The `ispunct` function in C++ checks if a given character is a punctuation character, returning a non-zero value if true and zero otherwise.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cctype>
int main() {
char ch = '!';
if (ispunct(ch)) {
std::cout << ch << " is a punctuation character." << std::endl;
} else {
std::cout << ch << " is not a punctuation character." << std::endl;
}
return 0;
}
What is `ispunct`?
`ispunct` is a function included in the C++ Standard Library that checks whether a given character is a punctuation character. Understanding this function is crucial for developers who deal with text processing, as punctuation can significantly affect the interpretation of data. Often, tasks such as input validation, text parsing, and string manipulation necessitate a discerning eye for punctuation.
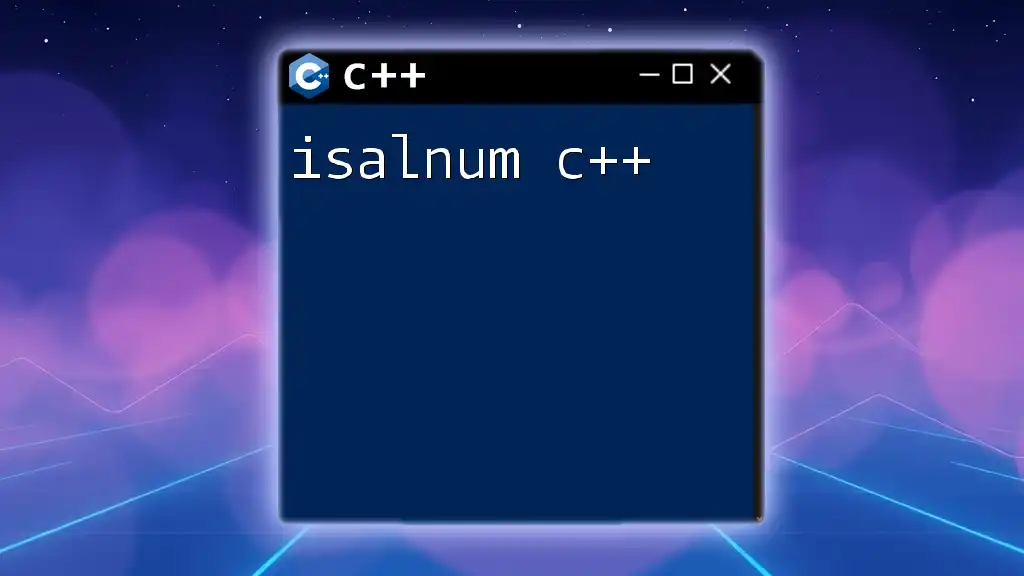
Why Use `ispunct`?
Using `ispunct` can be particularly advantageous in various scenarios, such as:
- Input Validation: Ensuring user-provided data meets required standards (e.g., usernames that should not contain punctuation).
- Text Parsing: Analyzing and modifying strings by identifying and separating punctuation during processing.
- Data Cleaning: Filtering out unnecessary punctuation from data sets can improve data quality before analysis.
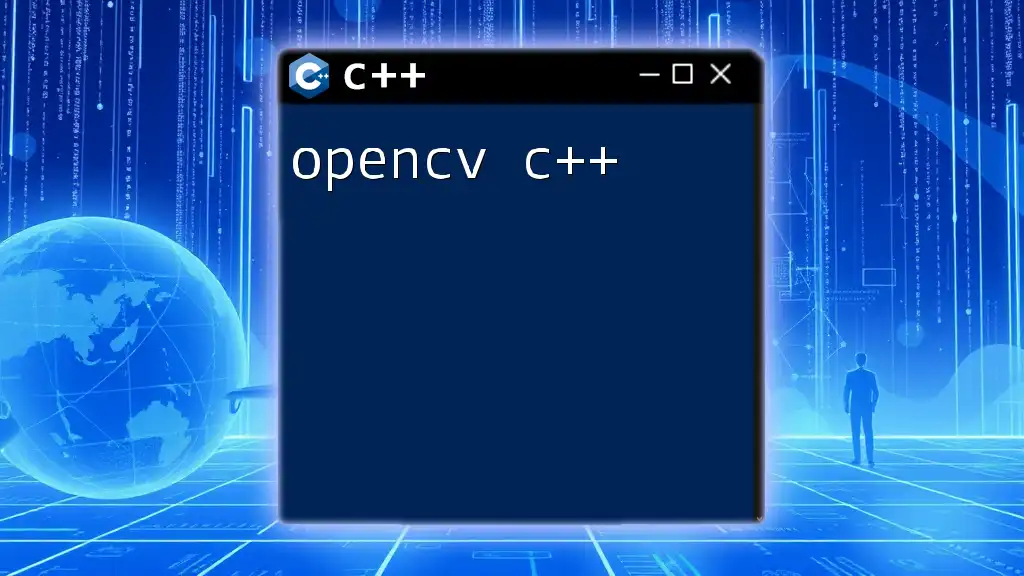
Understanding Character Classification
What are Character Classifications?
