C++ is a powerful programming language that can be utilized on Raspberry Pi for various projects, enabling users to control hardware and create applications efficiently.
Here’s a simple example of using C++ to blink an LED connected to a Raspberry Pi:
#include <wiringPi.h>
#include <iostream>
int main() {
wiringPiSetup(); // Initialize wiringPi
pinMode(0, OUTPUT); // Set GPIO pin 0 as output
while(true) {
digitalWrite(0, HIGH); // Turn LED on
delay(1000); // Wait for 1 second
digitalWrite(0, LOW); // Turn LED off
delay(1000); // Wait for 1 second
}
return 0;
}
Getting Started with C++ on Raspberry Pi
Setting Up Your Raspberry Pi
To begin your journey with C++ and Raspberry Pi, you first need to set up your Raspberry Pi device correctly.
Installing Raspberry Pi OS
- Download the Raspberry Pi Imager from the official Raspberry Pi website.
- Insert an SD card into your computer. Use the imager to flash Raspberry Pi OS onto the card.
- Insert the SD card into your Raspberry Pi and power it on. Follow the setup instructions on the screen to complete your initial configuration.
Setting Up Your Development Environment
Once your Raspberry Pi is ready, you'll want to set up the development environment suitable for programming in C++.
- You can install Visual Studio Code for a comprehensive experience with extensions for C++ development.
- Alternatively, you can opt for terminal-based editors like nano or vim for a lightweight option.
- To compile C++ code, install the GNU C++ compiler by running this command:
sudo apt install g++
Writing Your First C++ Program
Now that your development environment is set up, you can write your first C++ program to confirm that everything is working properly.
Creating a Simple Hello World Application
Open your text editor and create a new file named `hello.cpp`. Enter the following code:
#include <iostream>
int main() {
std::cout << "Hello, Raspberry Pi!" << std::endl;
return 0;
}
In this program:
- `#include <iostream>` allows you to include the Input Output Stream library needed for output (such as printing to the console).
- The `main` function is the entry point of your C++ application, where the code execution begins.
- `std::cout` is used to output text to the console, and `std::endl` ends the line.
To compile and run your program, execute the following commands in your terminal:
g++ hello.cpp -o hello
./hello
If everything is set up correctly, you should see the output: Hello, Raspberry Pi!
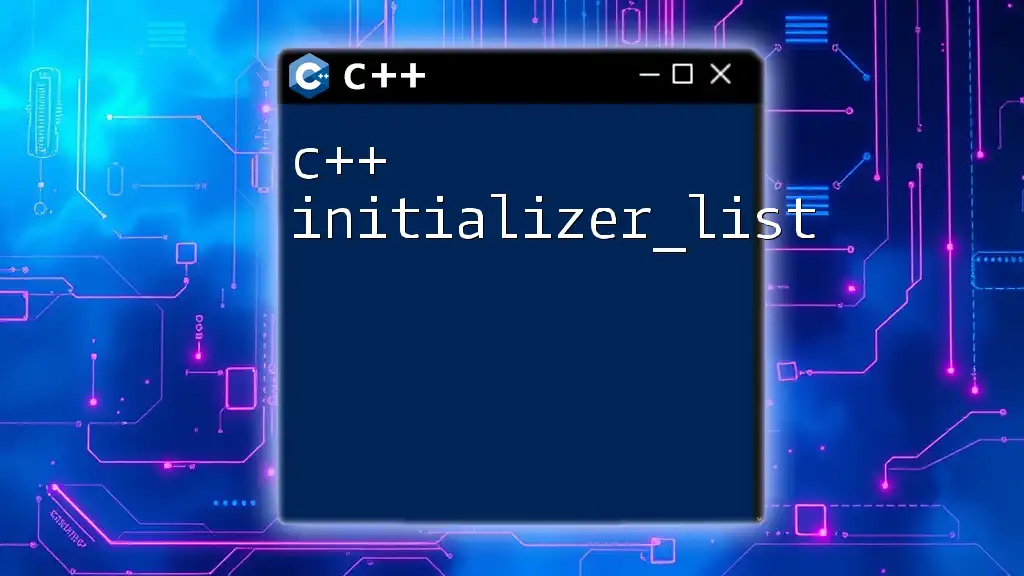
Advantages of Using C++ for Raspberry Pi Projects
C++ offers numerous advantages, especially when working on Raspberry Pi projects:
Performance and Efficiency
Speed: C++ is a compiled language, which allows programs to run faster than interpreted languages like Python. This performance boost is particularly beneficial for resource-constrained environments like the Raspberry Pi.
