In C++, the ampersand (`&`) is used as both a reference operator to create aliases for variables and as a bitwise AND operator in expressions.
Here’s a code snippet demonstrating its use as a reference:
#include <iostream>
void updateValue(int &ref) {
ref = 20; // modifies the original variable
}
int main() {
int original = 10;
updateValue(original);
std::cout << "Updated value: " << original << std::endl; // outputs 20
return 0;
}
What is the Ampersand in C++?
The ampersand symbol (`&`) holds significant importance in C++ programming. It serves dual purposes: as a reference operator and as part of various pointer operations. Understanding these functionalities is crucial for writing effective and efficient C++ code.
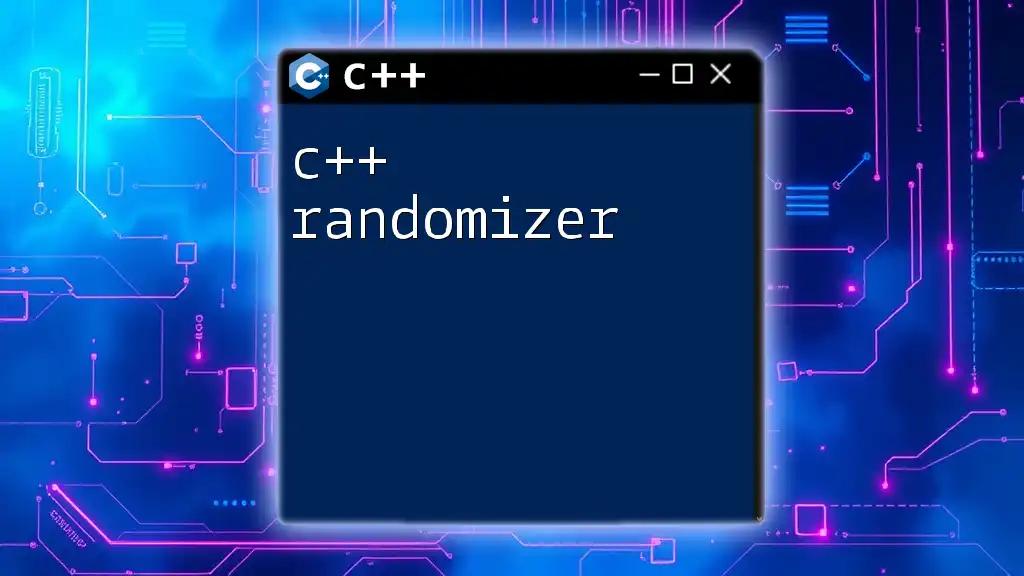
Uses of Ampersand in C++
Ampersand as a Reference Operator
In C++, the ampersand is primarily used to create references. A reference is essentially an alias for an existing variable, allowing you to access the variable without making a copy of it. This not only saves memory but also improves performance.
By declaring a reference with the ampersand, you bind a reference variable to an existing variable. For instance:
int x = 10;
int& refX = x; // refX is a reference to x
In this example, any changes made to `refX` directly affect `x` because they are both referring to the same memory location. Using references can simplify code, especially when dealing with larger data types or complex structures.
Ampersand in Pointer Context
The ampersand also plays a crucial role in working with pointers. While pointers store memory addresses, the ampersand fetches the address of a variable. This creates a powerful way to manipulate variables indirectly.
For example:
int y = 20;
int* ptrY = &y; // ptrY holds the address of y
In the code above, `ptrY` now contains the memory address of `y`. To alter the value of `y`, you can dereference `ptrY`:
*ptrY = 30; // Changes y to 30
Using pointers in combination with the ampersand allows for versatile programming techniques such as dynamic memory allocation and the creation of complex data structures.
Ampersand in Function Parameters
Pass by Reference
When utilizing the ampersand in function parameters, passing a variable by reference allows you to manipulate the original variable directly. This is highly efficient for large data types where copying would be resource-intensive.
Here’s how you can do it:
void increment(int& num) {
num++;
}
When calling this function, any changes to `num` inside the function directly impact the variable passed from the caller.
Pass by Address
Alternatively, you can pass variables by their memory addresses, utilizing pointers. This approach gives you control over the variable’s memory and allows modifications directly:
void reset(int* ptr) {
*ptr = 0;
}
When invoked, this function sets the integer pointed to by `ptr` to zero, demonstrating another layer of indirect manipulation.
Using Ampersand with the C++ Standard Library
Understanding the ampersand within the context of the C++ Standard Library (STL) can greatly enhance your coding skills. Many STL containers and algorithms rely heavily on references and pointers for optimal performance.
For example, when using vectors, references can help efficiently modify elements:
#include <vector>
#include <iostream>
void modifyVector(std::vector<int>& vec) {
vec.push_back(100);
}
int main() {
std::vector<int> myVec = {1, 2, 3};
modifyVector(myVec);
for(const auto &i : myVec) {
std::cout << i << " "; // Output: 1 2 3 100
}
return 0;
}
This code demonstrates passing a vector by reference, which allows the function `modifyVector` to update the original vector, showcasing the power of the ampersand in C++ programming.
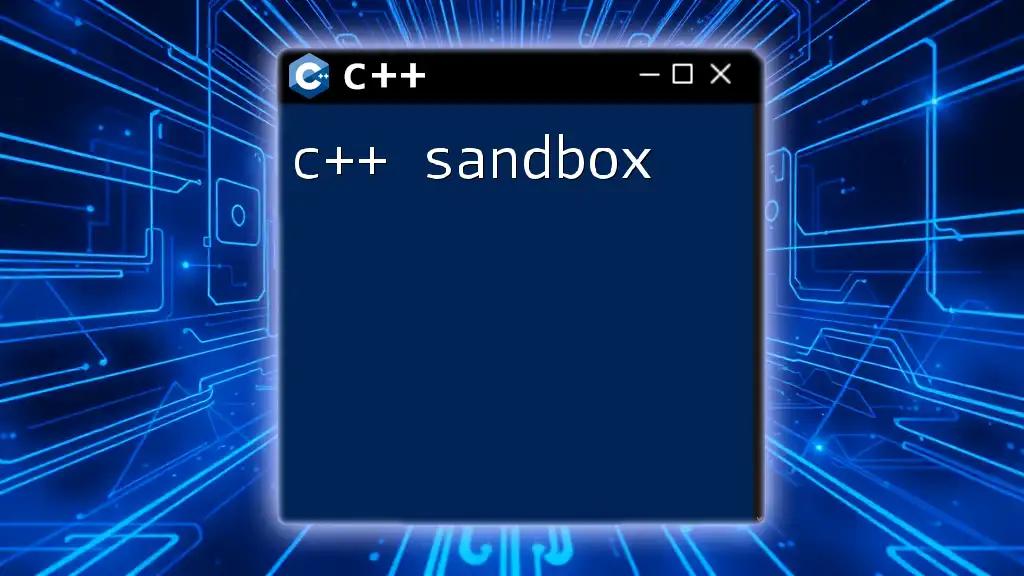
Common Mistakes to Avoid
Confusing Ampersands in Different Contexts
New programmers often confuse the ampersand's role, particularly when differentiating between references and pointers. Understanding that `&` can represent two core concepts—references and addresses—is essential.
Misusing References and Pointers
Another common pitfall involves the improper use of references and pointers. Failing to check for `nullptr` when dereferencing pointers can lead to runtime errors. It’s crucial to ensure that pointers are valid before attempting to dereference them.

