In C++, a `sorted_list` can be implemented using the `std::list` combined with the `std::sort` algorithm to maintain order upon insertion.
Here's a simple example demonstrating how to create and insert into a sorted list:
#include <iostream>
#include <list>
#include <algorithm>
void insertSorted(std::list<int>& lst, int value) {
lst.insert(std::upper_bound(lst.begin(), lst.end(), value), value);
}
int main() {
std::list<int> sortedList;
insertSorted(sortedList, 5);
insertSorted(sortedList, 1);
insertSorted(sortedList, 3);
for (int num : sortedList) {
std::cout << num << " ";
}
return 0;
}
Understanding the Basics of Sorted Lists in C++
What is a Sorted List?
A sorted list is a collection of elements that are arranged in a specific order, usually ascending or descending based on their natural ordering (for example, numbers or characters). In contrast, an unsorted list has no defined ordering, which can complicate tasks such as searching and retrieval. The primary use cases for sorted lists in C++ programming include:
- Efficient searching: Searching through sorted data can be performed using binary search algorithms, significantly reducing time complexity compared to linear search methods.
- Data retrieval: Quickly accessing sorted elements is invaluable in various applications, such as databases and algorithms.
Characteristics of a Sorted List
The main characteristic of a sorted list is that its elements are stored in a predefined order. Key benefits of using sorted lists include:
- Faster searching: Due to their ordered nature, searching can be performed more efficiently, with time complexity reduced to O(log n) using binary search.
- Compact data presentation: Sorted data is often easier to understand and analyze, making it particularly useful when presenting data in reports or visualizations.
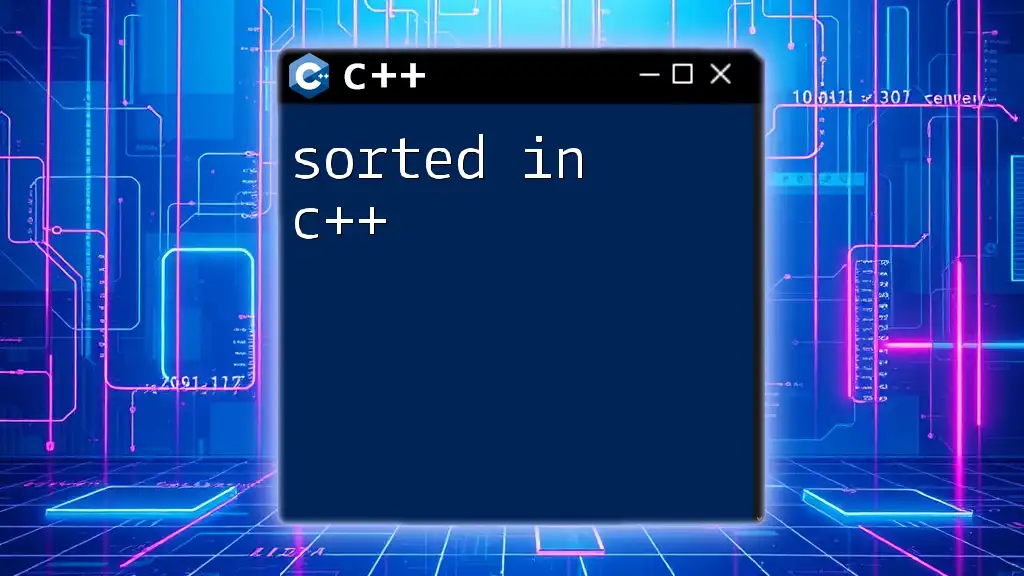
Implementing a Sorted List in C++
Choosing the Right Data Structure
When implementing a sorted list in C++, the choice of data structure is crucial. The two common options are:
- Arrays: Fixed-size collections that can be sorted and allows easy access to elements. However, inserting and deleting elements can be complex and inefficient as it typically requires shifting elements.
- Linked Lists: Dynamic collections that allow more efficient insertion and deletion, though searching for a specific element can be more challenging.