Resource Management: C++ gives you unparalleled control over system resources, including memory management. This is crucial when developing applications requiring efficient performance in real-time systems or embedded development.
Rich Libraries and Frameworks
C++ supports a wide range of libraries tailored for Raspberry Pi development. For example, WiringPi and pigpio make interfacing with GPIO pins straightforward and efficient, allowing you to harness the capabilities of your Raspberry Pi effectively.
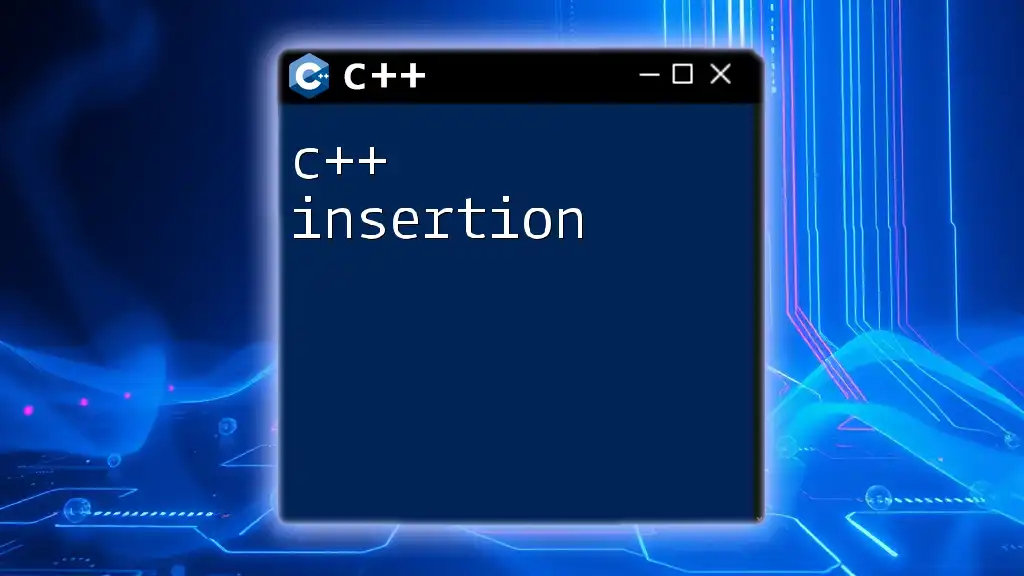
Setting Up Your C++ Development Environment on Raspberry Pi
Choosing an IDE or Text Editor
Choosing the right IDE or text editor can have a significant impact on your productivity as a C++ developer on Raspberry Pi.
- For beginners, Geany offers a lightweight, user-friendly interface while providing essential features like code completion.
- If you prefer a more advanced setup, Visual Studio Code includes excellent extensions for C++ coding, debugging, and Git version control.
Compiling and Running C++ Programs
Using the command line to compile C++ programs is essential for effective development on Raspberry Pi.
