A `static_cast` in C++ is used to perform conversions between types at compile-time, providing type safety while allowing explicit conversion of related types or primitive data types.
Here's an example of using `static_cast`:
#include <iostream>
int main() {
double num = 9.7;
int wholeNumber = static_cast<int>(num); // Converts double to int
std::cout << "Converted number: " << wholeNumber << std::endl; // Output: 9
return 0;
}
What is `static_cast` in C++?
`static_cast` is a type of casting operator in C++ that allows for explicit conversions between different data types. Unlike C-style casting, which can lead to less safe conversions, `static_cast` performs the conversion at compile-time, ensuring that you get meaningful results without unexpected behavior.
Purpose of `static_cast`
Use `static_cast` primarily when you need to convert between types such as numeric types or class pointers in a safe manner. This operator can handle conversions that have a clear and direct relationship, making it a preferred choice among C++ developers when type safety and code readability are important.

The Importance of Type Safety
Understanding Type Safety
Type safety is a principle in programming that prevents type errors, ensuring that a program only manipulates data in ways that are safe and logical according to the designated data types. In C++, type safety helps to catch errors at compile time rather than at runtime, which can save precious debugging time.
How `static_cast` Ensures Safety
`static_cast` stands out from other casting strategies—such as `reinterpret_cast`, `const_cast`, and C-style casts—because it provides better compile-time checks, reducing the chances of runtime errors. While `reinterpret_cast` allows for any conversion between types regardless of compatibility, `static_cast` ensures that the conversion is valid and can be safely performed.

Basic Syntax of `static_cast`
The basic syntax for using `static_cast` is:
static_cast<type>(expression)
Here, type can be any data type you want to convert to, and expression is the value you're converting. For example, consider the following:
int num = 10;
double dblValue = static_cast<double>(num);
In this example, `num`, which is an integer, is converted to a double. This explicit conversion allows for the precision of floating-point arithmetic and avoids possible data loss, making your code cleaner and safer.
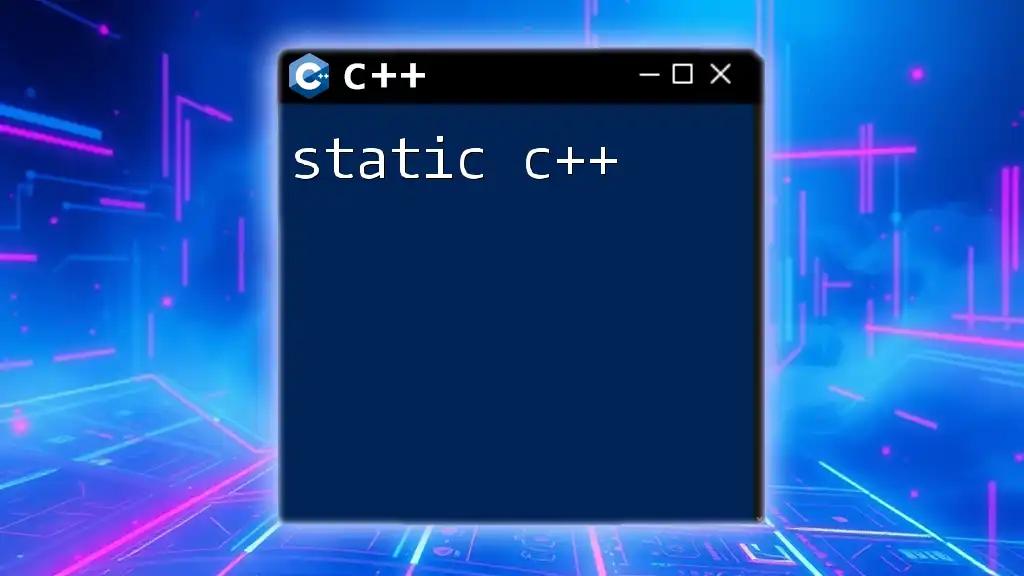
Common Use Cases for `static_cast`
Converting Numeric Types
One common application of `static_cast` is converting between numeric types, such as integers and floating-point numbers.
For instance, converting an integer to a float helps in performing calculations without causing truncation errors:
int myInt = 5;
float myFloat = static_cast<float>(myInt);
Here, `myInt` is explicitly converted to a float. The float will represent 5.0, and this avoids any ambiguity that might arise from implicit conversions.
Converting Pointers and References
`static_cast` is also widely used for converting pointers and references in inheritance hierarchies:
Upcasting and Downcasting
Upcasting refers to converting a pointer or reference from a derived class to a base class, which is generally safe. For example:
class Base {};
class Derived : public Base {};
Derived d;
Base* b = static_cast<Base*>(&d);
In this snippet, an object of type `Derived` is cast to a pointer of type `Base`. Since there’s a clear inheritance relationship, this is safe.
Downcasting is converting a base class pointer/reference back to a derived class type. While `static_cast` can accomplish this, it requires caution because it doesn't perform runtime checks to ensure that the object being cast is indeed of the derived class type.
Converting Enums to Integers
`static_cast` can also convert enumerated types to their underlying integer values effectively. For instance:
enum Color { Red, Green, Blue };
int colorValue = static_cast<int>(Color::Green);
In this case, the enum `Color::Green` is converted to its integer representation, which can be useful when you need to perform arithmetic or comparisons involving enum values.

