C++ can be used to interact with SQL databases by utilizing libraries such as SQLite or MySQL Connector, enabling developers to execute SQL commands directly from their C++ applications.
#include <mysql/mysql.h>
MYSQL *conn;
conn = mysql_init(NULL);
mysql_real_connect(conn, "localhost", "user", "password", "database", 0, NULL, 0);
mysql_query(conn, "SELECT * FROM table_name");
mysql_close(conn);
Understanding C++ and SQL
What is C++?
C++ is a powerful, high-level programming language that is widely used for system/software development, game programming, and performance-critical applications. Known for its flexibility and performance, C++ allows developers to write efficient code that can run close to the hardware. Key features of C++ include object-oriented programming, strong type checking, and rich function libraries, making it a robust choice for developers aiming to build complex systems.
What is SQL?
SQL, or Structured Query Language, is the standard language used to communicate with relational database management systems. It is essential for designing, querying, updating, and managing databases. SQL provides a systematic way to interact with data, enabling users to execute commands such as selecting, inserting, updating, and deleting records efficiently. Mastery of SQL is crucial for anyone looking to work with backend systems where data persistence and manipulation are key.
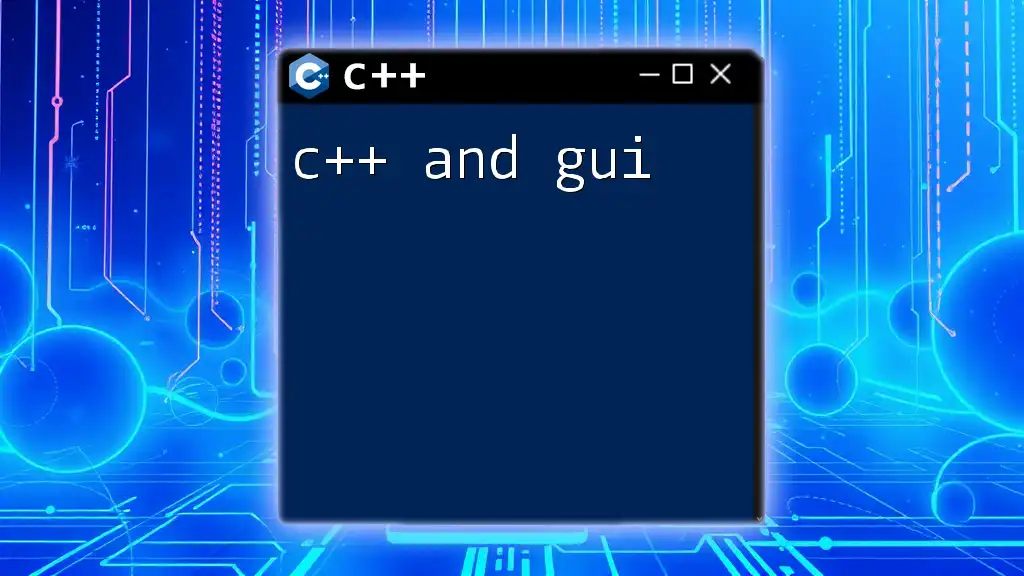
Why Combine C++ with SQL?
Benefits of Using C++ with SQL
Integrating C++ with SQL opens up a myriad of opportunities for developers. One of the primary advantages is performance optimization. C++ is renowned for its execution speed, which can be pivotal in applications requiring high efficiency, such as real-time data processing.
Furthermore, C++ enhances data manipulation capabilities. Using C++, developers can create powerful applications that handle complex logic while utilizing SQL for effective data storage. This combination allows for robust, performant solutions in various domains, including gaming, finance, and analytics.
Applications of C++ and SQL
Several real-world applications exemplify the power of merging C++ with SQL. In game development, C++ can be employed to manage game logic and computational tasks, while SQL efficiently handles player data and game states. In finance, applications can process large datasets in real-time using C++, while SQL facilitates transaction management and data retrieval. Similarly, data analytics software often leverages C++ for heavy computations and SQL for managing and querying large datasets.
