In C++, the `srand()` function seeds the random number generator, allowing the `rand()` function to produce different sequences of random numbers on each program run.
Here's a code snippet to demonstrate seeding the random number generator in C++:
#include <iostream>
#include <cstdlib> // For srand() and rand()
#include <ctime> // For time()
int main() {
// Seed the random number generator with the current time
srand(static_cast<unsigned int>(time(0)));
// Generate and print a random number
std::cout << "Random Number: " << rand() % 100 << std::endl; // Random number between 0 and 99
return 0;
}
What is C++ Rand Seed?
A rand seed is a crucial concept in random number generation within C++. It refers to the initial value used by a random number generator to produce a sequence of pseudo-random numbers. When you change the seed, you change the sequence of numbers generated. This is key in applications requiring randomness, such as simulations and games.
The seeding process allows users to ensure that results can be reproduced when using the same seed. If you always start with the same seed, you will get the same sequence of results every time—this controllability is often desirable during debugging or testing.
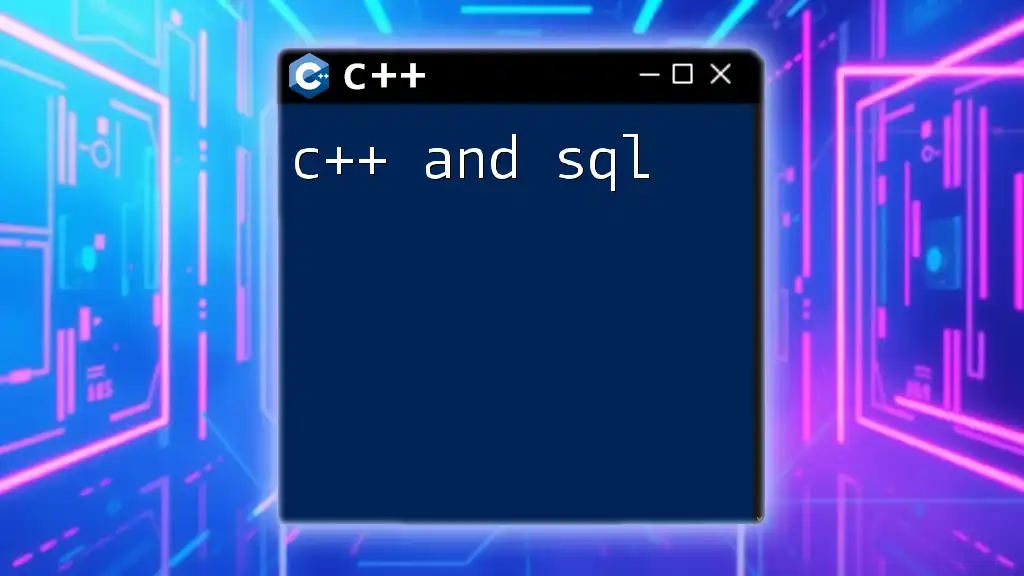
How the C++ Random Number Generator Works
In C++, the `rand()` function, provided by the `<cstdlib>` header, is commonly used for producing random integers. By default, the sequence generated by `rand()` starts at a pre-defined seed, which results in the same sequence being produced each time the program runs unless a different seed is set.
The Role of the Seed in Randomness
Seeding is important because it directly impacts how predictable the generated random numbers are. When you do not seed the random number generator, it uses a fixed internal seed, leading to a predictable sequence of random values. This predictability can be a problem in applications that require high levels of randomness, like games or cryptographic operations.
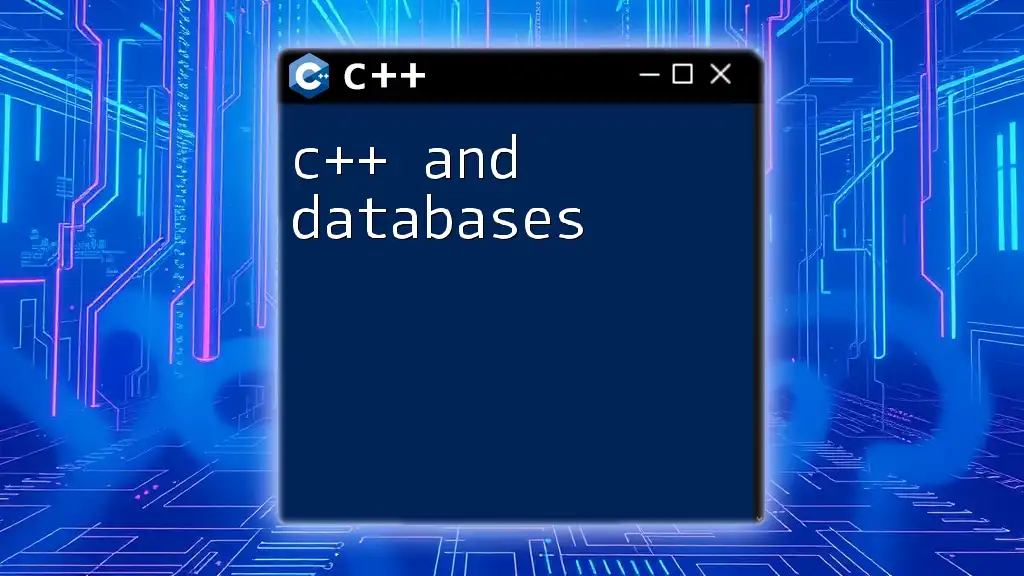
Using C++ Seed for Random Number Generation
Seeding the random number generator in C++ is done using the `srand()` function. This function sets the initial value (seed) for the sequence of pseudo-random numbers generated by `rand()`.
