In this post, we'll explore how to implement a simple command-line chess game in C++ to demonstrate the usage of CPP commands effectively.
Here's a basic example of initiating a chess board:
#include <iostream>
#include <vector>
void printBoard(const std::vector<std::vector<char>>& board) {
for (const auto& row : board) {
for (char square : row) {
std::cout << square << ' ';
}
std::cout << std::endl;
}
}
int main() {
std::vector<std::vector<char>> chessBoard(8, std::vector<char>(8, '-'));
printBoard(chessBoard);
return 0;
}
Introduction to C++ and Chess Game Development
C++ has earned a prominent place in the realm of game development due to its exceptional performance, extensive control over system resources, and rich set of libraries. As a powerful language, it allows developers to build responsive and efficient applications, including games.
Importance of C++ in Game Development
Performance is crucial in gaming; C++ provides remarkable speed that can make a significant difference during gameplay. The language allows for precise memory management, which is essential in large-scale projects. These features contribute to its popularity among developers working on high-performance games.
Overview of Chess as a Game
Chess is a strategic board game with a rich history, known for its deep tactics and complex positioning. The game involves two players competing to checkmate each other’s king, utilizing distinct pieces with unique movements. Understanding the basic rules of chess is necessary for creating an effective chess game in C++.

Getting Started with a C++ Chess Game
Setting Up Your Development Environment
To develop a chess game in C++, you need an appropriate development environment.
IDE Options: Popular choices include Visual Studio, Code::Blocks, and CLion. Each IDE offers unique features that can enhance productivity and ease of use.
Compiler Setup: Choose a C++ compiler like GCC or MSVC. Installing and configuring your compiler ensures your code is properly compiled and executed.
Basic Structure of a C++ Chess Game
Understanding the Game Loop
The game loop is a fundamental component of any game. It handles the flow of the game by continuously checking for player inputs, updating game states, and rendering the game elements.
while (gameRunning) {
handleInput();
updateGameState();
render();
}
In this loop, `handleInput()` captures player commands, `updateGameState()` updates the game conditions, and `render()` redraws the chess board and pieces.

Designing the Chess Board and Pieces
Representing the Chess Board
Using 2D Arrays in C++
A chess board can be visualized as an 8x8 grid. In C++, a 2D array can effectively represent this board:
char chessBoard[8][8] = {
{'r', 'n', 'b', 'q', 'k', 'b', 'n', 'r'},
{'p', 'p', 'p', 'p', 'p', 'p', 'p', 'p'},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{'P', 'P', 'P', 'P', 'P', 'P', 'P', 'P'},
{'R', 'N', 'B', 'Q', 'K', 'B', 'N', 'R'}
};
Here, lower-case letters represent black pieces while upper-case letters denote white pieces.
Creating Classes for Chess Pieces
Object-Oriented Design Principles
Using C++'s object-oriented features, you can model each chess piece with a class. For instance, a base `Piece` class can define common properties and methods for all pieces:
class Piece {
public:
char type;
bool isWhite;
virtual bool isValidMove(int startX, int startY, int endX, int endY) = 0; // Pure virtual method
};
You can then derive specific classes for each piece, implementing their movement rules:
class Rook : public Piece {
public:
bool isValidMove(int startX, int startY, int endX, int endY) override {
return (startX == endX || startY == endY); // Rook moves in straight lines.
