Doxygen is a powerful documentation generation tool for C++ that enables developers to create clear and organized API documentation from comments embedded in the source code. Here's a simple example of how to use Doxygen to document a class in C++:
/**
* @brief A simple class that demonstrates Doxygen usage.
*/
class MyClass {
public:
/**
* @brief Constructor for MyClass.
*/
MyClass();
/**
* @brief A simple method that adds two integers.
* @param a First integer.
* @param b Second integer.
* @return Sum of a and b.
*/
int add(int a, int b);
};
Introduction to Doxygen
What is Doxygen?
Doxygen is a powerful tool designed for generating documentation from annotated source code. It supports various programming languages, with its capabilities particularly well-suited for languages like C++. The primary objective of Doxygen is to facilitate easier access to project documentation, allowing developers to understand code functionality swiftly.
Why Use Doxygen for C++?
Using Doxygen for documenting C++ projects offers numerous advantages. It enhances code readability, making it easier for developers to share and maintain code over its lifecycle. Well-documented code also reduces the onboarding time for new team members and decreases potential misunderstandings in a collaborative environment.
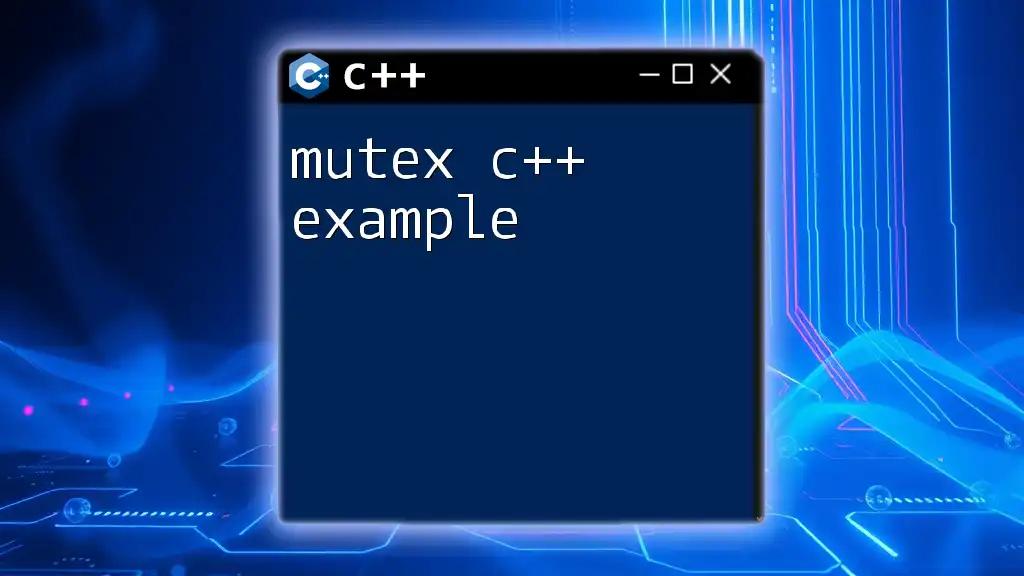
Setting Up Doxygen for a C++ Project
Installing Doxygen
Installing Doxygen varies slightly depending on the operating system. Here’s how to get it set up on different platforms:
- Windows: Download the installer from the Doxygen website and follow the installation wizard.
- macOS: You can install Doxygen via Homebrew. Run `brew install doxygen` in the terminal.
- Linux: Use the package manager of your distribution. For example, on Ubuntu, you can use `sudo apt-get install doxygen` to install Doxygen.
Once installed, it’s crucial to ensure Doxygen is added to your system's PATH so you can invoke it from the command line easily.
