In C++, a predicate is a function or function object that returns a boolean value, often used in algorithms to determine whether a certain condition is met.
Here's a simple code snippet that demonstrates a predicate function:
#include <iostream>
#include <vector>
#include <algorithm>
bool isEven(int number) {
return number % 2 == 0;
}
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
auto it = std::find_if(numbers.begin(), numbers.end(), isEven);
if (it != numbers.end()) {
std::cout << "First even number: " << *it << std::endl;
} else {
std::cout << "No even numbers found." << std::endl;
}
return 0;
}
What is a Predicate?
A predicate in programming refers to a function or an expression that evaluates to a boolean value—either true or false. In C++, predicates play a critical role in the decision-making processes of algorithms, particularly during filtering, searching, and sorting operations. Their primary purpose is to establish a condition that elements must satisfy for algorithms to take specific actions.

The Role of Predicates in C++
In C++, predicates can be expressed through function objects, lambda expressions, or even function pointers. They are heavily utilized within the Standard Template Library (STL) to enable operations that depend on conditional logic. Well-known built-in predicates include `std::greater` and `std::less`, which can be employed for sorting and comparisons. Understanding how to effectively create and use predicates allows for more elegant, efficient, and readable C++ code.

Creating Custom Predicates
Function Objects
Function objects, or functors, are objects that can be treated as though they are functions. To create a custom predicate as a function object, you define a class or struct and overload the `operator()`. This makes it possible to invoke instances of the class as if they were functions.
Here’s a simple example of a function object that checks if a number is even:
struct IsEven {
bool operator()(int n) const {
return n % 2 == 0;
}
};
Lambda Expressions
C++11 introduced lambda expressions, which provide a more concise way to create predicates in line with the code. With lambdas, you can define an unnamed function that can be called immediately. Here is an example of using a lambda expression to check if a number is odd:
auto isOdd = [](int n) { return n % 2 != 0; };
Lambdas are particularly handy for one-off predicates where writing a dedicated function object may feel excessive.
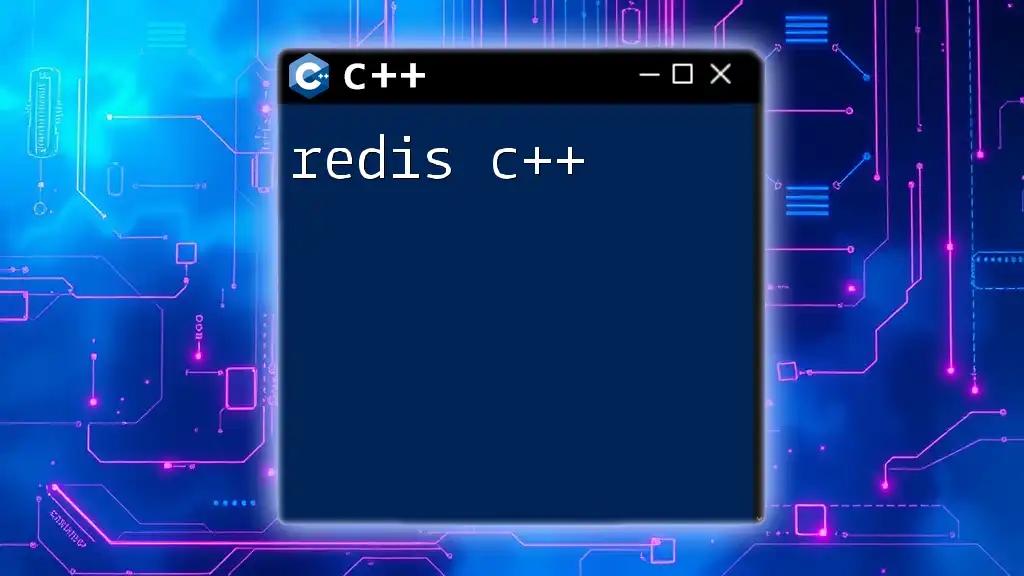
Using Predicates with Standard Algorithms
Filtering Data with `std::remove_if`
One of the powerful applications of predicates in C++ is filtering data using algorithms such as `std::remove_if`. This algorithm removes elements from a container if they satisfy the given predicate.
