C++ (often referred to as CPP) is a powerful, high-level programming language that supports object-oriented, procedural, and generic programming features, allowing developers to create efficient and reusable software applications.
Here’s a simple code snippet in C++ that demonstrates how to output "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a general-purpose programming language that is widely used for a variety of software development tasks. Initially developed as an enhancement to the C programming language, C++ was designed to include features for object-oriented programming (OOP), making it both a powerful and versatile tool for developers. Its ability to enable low-level memory manipulation, along with object-oriented aspects, makes it a crucial part of many software applications.
Key Features of C++
-
Object-Oriented Programming (OOP): C++ introduces the concept of classes and objects, allowing developers to model real-world entities and relationships in their code. This makes software more modular, reusable, and easier to maintain.
-
Performance and Efficiency: C++ is known for its high performance. It provides programmers with fine control over system resources, enabling the creation of time-critical applications where efficiency is paramount.
-
Portability: Programs written in C++ can run on multiple platforms without or with minimal modifications, making it a favored choice for cross-platform development.
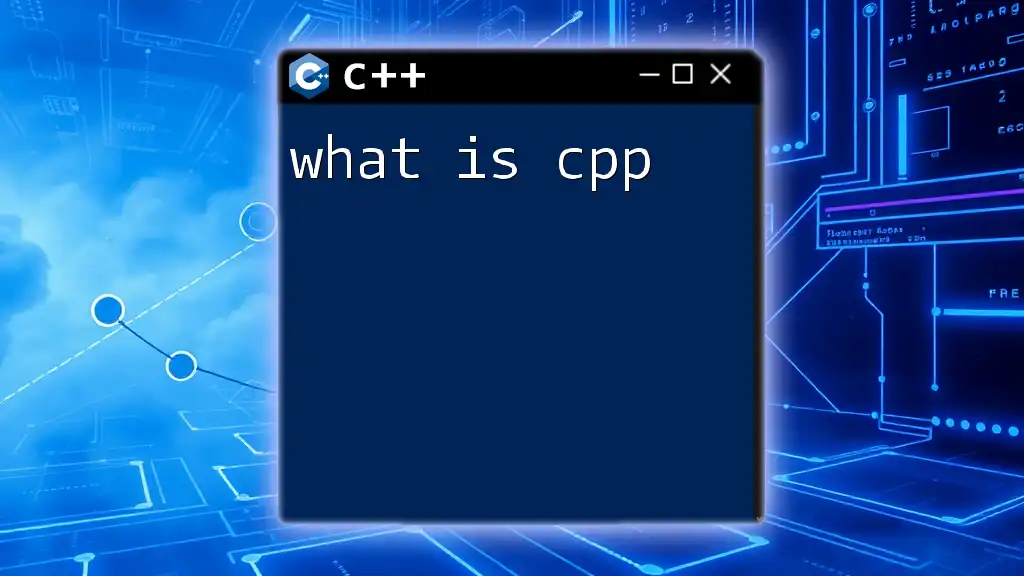
Why Learn C++?
Demand in the Job Market
C++ remains in high demand across various industries. Companies in sectors like gaming, finance, and systems programming require skilled C++ developers. The language is the backbone for many applications, ranging from sophisticated simulations to performance-intensive software.
Statistics reveal a continuing trend of job opportunities for C++ developers, emphasizing its significance in both the current job market and future technological advancements.
Versatility of C++
C++ boasts a wide range of applications. It is instrumental in:
-
Game Development: Major game engines, such as Unreal Engine, utilize C++ due to its speed and resource management capabilities.
-
Embedded Systems: Many embedded systems rely on C++ for performance-critical software due to its ability to directly manage hardware resources.
-
Desktop Applications: High-performance desktop software frequently leverages C++ for its efficiency.
A few well-known software products built with C++ include Adobe products, Microsoft Office, and many operating systems.
