A Redistributable C++ refers to a package that installs the necessary runtime components required to run applications developed with Microsoft C++, ensuring that all dependent libraries and files are available on the user's machine.
Here's a simple example of a C++ program that uses the standard input/output library:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Redistributable Packages
What are Redistributable Packages?
Redistributable packages are essential components for deploying applications that rely on specific libraries or frameworks. These packages provide shared libraries, DLLs (Dynamic Link Libraries), and other resources needed for applications to function correctly on a user’s machine. The core purpose of redistributable packages is to encapsulate necessary resources that an application may need, thus simplifying the installation process for end-users.
When you develop software using C++, it often relies on the C++ Standard Library and additional libraries that are part of a specific compiler's ecosystem. By distributing these libraries with a redistributable package, developers ensure that end-users do not face issues related to missing components and can run the software seamlessly.
Difference between Redistributable Packages and Static Linking
While redistributable packages offer significant benefits, it is essential to differentiate them from static linking.
- Redistributable Packages: These require the installation of shared libraries on the user's system. Applications dynamically link to these libraries at runtime, which means they rely on the presence of the libraries installed.
- Static Linking: In contrast, static linking includes the library code directly within the application executable. This approach increases the initial size of the application but eliminates the need for users to install additional components.
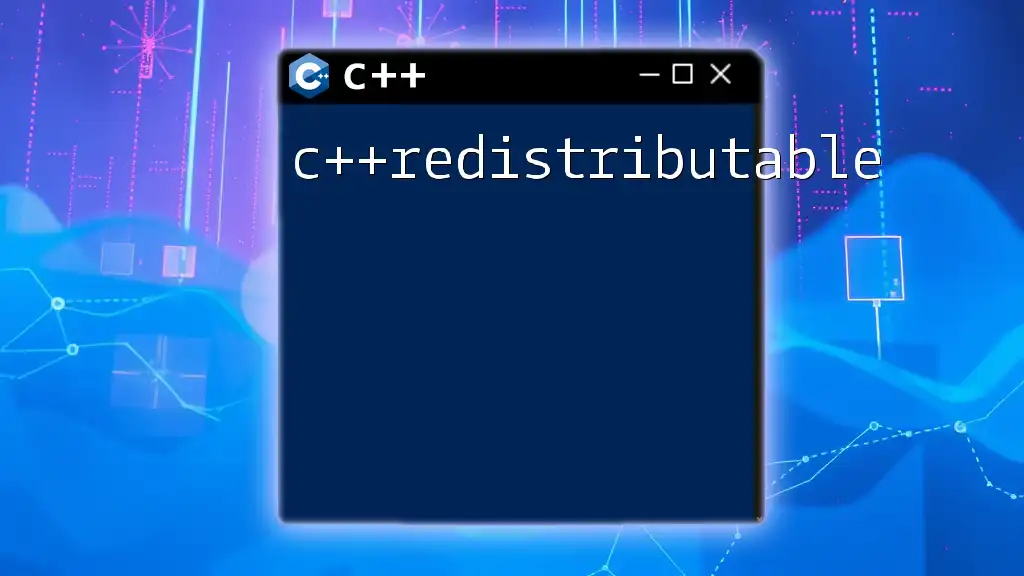
Overview of Microsoft Visual C++ Redistributable
Versions and Compatibility
Microsoft Visual C++ redistributables are perhaps the most widely recognized redistributable packages in C++ development. Various versions of these redistributables correspond to different versions of Visual Studio (e.g., 2015, 2017, 2019, and beyond). Each version is designed to be backward compatible with applications built using their respective compilers.
Importance of Microsoft Redistributables
Using the correct Microsoft Visual C++ redistributable ensures that your application has access to the right runtime libraries. If your application is built using Visual Studio 2019, for example, it will require the Visual C++ 2019 Redistributable on the target machine. Failure to provide the necessary runtime libraries can lead to runtime errors, preventing your application from functioning correctly.