}
};
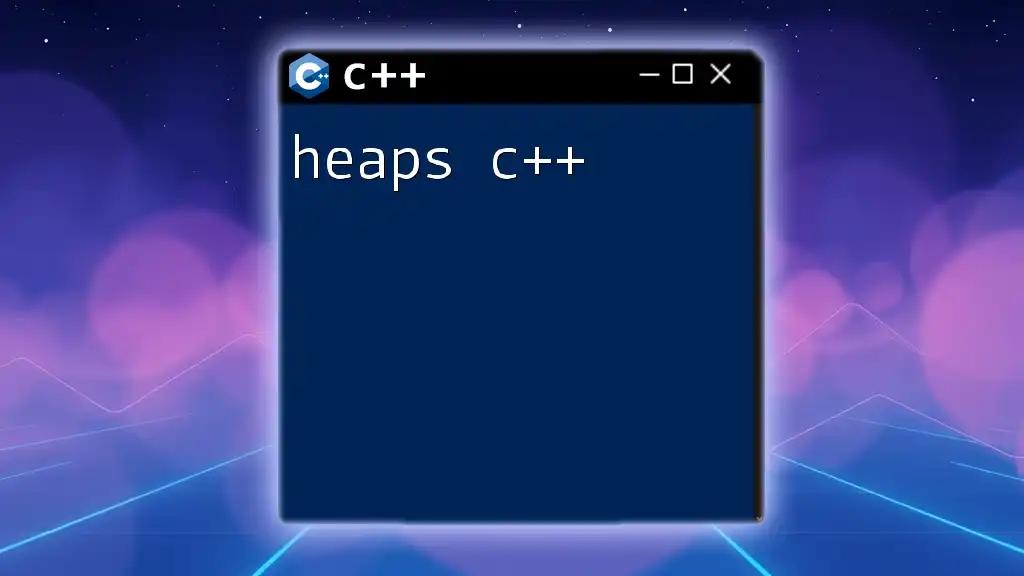
Implementing Game Rules and Logic
Validating Moves
The rules governing piece movements must be rigorously enforced to ensure fair play. Each piece's specific moving rules should be implemented in its respective class.
For example, to validate a knight's move, you might write:
class Knight : public Piece {
public:
bool isValidMove(int startX, int startY, int endX, int endY) override {
return (abs(startX - endX) == 2 && abs(startY - endY) == 1) ||
(abs(startX - endX) == 1 && abs(startY - endY) == 2); // Knight moves in L-shape
}
};
Checking for Game States
Win Conditions and Checkmate
Detecting game states is crucial for determining the outcome of the game. Implement logic to check for situations such as check, checkmate, and stalemate.
For example, to check if a player's king is in check, you might implement:
bool isKingInCheck(Player player) {
// Check all opposing pieces to see if they can attack the player's king
}
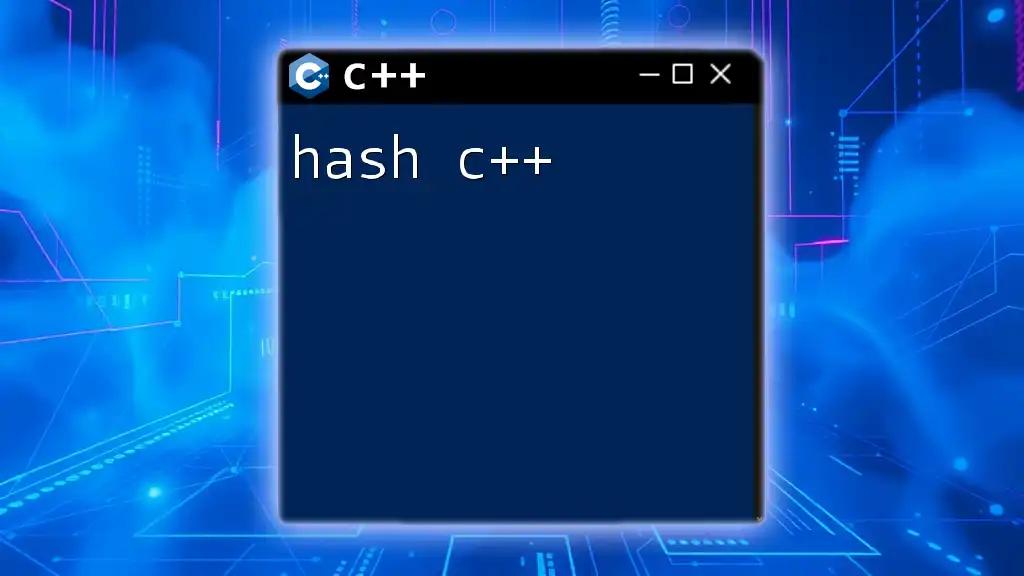
Implementing Player Interactions
Player Input Handling
Receiving and interpreting player input is essential for gameplay. You can utilize simple console input to capture moves:
std::string input;
std::cout << "Enter your move (e.g., e2 e4): ";
std::cin >> input;
Developing AI for Opponent Moves
Basic AI Implementations
Implementing an AI for your chess game can significantly enhance the gameplay experience. For a basic AI, you can incorporate simple heuristics to evaluate moves and choose the best one.
