In C++, a class serves as a blueprint for creating objects, encapsulating data and functions that operate on that data, as demonstrated in the following example:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Understanding Classes in C++
What is a C++ Class?
A C++ class can be understood as a blueprint for creating objects. In terms of Object-Oriented Programming (OOP), a class encapsulates data members (attributes) and member functions (methods) that define the behavior of the objects created from that blueprint. This encapsulation is fundamental as it promotes data hiding and prevents unauthorized access to class members, allowing a structured approach to programming.
Syntax of a C++ Class
To define a class in C++, you'll generally follow a basic syntax structure:
class ClassName {
// Data members
// Member functions
};
In this template, `ClassName` is your entity's name, while the sections for data members and member functions will define what attributes belong to the class and which actions can be performed with those attributes.
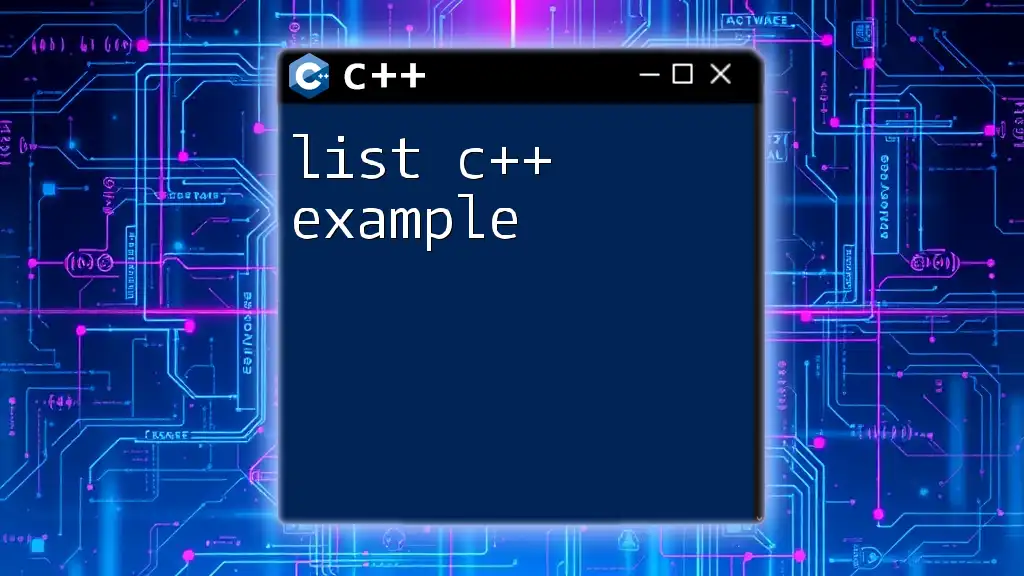
Sample C++ Class
Creating a Simple Class in C++
Let’s take a closer look with a practical example. Below is a simple C++ class called `Car`.
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
In this `Car` class, we have two public data members: `brand` (a string) and `year` (an integer). We also define a method called `displayInfo()` that outputs the brand and year of the car. The keyword `public` indicates that these attributes and methods are accessible from outside the class.
Instantiating Objects from a Class
Now that we've defined the class, we can create an object of this class and utilize its members.
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2020;
myCar.displayInfo();
Here, `myCar` is an instance of the `Car` class. We assign values to its `brand` and `year` attributes and then call `displayInfo()` to print the car's details. This illustrates how easy it is to create and work with objects derived from a class.