Using g++ Command Line
After writing your C++ program, use the g++ command to compile it. For example, to compile `hello.cpp`, you would run:
g++ hello.cpp -o hello
Here, `-o hello` specifies the output executable name. Following compilation, you can execute your program with:
./hello
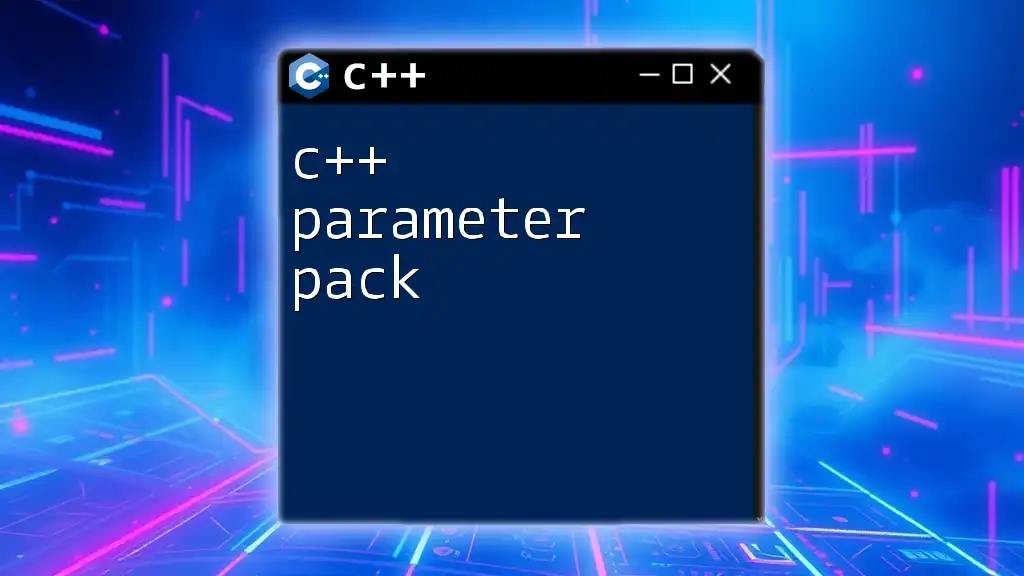
Exploring Hardware Interfacing with C++
GPIO Programming with C++
General Purpose Input/Output (GPIO) programming is a vital aspect of working with Raspberry Pi, enabling interaction with different electronic components.
Introduction to GPIO
Understanding GPIO is fundamental for creating IoT projects, such as controlling LEDs, motors, and sensors. Each GPIO pin can be configured as either an input or an output.
Using WiringPi with C++
One of the most popular libraries for GPIO programming in C++ is WiringPi. To install it, use:
sudo apt-get install wiringpi
Example Code for Blinking an LED
Here’s a simple program to blink an LED using WiringPi:
#include <wiringPi.h>
#include <iostream>
int main() {
wiringPiSetup(); // Initialize WiringPi
pinMode(0, OUTPUT); // Set pin 0 as output
while(true) {
digitalWrite(0, HIGH); // Turn LED on
delay(1000); // Wait 1 second
digitalWrite(0, LOW); // Turn LED off
delay(1000); // Wait 1 second
}
return 0;
}
Code Explanation:
- `pinMode(0, OUTPUT)` sets the GPIO pin 0 as an output.
- The `while(true)` loop continuously blinks the LED on and off every second using `digitalWrite` and `delay`.
Sensor Interfacing with C++
Interfacing different sensors with your Raspberry Pi using C++ adds tremendous functionality to your projects.
Overview of How to Connect Sensors
Common sensors like temperature and humidity sensors (e.g., DHT11) can be easily interfaced. You will typically use I2C or SPI protocols for communication.
Example Reading Sensor Data
After setting up the sensor's library, you can read values using C++. Here’s a hypothetical example of reading temperature data:
#include <DHT.h>
// Define sensor attributes
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
int main() {
dht.begin(); // Initialize the sensor
while(true) {
float h = dht.readHumidity(); // Read humidity
float t = dht.readTemperature(); // Read temperature
// Print values
std::cout << "Humidity: " << h << "%, Temperature: " << t << "°C" << std::endl;
delay(2000); // Wait for 2 seconds
}
return 0;
}
Note: Don’t forget to install the necessary libraries for the sensor you choose!
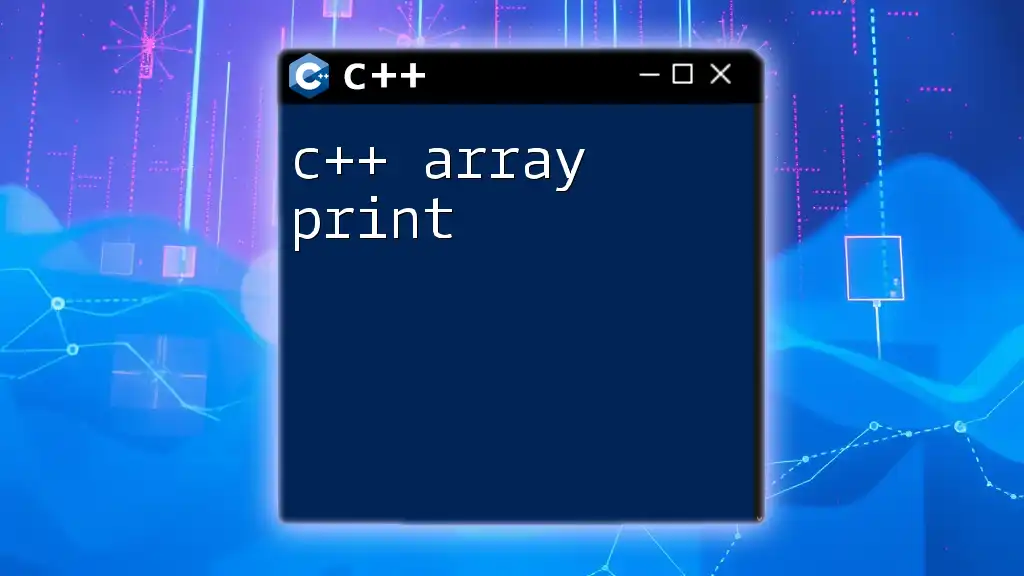
Projects You Can Build with C++ on Raspberry Pi
Home Automation System
Creating a home automation system can be incredibly rewarding. Using C++, you can control lights, monitor temperatures, or even manage security systems—all from a Raspberry Pi.
