The C++ Redistributable is a package required to run applications developed with Microsoft Visual C++, as it installs necessary runtime components like libraries and DLLs needed by the application.
Here's an example of how to check for the presence of the C++ Redistributable in your C++ code:
#include <iostream>
#include <Windows.h>
int main() {
if (IsDebuggerPresent()) {
std::cout << "C++ Redistributable is installed!" << std::endl;
} else {
std::cout << "C++ Redistributable not found." << std::endl;
}
return 0;
}
What is C++ Redistributable?
C++ redistributable packages are critical components designed to ensure that applications developed using C++ can run on different devices without the need for the users to manually install the necessary libraries and support files. These packages contain runtime components of Visual C++ libraries, which an application built with Visual Studio might depend on.
Importance: Without the appropriate C++ redistributable, users may encounter run-time errors when attempting to launch applications, making it essential to understand how to properly manage and distribute these packages in your projects.

Why You Need C++ Redistributables
C++ redistributables function as a bridge, allowing an application to access specific libraries it was compiled against. The benefits include:
- Ease of Use: Users do not need to understand the technical details; they can simply install the redistributable to run your app.
- Version Management: Developers can focus on their code without worrying if the user has the right libraries.
- Reduced Errors: Helps in minimizing runtime errors that arise from missing components.

Understanding C++ Redistributables
Components of C++ Redistributables
C++ redistributables include various libraries and runtime files that an application utilizes to operate smoothly. Key components include:
- Libraries: These files contain pre-written code that applications can call upon to perform common tasks.
- Runtime Files: These files are necessary for the execution of C++ applications, ensuring they can run effectively within the operating environment.
Different Versions of C++ Redistributables
Microsoft Visual C++ Redistributables come in various versions, each associated with different releases of Visual Studio. Here are some of the common versions:
- 2005: Widely used for applications created with Visual Studio 2005.
- 2008: Introduced enhancements and security updates.
- 2010: Provided support for new features in the C++ language.
- 2015-2019: Combined redistributables, allowing compatibility across multiple versions.
- 2022: The latest version, optimized for modern application needs.
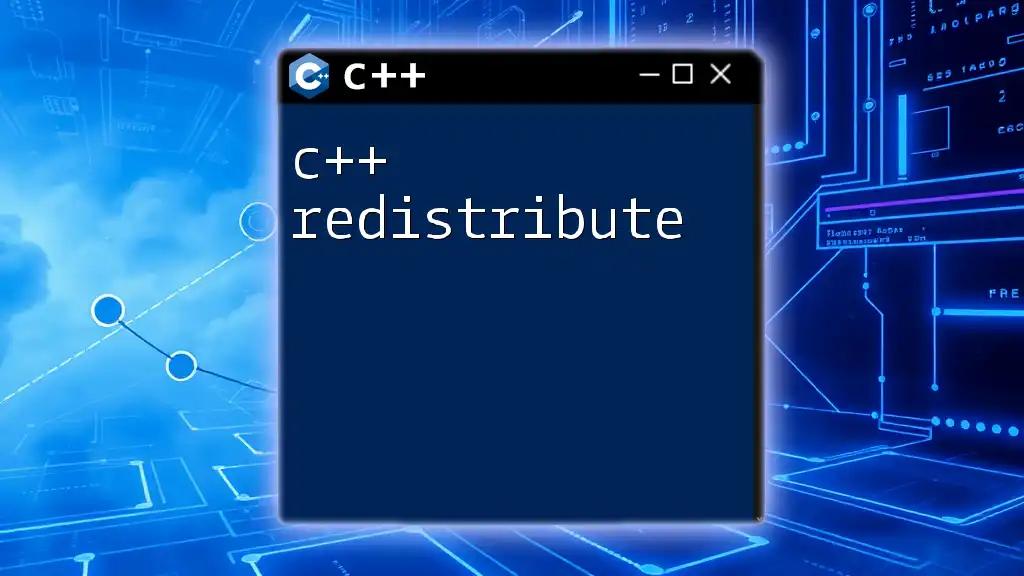
Installation of C++ Redistributables
How to Download C++ Redistributables
Downloading the correct C++ redistributable is crucial. You can find the official packages on the Microsoft website. Here’s how:
- Visit the official Microsoft Visual C++ Redistributable download page.
- Select your version based on the dependencies needed for your application.