Using the `std::list` Container from the Standard Template Library (STL)
C++ provides a built-in container called `std::list` which is an ideal starting point for a sorted list implementation.
Creating a Sorted List Using `std::list`
To work with a sorted list using `std::list`, you can insert elements and then sort them. Here's an example:
#include <iostream>
#include <list>
#include <algorithm>
int main() {
std::list<int> sortedList;
// Inserting elements
sortedList.push_back(3);
sortedList.push_back(1);
sortedList.push_back(2);
sortedList.sort(); // sorting the list
// Display the sorted list
for (const auto& item : sortedList) {
std::cout << item << " ";
}
return 0;
}
In this code snippet, we first declare a `std::list` called `sortedList`. We insert three integers, and then call the `sort()` method to arrange them in ascending order. This is a quick and straightforward way to utilize the C++ Standard Library for sorted data.
Custom Sorted List Implementation
For more control over the behavior of sorted lists, you may want to implement your own class. This approach allows you to define custom insertion logic, searching mechanisms, and data handling.
Creating Your Own Sorted List Class
Below is a basic structure for a `SortedList` class:
template <typename T>
class SortedList {
private:
std::vector<T> elements; // Use vector as underlying container
public:
void insert(const T& value);
void display() const;
};
This implementation uses a `std::vector` as the underlying container for the sorted elements.
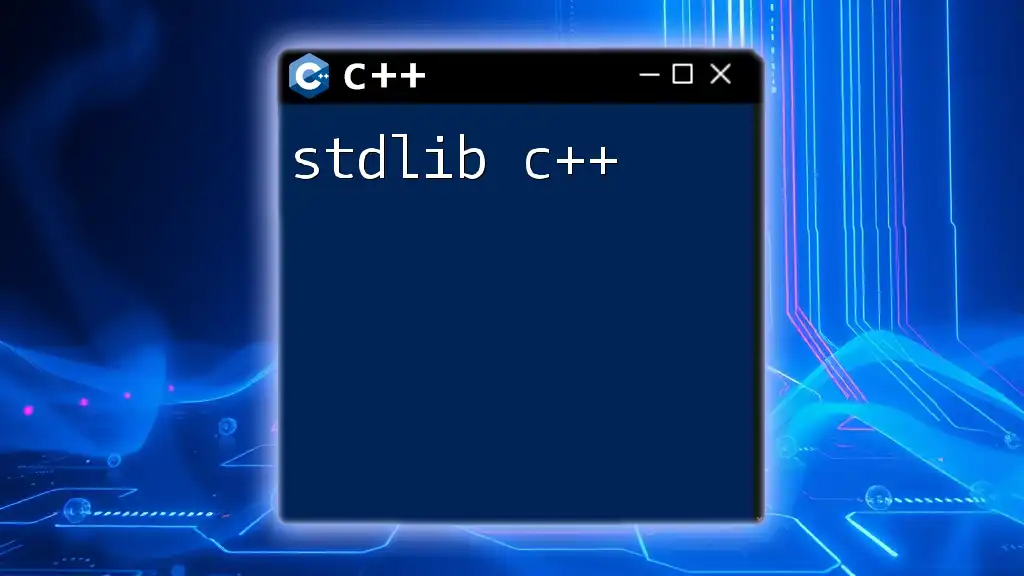
Inserting Elements in a Sorted List
Strategies for Insertion
When adding new elements to a sorted list, maintaining the order is essential. You can achieve this using:
- Linear search: Not recommended when efficiency is a concern, particularly with large datasets.
- Binary search: This approach is more efficient as it reduces the search space quickly.
Example Insertion Method Implementation
Here's an example of an insertion method that maintains the sorted order:
template <typename T>
void SortedList<T>::insert(const T& value) {
// Using binary search to find the correct position
auto it = std::lower_bound(elements.begin(), elements.end(), value);
elements.insert(it, value); // Insert element in sorted position
}
This code attempts to find the appropriate insertion point for a new element using `std::lower_bound()` and then inserts the value without disrupting the sorted order.
