The `isupper` function in C++ is used to check if a given character is an uppercase letter.
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (isupper(ch)) {
std::cout << ch << " is uppercase." << std::endl;
} else {
std::cout << ch << " is not uppercase." << std::endl;
}
return 0;
}
What is `isupper` in C++?
Definition of `isupper`
The `isupper` function in C++ is part of the `<cctype>` library and is used to check if a given character is an uppercase letter. It is essential for character classification and serves a fundamental role in text processing and validation within C++ programming.
How `isupper` Works
The `isupper` function determines whether the provided character falls within the range of uppercase letters in the ASCII table, specifically from 'A' to 'Z'. The function returns a non-zero value (interpreted as `true`) if the character is uppercase and zero (interpreted as `false`) otherwise. It is effective for string manipulation and validation tasks that require strict adherence to character case rules.

Using `isupper` in C++ Programs
Including Required Headers
To utilize the `isupper` function, you must include the `<cctype>` header file at the beginning of your program. This file contains the declarations for character classification functions.
#include <cctype>
Basic Syntax and Usage
Syntax of `isupper`
The syntax for the `isupper` function is straightforward:
isupper(character)
Example with Explanation
Let’s look at a basic example to understand how `isupper` can be effectively used in a program.
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (isupper(ch)) {
std::cout << ch << " is an uppercase letter." << std::endl;
} else {
std::cout << ch << " is not an uppercase letter." << std::endl;
}
return 0;
}
In this example, we declare a character variable `ch` and assign it the value `'A'`. When we use `isupper(ch)`, the program checks if `ch` is an uppercase letter. Since it is, the output will affirm this by printing, "A is an uppercase letter." If we were to change `ch` to a lowercase letter, such as `'a'`, the output would indicate that it is not uppercase.
Validating Uppercase Characters in Strings
Using `isupper` in a Loop
Often, you’ll want to validate multiple characters, such as those in a string. In this case, using a loop becomes beneficial.
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string str = "Hello World!";
for (char ch : str) {
if (isupper(ch)) {
std::cout << ch << " is uppercase." << std::endl;
}
}
return 0;
}
In this snippet, we iterate through each character in the string `str`. The `isupper` function checks each character, and if it identifies an uppercase letter, it prints that letter along with the affirmation "is uppercase." This is particularly useful in scenarios involving user input or strings fetched from databases, where uppercase letters might carry specific meanings.

Real-World Applications of `isupper`
Input Validation
One common application of the `isupper` function is during input validation. For example, if you’re building a registration system that requires a password to contain at least one uppercase character, you can leverage `isupper` effectively.
#include <iostream>
#include <cctype>
#include <string>
bool isValidPassword(const std::string& password) {
bool hasUpper = false;
for (char ch : password) {
if (isupper(ch)) {
hasUpper = true; // Flag is set
break; // Exit loop early since we found an uppercase letter
}
}
return hasUpper;
}
int main() {
std::string password;
std::cout << "Enter your password: ";
std::cin >> password;
if (isValidPassword(password)) {
std::cout << "Password is valid." << std::endl;
} else {
std::cout << "Password must contain at least one uppercase letter." << std::endl;
}
return 0;
}
In this code, the function `isValidPassword` checks whether an entered password has at least one uppercase letter. If not, it prompts the user for a valid input. This kind of validation can substantially enhance the security of your application.
Formatting Text
Another practical use of `isupper` is in formatting text, where the application may depend on titles or headings needing to be validated for proper casing. For instance, ensuring that the first character of a title is uppercase can maintain consistency in text presentation.
#include <iostream>
#include <cctype>
#include <string>
std::string formatTitle(const std::string& title) {
std::string formattedTitle;
for (char ch : title) {
if (formattedTitle.empty() && islower(ch)) {
formattedTitle += toupper(ch); // Convert the first character to uppercase
} else {
formattedTitle += ch; // Keep the rest unchanged
}
}
return formattedTitle;
}
int main() {
std::string title = "the great gatsby";
std::cout << formatTitle(title) << std::endl; // Outputs: The great gatsby
return 0;
}
In this example, the function `formatTitle` takes a string and converts its first character to uppercase if it is lowercase. This can be useful for ensuring that titles are presented correctly.