Creating a Doxygen Configuration File
After Doxygen is successfully installed, you need to create a configuration file. Run the following command in your project directory:
doxygen -g
This command generates a default configuration file named `Doxyfile`. You’ll need to customize several key parameters within this file:
-
INPUT: Specify the directory where the source code resides. For example:
INPUT = src/
-
OUTPUT_DIRECTORY: Choose where the generated documentation will be stored. For instance:
OUTPUT_DIRECTORY = doc/
-
RECURSIVE: Decide whether Doxygen should search through subdirectories. Setting this to `YES` is useful if your codebase is organized into multiple folders.
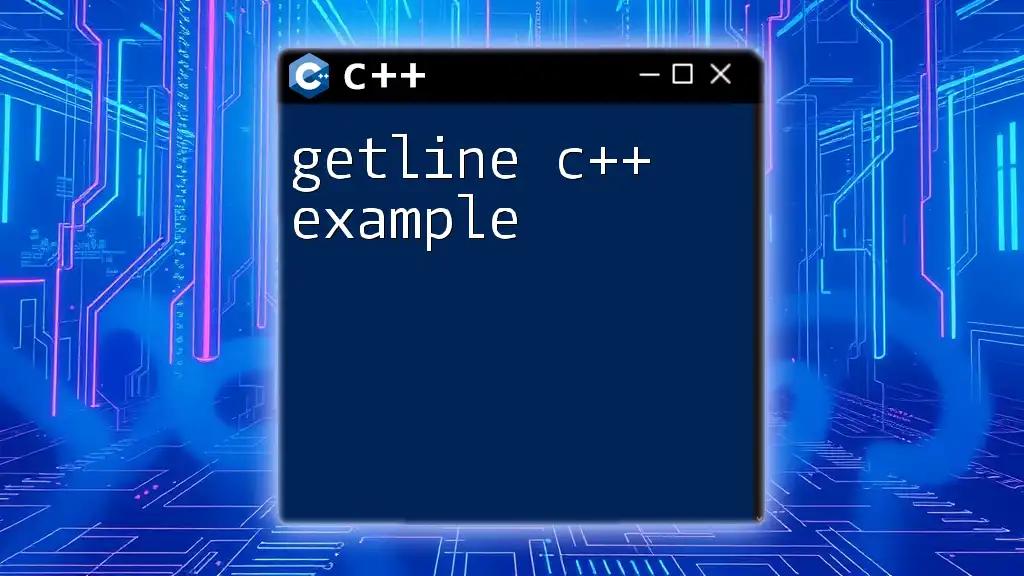
Writing Doxygen Comments in C++
Doxygen Comment Syntax
When documenting code with Doxygen, understanding the comment styles is vital. Doxygen supports both single-line and multi-line comments. Multi-line comments are particularly useful for more detailed descriptions.
Documenting Classes and Functions
To illustrate how to document a class and its functions, consider the following example:
/**
* @class ExampleClass
* @brief This class demonstrates the usage of Doxygen comments.
*/
class ExampleClass {
public:
/**
* @brief Constructs a new ExampleClass object
* @param value An integer value for initialization.
*/
ExampleClass(int value);
/**
* @brief Gets the value of the private member.
* @return The current value of the member.
*/
int getValue() const;
private:
int value; ///< Holds the integer value.
};
In this example:
- The `@class` tag identifies the class.
- The `@brief` tag provides a short description of the class.
- The `@param` tag describes the parameters an input function takes.
- The `@return` tag explains what the function returns.
- The private member `value` has an inline comment using `///<`, which is also helpful for quick references.
Documenting Variables and Enumerations
Enumerations are another essential part of C++ programming. Here’s an example of documenting an enum with Doxygen:
/**
* @enum Color
* @brief Represents the color options.
*/
enum Color {
RED, ///< Color red.
GREEN, ///< Color green.
BLUE ///< Color blue.
};
Using the `@enum` and `@brief` tags, the enumerations are thoroughly documented for clarity.