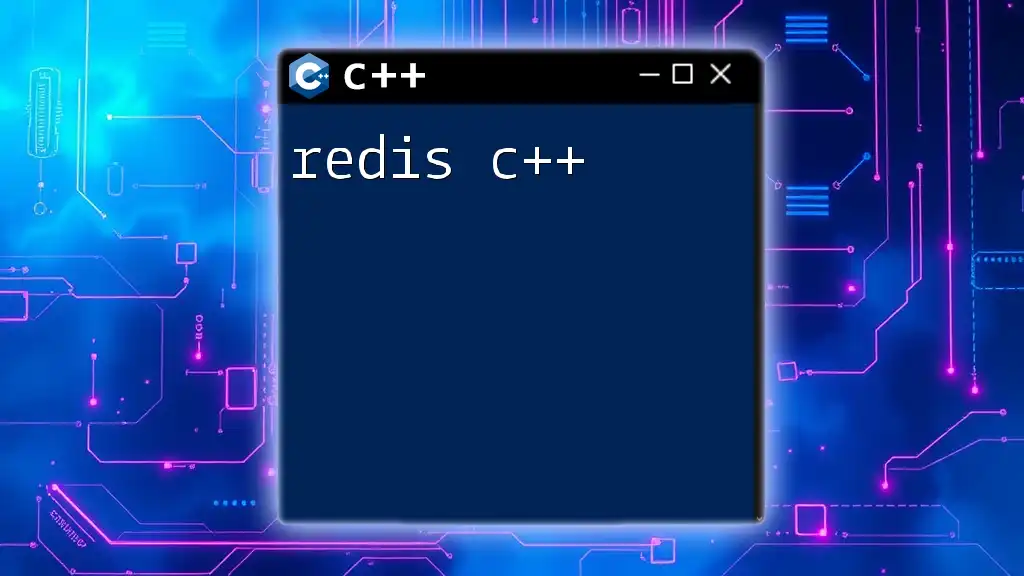
Searching in a Sorted List
Benefits of Searching in a Sorted List
Searching through sorted lists can be performed using binary search algorithms, which vastly improve performance. The time complexity of a binary search is O(log n), significantly faster than the O(n) time complexity found in linear searches.
Implementation of Search Methods
Here's how you can implement a binary search method in your `SortedList` class:
template <typename T>
bool SortedList<T>::find(const T& value) const {
return std::binary_search(elements.begin(), elements.end(), value);
}
This method utilizes `std::binary_search()` to determine whether the specified value exists in the sorted list.
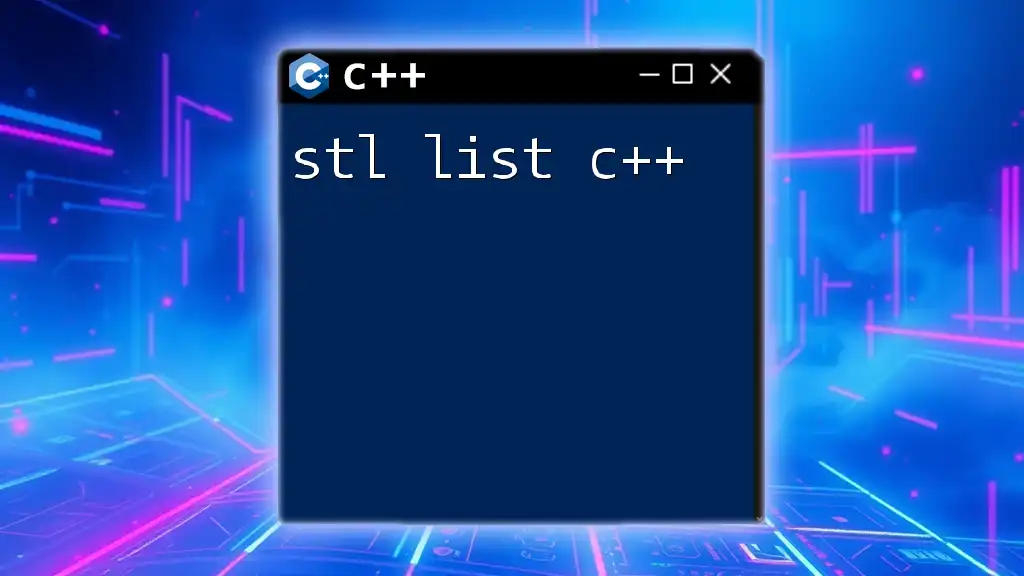
Removing Elements from a Sorted List
Strategies for Deletion
Removing elements from a sorted list can be more complex than inserting, as it involves both finding the element and ensuring that the ordering remains intact.