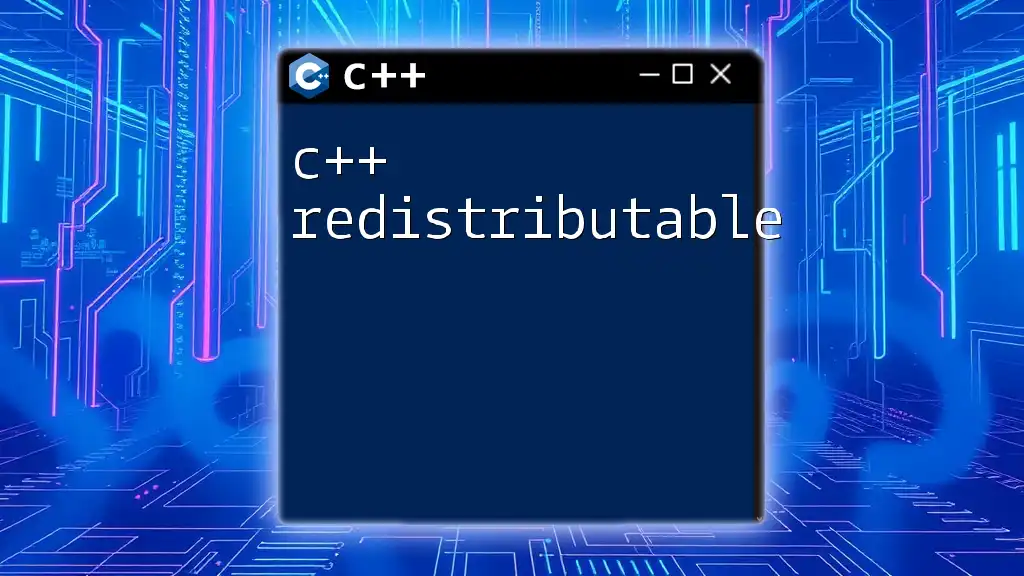
Role of Redistributable C++ in Application Deployment
How Redistributable C++ Affects Application Distribution
Including redistributables in your application deployment strategy is paramount. By using these packages, you can ensure your end-users have the required components for your application to run smoothly. This reduces installation size and complexity, as you do not need to bundle all the necessary libraries with your application. Instead, you can rely on users having the redistributable already installed or guiding them to install it as part of your application's setup process.
Case Studies of Application Deployment
Example 1: A Simple Console Application
Consider a simple console application developed with Visual Studio. Without redistributables, the application might fail to run on a user's machine due to missing dynamic libraries. By providing the Visual C++ Redistributable as part of your installation package, you ensure the necessary libraries are available, improving user experience and adoption rates.
Example 2: Complex GUI Application
In scenarios involving complex GUI applications, the importance of redistributables grows. A GUI application might rely on various libraries for functionalities like graphics rendering or user interface controls. By packaging the appropriate redistributables, you facilitate smooth application performance and allow users to focus on using the application rather than troubleshooting installation issues.
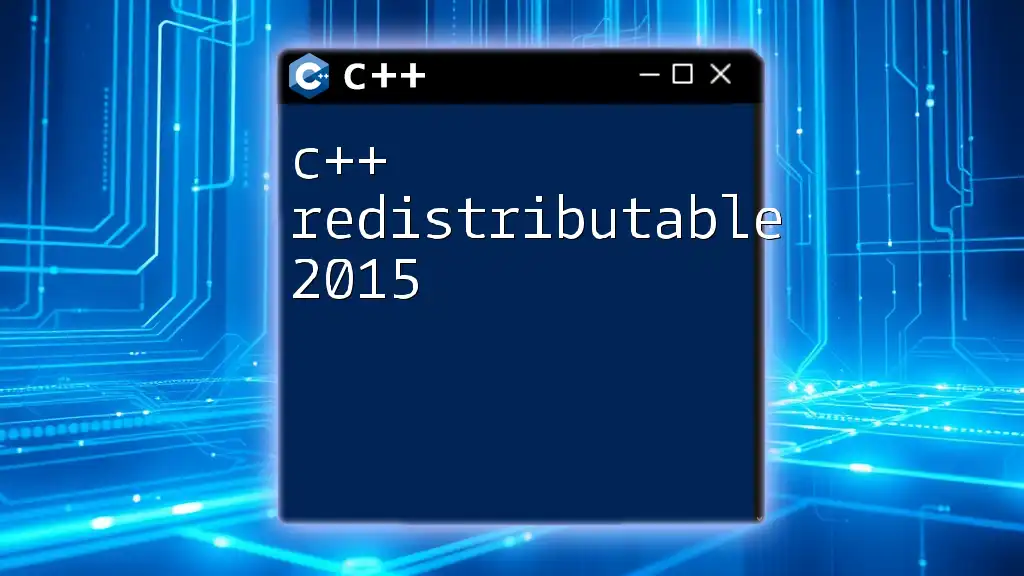
Installing Redistributable C++
Downloading Redistributables
The first step in using redistributable C++ is downloading the appropriate packages. You can find the relevant versions on the official Microsoft website. Ensuring you download from official sources minimizes the risk of installing outdated or potentially malicious software.