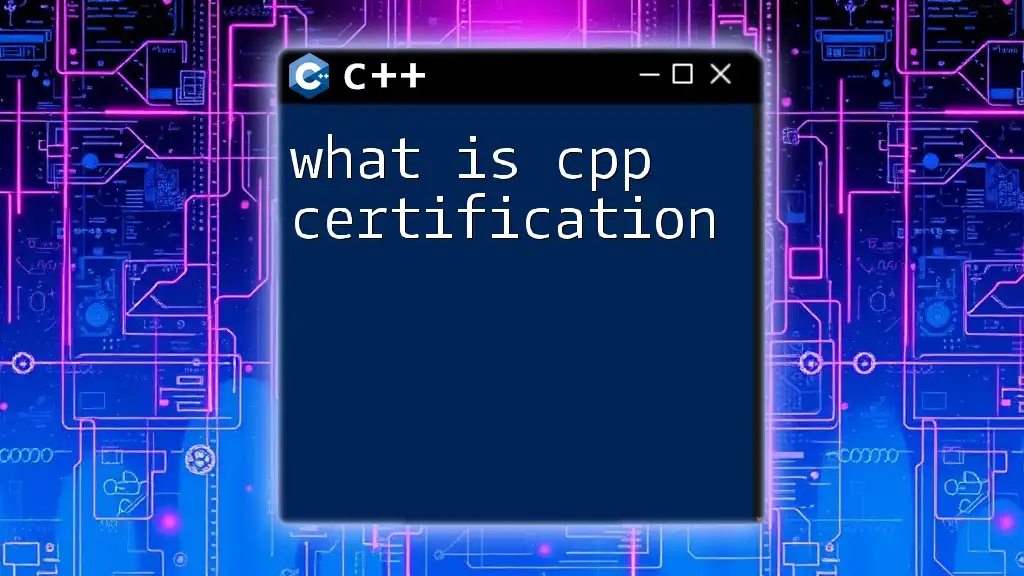
Getting Started with C++
Installing a C++ Compiler
To start coding in C++, the first step is to download and install a C++ compiler. Popular options include:
- GCC (GNU Compiler Collection)
- Clang
- Visual Studio (especially for Windows)
Each of these compilers has installation guides available on their respective websites, ensuring a smooth setup process based on your operating system.
Writing Your First C++ Program
Creating your first C++ application is simple. Here’s a classic example of a "Hello, World!" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Explanation:
- `#include <iostream>`: This line tells the compiler to include the input-output stream library, which is essential for outputting text to the console.
- `using namespace std;`: This directive allows access to standard library functions without the need for the `std::` prefix.
- `int main()`: This defines the main function where program execution begins.
- `cout << "Hello, World!" << endl;`: This line outputs the text to the console.
- `return 0;`: Indicates that the program executed successfully.
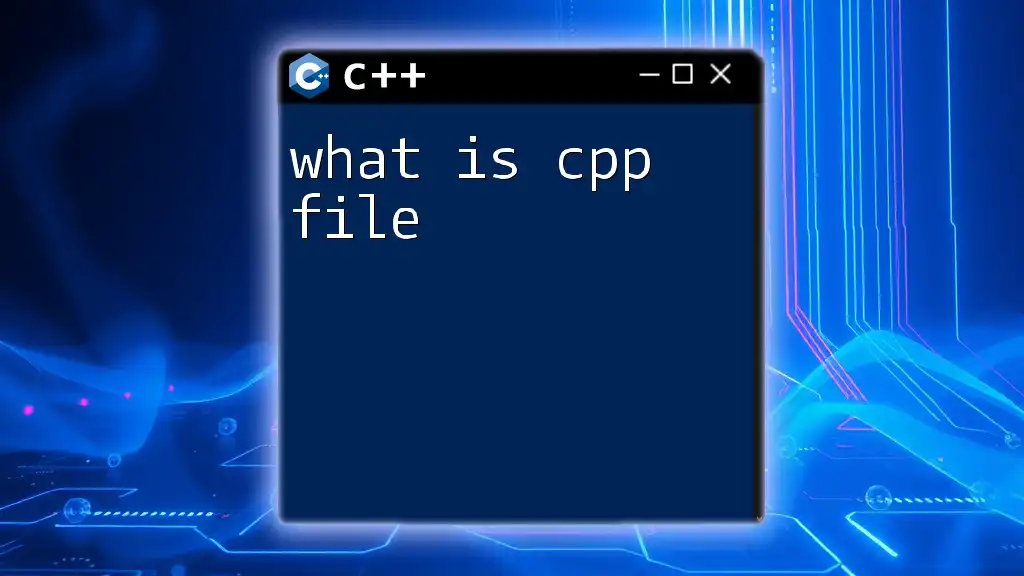
Basics of C++ Syntax
Data Types and Variables
C++ offers several primitive data types:
- int: Represents integer values.
