In C++, a static class is a class that can only contain static members, meaning all its members belong to the class itself rather than any specific instance, effectively making it a collection of static methods and variables.
Here's a simple example in C++:
class MyStaticClass {
public:
static int staticVariable;
static void staticFunction() {
// Function logic here
}
};
int MyStaticClass::staticVariable = 0; // Initialize static variable
Understanding Static Members in C++
What are Static Members?
In C++, static members are variables or methods that belong to the class rather than any specific instance of the class. When a member is declared as static, it is shared among all instances of the class. This means that all objects of the class share the same static variable, and if one instance modifies the static variable, the change reflects in all other instances.
Key Differences between Static and Non-Static Members:
- Static members are initialized when the class is loaded, irrespective of instance creation. On the other hand, non-static members are initialized when an instance of the class is created.
- There can be only one copy of a static member for the entire class, while each instance has its own copy of non-static members.
Accessing Static Members
Static members can be accessed using the class name followed by the scope resolution operator `::`. For example:
class MyClass {
public:
static int staticVar;
};
// Definition of static variable
int MyClass::staticVar = 5;
int main() {
// Accessing static member using class name
std::cout << MyClass::staticVar << std::endl; // Outputs: 5
return 0;
}
In this code, `staticVar` is a static member of `MyClass` and is accessible without creating an instance of `MyClass`.
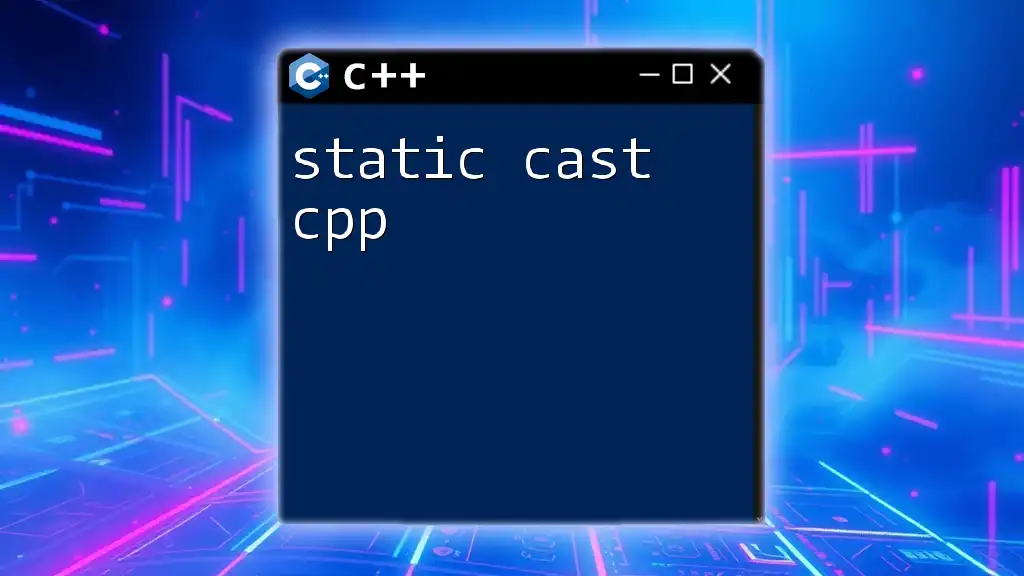
Creating a Static Class in C++
Syntax for Declaring a Static Class
Unlike some other programming languages, C++ does not have a dedicated `static class` keyword. However, you can create a class with all static members, making it similar to a static class concept.
class StaticClass {
public:
static void display() {
std::cout << "Static class functionality." << std::endl;
}
};
Example: Simple Static Class
Let's create a simple static class that contains a static variable and static method:
class Counter {
public:
static int count;
static void increment() {
count++;
}
static void displayCount() {
std::cout << "Count: " << count << std::endl;
}
};
// Definition of static variable
int Counter::count = 0;
int main() {
Counter::increment();
Counter::displayCount(); // Outputs: Count: 1
Counter::increment();
Counter::displayCount(); // Outputs: Count: 2
return 0;
}
In this example, `Counter` acts like a static class. The static variable `count` keeps track of how many times the `increment()` method is called. This behavior showcases the power of static members in maintaining a shared state.