Installation Process
Once you have downloaded the redistributable package, the installation process is straightforward:
- Run the installer: Double-click the downloaded file.
- Follow the prompts: The installation wizard typically guides you through the necessary steps.
- Verify installation: After installation, check for any errors during the process to ensure the package installed correctly.
Troubleshooting Common Installation Issues
While installing redistributables is often trouble-free, issues can arise. Common problems include incompatible versions or missing administrative rights. If users encounter difficulties, ensure that they:
- Have administrative privileges to install software.
- Download the correct architecture (32-bit vs. 64-bit) based on their system and application requirements.
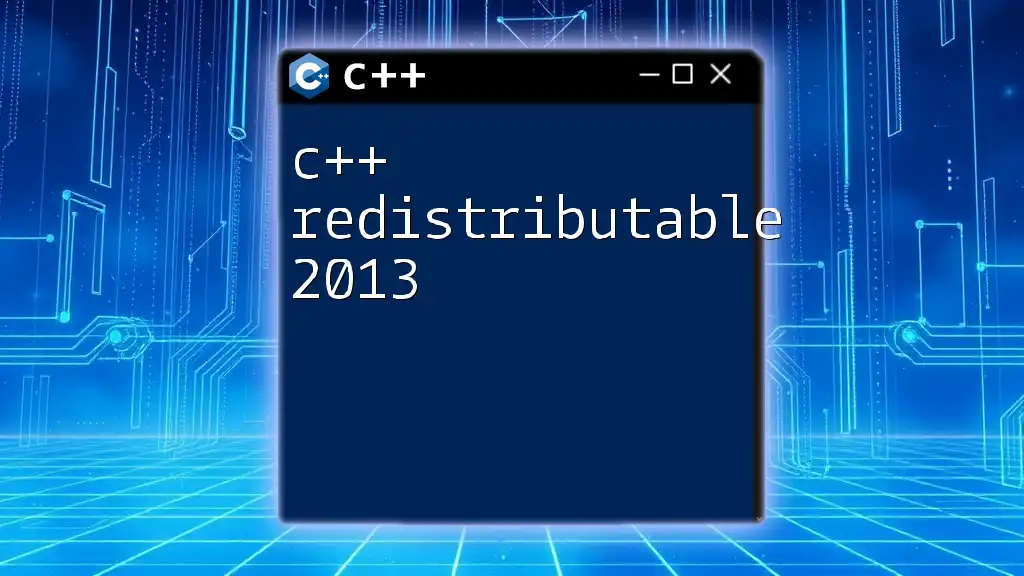
Managing Redistributable C++ Versions
Importance of Version Management
Version management is critical when your application relies on redistributable C++. Developers must acknowledge the difference between versions installed on user systems, as using an incorrect version can lead to conflicts or errors.
How to Check Installed Versions
To ensure the proper version is installed, use command-line tools to verify installed redistributable packages. Running the following command can help in diagnostics:
wmic product where "name like 'Microsoft Visual C++%'" get name, version
This command retrieves a list of installed Visual C++ redistributables and their respective versions.