Robotics and C++
C++ is an excellent choice for developing robotics projects on Raspberry Pi. Its performance and efficiency meet the demands of real-time control systems.
Example Project: Building a Line-Following Robot
For this project, you can use IR sensors to detect the line and control motors based on sensor input. Integrating C++ allows you to create a responsive and efficient control algorithm.
Media Center using C++
Building a media center involves not just programming but also creative design. With C++, you can efficiently manage playback of various media files and develop a user-friendly interface for navigating through your media library.
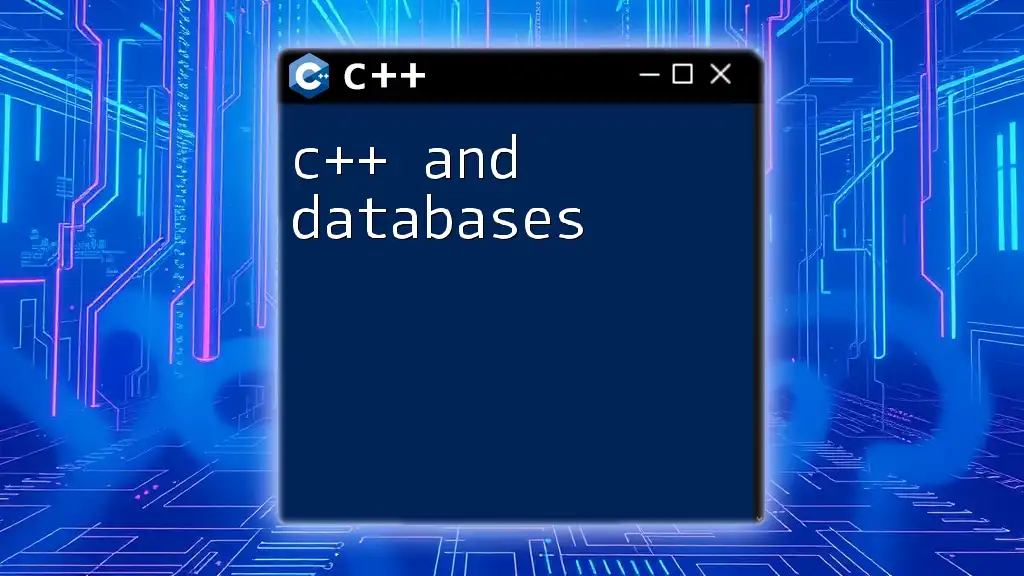
Troubleshooting Common Issues
Common Setup Problems
While setting up your environment to program C++ on Raspberry Pi, you might encounter some standard issues, such as:
- Header file not found errors: This usually means the library isn’t installed or configured correctly.
- GPIO permissions issues: If you find that a GPIO pin won’t respond, you might need to run your program with `sudo` for permission.
Debugging C++ Code on Raspberry Pi
When debugging C++ code, make use of tools like gdb (GNU Debugger). It can help you step through your code, monitor variables, and isolate issues effectively.