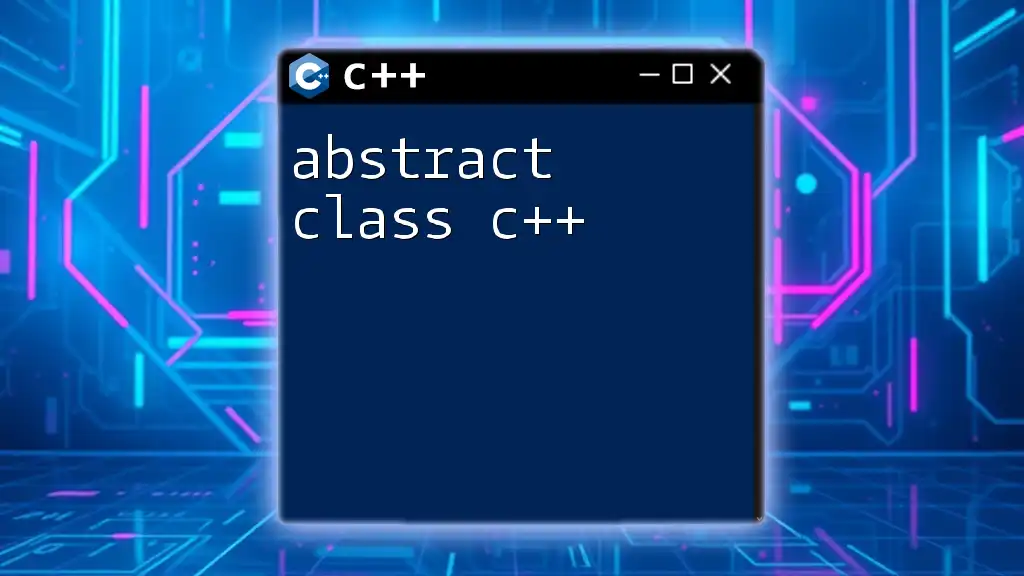
Benefits of Using Static Classes in C++
Memory Management
Static classes can lead to efficient memory usage. Since static members are stored in a single memory location, it reduces the overall memory footprint, especially when multiple instances of a class exist. Static classes essentially reside in the data segment of memory, unlike instance members that may reside in the stack.
Shared State Across All Instances
One of the major advantages of static classes is the capability to maintain a shared state across all instances. This can be particularly useful in scenarios where you need a single point of reference, such as a configuration setting or a counter.
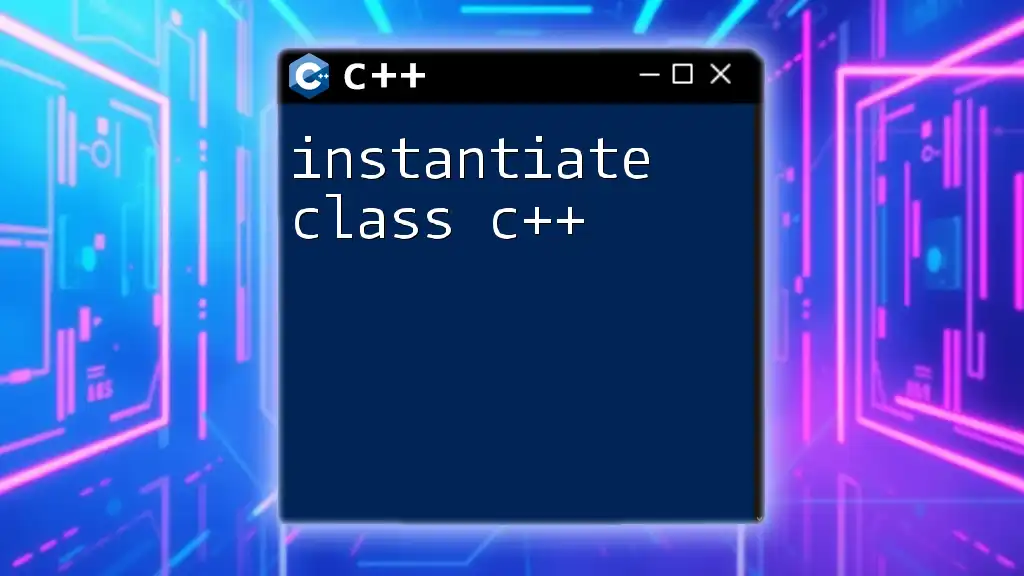
Limitations of Static Classes in C++
No Instance Creation
The design of static classes in C++ prevents instance creation. This can be beneficial in many scenarios, as it ensures all state is shared and controlled. However, it also limits flexibility; you can't create multiple variations of the class with different states.