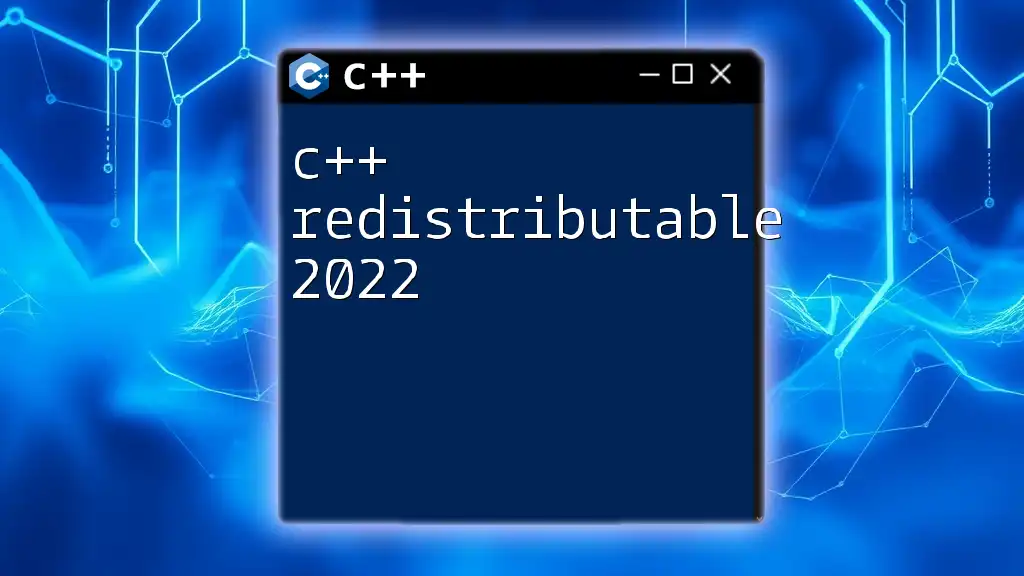
Best Practices for Using Redistributable C++
Recommendations for Developers
To maximize the efficiency of redistributables within your applications, keep the following best practices in mind:
-
Always include Redistributable Check in Your Installer: Implement checks within your installer that verify if the necessary redistributable packages are installed.
-
Testing Your Application with Different Redistributable Versions: Rigorously test your application against various installation scenarios to catch potential issues with redistributable packages early on.
Example Code Snippet: Handling Missing Redistributables
Handling missing redistributables gracefully can improve user experience. Here’s a simple routine to implement in your C++ application:
if (IsRedistributableInstalled())
{
// Continue with application execution
}
else
{
ShowMessage("Please install the required Redistributable Package.");
}
This snippet checks if the required redistributable is installed and prompts users to install it if not, guiding them through the process.
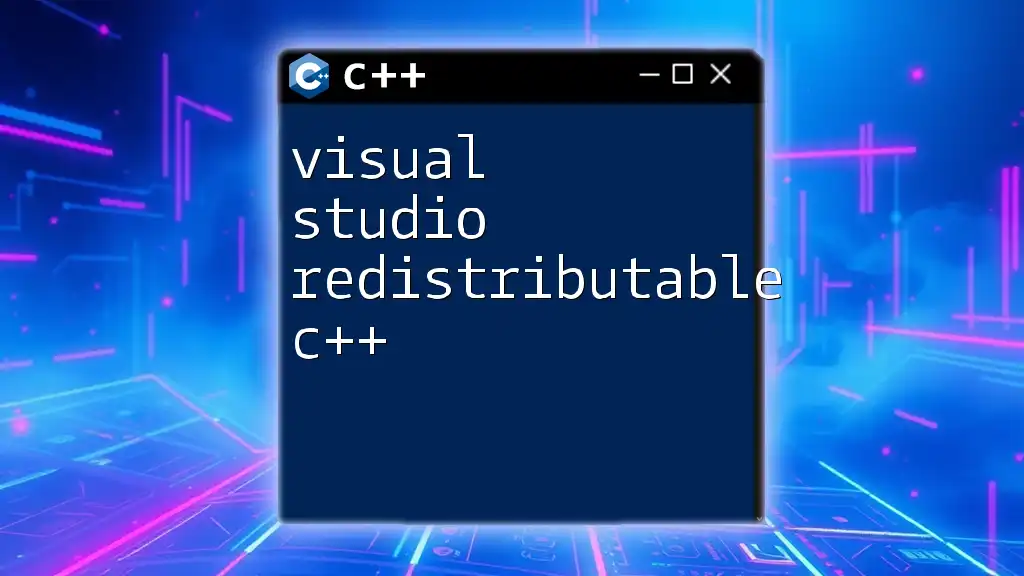
Redistributable C++ for Different Architectures
Understanding 32-bit and 64-bit Distributions
Architecture compatibility is significant when working with redistributables. Knowing whether your application is 32-bit or 64-bit impacts which redistributable packages need to be included.
