"Testing in C++ involves utilizing various methods to ensure that your code behaves as expected, often employing assertions to verify conditions at runtime."
Here’s a simple code snippet that demonstrates how to use assertions for testing in C++:
#include <cassert>
#include <iostream>
int add(int a, int b) {
return a + b;
}
int main() {
assert(add(2, 3) == 5); // This should pass
assert(add(2, 2) == 5); // This will fail
std::cout << "All tests passed!" << std::endl;
return 0;
}
What is Testing in C++?
Testing in C++ refers to the process of executing a program to identify any errors, gaps, or missing requirements in the code. It plays a critical role in software development by ensuring that the software behaves as expected and meets the specified requirements. Testing helps developers catch issues early in the development process, ultimately leading to higher-quality and more reliable code.
While testing might seem daunting at first, especially in a language as nuanced as C++, understanding the different types of tests and how to properly implement them can significantly enhance the development workflow.
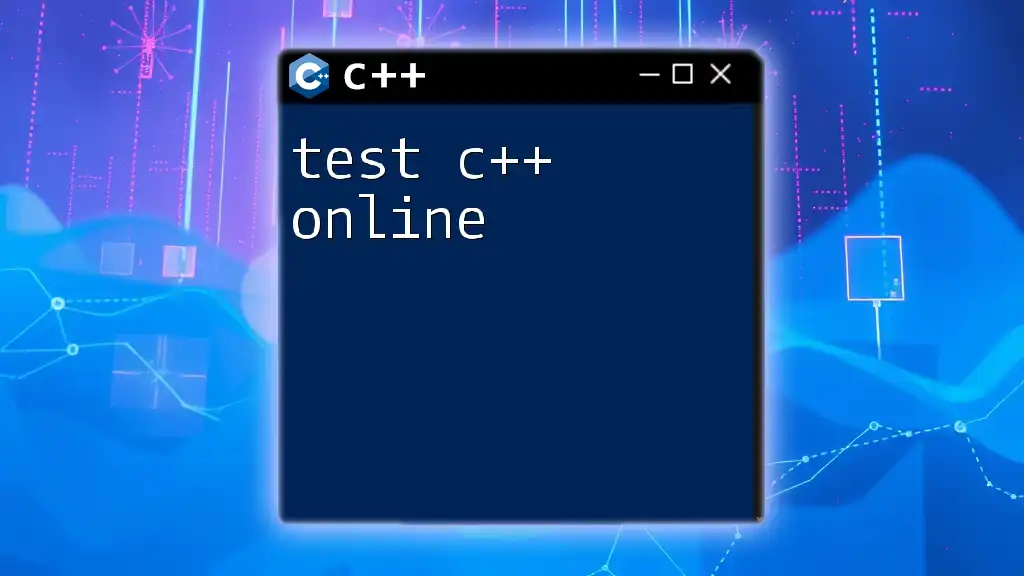
Types of Testing
Unit Testing
Unit testing involves testing individual components of the code (or "units") in isolation. It is an essential practice in C++ development, as it helps ensure that each part of the code behaves as expected. By focusing on the smallest parts of the application, unit testing makes it easier to catch errors before they propagate through the system.
Example of Unit Test in C++ using Google Test:
To begin unit testing, you often use frameworks such as Google Test. Here’s a simple example:
#include <gtest/gtest.h>
int Add(int a, int b) {
return a + b;
}
TEST(AdditionTest, HandlesPositiveInput) {
EXPECT_EQ(Add(1, 2), 3);
EXPECT_EQ(Add(0, 5), 5);
}
In this example, the `Add` function is tested to ensure it returns the correct sums for the specified inputs. This guarantees that any changes or refactorings to the `Add` function can be validated quickly.
Integration Testing
Integration testing is the next step after unit testing, where unit components are combined to test their interactions. This type of testing checks if the interfaces between units work correctly.
The importance of integration testing cannot be overstated; it reveals issues that may not be apparent when testing units in isolation.