- float: Suitable for single-precision floating-point values.
- double: Used for double-precision floating-point values.
- char: Represents a single character.
Variable declaration and initialization in C++ look like this:
int age = 25;
float height = 5.9;
Control Structures
Control structures in C++ help make decisions and loop through tasks. For instance, if statements are used for conditional execution:
if (age >= 18) {
cout << "Adult" << endl;
}
Loops, such as `for` and `while`, allow for repetitive execution of a block of code based on specific conditions.
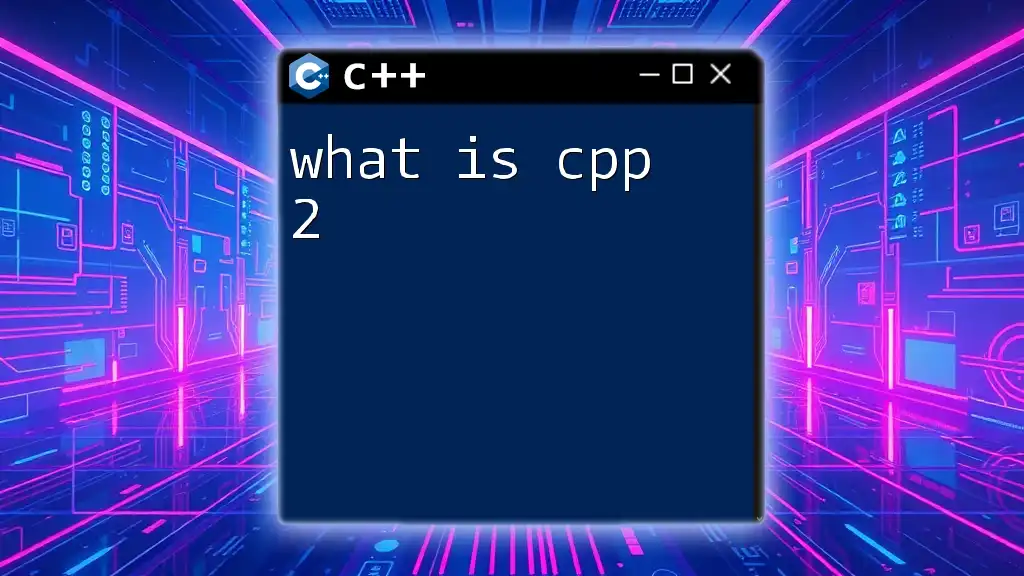
Object-Oriented Programming in C++
Classes and Objects
C++ is renowned for its object-oriented capabilities. Classes serve as blueprints for creating objects. Here’s a simple class example:
class Car {
string brand;
int year;
public:
void setDetails(string b, int y) {
brand = b;
year = y;
}
void displayDetails() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
In this code, we define a Car class with private member variables (`brand` and `year`) and public methods to set and display their values.
Inheritance and Polymorphism
Inheritance allows for creating a new class based on an existing one, promoting code reuse. For instance, if there’s a base class Vehicle, you can derive classes like Car and Truck, inheriting common features while adding specific attributes.
Polymorphism introduces the ability to process objects differently based on their data type or class. This can be achieved through function overloading and overriding.