Seeding the Random Number Generator
Here’s an example of how you can introduce a seed in your code:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed the random number generator with the current time
std::cout << std::rand() << std::endl; // Generate a random number
return 0;
}
In the example above, `std::time(0)` provides the current time in seconds since the epoch, which ensures that a new seed (and hence a new sequence of random numbers) is used each time the program is run. This approach is very handy for ensuring different outputs in sequential runs.
Different Techniques for Seeding
Seeding with Time
Using time as a seed guarantees that outputs will vary with each execution of your program. This approach is straightforward and commonly used. The line `std::srand(std::time(0));` effectively guarantees that each run will have different results unless executed multiple times in rapid succession within the same second.
Custom Seed Values
Alternatively, you can set a fixed seed value intentionally for testing or debugging purposes. This can help you reproduce a specific set of results. For example:
std::srand(12345); // Fixed seed
for (int i = 0; i < 5; i++) {
std::cout << std::rand() << std::endl; // Will always produce the same sequence
}
By using a fixed seed like `12345`, you'll always produce the same output sequence each time your program runs, which is useful for debugging or performing consistent tests.
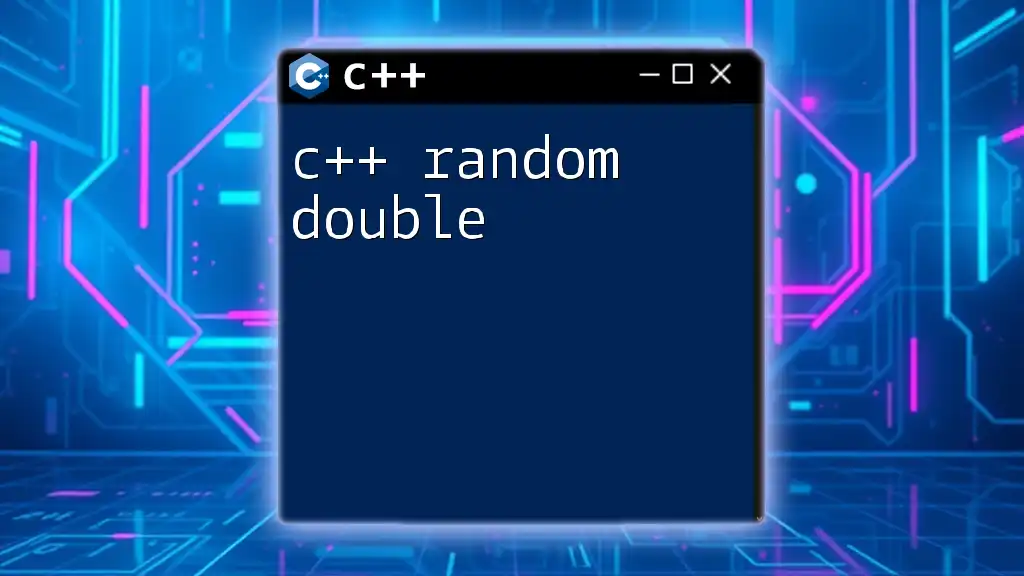
Alternatives to rand() and srand()
Since C++11, the `<random>` header has provided a superior alternative to the traditional `rand()` function. This modern random number generation system offers better randomness and more control over random distributions.
Introduction to C++11 Random Library
The new features found within the C++11 `<random>` library allow developers to specify not just the seed but also the type of distribution for the generated numbers. This improved flexibility is particularly advantageous for complex applications.
Using std::default_random_engine
Here’s how you can seed a random number generator using modern C++ with `<random>`:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator(std::time(0)); // Seed the engine
std::uniform_int_distribution<int> distribution(1, 100); // Specify a uniform distribution range
std::cout << distribution(generator) << std::endl; // Generate random number within range
return 0;
}
In this example, `std::default_random_engine` is seeded with the current time, while `std::uniform_int_distribution` specifies a range for the random number (from 1 to 100). This combination provides more useful control over random number generation, making it suitable for various applications.