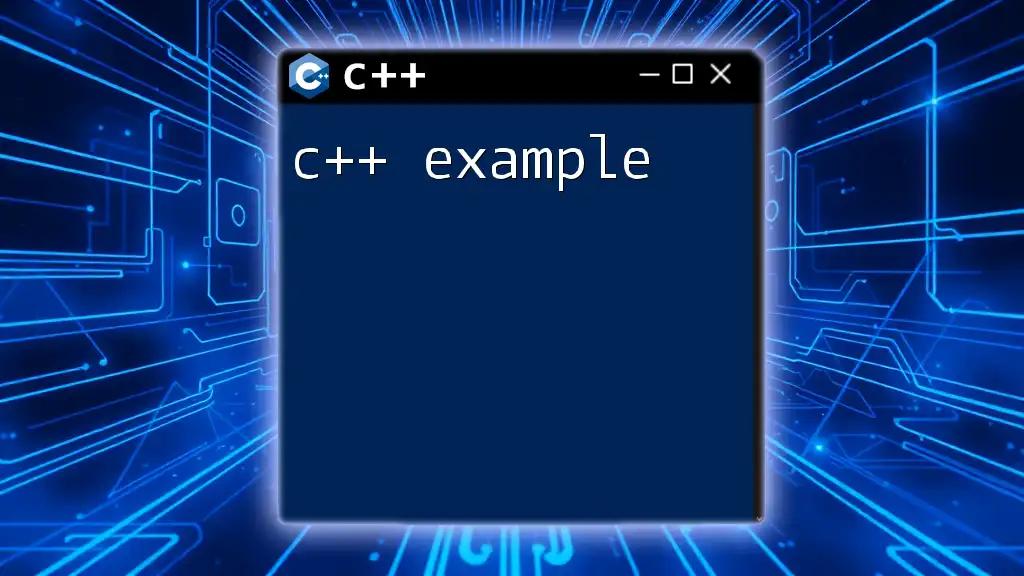
Generating Documentation with Doxygen
Running Doxygen
Once the comments are embedded in the source code, it’s time to generate the documentation. Navigate to the directory with your `Doxyfile` and run:
doxygen Doxyfile
This command reads the configuration file and creates formatted documentation based on your source code comments.
Understanding the Generated Output
Doxygen can produce documentation in various formats, including HTML and LaTeX. The HTML format is the most commonly used, as it provides a user-friendly interface for navigating through the generated documentation. You can explore the output files by opening the `index.html` file created in the output directory.
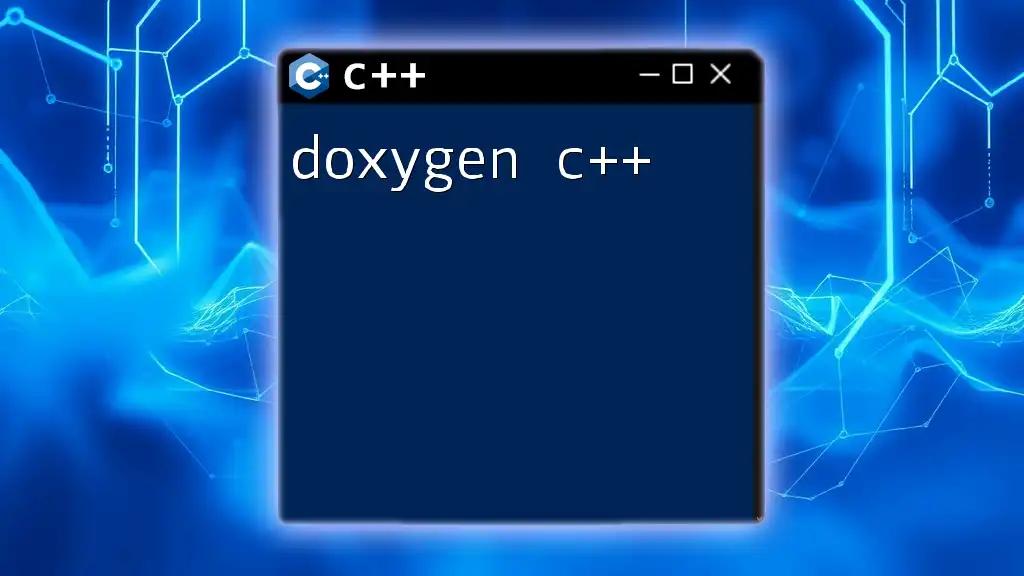
Advanced Doxygen Features
Using Graphical Representation with Graphviz
Doxygen can integrate with Graphviz to create visual representations of your code structure. This helps in understanding complex systems visually. Ensure Graphviz is installed and then enable it in the configuration file with:
HAVE_DOT = YES
With this feature enabled, you can include diagrams and call graphs in your documentation.