A foundational AI could employ a mini-max algorithm that evaluates possible outcomes based on available moves. Here’s a simplified approach:
int minimax(int depth, bool isMaximizing) {
if (isGameOver()) {
return evaluateBoard();
}
if (isMaximizing) {
int bestValue = -1000;
// Loop through moves, calling minimax recursively
return bestValue;
} else {
int bestValue = 1000;
// Loop through moves, calling minimax recursively
return bestValue;
}
}
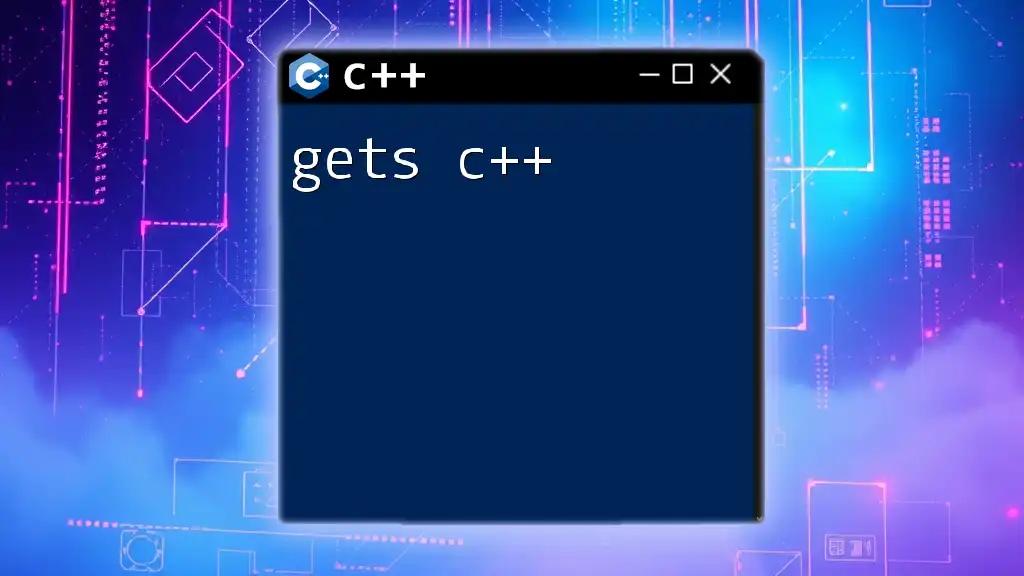
Enhancing the User Experience
Adding a Graphical User Interface (GUI)
A GUI can enhance the chess game significantly, making it more engaging for users. Libraries such as Qt or SFML can be utilized to create visually appealing interfaces.
To set up a GUI with Qt, for example, you'd begin with a project that integrates your chess logic. Drawing the board and pieces graphically requires a different approach than console output, but the basics of the game remain unchanged.
Sound and Music Integration
Incorporating sound effects and music can elevate the gaming experience. Use libraries like SDL_mixer to add audio feedback when a piece moves or when a check is announced.
Integrate sound like this:
Mix_Chunk *moveSound = Mix_LoadWAV("move.wav");
Mix_PlayChannel(-1, moveSound, 0);
This provides immediate feedback and enriches player interaction.