Compatibility Considerations
Developers must ensure that they deliver the correct architecture version of the redistributable package along with their applications. A 32-bit application will not run on a 64-bit-only redistributable without the appropriate libraries.
Download Links for Different Architectures
You can find both 32-bit and 64-bit versions on the Microsoft download page. Ensure you specify the correct version in your deployment documentation to prevent user confusion.
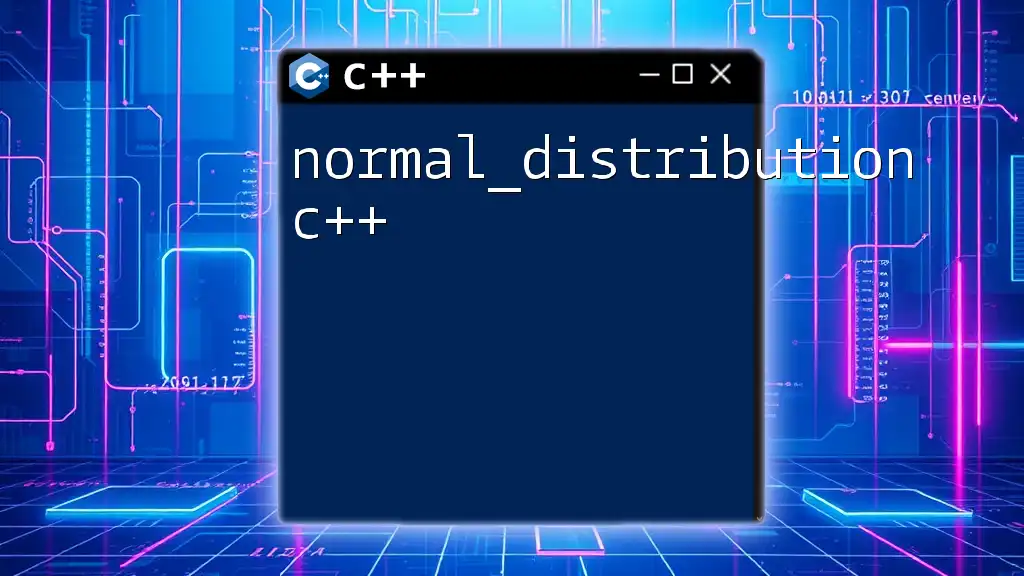
Common Issues with Redistributable C++
Typical Problems Encountered
Even with the best practices, end-users may experience issues related to redistributables, such as:
- Missing DLL Files: This often indicates that the redistributable package did not install correctly or is absent.
- Version Mismatch Errors: Applications may behave unexpectedly if running against the wrong version of a redistributable.
Strategies for Troubleshooting
Have a solid troubleshooting plan in place that includes:
- Identifying the Problem: Empower users to collect error logs or screenshots that reveal what went wrong during installation or execution.
- Solutions for Each Common Issue: Provide guidance for how to resolve issues like those mentioned above, including steps to reinstall redistributables.
- Logging and Reporting Errors: Encourage users to report their experiences to help improve your application and the installation process.
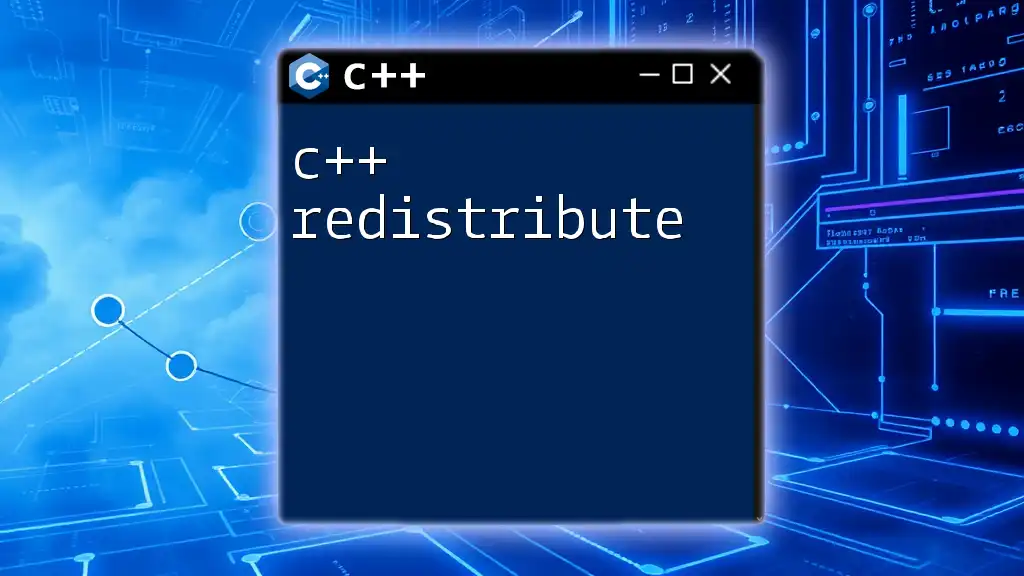
Advanced Topics in Redistributable C++
Static vs. Dynamic Linking
When deciding between static and dynamic linking for your C++ application, consider your project requirements. Static linking embeds the library directly into the application, yet often results in larger executable sizes. However, it removes the dependency on redistributable packages, simplifying deployment.