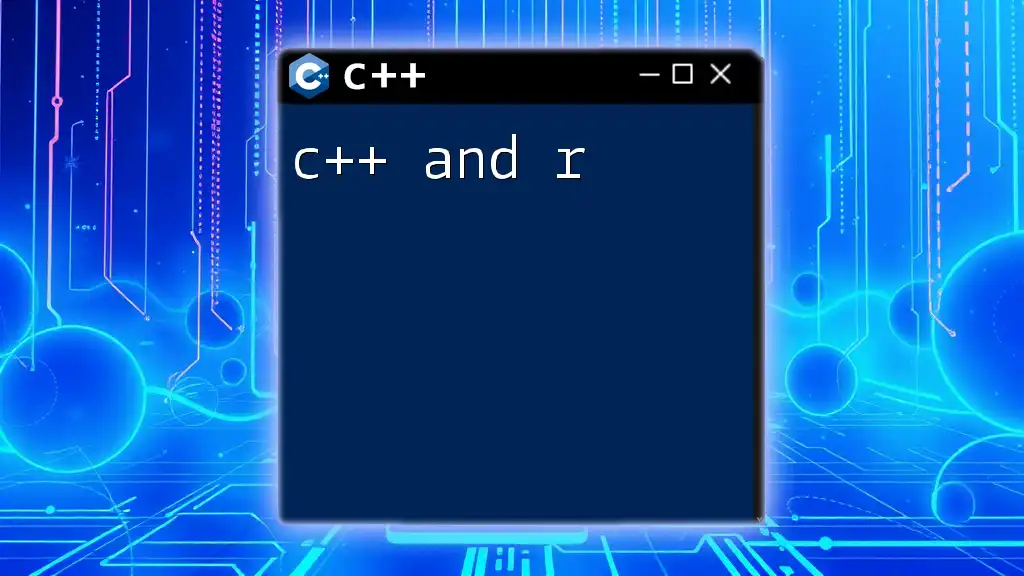
Setting Up Your C++ Environment for SQL
Required Tools and Libraries
To start integrating C++ with SQL, you need several essentials: a reliable C++ compiler (like GCC or Visual Studio), and a database management system, such as SQLite or MySQL. Depending on your choice of database, you may also require specific libraries to facilitate the connection and interaction between C++ and SQL. Popular options include SQLite’s C interface and MySQL Connector/C++.
Installing a Database
Getting your chosen database up and running is the next step. For SQLite, you can simply download precompiled binaries of the SQLite database. For MySQL, follow the installation guide provided on the official MySQL website. Make sure to set up the required folders and user permissions to allow your applications to connect to the database securely.
Compiling Your First C++ Project
Once the environment is set, you can write your first C++ project that connects to SQL. Here is an example code snippet that demonstrates how to set up a connection to MySQL:
#include <iostream>
#include <mysql/mysql.h>
int main() {
MYSQL *conn;
conn = mysql_init(NULL);
// Add error handling
if (conn == NULL) {
std::cerr << "mysql_init() failed" << std::endl;
return EXIT_FAILURE;
}
// Connect to database
if (mysql_real_connect(conn, "localhost", "user", "password", "database", 0, NULL, 0) == NULL) {
std::cerr << "mysql_real_connect() failed" << std::endl;
mysql_close(conn);
return EXIT_FAILURE;
}
std::cout << "Connected successfully!" << std::endl;
mysql_close(conn);
return EXIT_SUCCESS;
}
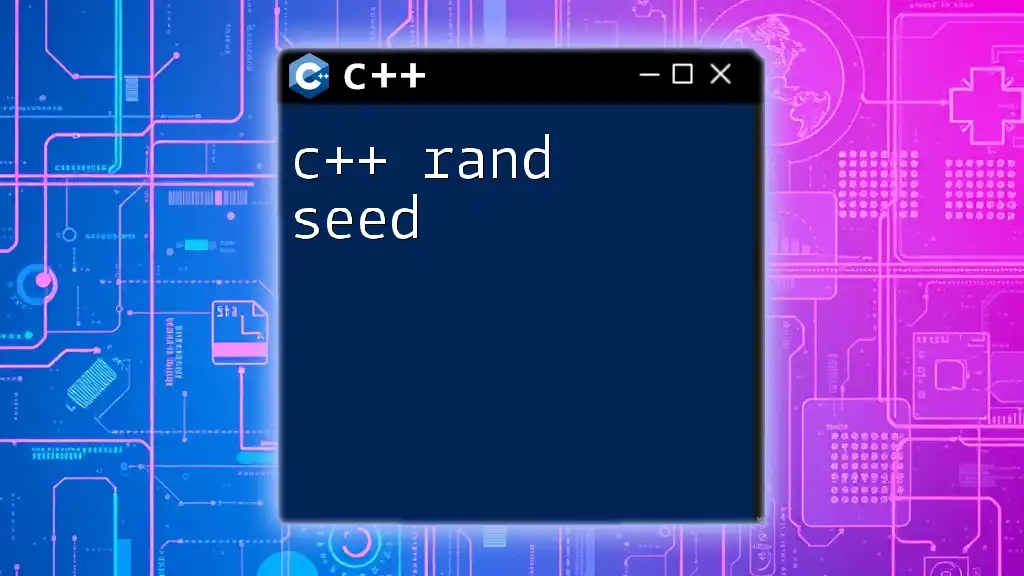
SQL Commands in C++
Basic SQL Commands
Selecting Data
To retrieve data from a database, the SELECT command is employed. This powerful command allows you to specify which columns to fetch and from which tables. Below is an example demonstrating how to execute a SELECT query using C++:
std::string query = "SELECT * FROM table_name";
if (mysql_query(conn, query.c_str())) {
std::cerr << "SELECT failed: " << mysql_error(conn) << std::endl;
}
By executing this code, you can retrieve all entries from the specified table. Remember to handle any potential errors that may arise during the execution.