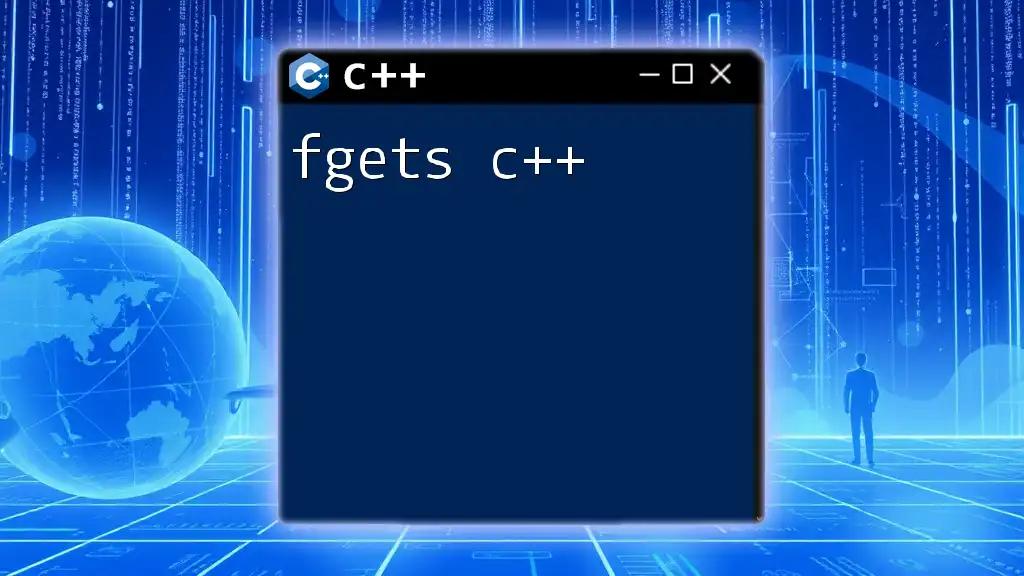
Testing Your C++ Chess Game
Importance of Testing in Game Development
Testing is a critical phase in game development. It helps identify bugs and ensures that your game mechanics function as intended.
Different types of testing can be applied, including unit testing to verify individual components, and integration testing to ensure they work well together.
Debugging Techniques
Debugging can be challenging, but there are methods to ease the process. Using GDB or built-in IDE debuggers can help trace errors and monitor variable states during execution.
Incorporate print statements or logging to check the progress and state of your program, making it easier to locate issues during development.
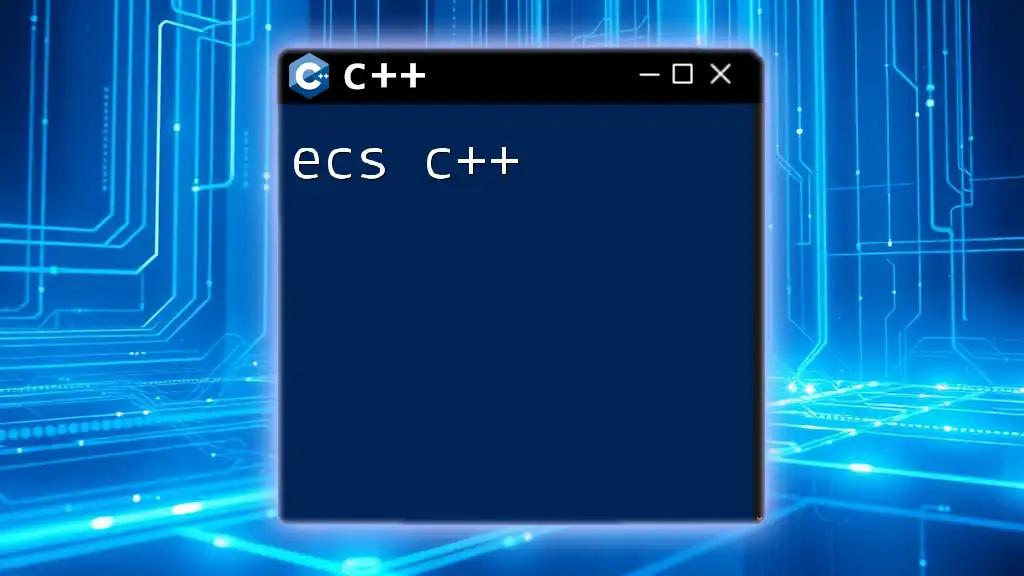
Conclusion
Creating a chess game in C++ is an enriching experience that combines programming skills with strategic thinking. As you continue improving your project, consider implementing advanced features such as online multiplayer support, advanced AI, or enhanced graphics.
This journey will provide profound insights into both C++ programming and game development. Expand your knowledge further with resources like books, online courses, and community forums dedicated to C++ and game design.
Encouragement is key: dive into your chess game development, experiment, and let your creativity lead the way!