For example, to remove all even numbers from a vector, you can use the custom predicate `IsEven` like this:
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
numbers.erase(std::remove_if(numbers.begin(), numbers.end(), IsEven()), numbers.end());
This will modify the `numbers` vector to only contain odd numbers, demonstrating the direct application of a predicate in data manipulation.
Sorting with Predicates
Predicates can influence how elements are sorted in a collection. The `std::sort` algorithm accepts a predicate to determine the order of sorting. Here's how to sort a vector in descending order using a lambda expression:
std::sort(numbers.begin(), numbers.end(), [](int a, int b) {
return a > b; // Sort in descending order
});
The flexibility of predicates allows for tailored sorting behavior, enhancing functionality without convoluted logic.
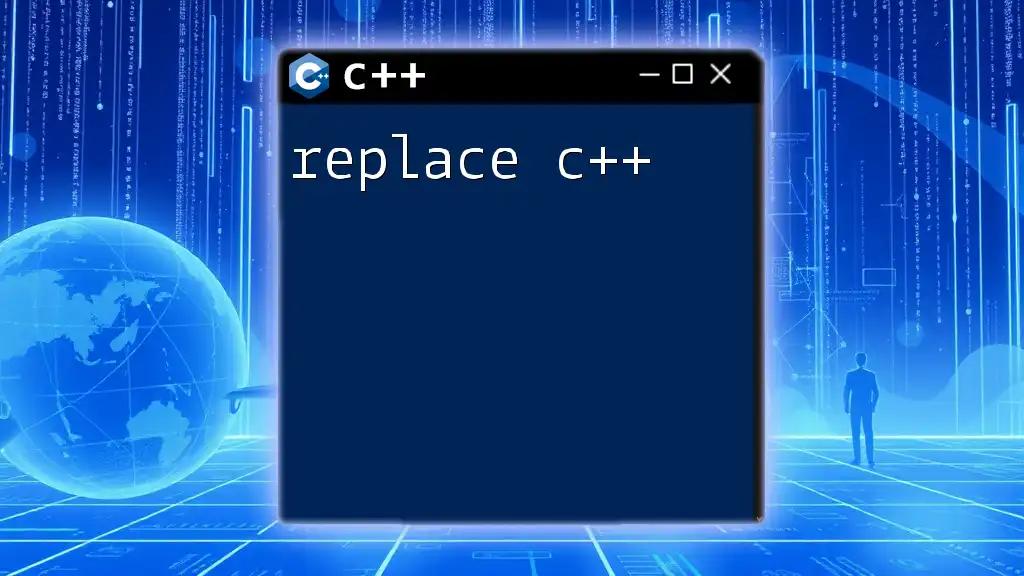
Common Predicate Scenarios
Validating Input
Predicates can be particularly useful in validating user input, ensuring the data meets specific criteria before further processing. For example:
bool isValidInput(const std::string& input, const std::function<bool(char)>& predicate) {
return std::all_of(input.begin(), input.end(), predicate);
}
In this function, you can pass any predicate that checks the validity of characters in a string, improving code modularity and reusability.
Finding Elements
Using predicates, you can easily traverse a collection to find specific elements. The `std::find_if` algorithm utilizes a predicate to locate the first element satisfying a given condition. An example using `IsEven` to find the first even number would look like this:
auto it = std::find_if(numbers.begin(), numbers.end(), IsEven());
if (it != numbers.end()) {
// Found an even number
}
This showcases how predicates are essential in searching operations, allowing you to express complex conditions succinctly.
