"Professional C++ encompasses best practices, advanced techniques, and efficient code styling to develop robust and high-performance applications."
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to Professional C++!" << endl;
return 0;
}
Understanding C++ Fundamentals
The Basics of C++
C++ is a powerful, versatile programming language known for enabling high-performance application development. It combines the efficiency of low-level programming found in languages like C with the functionality of high-level languages like Python. Its characteristic features include a rich standard library, support for procedural, object-oriented, and generic programming paradigms, making it a preferred choice for many developers in various sectors, from systems software to game development.
The syntax of C++ is relatively straightforward yet flexible. Here’s a simple example of a C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, we include the iostream library, which is essential for input and output operations. The `main()` function is the entry point of any C++ program.
Core Concepts
Data Types and Variables
C++ supports a rich set of data types. Understanding these is crucial for effective programming. C++ data types can be broadly categorized into:
- Primitive Types: int, char, float, double, etc.
- Derived Types: arrays, pointers, classes, etc.
Here's an example of variable declaration and initialization:
int age = 25;
char grade = 'A';
float salary = 50000.50;
Control Structures
Control structures allow developers to dictate the flow of the program. Common structures include if statements and loops. For instance, you can use a loop to iterate through an array:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
cout << arr[i] << " ";
}
In this example, we declare an array of integers and print each value using a for-loop.
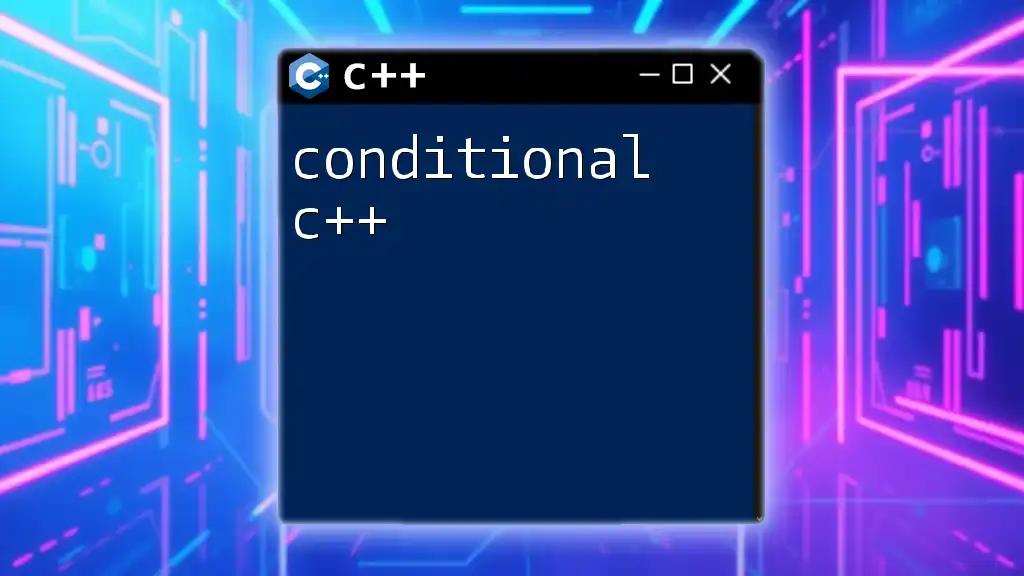
Object-Oriented Programming in C++
Understanding OOP Principles
C++ is fundamentally driven by Object-Oriented Programming (OOP), which is characterized by four key principles: Encapsulation, Inheritance, Polymorphism, and Abstraction.
- Encapsulation restricts direct access to some of an object's components and can prevent accidental modification of data.
- Inheritance allows for class hierarchies, enabling the creation of new classes based on existing classes, promoting code reuse.
- Polymorphism enables standardization of interfaces and methods across different classes.
To illustrate OOP in C++, consider the following definition of a class:
class Animal {
public:
void speak() {
cout << "Animal speaks" << endl;
}
};
C++ Classes and Objects
In C++, classes serve as blueprints for creating objects. Here’s how to define and utilize a `Dog` class derived from the `Animal` class:
class Dog : public Animal {
public:
void speak() {
cout << "Woof!" << endl;
}
};
In this example, `Dog` inherits from `Animal`, showcasing the concept of inheritance, where the `Dog` class can utilize or override the `speak` method of the `Animal` class.