Character classification refers to the categorization of characters based on certain traits, such as whether they are letters, digits, whitespace, or punctuation marks. This classification aids in implementing logic that requires an understanding of the content of strings.
Overview of C++ Character Libraries
C++ provides a rich set of functionalities for character classification through the `<cctype>` library. Besides `ispunct`, it includes functions like `isalnum`, `isalpha`, `isdigit`, and many more, allowing developers to make robust and clear decisions based on character types.
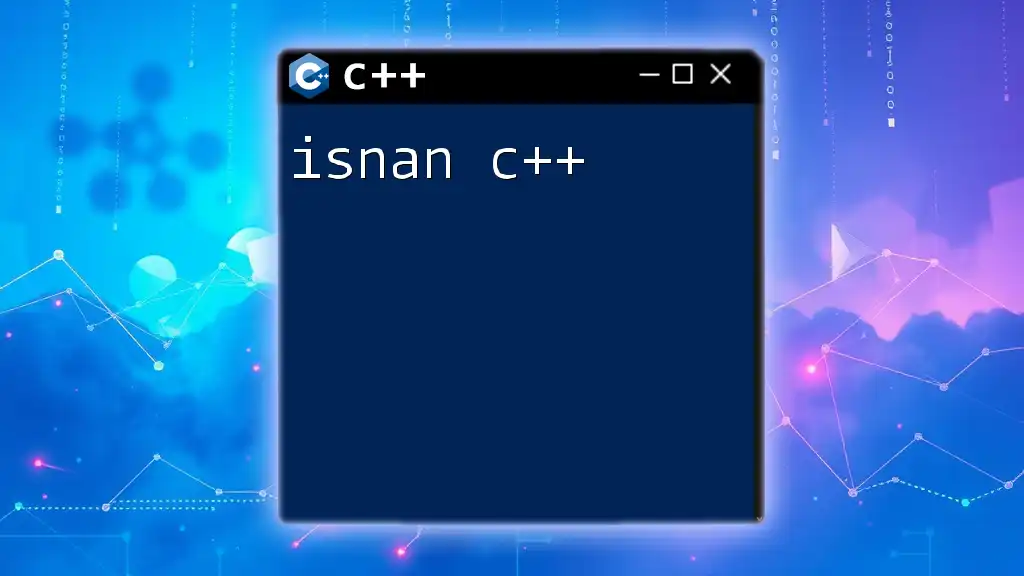
Using `ispunct` Function
Syntax of `ispunct`
The syntax of the `ispunct` function is straightforward:
#include <cctype>
bool ispunct(int ch);
- The parameter `ch` represents any character (as an integer), and the function returns a `bool` indicating whether the character is classified as punctuation.
How `ispunct` Works
The `ispunct` function detects whether a character is punctuation based on a predefined set of punctuation characters defined in the C++ standard. Common punctuation characters include `!`, `"`, `#`, `$`, `%`, `&`, `'`, `(`, `)`, `*`, `+`, `,`, `-`, `.`, `/`, `:`, `;`, `<`, `=`, `>`, `?`, `@`, `[`, `\`, `]`, `^`, `_`, `{`, `|`, `}`, and `~`.
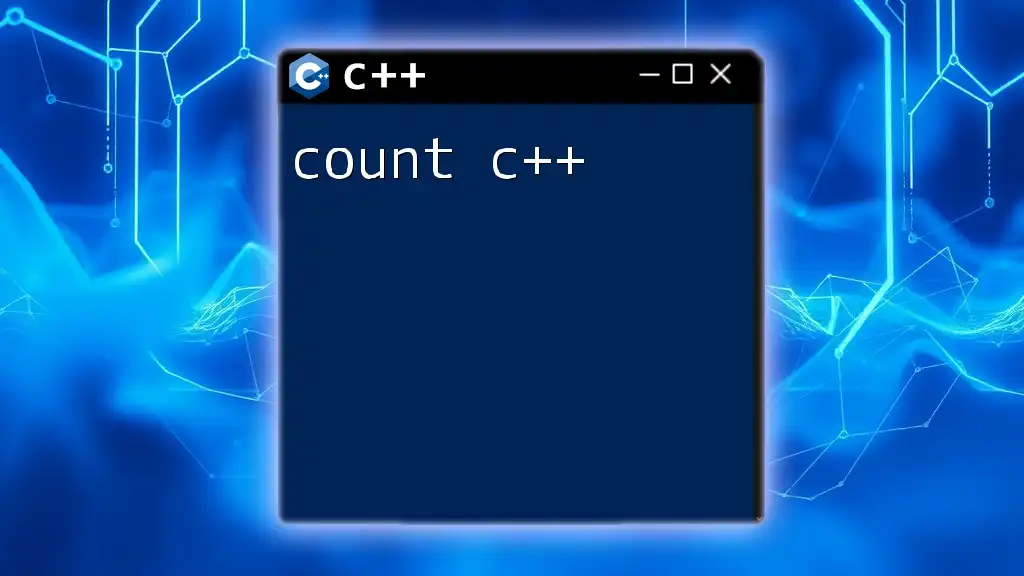
Examples of Using `ispunct`
Basic Example
Let’s look at a simple illustration of how to use the `ispunct` function to determine if a given character is punctuation:
#include <iostream>
#include <cctype>
int main() {
char testChar = ';';
if (ispunct(testChar)) {
std::cout << testChar << " is a punctuation character." << std::endl;
} else {
std::cout << testChar << " is not a punctuation character." << std::endl;
}
return 0;
}
In this example, the program checks if `testChar` is a punctuation character. The output will indicate that `;` is indeed a punctuation character.