For instance, if two components rely on one another, they might function independently but fail when integrated. Here’s a brief code structure for integration tests:
#include <gtest/gtest.h>
// Assume Database and UserAuthenticator are two components being tested
TEST(UserAuthIntegrationTest, AuthenticatesValidUser) {
Database db;
UserAuthenticator auth(&db);
db.AddUser("username", "password");
EXPECT_TRUE(auth.Authenticate("username", "password"));
}
System Testing
System testing is the evaluation of the complete and fully integrated software product. In C++, system testing often involves testing the entire application’s functionality.
This form of testing verifies that the system meets the specified requirements in real-world scenarios, including performance and user experience.
A detailed test case for system testing might involve:
- Simulating a user login process and verifying each step.
- Testing valid and invalid inputs, and checking that the system responds appropriately.
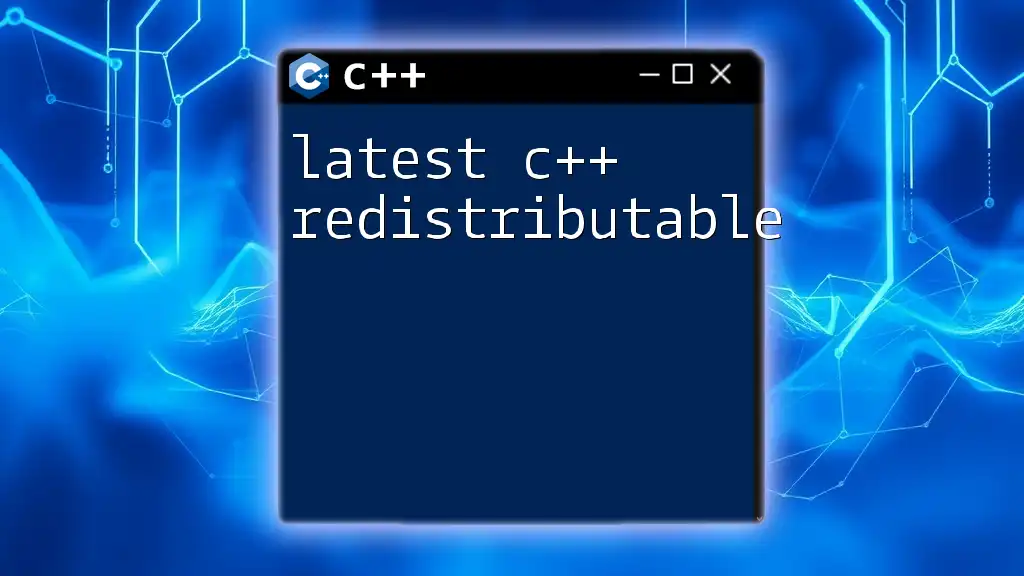
Setting Up a Testing Environment
Choosing the Right Testing Framework
Selecting the appropriate testing framework is paramount to efficient testing practices in C++. Common frameworks include:
- Google Test: Offers advanced features and is widely used in the C++ community.
- Catch2: Known for its ease of use and requires minimal setup.
- Boost Test: A part of the Boost libraries, it provides a rich set of features.
When choosing a framework, consider factors such as project requirements, community support, and ease of integration into your existing development workflow.
Installation and Configuration
Installing Google Test can be accomplished in a few straightforward steps. Here's how you can set it up in your project:
-
Create a build directory:
mkdir build cd build
-
Run CMake to configure the build:
cmake ..
-
Compile the library:
make
Best Practices for Directory Structure
A well-organized directory structure is fundamental for maintaining clarity in your testing code. A commonly used layout might be:
/your_project
/src
/include
/tests
/unit
/integration
/system
This structure allows for clear navigation and easy management of test files, facilitating enhanced collaboration in larger teams.