Pros:
- Eliminates ambiguity about instance state.
- Reduces memory consumption.
Cons:
- Lacks the ability to customize separate instances.
- Makes it challenging to incorporate polymorphism.
Static Initialization Conflicts
Static initialization can lead to conflicts, especially when resources depend on each other. For example, if you have multiple static members in different files, their initialization order can become problematic.
class A {
public:
static B b; // Declaring static member B
};
class B {
public:
B() {
// Constructor may depend on A
}
};
// Potential conflict when A and B are initialized
To avoid this, always ensure that static members are initialized only when required, possibly using lazy initialization.
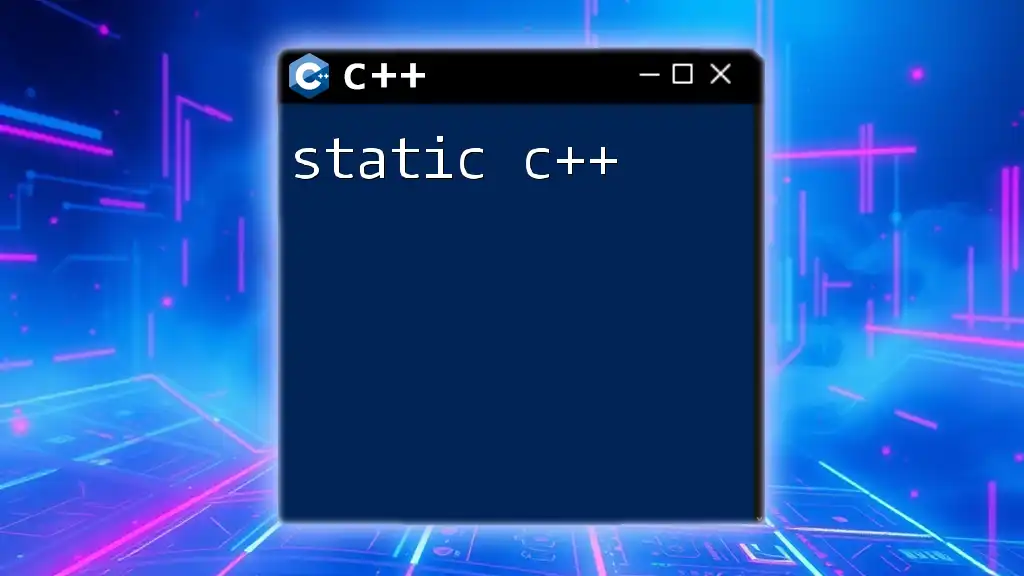
Practical Applications of Static Classes in C++
Utility Static Classes
Static classes are often employed as utility classes that provide functionalities without the need for instance creation. A typical example includes mathematical operations,
class MathUtil {
public:
static int add(int a, int b) {
return a + b;
}
static int multiply(int a, int b) {
return a * b;
}
};
int main() {
int sum = MathUtil::add(5, 10);
std::cout << "Sum: " << sum << std::endl; // Outputs: Sum: 15
return 0;
}
In this example, `MathUtil` provides utility methods without needing an instance, thus highlighting the practicality of static classes.
Singleton Design Pattern
The Singleton design pattern is a design principle that restricts a class from instantiating multiple times. It uses static members to provide a single global access point to the instance of the class.
class Singleton {
private:
static Singleton* instance;
// Private constructor to prevent instantiation
Singleton() {}
public:
static Singleton* getInstance() {
if (!instance) {
instance = new Singleton();
}
return instance;
}
};
// Initialize the static member
Singleton* Singleton::instance = nullptr;
int main() {
Singleton* s1 = Singleton::getInstance();
Singleton* s2 = Singleton::getInstance();
std::cout << (s1 == s2); // Outputs: 1 (true), both point to the same instance
return 0;
}
In this example, the `Singleton` class ensures that it has only one instance throughout the application, demonstrating one of the most renowned patterns facilitated by static class functionality.