When to Use Each Approach
Use dynamic linking when:
- You want to share common code across multiple applications.
- You want to reduce installation size.
Opt for static linking when:
- You're developing a single-user application that won’t require updates frequently.
- You need to simplify the installation process entirely for the end-user.
Creating Your Own Redistributable Package
For projects with specific dependencies, you might create custom redistributable packages. However, consider the following best practices:
- Documentation: Ensure all dependencies are well-documented for users.
- Testing: Rigorously test your custom package on different environments to ensure compatibility.
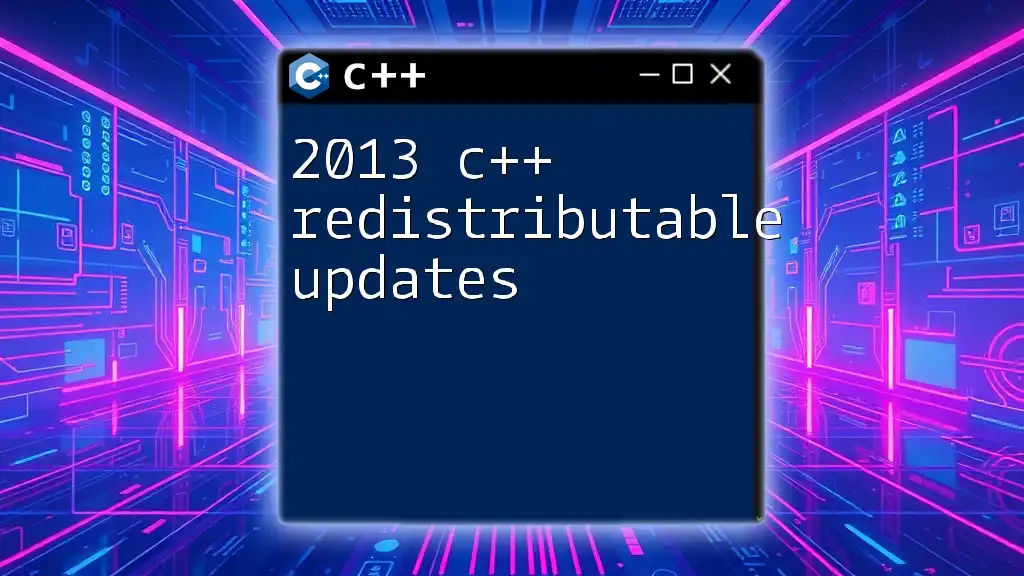
Future of Redistributable C++
Trends in Redistributable C++ Development
As development trends evolve, the use of redistributables is also changing. Developers are increasingly adopting frameworks that allow cross-platform development, which may reduce the need for specific redistributable packages. However, C++ will remain a robust language requiring careful management of dependencies.
The Evolution of C++ Standards and Its Effects on Redistributables
The C++ standards continue to evolve, leading to new libraries and practices related to redistributables. Keeping an eye on these changes ensures you remain prepared to adapt your applications to be compliant and functional with the latest standards.