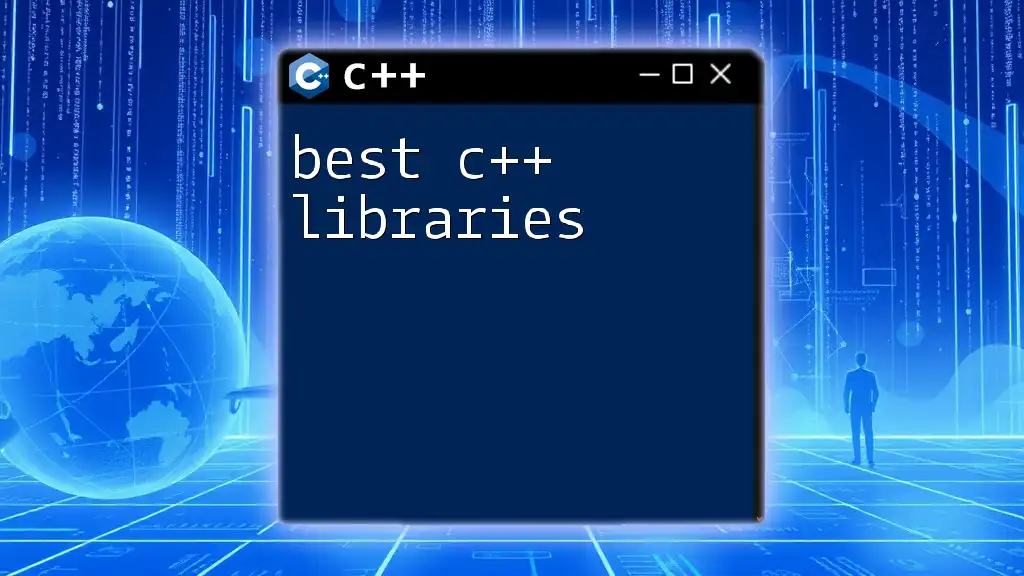
Writing Effective Test Cases
Structuring Your Test Cases
Utilizing a structured approach to writing test cases can significantly improve their readability and maintainability. A recommended format is the given/when/then pattern:
- Given a specific scenario.
- When an action is taken.
- Then the expected result occurs.
Consider the following structured test case:
void TestAddFunction() {
// Given
int x = 1;
int y = 2;
// When
int result = Add(x, y);
// Then
ASSERT_EQ(result, 3);
}
This structure makes it clear what the initial conditions are, what action was taken, and what the expected outcome is, ensuring that tests remain understandable over time.
Common Pitfalls in Test-Coding
There are several common mistakes developers often make while writing test cases:
- Testing Implementation Details: Focus on input/output without being tied to the internal workings of a function.
- Overly Complex Tests: Keep your tests simple and focused. Each test should verify a single behavior.
- Lack of Test Coverage: Ensure that all functionalities, both positive and negative paths, are covered adequately.
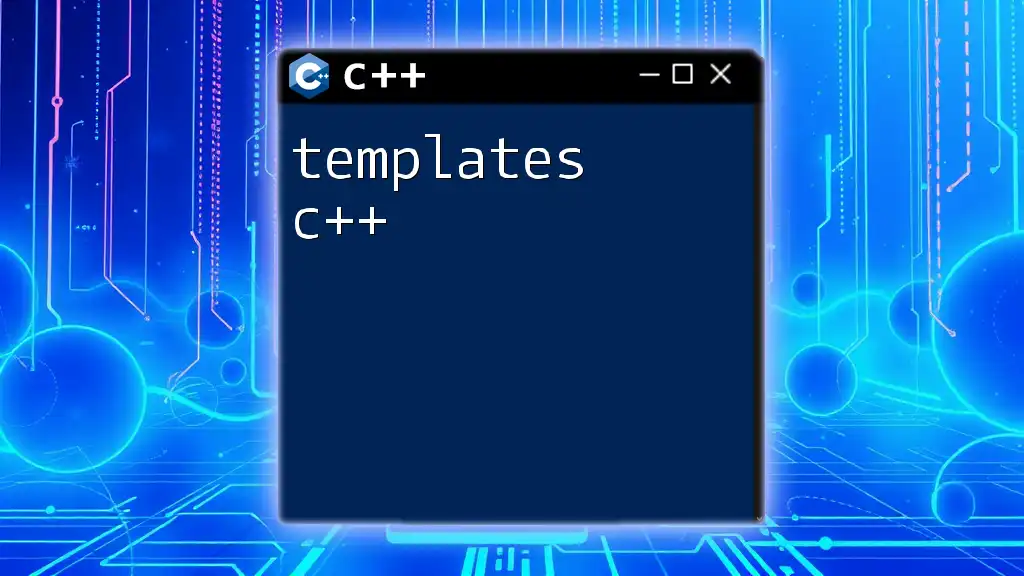
Running Tests
Command Line Basics
Once your tests are written, you can execute them via the command line. For Google Test, simply compile your test files into an executable and run it:
./your_test_executable
This straightforward command will run all the defined test cases and provide output indicating which tests passed and which failed.