When to Avoid `static_cast`
Limitations of `static_cast`
While `static_cast` is useful, there are scenarios where you should avoid using it:
- Incompatible Types: If you're attempting to convert incompatible types, such as a pointer to an unrelated class, `static_cast` will lead to compilation errors, helping to avoid unforeseen issues in your code.
Alternative Casting Methods
In cases where you need to perform less safe operations or manipulate constness, consider using `const_cast` for removing const qualifiers, or `reinterpret_cast` for raw memory manipulation. Always choose the casting method that best suits your needs while adhering to the principles of type safety.
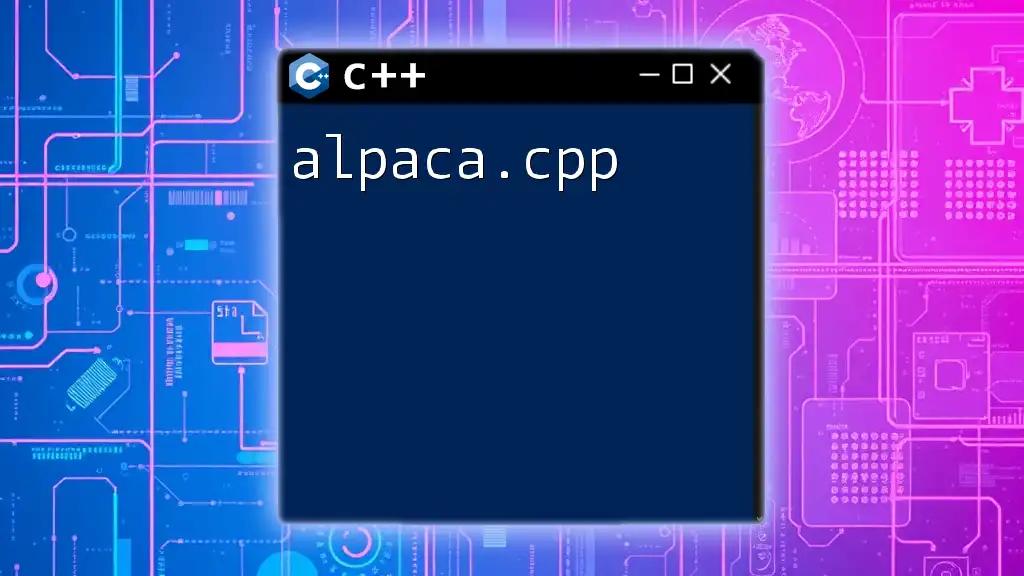
Best Practices for Using `static_cast`
Readability and Maintainability
When using `static_cast`, aim for clear and understandable code. Providing direct casts improves the readability of your code, making logical relationships between types more apparent to other developers or even your future self.
Potential Pitfalls to Avoid
Be cautious of common mistakes associated with `static_cast`:
- Downcasting Issues: Always ensure that the object actually points to the correct derived type; otherwise, you could encounter undefined behavior during runtime.
- Data Loss: Be aware that converting from a larger data type to a smaller one (e.g., from `double` to `int`) may result in lost precision.

Conclusion
In summary, `static_cast` is a powerful tool in C++ for safely converting between data types while enhancing both type safety and code clarity. Understanding how to properly utilize it, as well as recognizing its limitations, will undoubtedly improve both the reliability and readability of your C++ projects. Practice implementing `static_cast` in your own code, and watch your programming skills soar!

Additional Resources
For those looking to deepen their understanding of C++ type casting, numerous books and online tutorials provide extensive information. Additionally, you can reinforce your knowledge by tackling coding exercises that require the use of `static_cast`. Keep practicing, and soon you'll master this important aspect of C++!