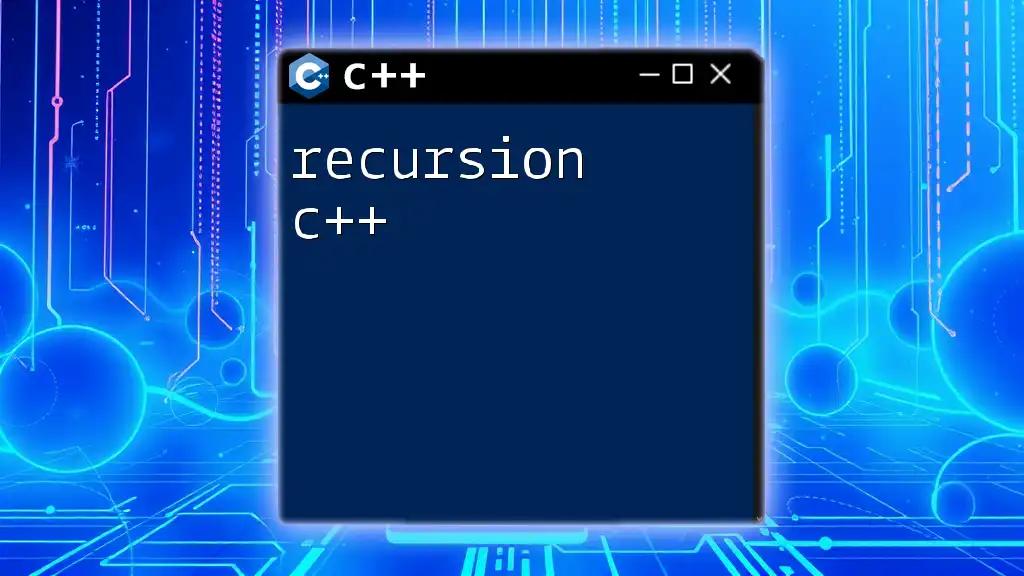
Advanced C++ Concepts
Memory Management
Understanding Pointers and References
Memory management is critical in C++. Pointers provide direct access to memory addresses, which can optimize performance but also pose risks such as memory leaks. Here's a quick look at pointers:
int x = 10;
int* p = &x;
cout << *p; // Outputs: 10
In this example, we define a pointer `p` that holds the address of the variable `x`. The `*` operator dereferences the pointer, allowing us to access the stored value.
Dynamic Memory Allocation
C++ allows for dynamic memory management using the `new` and `delete` keywords. This capability provides flexibility in memory handling. Here’s an example:
int* arr = new int[5]; // Dynamically allocate an array of 5 integers
delete[] arr; // Deallocate the memory
Remember to deallocate memory to avoid leaks, which is a crucial aspect of professional C++ development.
Templates and STL
Introduction to Templates
Templates allow for writing flexible and reusable code. They enable developers to create functions or classes that work with any data type. Here’s a simple function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can add integers, floats, or any other data type that supports the `+` operator.
Standard Template Library (STL)
The STL is a comprehensive library that provides a rich set of methods and classes, simplifying complex coding tasks. Utilizing vectors is a common practice:
#include <vector>
using namespace std;
vector<int> vec = {1, 2, 3, 4};
Vectors offer dynamic sizing, memory management, and built-in operations that make them easier to work with than traditional arrays.
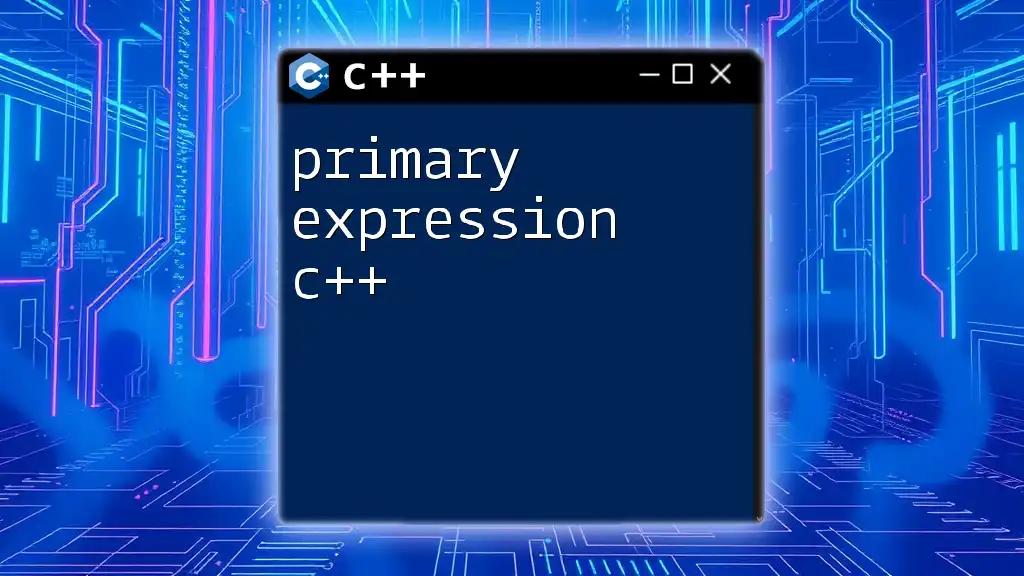
Best Practices for Professional C++ Coding
Writing Clean and Maintainable Code
To achieve professional-level code quality, writing clean and maintainable code is paramount. This includes adhering to consistent naming conventions, organizing your code into well-defined modules, and thoughtfully commenting your code. This approach makes your code easier to read and maintain, especially in larger projects.
Performance Optimization Techniques
Understanding how to optimize C++ code is invaluable. Profiling and benchmarking your code can highlight areas for improvement. Tools like `gprof` or `Valgrind` can help identify performance bottlenecks.