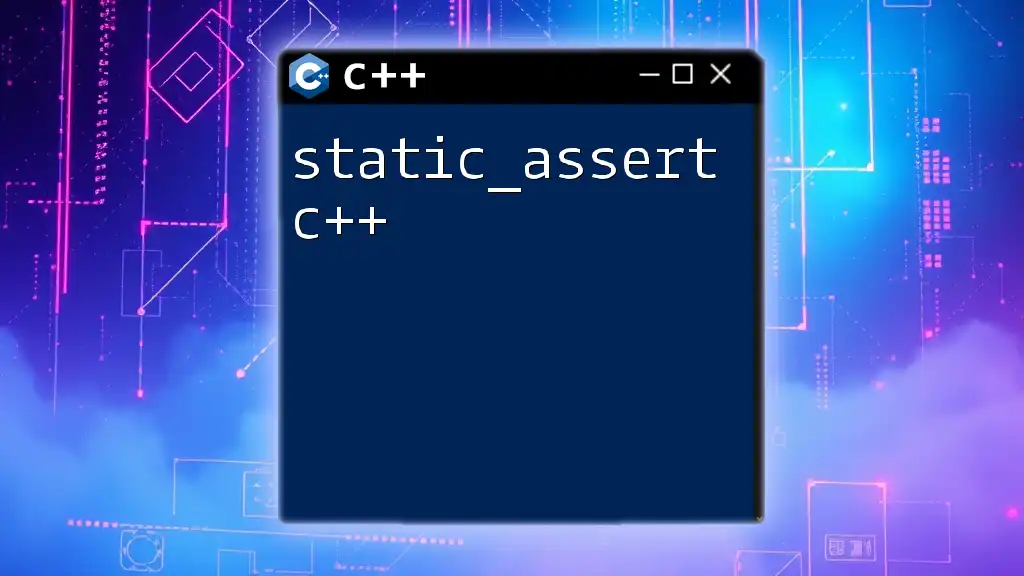
Conclusion
In summary, static classes in C++ provide a powerful mechanism for maintaining shared state and functionality across instances without the overhead of object instantiation. While they come with limitations, such as the inability to create instances and possible initialization conflicts, their advantages are substantial in specific use cases, such as utility classes and patterns like Singleton. It's crucial to understand when to use static classes and how they can optimally serve your programming needs.
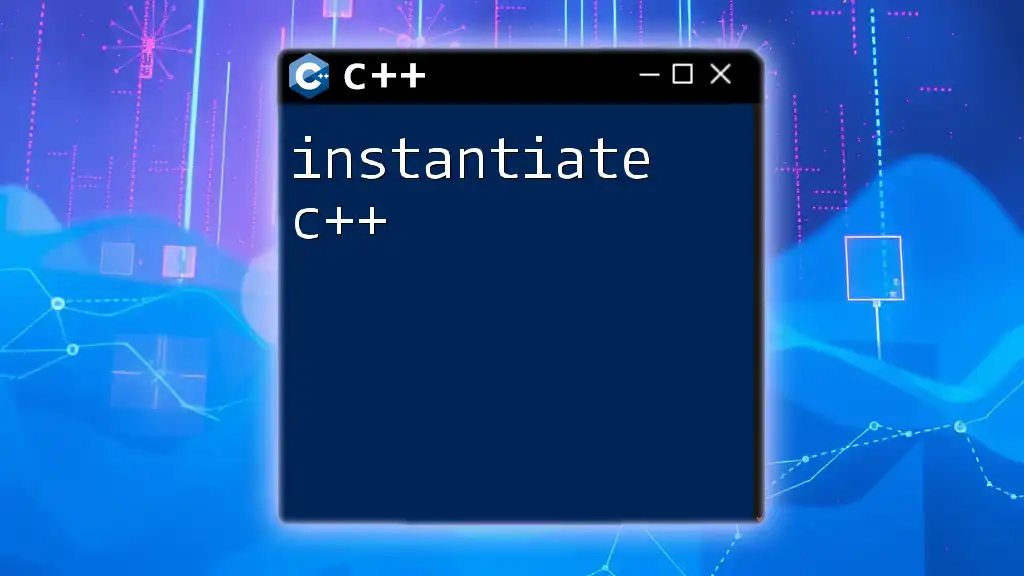
FAQs About Static Classes in C++
Common Questions
What is a static class in C++?
A static class refers to a class where all members are static. This means that the members are shared across all instances, and the class cannot be instantiated.
Can static classes have constructors?
No, since you cannot instantiate static classes in C++, they do not require constructors in the traditional sense. However, static member variables can be initialized.
How do static methods differ from instance methods?
Static methods belong to the class rather than instances, which means they can be called without creating an object of the class. Instance methods, however, require an object to be invoked and can access both instance and static members.