Customizing Doxygen Documentation
Customization options allow you to tailor the appearance and structure of the generated documentation. For example, you can change themes or layouts to better suit your project needs. Configuring modules and namespaces helps in organizing the documentation logically, making it easier to navigate.
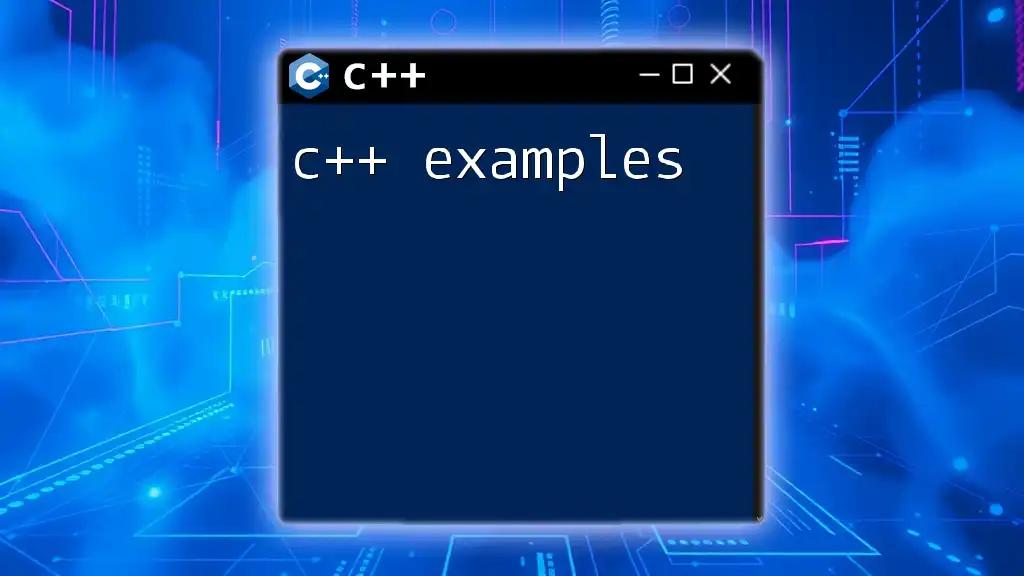
Best Practices for Doxygen in C++
Consistency in Documentation
Establishing a set of documentation conventions and adhering to them throughout your codebase is essential. Consistent use of tags and styles allows developers to quickly familiarize themselves with the documentation structure, enhancing efficiency.
Periodic Documentation Reviews
Don't let documentation become stale. Make it a practice to conduct regular reviews as your code evolves. This ensures that the documentation stays relevant and accurately reflects the current state of the codebase.