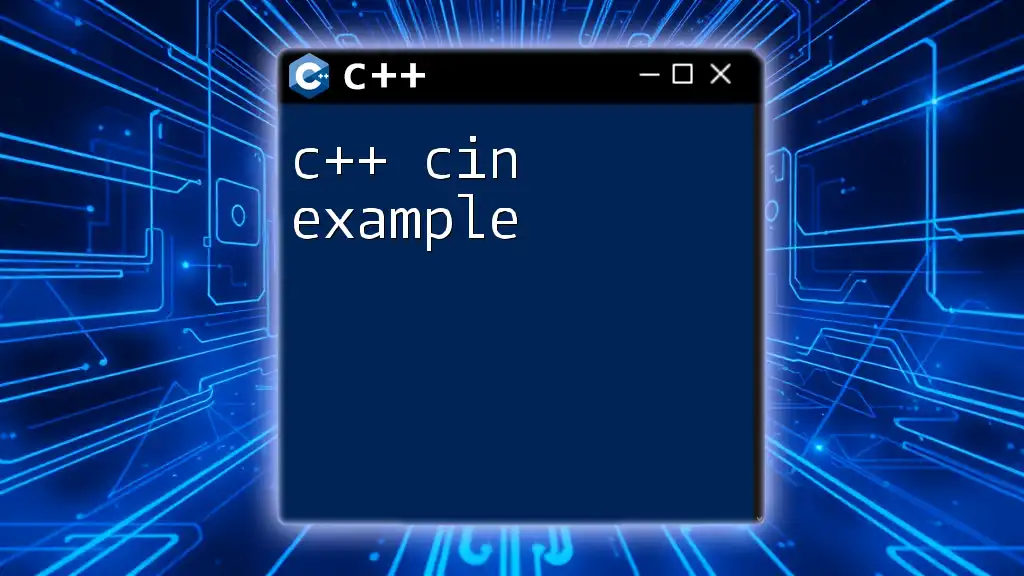
Advanced Class Concepts
Constructors and Destructors
Constructors are special member functions that are automatically called when an object of a class is created. They help initialize an object with default or specified values.
Here is an example of a constructor within the `Car` class:
class Car {
public:
string brand;
int year;
// Constructor
Car(string b, int y) {
brand = b;
year = y;
}
};
In this instance, the constructor `Car(string b, int y)` initializes the `brand` and `year` attributes.
Similarly, destructors are used to free resources once an object goes out of scope or is deleted. A simple destructor might look like:
~Car() {
// Cleanup code here
}
While it's optional to define a destructor in many classes, it can be critical if resources must be managed explicitly.
Inheritance in C++
Inheritance allows a class to inherit attributes and methods from another class, promoting code reusability.
For instance, consider a base class `Vehicle` and a derived class `Car`:
class Vehicle {
public:
void start() {
cout << "Vehicle started." << endl;
}
};
class Car : public Vehicle {
public:
void honk() {
cout << "Car honks!" << endl;
}
};
In this example, `Car` inherits from `Vehicle`, which means it can utilize the `start()` method and its own method, `honk()`. Using inheritance streamlines programming and allows for logical class structures.
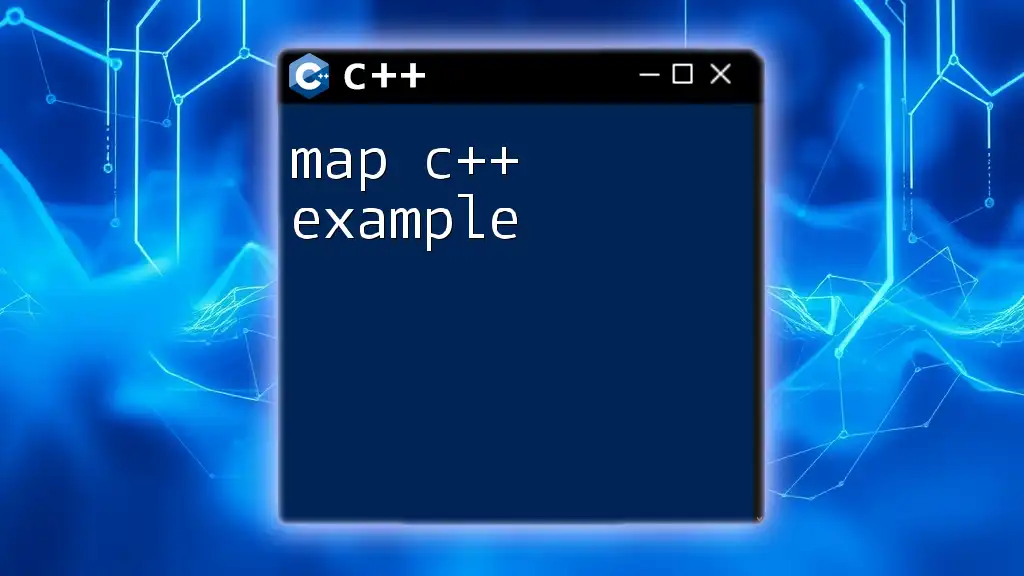
Class Access Modifiers
Public, Private, and Protected Access
Access modifiers determine the visibility of class members. The three primary access modifiers in C++ are public, private, and protected.