Example Deletion Method
Here's a simple deletion method for the `SortedList` class:
template <typename T>
void SortedList<T>::remove(const T& value) {
auto it = std::find(elements.begin(), elements.end(), value);
if (it != elements.end()) {
elements.erase(it); // Erase element if found
}
}
In this code, we first look for the specified value using `std::find()`. If the value exists, we erase it from the list. This operation maintains the list's integrity.
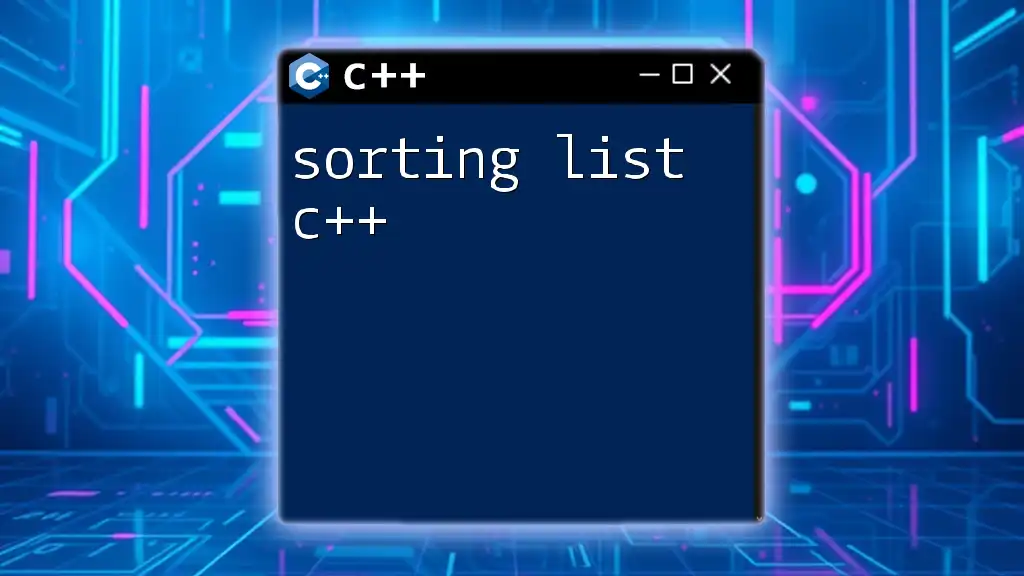
Handling Edge Cases in Sorted Lists
Empty Lists
When performing operations on empty lists, ensure that your methods can handle such cases gracefully. This might mean returning early or providing informative feedback to the user.
Duplicate Values
Managing duplicates in a sorted list can be challenging. Depending on your requirements, you might enforce unique elements or allow duplicates while ensuring the list remains sorted.
Performance Considerations
It's crucial to analyze the time and space complexity of each operation within sorted lists to optimize performance. For instance, the search operation remains efficient with O(log n), whereas insertion and deletion could vary between O(n) and O(log n), depending on implementation style.