Common Mistakes and Troubleshooting
Understanding Character Types
One common pitfall when using `isupper` is not recognizing the importance of the character data type. If you mistakenly pass an integer or a string without handling the character type appropriately, it could lead to unexpected behavior.
#include <iostream>
#include <cctype>
int main() {
int ch = 65; // ASCII value for 'A'
if (isupper(static_cast<char>(ch))) {
std::cout << ch << " corresponds to an uppercase letter." << std::endl;
}
return 0;
}
Here, we demonstrate correct casting of an integer to a character for `isupper`. Ignoring the type requirement will cause compilation errors or unpredictable outcomes.
Misinterpreting the Return Value
Another common issue is misunderstanding the return value of `isupper`. Many developers may expect it to return `true` or `false`, but in C++, it returns a non-zero value for true and zero for false.
It is important to understand:
- Any non-zero return indicates that the character is uppercase.
- A return value of zero indicates that it is not uppercase.
Always use conditional statements to interpret the results correctly.

Conclusion
The `isupper` function is a crucial part of handling character classification in C++. Its ability to swiftly identify uppercase letters enables developers to enhance user input validation, format text correctly, and enforce stringent data processing rules. Understanding and implementing `isuppercase c++` in your projects can lead to robust, user-friendly applications.
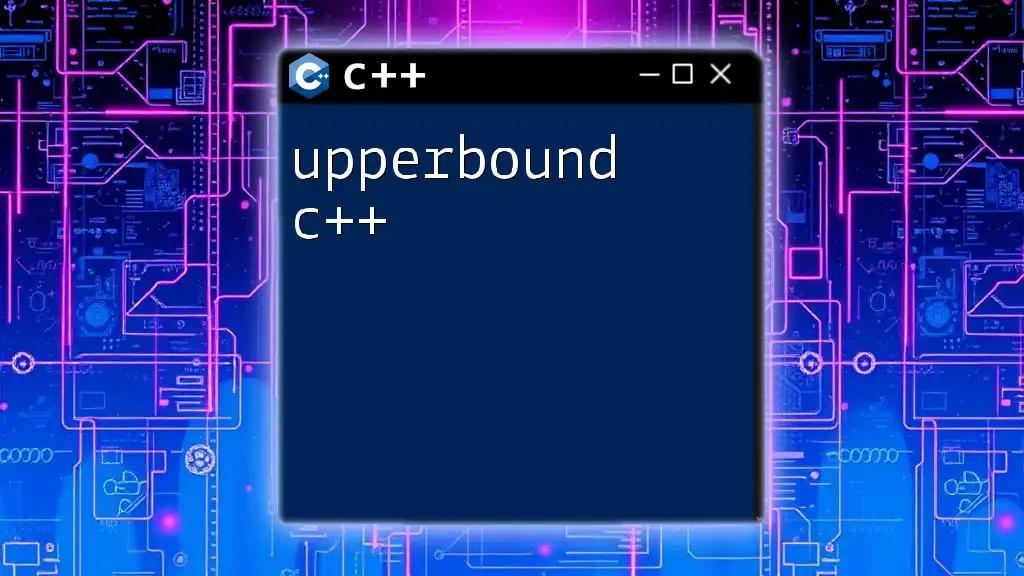
Further Reading and Resources
For those interested in deepening their knowledge of C++, especially character functions, the following resources are valuable:
- The official C++ Standard Library documentation on `<cctype>`
- C++ programming textbooks focusing on standard library utilities and best practices
- Online C++ coding platforms to practice using character classification functions in realistic scenarios