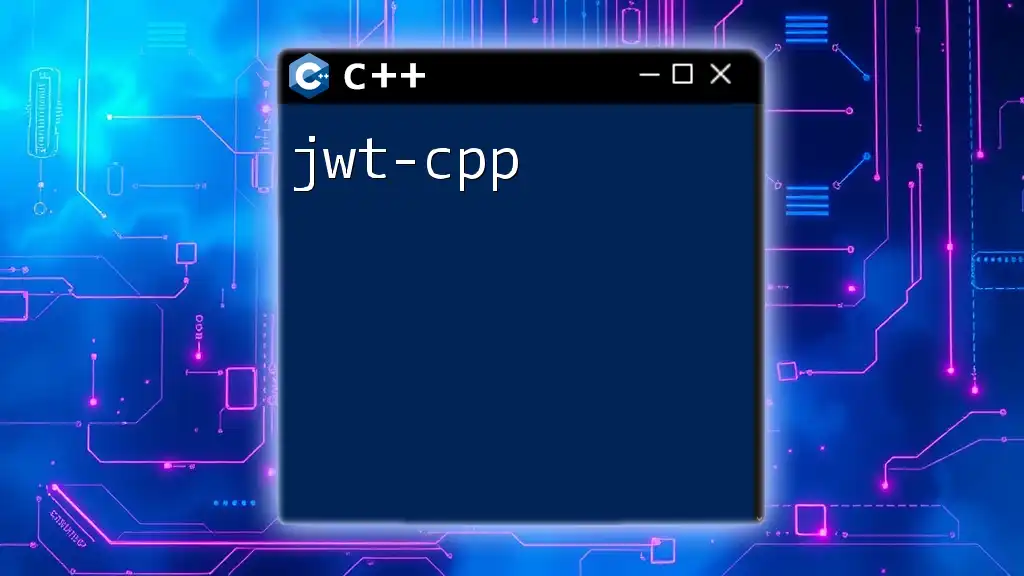
Advanced C++ Concepts
Templates
Templates provide a way to write generic and reusable code. They enable functions and classes to operate with different data types. Here’s a simple template function for adding two numbers:
template <typename T>
T add(T a, T b) {
return a + b;
}
This flexibility means that the `add` function can accept integers, floats, or any type specified by the user.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides a rich set of functionalities such as:
- Containers: Data structures like vectors, maps, and sets allow for efficient data management.
- Iterators: These provide a simple way to traverse through the elements of a container.
- Algorithms: A collection of algorithms for tasks like sorting, searching, and manipulation.
An example of using an STL container can be shown through vectors:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
cout << num << " ";
}
return 0;
}
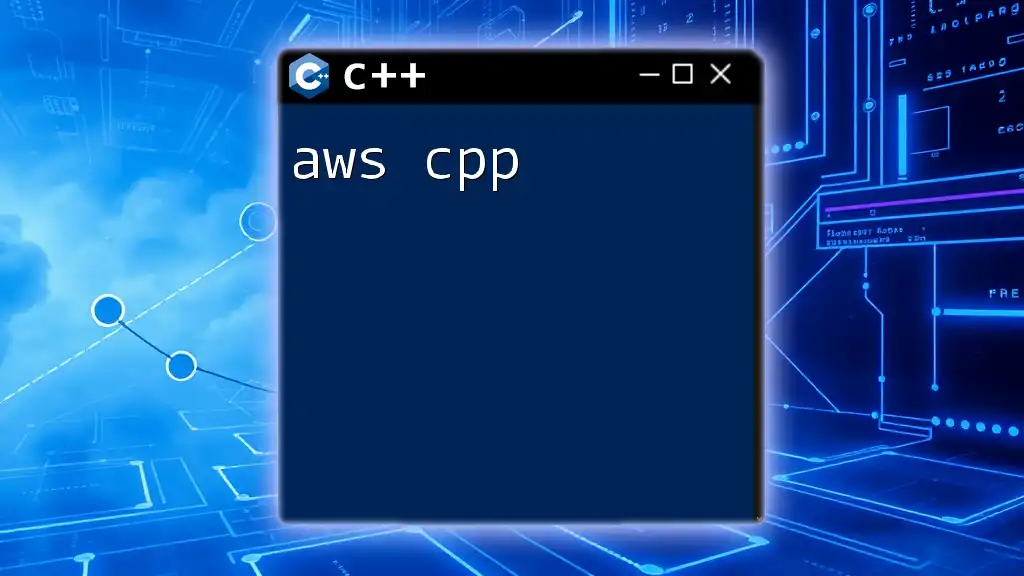
Best Practices for Learning C++
Recommended Learning Resources
As you embark on your C++ journey, consider following resources:
- Books: Titles like "The C++ Programming Language" by Bjarne Stroustrup are invaluable.
- Online Courses: Platforms like Udemy and Coursera offer structured learning paths.
- Communities: Engage with fellow learners on forums like GitHub and Stack Overflow.
Building a Portfolio
Creating software projects is essential to reinforce what you've learned. Consider contributing to open-source projects, as it provides experience with real-world codebases and collaboration with other developers.