Here’s how you can utilize these modifiers to control access:
class Person {
private:
string name;
public:
void setName(string n) {
name = n;
}
string getName() {
return name;
}
};
In this example:
- `name` is a private member, meaning it cannot be accessed directly from outside the `Person` class.
- `setName()` and `getName()` are public methods that control access to the private data member. This encapsulation is central to solid OOP practices.
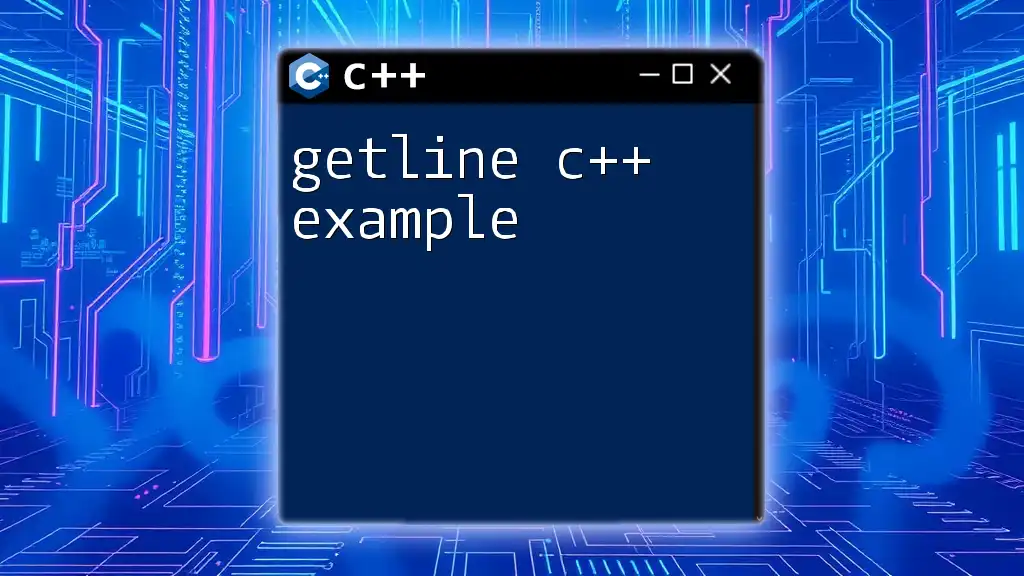
Useful C++ Class Features
Operator Overloading
C++ allows operator overloading, enabling developers to define custom behaviors for operators based on class types. For instance, consider a `Complex` number class where you can overload the `+` operator:
class Complex {
public:
float real;
float imag;
Complex operator + (const Complex& obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
};
In this example, when you add two `Complex` objects, a new `Complex` object is returned that represents their sum. This leads to cleaner and more intuitive code.
Friend Functions
A friend function can access the private and protected members of a class even if it’s not a member of that class. Here’s a simple example to illustrate this:
class Box;
class BoxManager {
public:
void displayVolume(Box& box);
};
class Box {
private:
int length;
public:
Box(int l) : length(l) {}
friend void BoxManager::displayVolume(Box& box);
};
void BoxManager::displayVolume(Box& box) {
cout << "Volume: " << box.length * box.length * box.length << endl;
}
In this case, `BoxManager` is a friend of `Box`, allowing `displayVolume()` to access the private `length` member directly, showcasing the flexibility of C++ regarding friend functions.