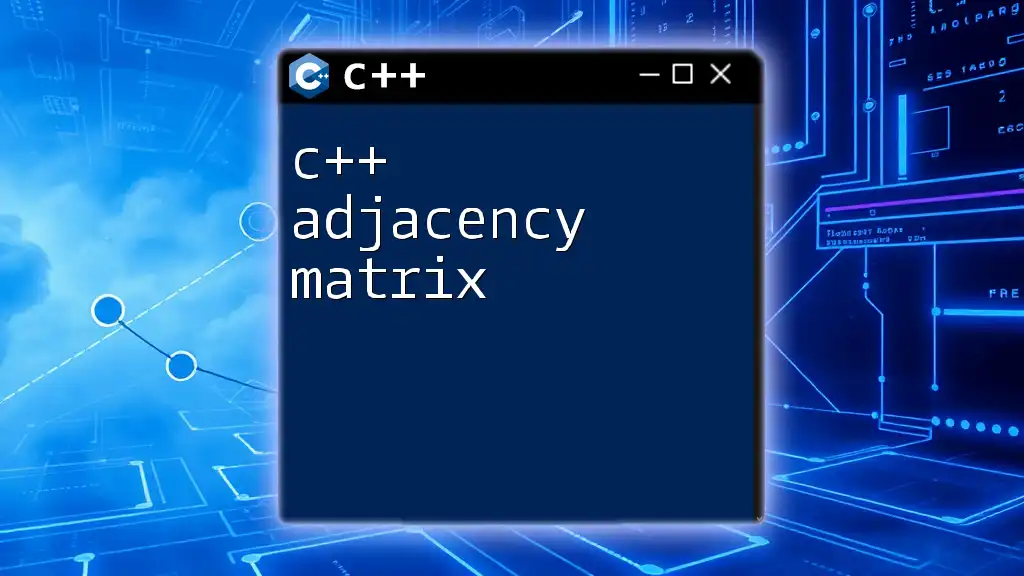
Conclusion
Using C++ and Raspberry Pi opens up a world of possibilities in programming and hardware interaction. This powerful combination allows you to build innovative projects, from simple LED controls to complex robotics and home automation systems. The skills you develop while mastering C++ programming on Raspberry Pi will serve you well in various applications in computing and engineering.
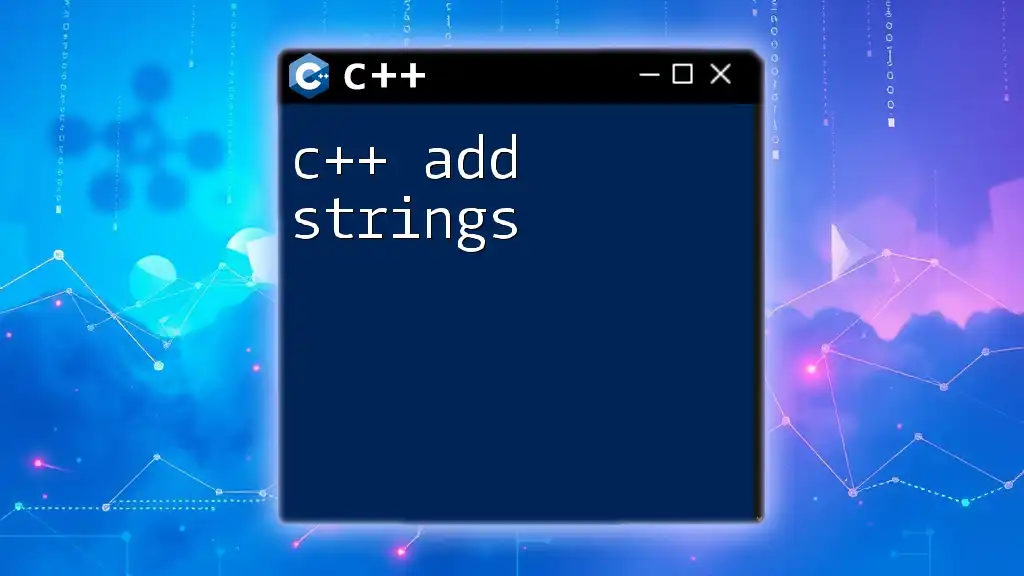
Additional Resources
To continue your education in C++ and Raspberry Pi development:
- Explore online tutorials that take a deep dive into specific topics.
- Engage with forums where like-minded developers share their projects.
- Consider investing time in recommended books and online courses that focus on advanced C++ programming techniques and their applications on Raspberry Pi.
By arming yourself with these resources, you’ll be well-prepared to tackle increasingly complex projects and become proficient in utilizing C++ and Raspberry Pi effectively.