Looping Through a String
Checking Each Character
You can also use `ispunct` in conjunction with loops to process entire strings. Here’s a demonstration:
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string text = "Hello, World!";
for (char ch : text) {
if (ispunct(ch)) {
std::cout << ch << " is a punctuation character." << std::endl;
}
}
return 0;
}
In this example, the program iterates through each character in the string `text`, checking if it's a punctuation character. It will output the punctuation characters found, which in this case is a comma `,` and an exclamation mark `!`.
Real-World Applications
Sanitizing User Input
A practical application of the `ispunct` function involves input validation for usernames. Here’s how you can implement it:
#include <iostream>
#include <string>
#include <cctype>
bool isValidUsername(const std::string& username) {
for (char ch : username) {
if (ispunct(ch)) {
return false; // Invalid if any punctuation is found
}
}
return true; // Valid otherwise
}
int main() {
std::string username;
std::cout << "Enter a username: ";
std::cin >> username;
if (isValidUsername(username)) {
std::cout << "Username is valid!" << std::endl;
} else {
std::cout << "Username must not contain punctuation!" << std::endl;
}
return 0;
}
In this code snippet, the `isValidUsername` function checks if the username contains any punctuation. If it does, the function returns `false`, guiding users to select valid usernames.
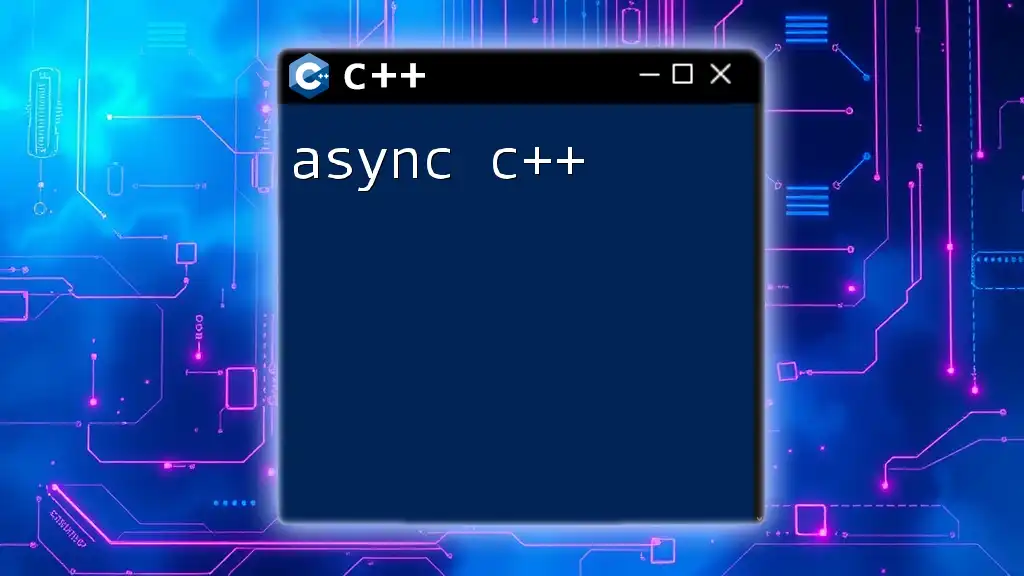
Performance Considerations
Efficiency of `ispunct`
Using `ispunct` is generally efficient and has a constant runtime complexity of \( O(1) \), as it simply checks if the character is in a fixed set of punctuation marks. However, when processing large strings or datasets, perform extensive testing to ensure performance does not degrade.