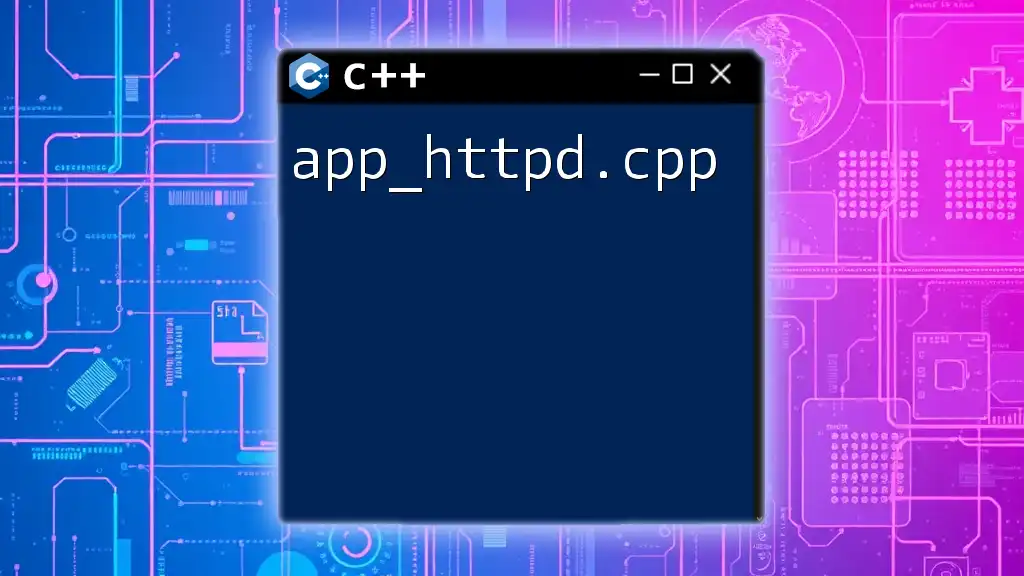
Conclusion
Learning C++ opens up numerous opportunities in the programming world. Its rich set of features and widespread application make it a valuable asset for any developer. The road to mastery involves continual practice and exploration of advanced concepts.
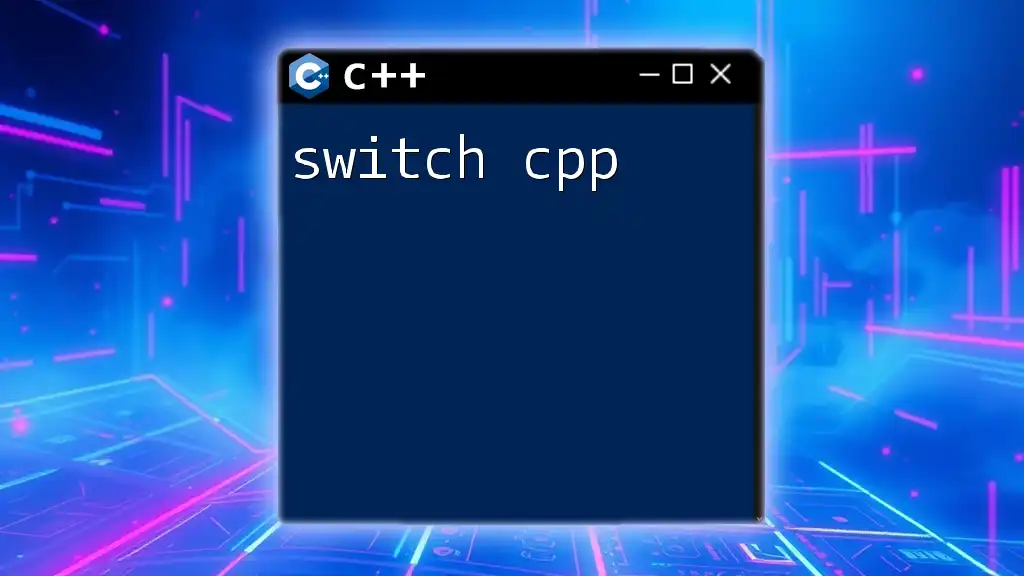
Call to Action
We encourage readers to share their thoughts or questions about C++ in the comments below. Explore our upcoming courses to delve deeper into the fascinating world of C++ programming!