Conditional statements in C++ allow you to execute specific blocks of code based on whether certain conditions are true or false.
#include <iostream>
int main() {
int number = 10;
if (number > 0) {
std::cout << "The number is positive." << std::endl;
} else {
std::cout << "The number is not positive." << std::endl;
}
return 0;
}
Understanding C++ Conditional Operators
Conditional operators are fundamental tools in C++ that allow you to evaluate conditions and make decisions in your code. They work by comparing values and returning a boolean result (true or false) that influences the flow of your program.
Types of Conditional Operators in C++
C++ supports several types of conditional operators:
-
Relational Operators: These operators allow you to compare two values. Common relational operators include:
- `<`: less than
- `>`: greater than
- `<=`: less than or equal to
- `>=`: greater than or equal to
-
Equality Operators: Used to check for equality or inequality:
- `==`: equal to
- `!=`: not equal to
-
Logical Operators: These operators combine multiple conditions:
- `&&`: logical AND
- `||`: logical OR
- `!`: logical NOT
Using Conditional Operators in C++
To effectively use conditional operators, consider the following example where we determine if a number is positive or negative.
int number = -5;
if (number > 0) {
std::cout << "The number is positive." << std::endl;
} else {
std::cout << "The number is negative or zero." << std::endl;
}
In this example, the `if` statement uses the greater than operator to evaluate whether `number` is positive.

Conditional Expressions in C++
A conditional expression allows you to conditionally assign values or execute expressions. The most common type of conditional expression in C++ is the ternary operator. The syntax is as follows:
condition ? value_if_true : value_if_false
Examples of Conditional Expressions
Consider the following straightforward example where we use the ternary operator to evaluate a person's age:
int age = 18;
std::string status = (age >= 18) ? "Adult" : "Minor";
std::cout << "Status: " << status << std::endl;
In this case, if `age` is 18 or older, `status` is assigned the value "Adult"; otherwise, it gets "Minor".
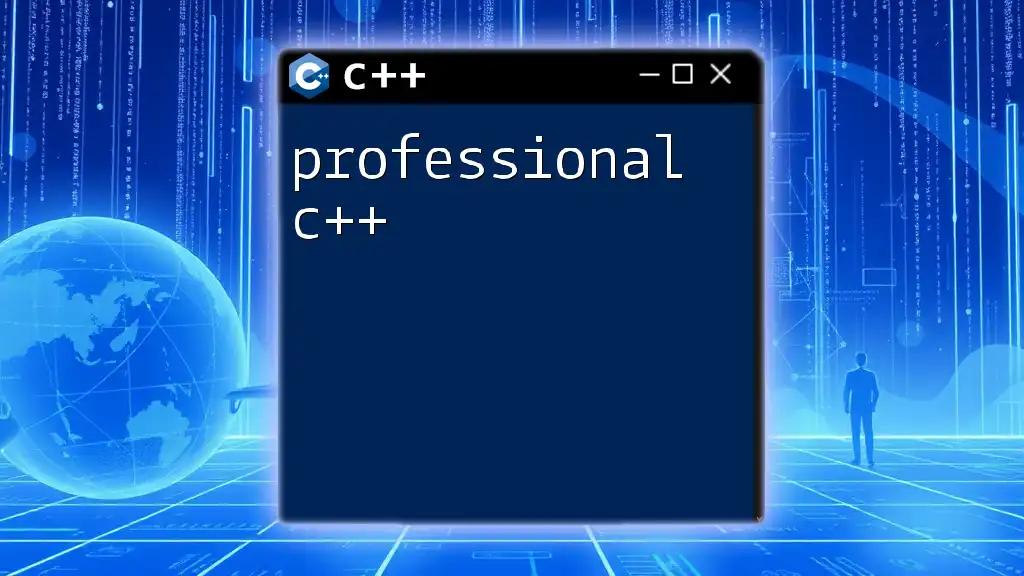
Conditional Assignment in C++
Conditional assignment is a useful technique where you can assign a value based on the outcome of a condition. It enhances code readability and efficiency, particularly when dealing with simple conditions.
Using the Ternary Operator for Conditional Assignments
You can streamline your code by adopting the ternary operator for conditional assignments. Here's how it looks in practice:
int score = 76;
std::string grade = (score >= 75) ? "Pass" : "Fail";
std::cout << "Grade: " << grade << std::endl;
In the above code, if `score` is 75 or above, `grade` is set to "Pass"; otherwise, it is "Fail". This method minimizes the verbosity of code that would typically require an `if-else` block.