Alternatives to `ispunct`
There are alternative functions like `isalpha`, `isdigit`, or `isspace`, which serve different character classification roles. Evaluating the specific requirements of your task will help you select the appropriate function.
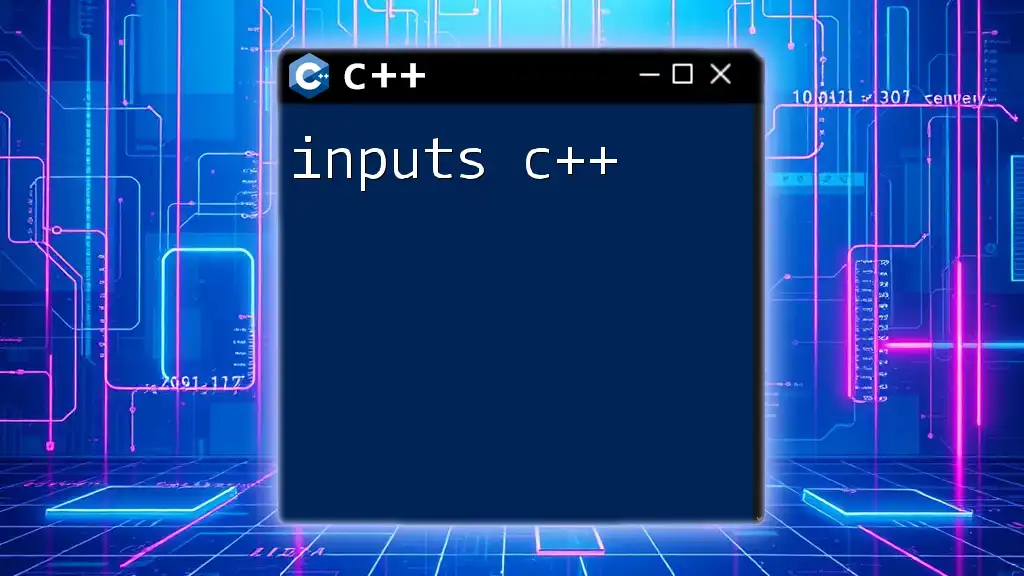
Common Pitfalls
Misunderstanding Function Behavior
It’s important to note that `ispunct` may not recognize all punctuation marks, especially if dealing with characters outside the ASCII range or multilingual text. This can lead to unintended results in applications that process international characters.
Case Sensitivity and Type Conversion
Be mindful of the character types and cases. The `ispunct` function works on integer inputs, where care should be taken to ensure characters are correctly passed.
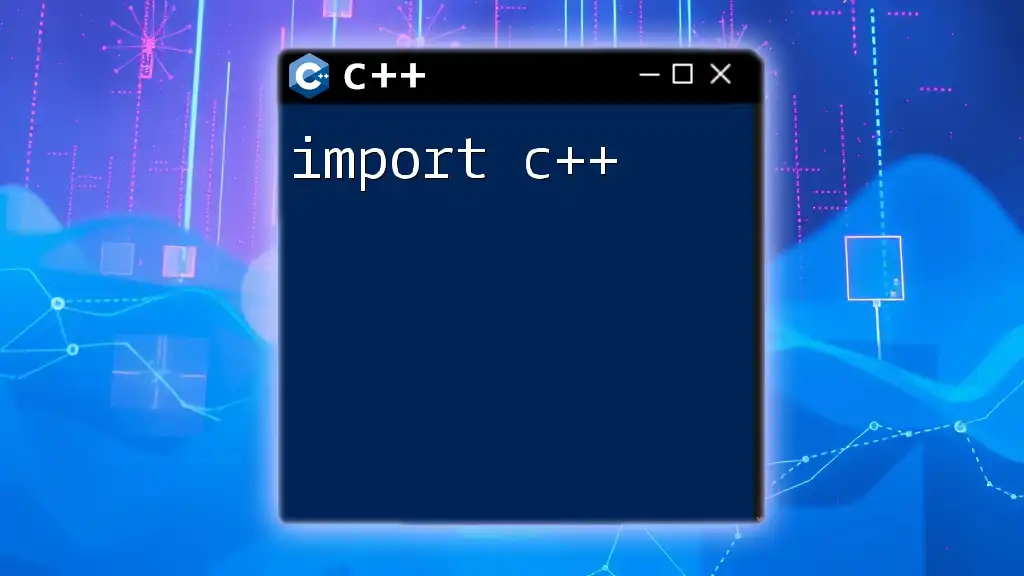
Conclusion
In summary, understanding `ispunct c++` and its applications is essential for any programmer working with text data. It provides an efficient way to classify punctuation and can significantly improve code robustness in text handling tasks. By experimenting with `ispunct`, you can enhance your C++ programming skills and develop sophisticated text processing applications.