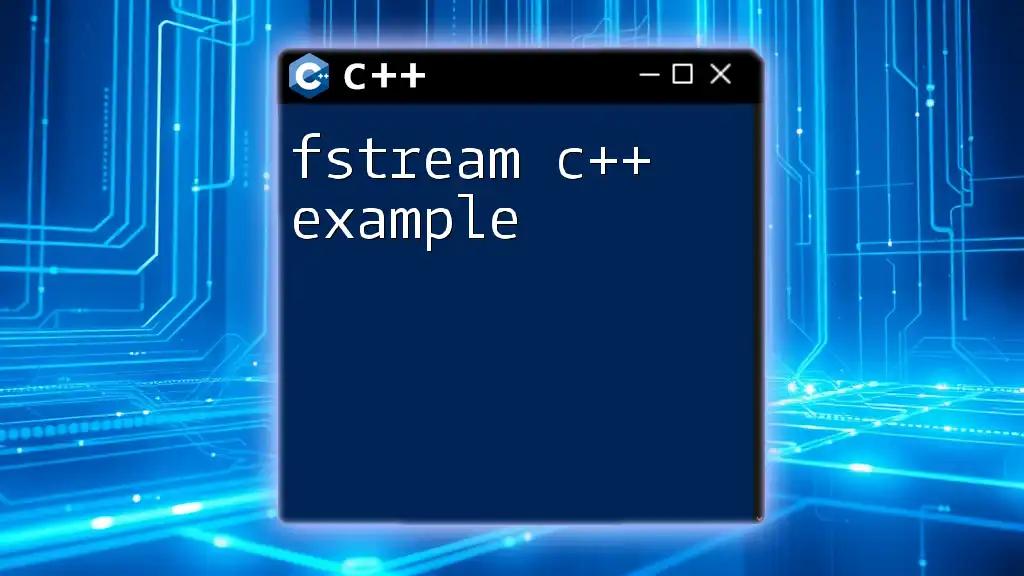
Conclusion
Using Doxygen as a documentation tool in your C++ projects not only streamlines the documentation process but also enhances code clarity and collaboration among developers. By following the techniques illustrated in this guide, you're well on your way to harnessing the full power of Doxygen for your C++ coding practices, ensuring an efficient and well-documented workflow for you and your team.
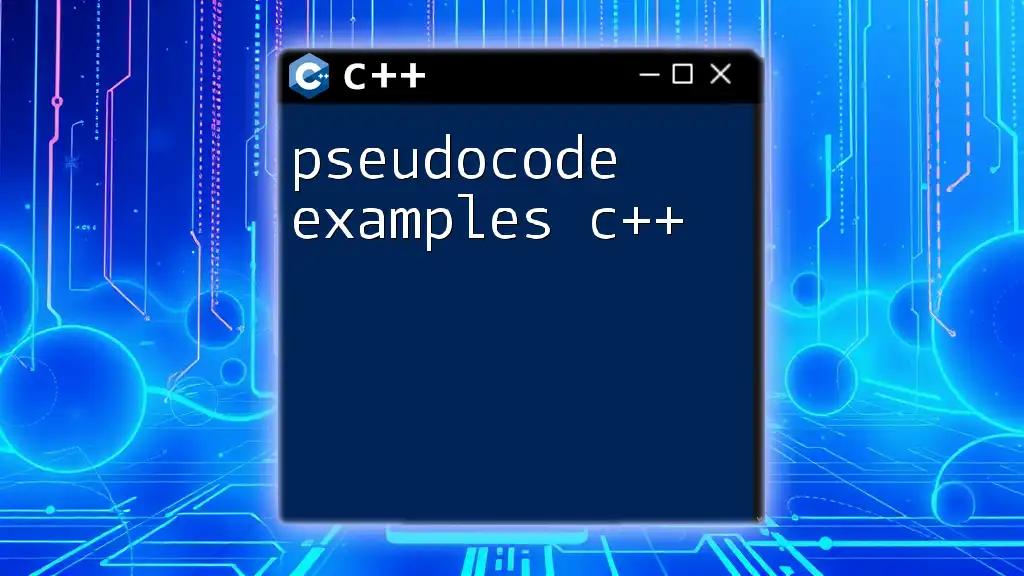
Additional Resources
To further enhance your Doxygen experience, consider referring to the official Doxygen documentation for in-depth features and advanced configurations. Engaging with C++ best practices guides and participating in forums and communities will also provide invaluable insights into optimizing your use of Doxygen in software projects.