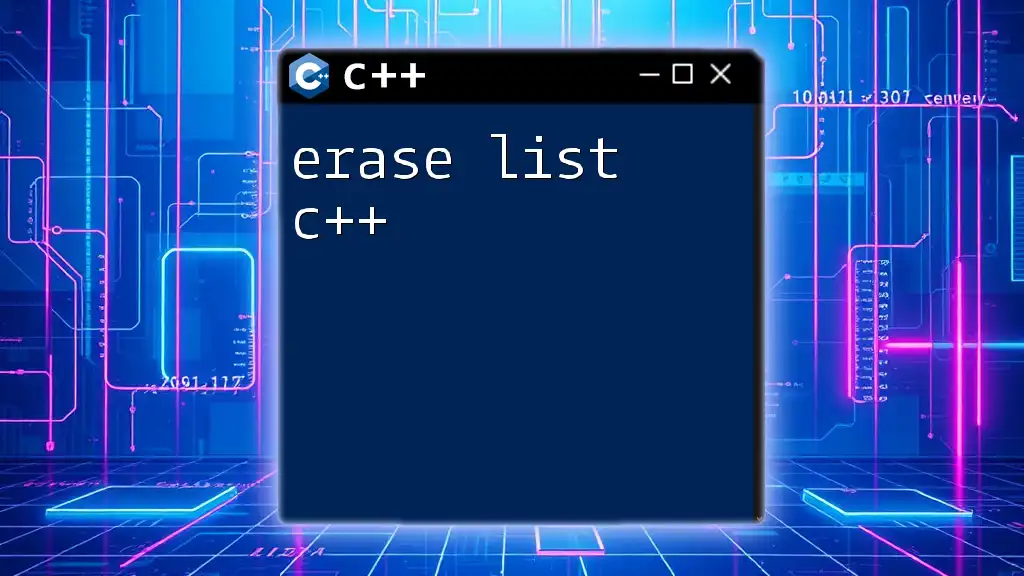
Conclusion
In summary, sorted lists in C++ are powerful tools that facilitate efficient searching, data retrieval, and organization. By understanding their implementation, characteristics, and the various underlying data structures, you can leverage sorted lists to enhance the performance and clarity of your C++ applications. Take the time to practice these concepts to solidify your understanding and mastery of sorted lists in your programming journey.
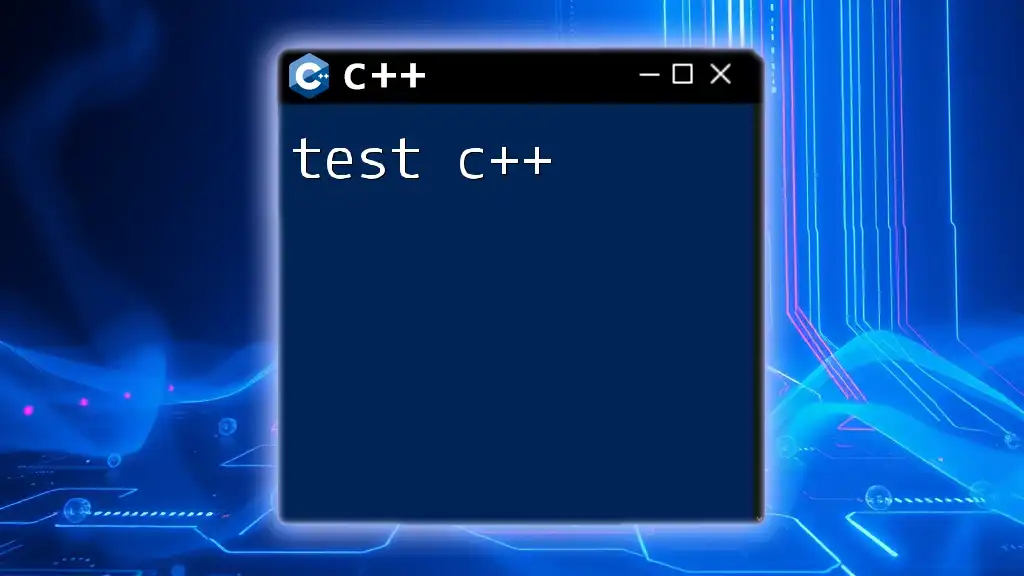
Additional Resources
For those looking to delve deeper into C++ and sorted lists, consider exploring recommended textbooks, online tutorials, and documentation related to the C++ Standard Template Library (STL) and data structures. Happy coding!