Inserting Data
The INSERT command is vital for adding new records to a database. Here is an example of how to insert data using C++:
std::string insert_query = "INSERT INTO table_name (column1, column2) VALUES ('value1', 'value2')";
if (mysql_query(conn, insert_query.c_str())) {
std::cerr << "INSERT failed: " << mysql_error(conn) << std::endl;
}
The above code snippet demonstrates how straightforward it is to insert new rows into a table using SQL commands from within a C++ application.
Advanced SQL Techniques in C++
Joins and Relationships
Understanding joins is crucial for efficient data retrieval from multiple tables. You can perform several types of joins, such as INNER JOIN and LEFT JOIN, to combine rows from different tables based on related columns. Below is a basic example of an INNER JOIN in C++:
std::string join_query = "SELECT a.column1, b.column2 FROM table_a AS a INNER JOIN table_b AS b ON a.id = b.foreign_id";
if (mysql_query(conn, join_query.c_str())) {
std::cerr << "JOIN failed: " << mysql_error(conn) << std::endl;
}
By utilizing joins, you can deepen your data analysis capabilities.
Transactions
Transactions are essential for maintaining data integrity, especially when performing multiple related operations. Here’s how you can use transactions in C++:
mysql_query(conn, "START TRANSACTION");
// Perform your SQL operations
mysql_query(conn, "COMMIT");
If any error occurs during your operations, you can easily roll back to maintain consistency in the database.
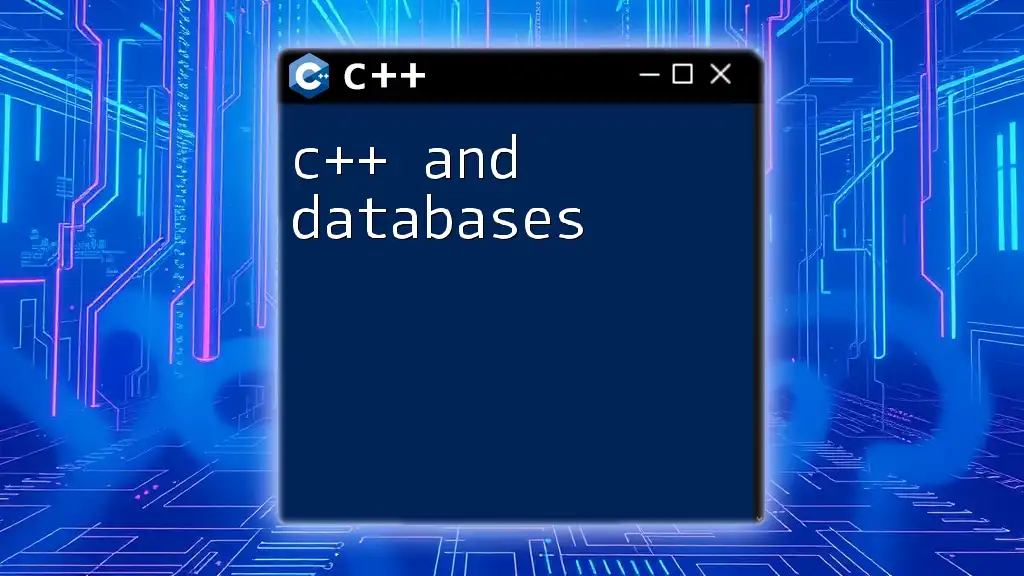
Error Handling in C++ and SQL
Common Issues and Solutions
When working with C++ and SQL, error handling is paramount. Common issues might include connection timeouts or syntax errors in SQL queries. It is crucial to always check the return values of your SQL operations. For instance:
if (mysql_query(conn, query.c_str())) {
std::cerr << "Query failed: " << mysql_error(conn) << std::endl;
}
This simple error-handling technique ensures that any issues can be identified and addressed quickly.
Debugging Tips
Effective debugging is key to a successful development process. Utilize tools such as gdb for C++ to step through your code while examining the values of variables during execution. Additionally, enabling SQL query logging in your database can help trace problems in your queries.