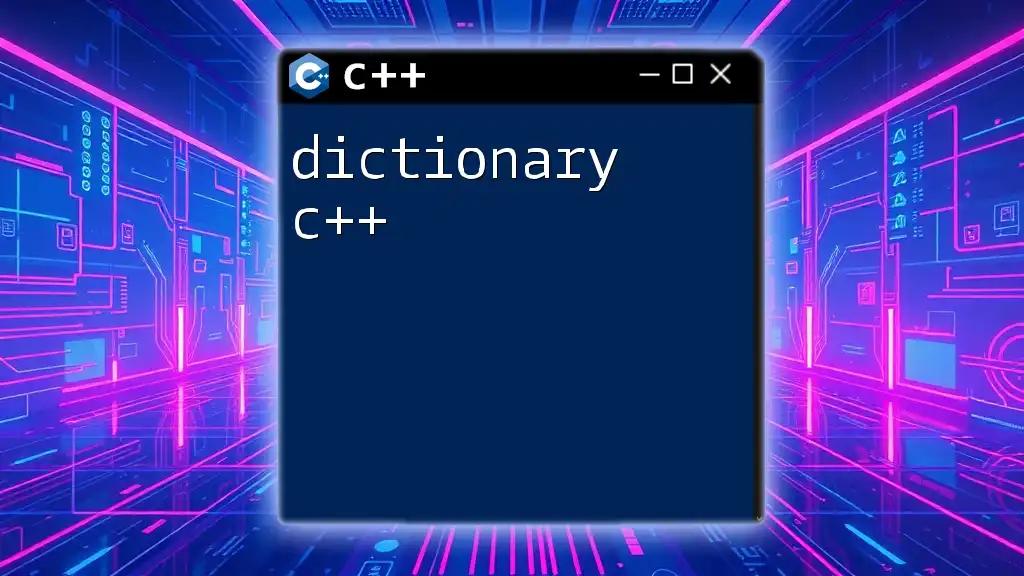
Control Flow Statements in C++
Control flow statements are essential in directing the execution of the program based on certain conditions. The primary control flow statements in C++ include if, else, else-if, and switch.
If Statement
The `if` statement is a fundamental building block in C++. It allows you to execute a block of code based on a specific condition.
int score = 88;
if (score > 90) {
std::cout << "Excellent!" << std::endl;
}
In this example, the message is displayed only if the score exceeds 90.
Else and Else-If Statements
The `else` statement provides an alternative action when the condition of the `if` statement is false. The `else-if` allows for additional conditions.
if (score > 90) {
std::cout << "Excellent!" << std::endl;
} else if (score >= 75) {
std::cout << "Good!" << std::endl;
} else {
std::cout << "Needs Improvement!" << std::endl;
}
This structure evaluates multiple conditions in a clean manner, improving readability.
Switch Statement
The `switch` statement serves as an efficient way to handle multiple potential conditions based on the value of a single variable. It’s particularly useful when you need to compare the same variable against different constant values.
char grade = 'B';
switch (grade) {
case 'A':
std::cout << "Excellent!" << std::endl;
break;
case 'B':
std::cout << "Good!" << std::endl;
break;
default:
std::cout << "Invalid Grade" << std::endl;
}
When `grade` is evaluated, it matches against the defined cases. If the case corresponds to 'B', it outputs "Good!".
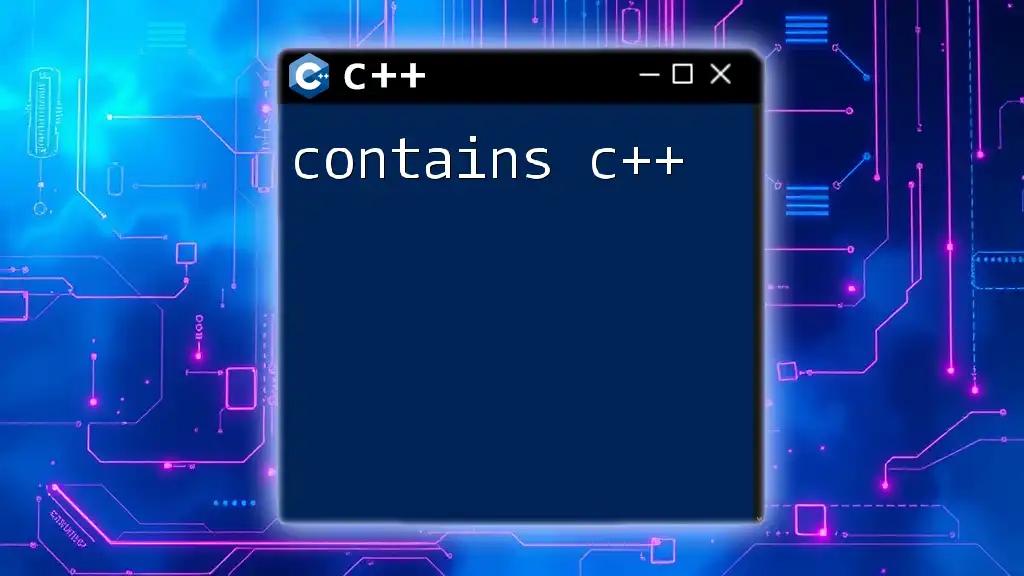
Best Practices for Using Conditionals in C++
When utilizing conditionals in your C++ programs, keep the following best practices in mind:
-
Maintainability and Readability: Always strive for code that is easy to read and understand. Favor clear and descriptive variable names and structure your conditionals in an easily understandable way.
