0;
}
In this snippet, the regex pattern `Hello \\d+` matches any instance of "Hello" followed by one or more digits. The `std::regex_replace` function replaces all matches in the string, demonstrating the power of regex for bulk replacements. Understanding regex can greatly enhance your string manipulation capabilities in C++.
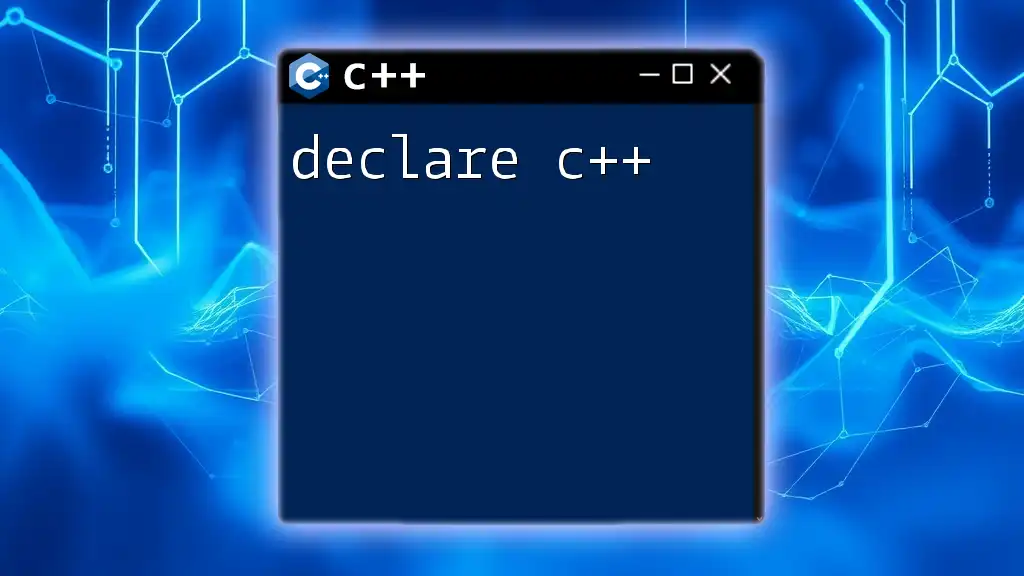
Practical Examples
Developers often face specific use cases where string replacement is crucial. Here are some common scenarios that demonstrate practical application.
Replacing Sensitive Information (Email Masking)
For privacy or user-friendly outputs, you might want to mask sensitive information such as email addresses. This example shows how to replace characters while keeping the structure intact.
Code Example: Email Masking
#include <iostream>
#include <string>
std::string maskEmail(const std::string& email) {
std::string masked = email;
size_t at_pos = masked.find('@');
if (at_pos != std::string::npos) {
masked.replace(1, at_pos - 1, "*");
}
return masked;
}
int main() {
std::string email = "example@gmail.com";
std::cout << maskEmail(email); // Outputs: *xample@gmail.com
return 0;
}
This function replaces all but the first character of the email with an asterisk (*), making it more secure for display without completely losing the context.