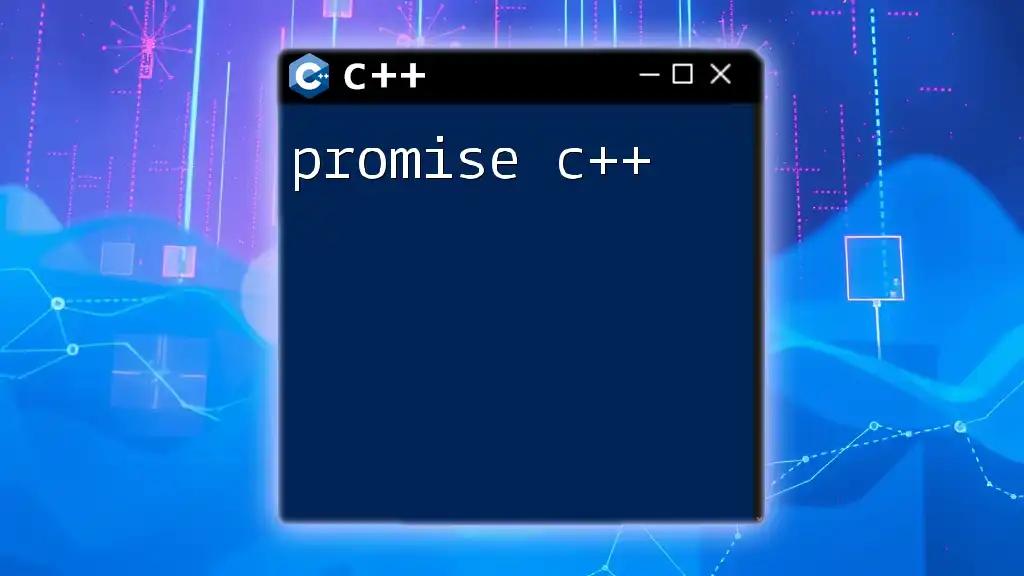
Performance Considerations
Predicate Overhead
It’s important to consider the performance implications of using predicates in your code. While they simplify many tasks, complex predicates can introduce computational overhead, especially in large datasets. Keeping predicates simple and leveraging built-in ones when applicable can mitigate potential performance issues.
Real-World Examples
In real-world applications, predicates can lead to significant performance improvements. For instance, in a data processing system that filters log entries based on specific criteria, using efficient predicates can dramatically reduce the processing time, leading to quicker insights.
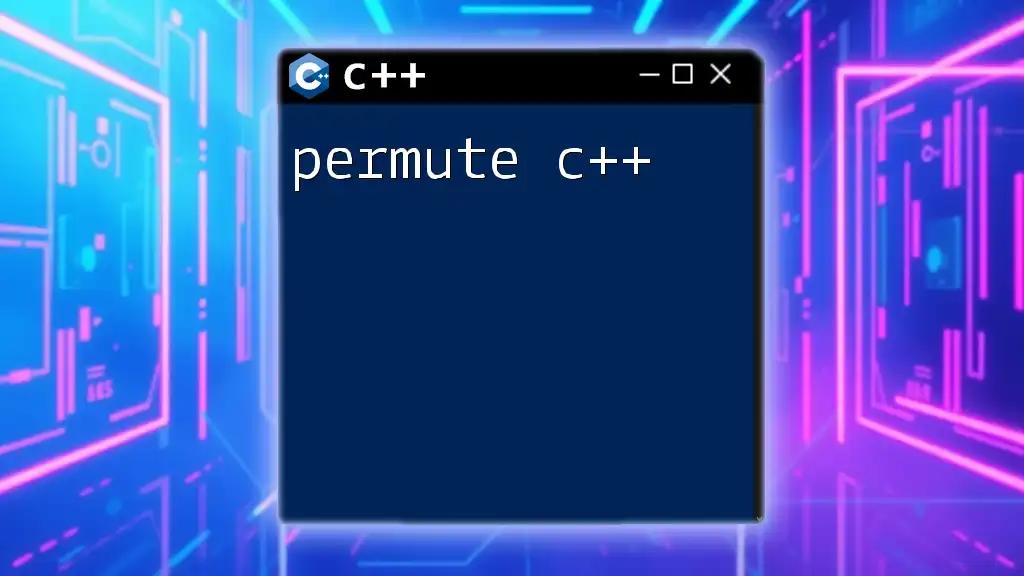
Summary of Predicates in C++
In summary, predicates in C++ are invaluable tools that enhance algorithm functionality and readability. They allow for expressive code that powerfully manipulates data with concise conditions. Whether through custom function objects, lambda expressions, or built-in functions from the STL, the effective use of predicates can significantly improve your programming practices.