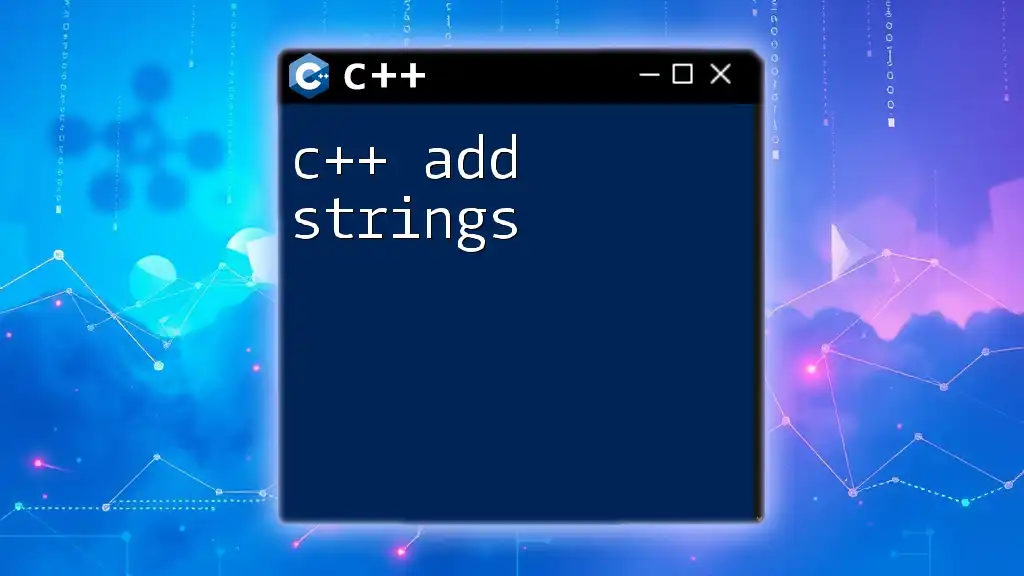
Conclusion
Integrating C++ and SQL brings powerful capabilities to your applications, allowing for efficient data management and optimized performance. As you explore the fascinating intersection of these technologies, remember to focus on mastering the essential tools and libraries, as well as adeptly applying SQL commands within your C++ projects. The combination of these two languages opens up countless possibilities for data-driven applications, and the journey towards mastering them promises to be rewarding.
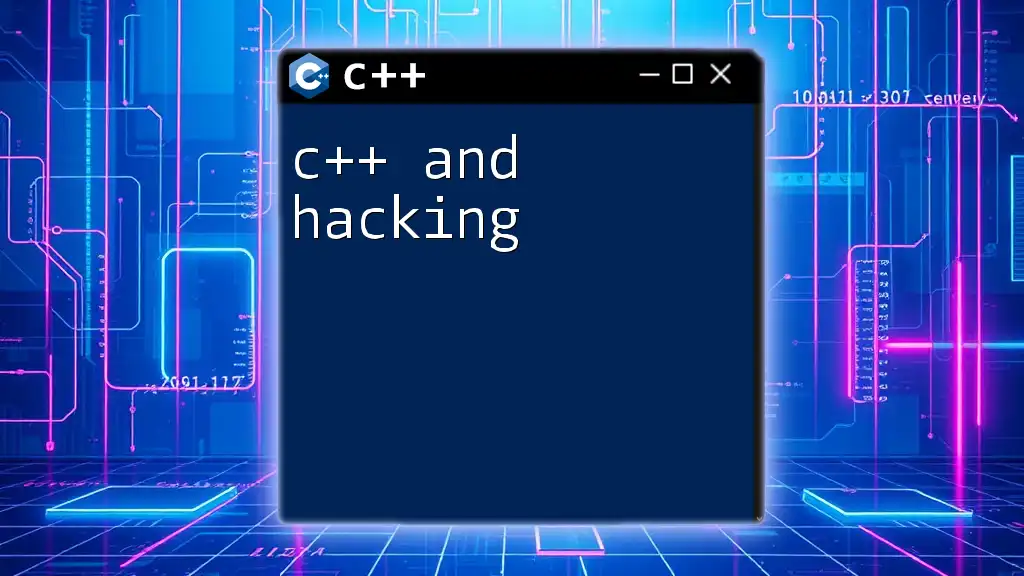
Additional Resources
For further exploration of C++ and SQL, consider checking out authoritative books, enrolling in online courses, and consulting documentation provided by database vendors. Engaging with online communities dedicated to these technologies can also enhance your learning experience and provide invaluable support as you develop your skills.