Best Practices for Using Ampersand in C++
When working with the C++ ampersand, consider the following best practices:
- Prefer references over pointers wherever possible. References are safer and more elegant. They cannot be null, unlike pointers, which contributes to cleaner code.
- Use the ampersand judiciously in function parameters to balance performance with clarity.
- Always ensure pointers are initialized before use to avoid dereferencing `nullptr`.

Conclusion
Understanding the C++ ampersand is vital for mastering the language. It facilitates reference operations, pointer manipulations, and efficient function parameter handling. By deeply grasping these concepts, you will become a more proficient C++ programmer capable of writing optimized and clean code.

Additional Resources
Explore C++ documentation, recommended learning materials, and online coding platforms to further enhance your knowledge and skills.
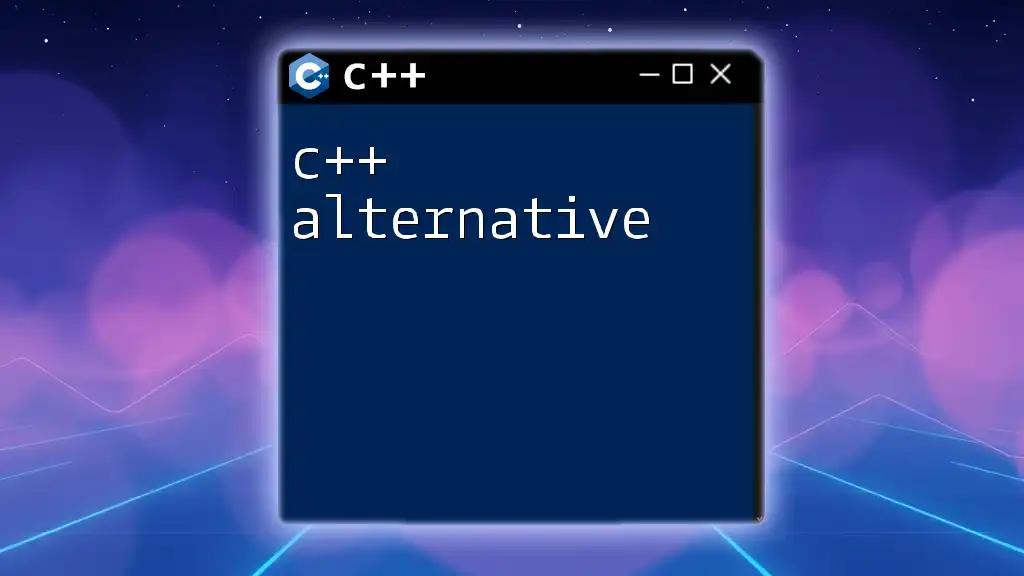
Call to Action
We encourage you to share your experiences with the C++ ampersand in the comments. Do you have questions or need clarification? Join the conversation!