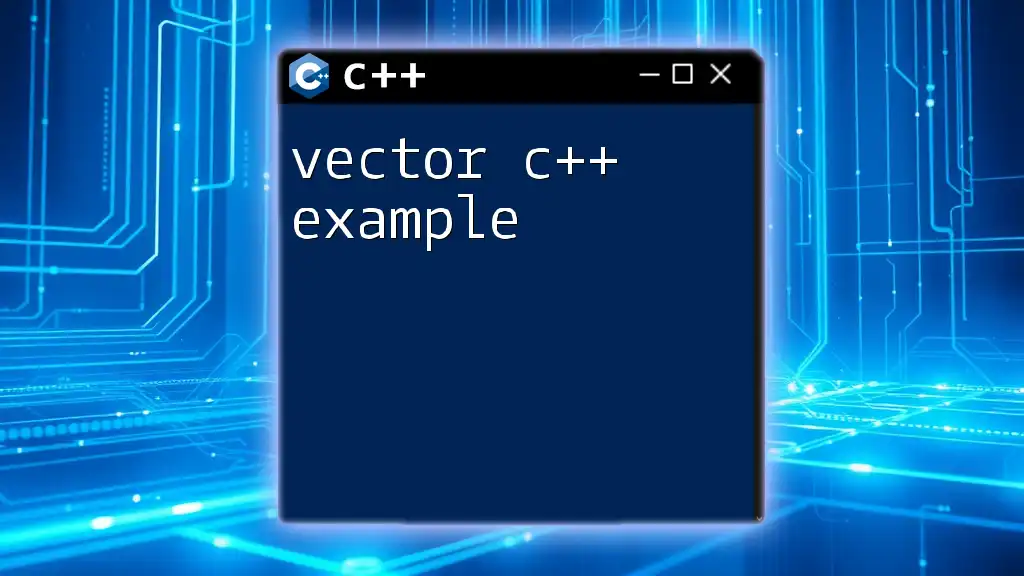
Conclusion
Through this comprehensive guide, we have explored various aspects of a class cpp example – from understanding the fundamental structure, creating simple classes and objects, to exploring advanced concepts such as inheritance, constructors, destructors, and more. Mastering these concepts is paramount for anyone aspiring to become proficient in C++. As you continue to practice and employ these techniques, you will significantly enhance your programming skills in C++.
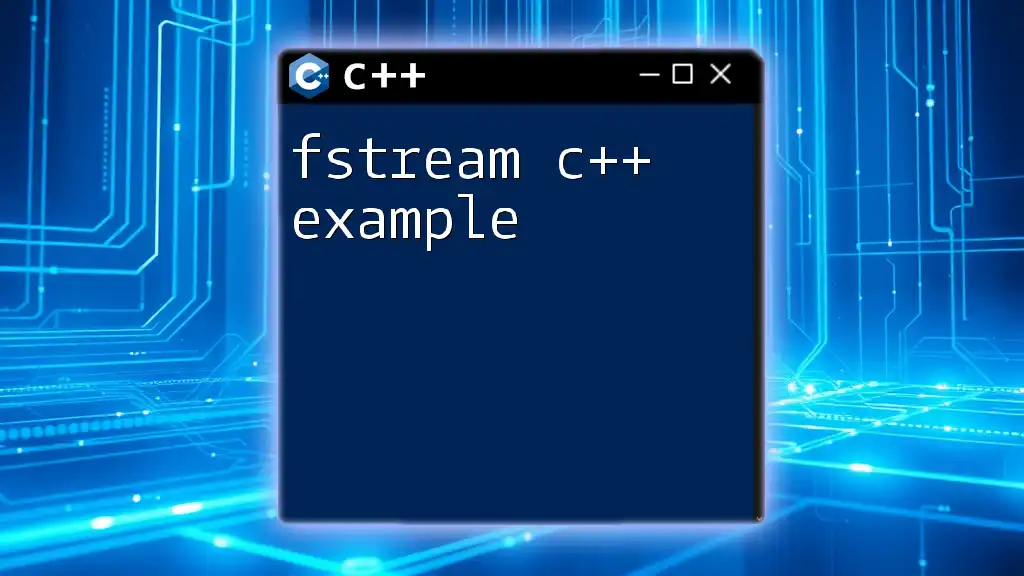
Additional Resources
For further learning, consider exploring the following resources:
- C++ documentation for class constructs
- Online tutorials on C++ classes and OOP
- Recommended courses for in-depth C++ programming training