Continuous Integration (CI)
Integrating testing into a CI pipeline is vital for maintaining code quality across the development lifecycle. CI tools like Travis CI and GitHub Actions can automate the execution of tests whenever code changes are made, ensuring that any issues are caught early.
For example, a basic configuration for running tests in GitHub Actions might look like this:
name: C++ CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run Tests
run: |
mkdir build
cd build
cmake ..
make
./your_test_executable
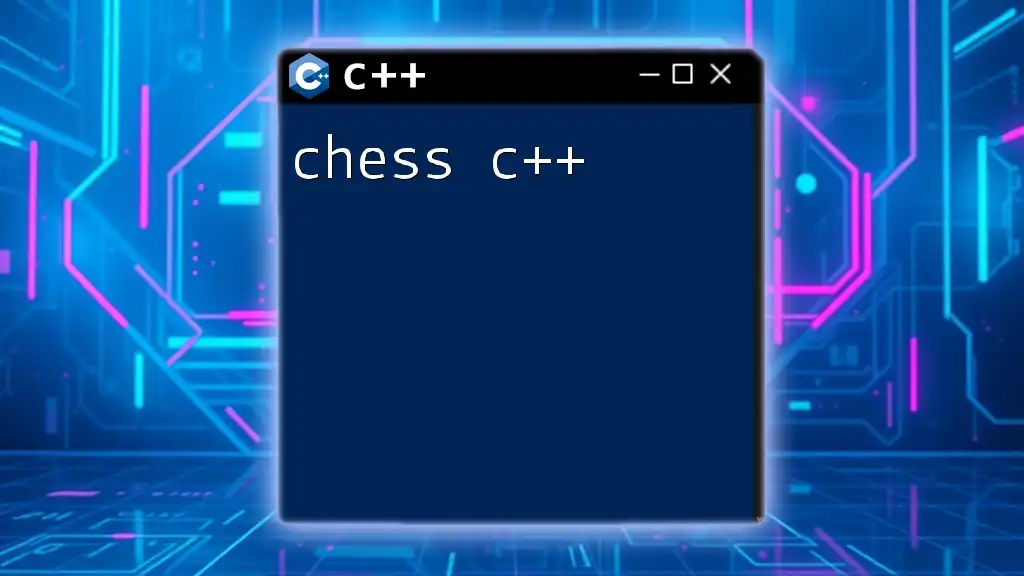
Debugging Failed Tests
Identifying Test Failures
When a test fails, diagnosing the underlying reason can be challenging yet necessary. Using debugging tools such as gdb allows for a detailed examination of the program at runtime.
You can start by running:
gdb ./your_test_executable
This provides an interactive environment to analyze test failures.
Correcting Test Failures
Strategies to fix failed tests should focus on identifying the problem source. This may involve reviewing code changes leading to the failure, adjusting test conditions, or enhancing code robustness. Using logs to track test execution can also aid in understanding points of failure.