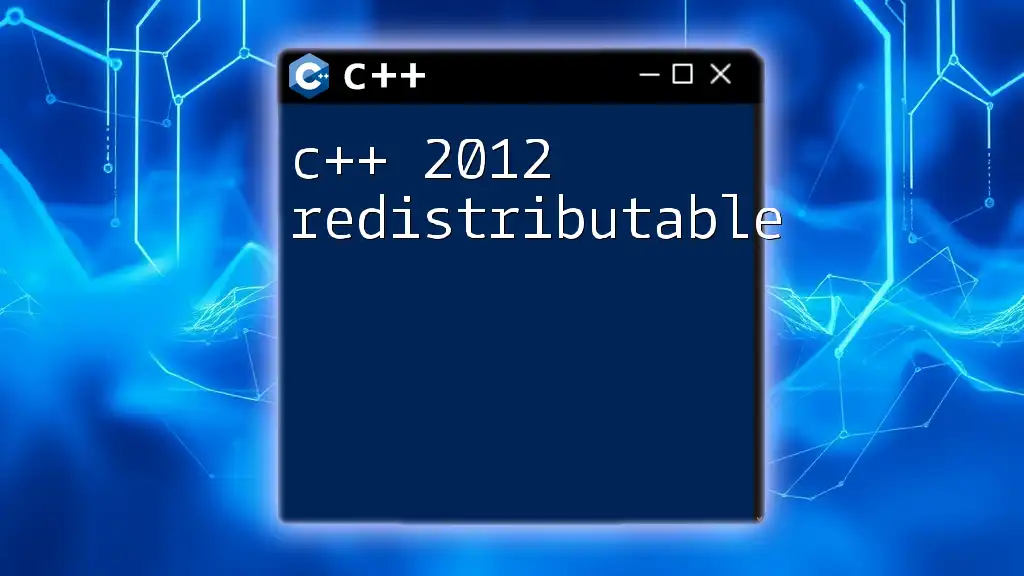
Conclusion
Redistributable C++ plays a vital role in application deployment, ensuring that necessary libraries are available for software to function correctly on users' systems. By understanding how redistributable packages work, learning to install and manage these resources, and following best practices in your development process, you can enhance the user experience and reduce runtime errors related to dependency issues.
Fostering good practices in redistributable management will ultimately benefit both developers and end-users, paving the way for successful C++ applications in today's software landscape.
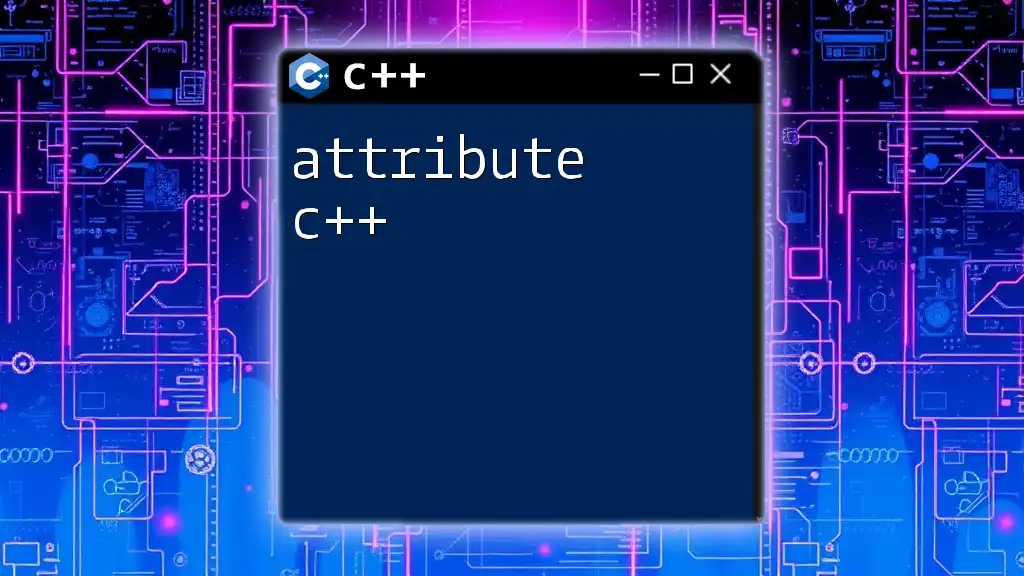
Additional Resources
For further reading, consider checking the official Microsoft documentation, recommended tutorials, and community forums to deepen your understanding and resolve any specific concerns regarding redistributable C++. Don’t forget to stay connected with the C++ community for ongoing insights and support.