-
Avoiding Nested Conditionals: Minimizing nested `if` statements can significantly enhance readability. Flattening nested conditions into single-level structures can lead to less error-prone code.
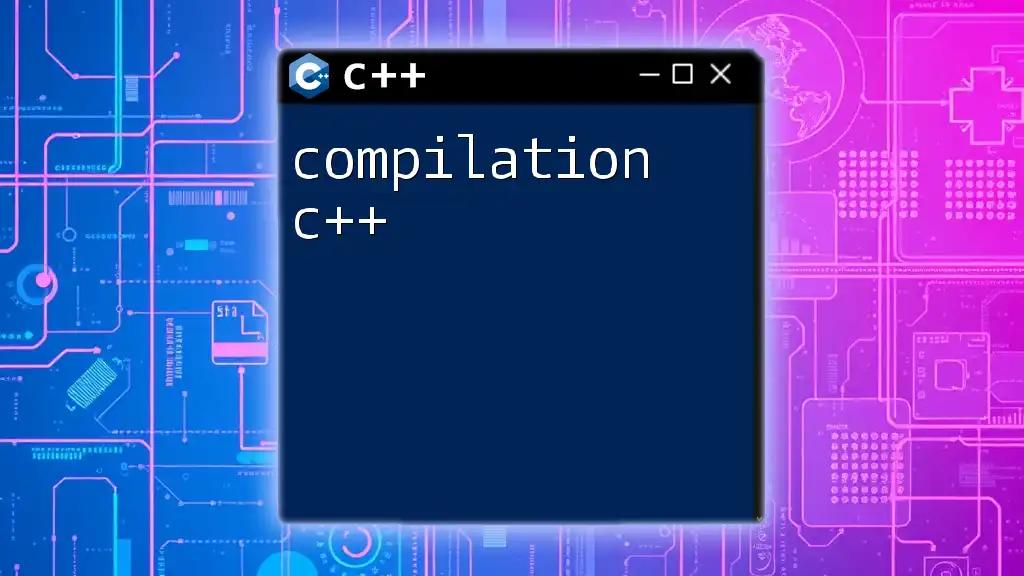
Common Mistakes with Conditionals in C++
Even experienced programmers can fall victim to common pitfalls related to conditionals. Here are a few frequent errors and misconceptions:
-
Errors with Conditional Operators: Confusing `=` (assignment) with `==` (equality) can lead to unexpected results. Always ensure you’re using the correct operator for your intended logic.
-
Understanding Boolean Contexts: Remember that any non-zero value in C++ is considered `true`, and zero is considered `false`. Understanding this can help avoid logical errors in condition evaluations.
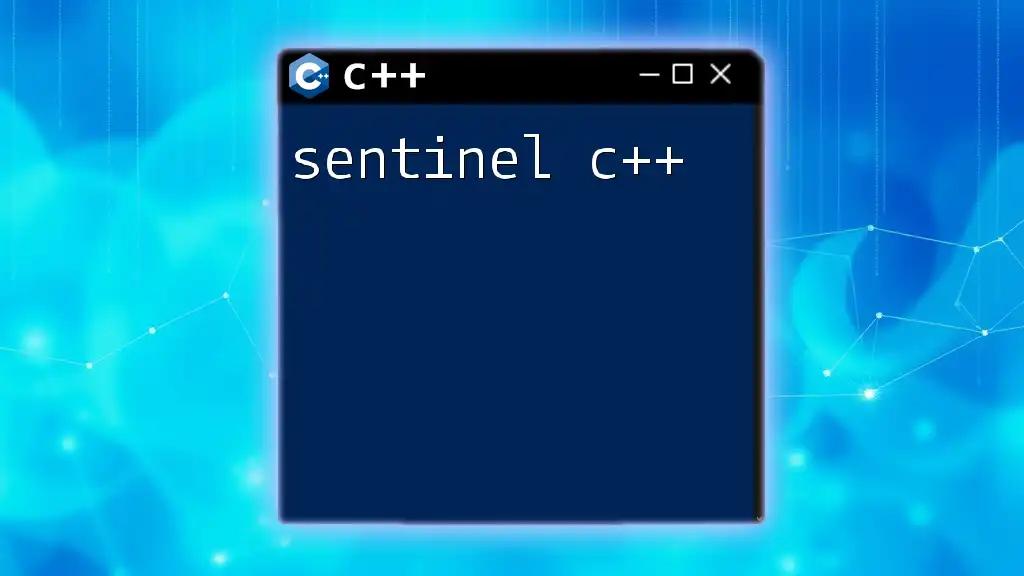
Conclusion
Mastering conditional C++ is crucial for developing robust and efficient applications. By understanding and implementing various conditional operators, expressions, and control statements, you can effectively dictate the flow of your programs. Regular practice and consideration of best practices will improve your coding skills and enhance the maintainability of your code.
With continued learning, you can explore more advanced techniques and build a solid foundation in C++ programming.