Some common optimization strategies include:
- Loop unrolling to decrease the overhead of loop control.
- Memory caching to enhance the performance of frequently accessed data.
By implementing these techniques, you can significantly enhance the efficiency of your C++ applications.
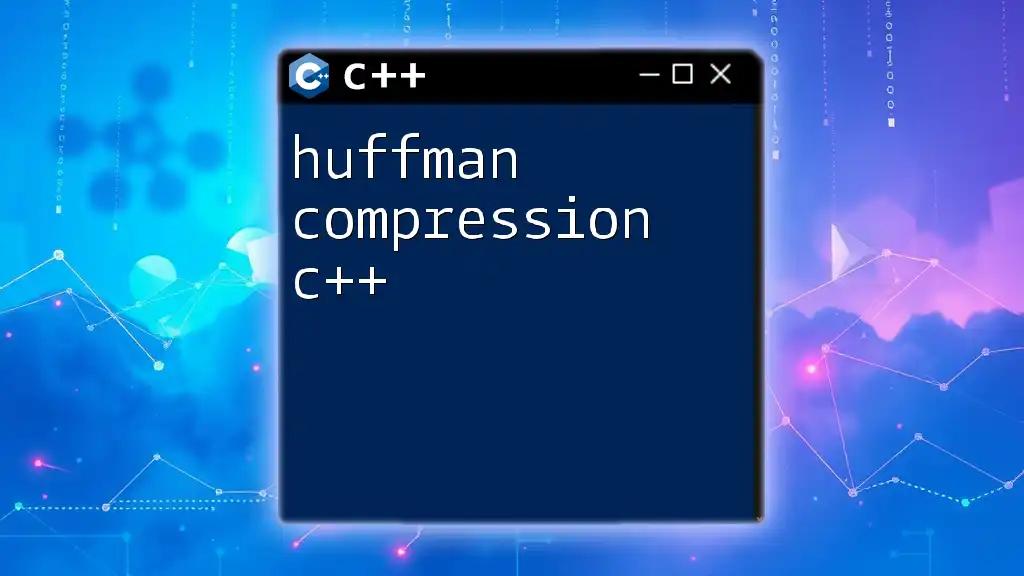
Conclusion
Becoming a skilled C++ developer hinges on a solid understanding of both the fundamental and advanced concepts of the language. As you progress, remember that continuous learning and engaging with the C++ community are essential. Participating in forums, contributing to open-source projects, and exploring new libraries will further bolster your expertise in professional C++.
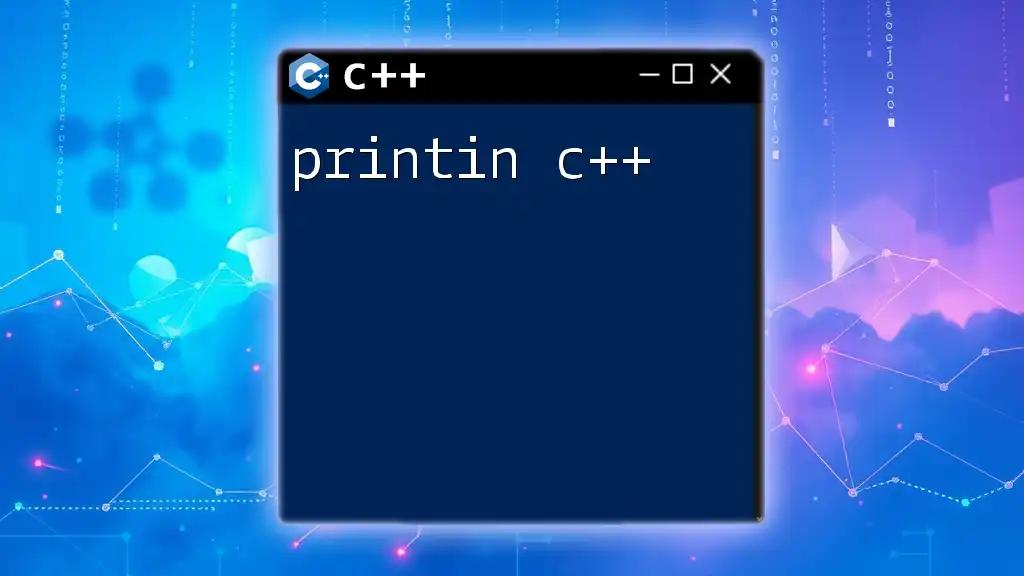
Additional Resources
For those interested in further expanding their C++ knowledge, consider the following:
- Books: Titles like "Effective C++" by Scott Meyers or "C++ Primer" by Lippman are excellent resources.
- Online Courses: Platforms like Coursera and Udemy offer structured courses ranging from beginner to advanced levels.
- Forums and Communities: Joining platforms such as Stack Overflow and Reddit's r/cpp can help you connect with other developers and gain insights from their experiences.
With dedication and practice, you can become a proficient and professional C++ developer.