- Download the appropriate x86 or x64 version, depending on your target architecture.
Installation Process
Installing the C++ redistributable is straightforward:
- Locate the downloaded installer on your system.
- Run the installer by double-clicking it.
- Follow the on-screen instructions to complete the installation.
Common Installation Issues: Sometimes, users might face issues during installation, such as permission problems. Be sure to run the installer as an administrator to avoid these issues.

Working with C++ Redistributables
Identifying Required Redistributables
Determining which redistributable your application needs can be done through tools like Dependency Walker or by checking your project’s references in Visual Studio.
Distributing C++ Redistributables with Your Application
When distributing your application, it’s often best to include the necessary redistributable packages. Here's an example code snippet for setting up an installer that includes the redistributable:
// Pseudo-code for a setup script
InstallRedistributable("vcruntime140_x64.exe");
InstallYourApplication("MyApp.exe");
By ensuring that the runtime libraries are included with your application installer, you create a smoother user experience.
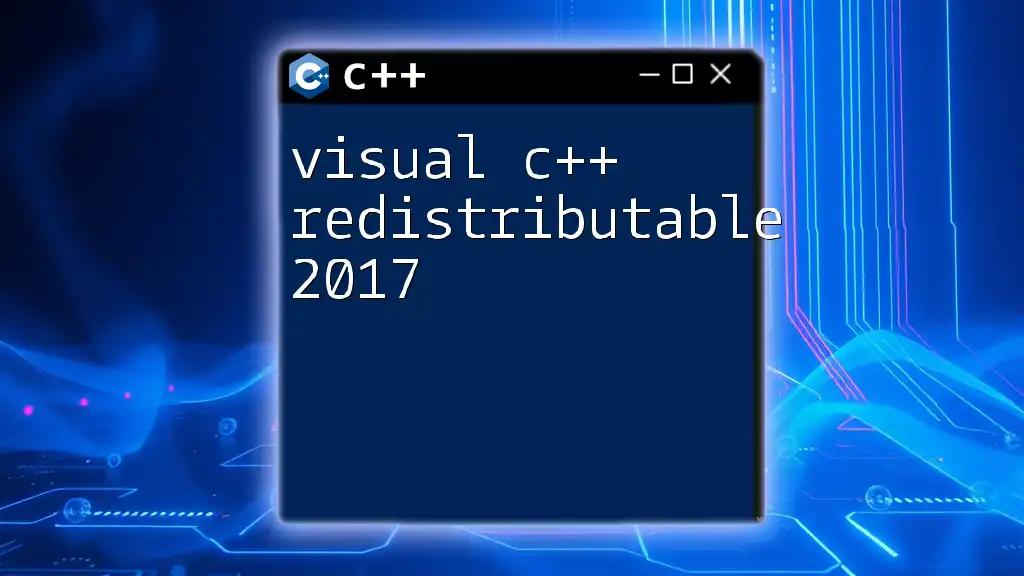
Common Issues and Solutions
Error Messages Related to C++ Redistributables
One of the common error messages users encounter is:
"The program can't start because MSVCR.dll is missing."
This indicates that the necessary C++ runtime library is not present. To resolve this:
- Identify the missing DLL.
- Download and install the corresponding version of the C++ redistributable.
- Restart the application.
Resolving Compatibility Issues
Managing multiple versions of redistributables can become complex. To ensure compatibility:
- Always check the application requirements.
- Use an installer that ensures the correct redistributable is downloaded based on the system specifications.
- If possible, aim for the latest redistributable version as they are often backward compatible.

Advanced Topics
Custom Build of C++ Redistributables
In some cases, you may want to create a customized build of C++ redistributables. This may involve:
- Configuring your project settings to link against the required libraries.
- Building the application in Release mode to generate optimized binaries.
One example scenario would be when you're creating a specialized version for a particular system configuration.
Using Redistributables in Cross-Platform Development
For developers targeting multiple operating systems, managing redistributables can be challenging. It’s important to:
- Layer your project architecture to accommodate different environments.
- Use preprocessor directives to check the target environment and adjust the application’s requirements accordingly:
#ifdef _WIN32
// Code specific for Windows
#elif __linux__
// Code specific for Linux
#endif
This approach enhances compatibility and eases the deployment process across different platforms.