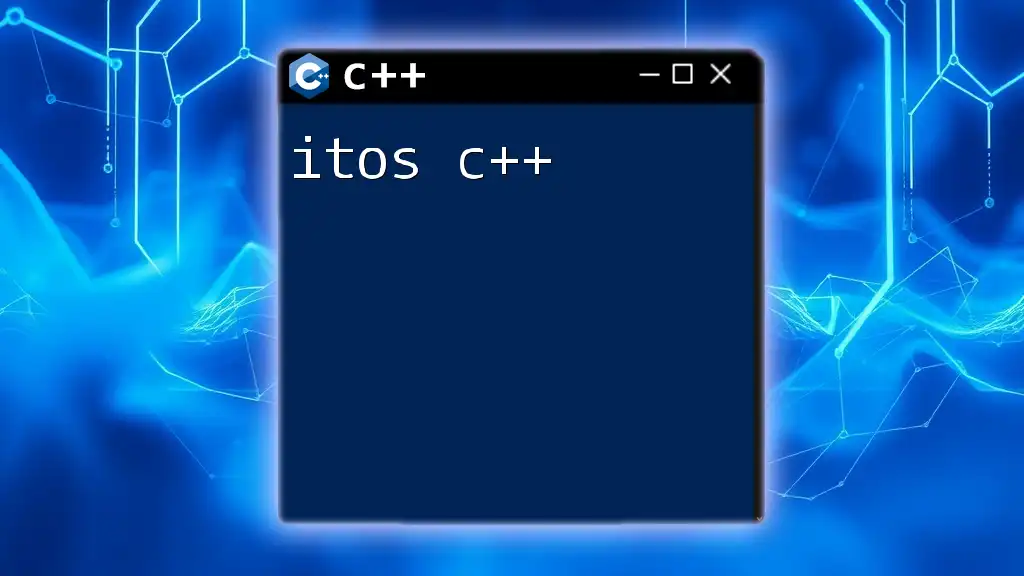
Conclusion
Testing is an integral part of the software development lifecycle in C++. Mastering the different types of testing, setting up a proper testing environment, and writing effective test cases are crucial steps toward ensuring the quality and reliability of your code. By integrating tests early and often, you enhance your development process and contribute to delivering robust software solutions.
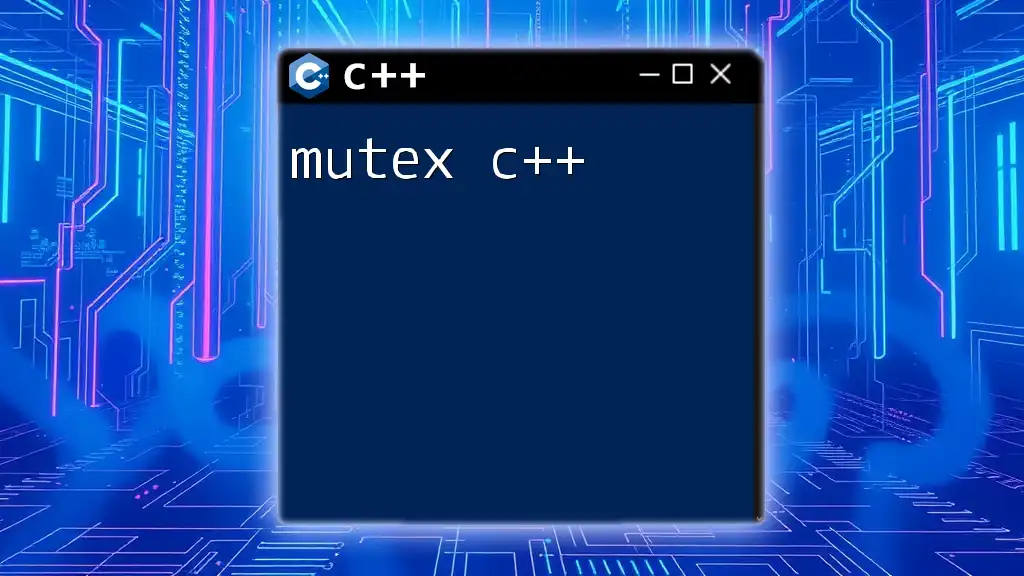
Additional Resources
To further enhance your understanding of test C++ practices, consider exploring the following resources:
- Books on C++ programming and testing methodologies.
- Online courses that focus specifically on testing techniques.
- Community forums and articles where you can ask questions and share experiences.