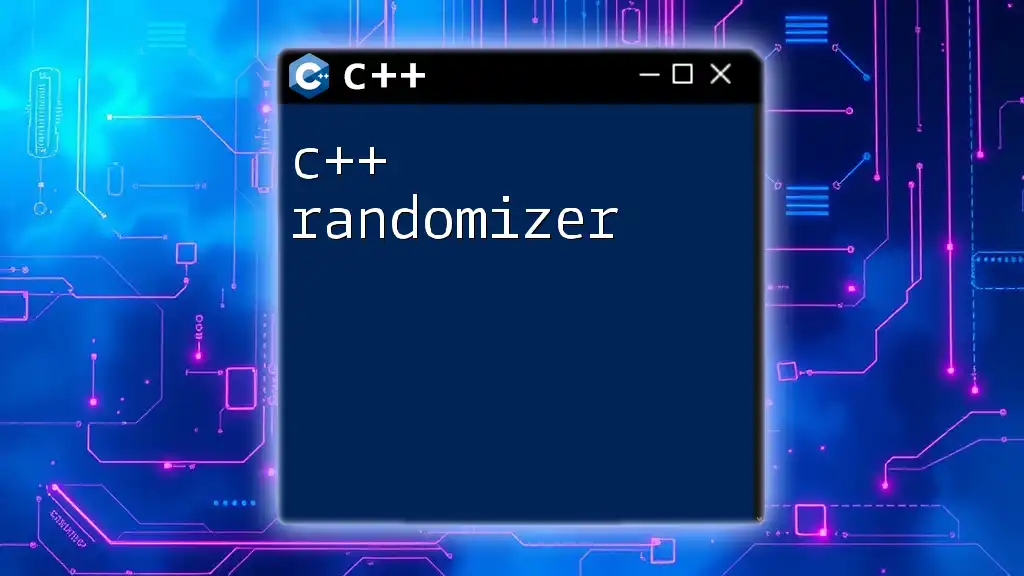
Best Practices for Seeding Random Number Generators
To leverage the power of randomness effectively, it’s essential to follow some best practices when it comes to seeding:
-
Common Pitfalls to Avoid
Avoid seeding the generator multiple times in a single program run. Seeding repeatedly with the same value can yield unexpected results. -
Using Non-Random Sources for Seeds
Always opt for sources with sufficient entropy, like system time, to ensure the seed is genuinely random.
When to Seed in your Programs
It’s best to seed your random number generator once at the beginning of your program. This ensures that all random numbers generated during that execution come from a single, coherent random sequence.
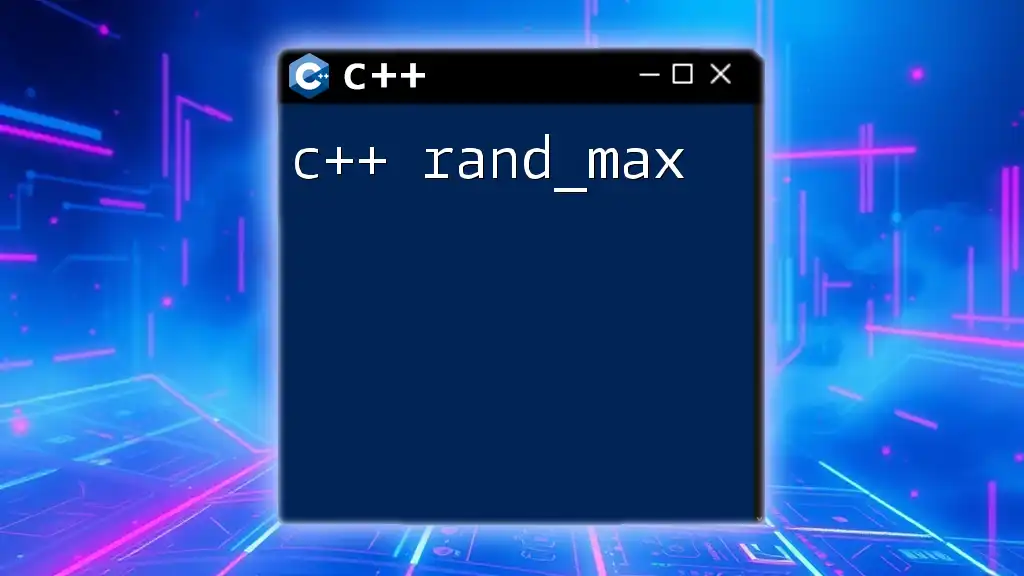
Conclusion
In this guide, we have explored c++ rand seed and its importance in navigating the complexities of random number generation. Understanding how to seed random number generators is essential for creating programs that require randomness, ensuring both reproducibility and unpredictability where necessary.
Feel encouraged to experiment with various seeding techniques and libraries in C++. As you begin to apply these concepts in practical scenarios, you'll gain a deeper understanding of randomness in programming and its vital role in various applications.