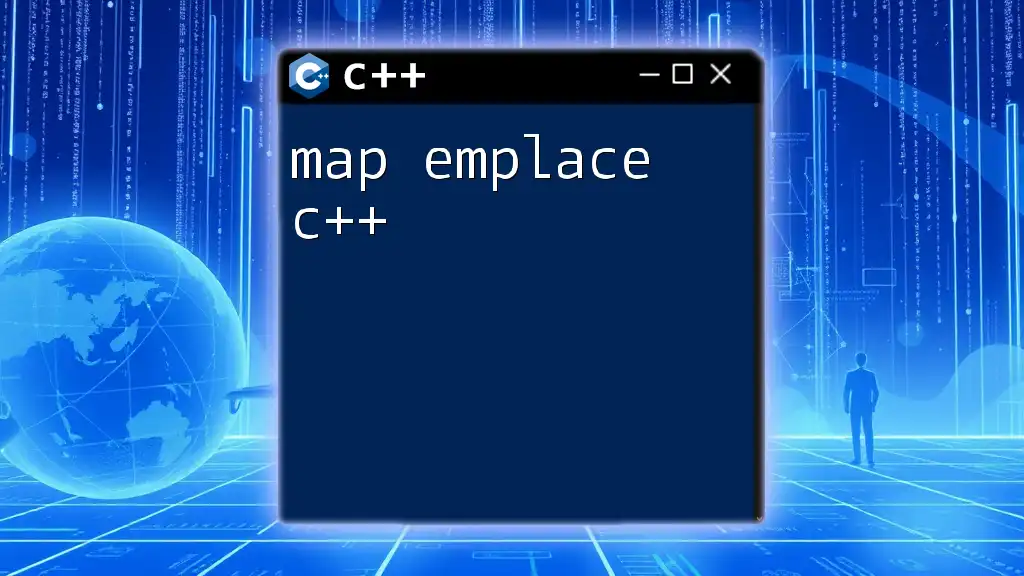
Performance Considerations
When working with string replacement, it is essential to consider the efficiency of the chosen method. For example, `std::string::replace()` is generally more efficient for known indices, while regex operations can be slower due to the complexity of pattern matching.
Careful evaluation of your specific requirements will help you select the best approach. Performance can vary depending on string size and replacement frequency, thus profiling different methods in your context can yield the most optimized solution.
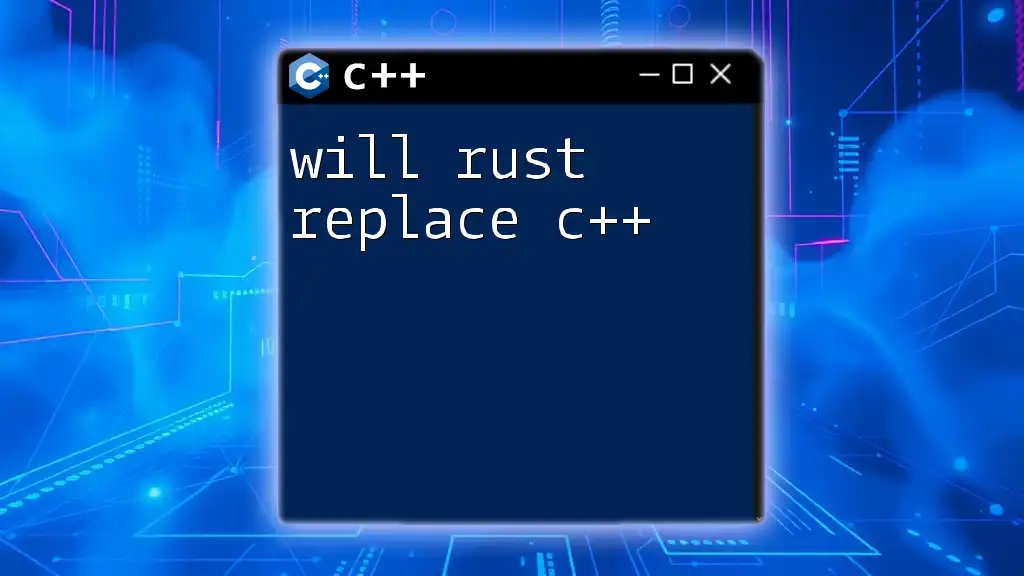
Best Practices for String Replacement in C++
To ensure your code remains robust, follow these best practices when implementing string replacement operations:
- Handle Edge Cases: Always anticipate inputs like null strings or substrings that do not exist. Utilize checks with `std::string::npos` to avoid potential crashes.
- Maintain Code Readability: Well-commented code can save significant time for future maintenance. Clear documentation of what each section does can aid both yourself and other developers.
In summary, utilizing effective string replacement methods in C++ is crucial for manipulating text efficiently. Understanding the different methods and their applications, from `std::string` methods to regex, allows you to adapt to various programming needs. As you learn and implement these concepts, you can greatly enhance your programming proficiency and code quality.