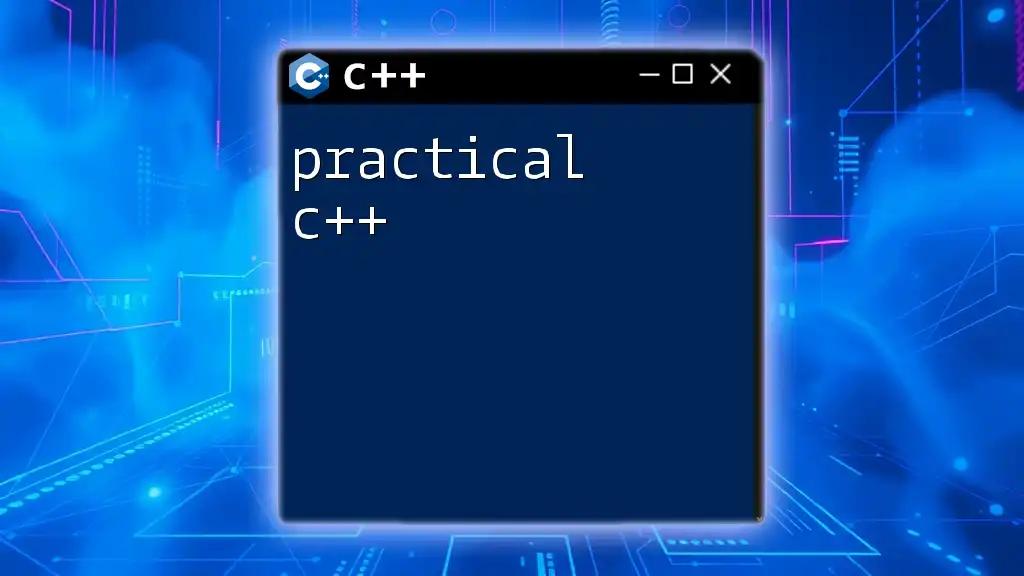
Further Reading and Resources
To deepen your understanding of predicate C++, consider exploring the official C++ documentation, guides on the Standard Template Library, and best practices in functional programming within C++. Engaging with community forums and open-source projects can also provide practical insights into leveraging predicates effectively.
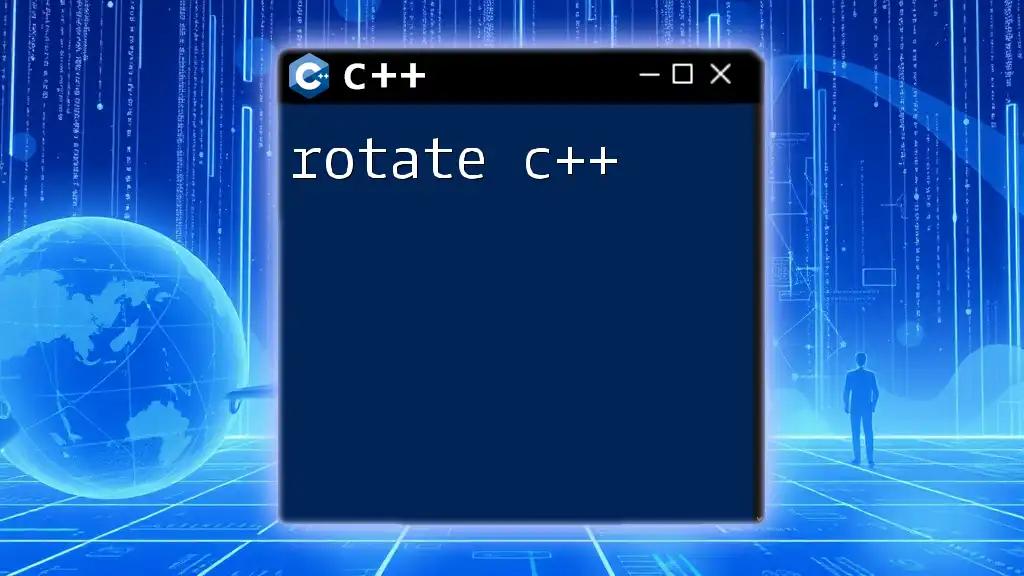
Conclusion
In conclusion, mastering predicates in C++ can lead to more efficient, maintainable, and elegant code. By implementing your own predicates and understanding their applications within STL algorithms, you can harness the full potential of C++ programming, paving the way for optimized applications and powerful data handling.