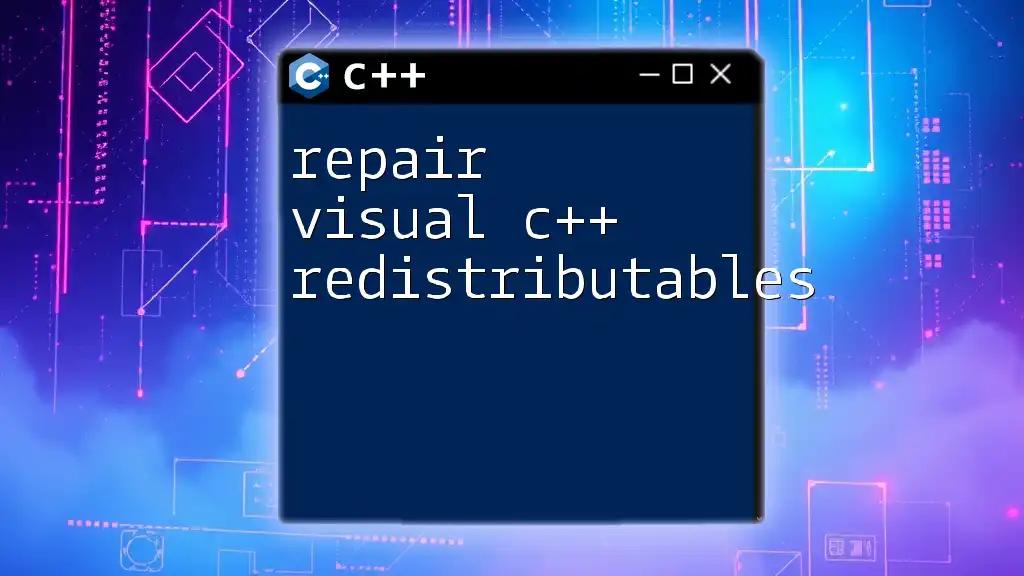
Conclusion
Understanding C++ redistributables is essential for both developers and users. Properly managing these components ensures a smoother experience while reducing runtime errors associated with missing libraries. By following best practices in identification, distribution, and installation, you can significantly enhance the usability of your C++ applications.
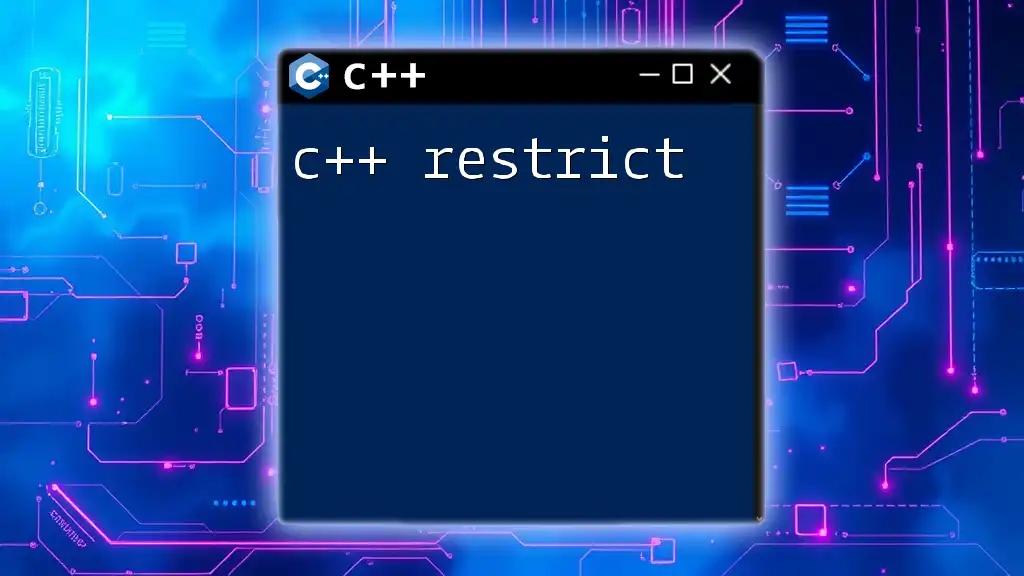
Additional Resources
For further reading and more comprehensive understanding, check the official Microsoft documentation, and explore community forums for shared experiences and solutions. Recommended books and online courses can provide deeper insights into both C++ and redistributable management, helping you become proficient in tackling related challenges.