The `rbegin()` function in C++ returns a reverse iterator pointing to the last element of a container, allowing you to traverse it backwards.
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int>::reverse_iterator rit = vec.rbegin();
for (; rit != vec.rend(); ++rit) {
std::cout << *rit << " "; // Outputs: 5 4 3 2 1
}
return 0;
}
Understanding Iterators in C++
What are Iterators?
Iterators are a fundamental concept in C++ that provide a way to traverse the elements of a container (like arrays, vectors, or lists) without needing to know the underlying structure. Iterators abstract the idea of pointers and allow developers to write generic algorithms that can be applied to different data structures.
Iterators can be classified into several types:
- Input Iterators: Read elements in a forward direction.
- Output Iterators: Write elements in a forward direction.
- Forward Iterators: Can read/write elements only in a single pass.
- Bidirectional Iterators: Can move in both forward and backward directions.
- Random Access Iterators: Can jump to any position in a container in constant time.
Importance of Reverse Iterators
Reverse iterators are a special type of iterator that allow you to traverse containers in reverse order. This flexibility is particularly useful when you need to process elements from the end toward the beginning, which can often lead to simpler code and improved performance in certain scenarios.

What is rbegin in C++?
Definition of rbegin
In C++, `rbegin()` is a member function of several Standard Library containers that returns a reverse iterator pointing to the last element of the container. This allows us to iterate backward through the container, making it easy to access elements in reverse order without needing complex index calculations.
Syntax of rbegin
The syntax for calling `rbegin()` is straightforward:
container_name.rbegin();
The return type is a reverse iterator that allows you to access elements in reverse sequence. The `rbegin()` function does not take any parameters and works on a variety of containers such as vectors, lists, deques, and strings.
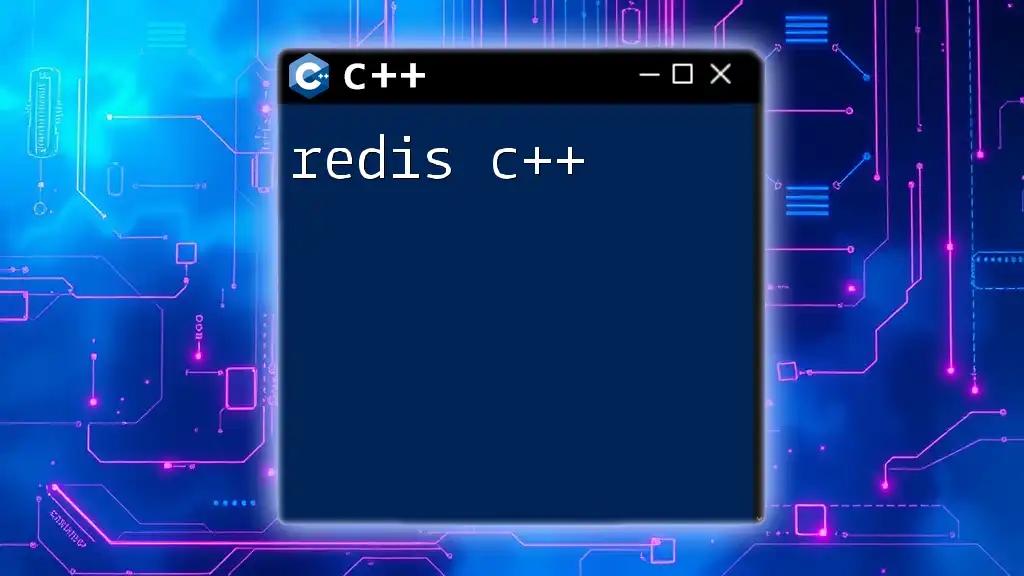
Working with rbegin in C++
Using rbegin with Different Containers
Vectors
Using `rbegin()` with vectors allows us to easily iterate through the vector from the last element to the first.
Code Example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (auto it = vec.rbegin(); it != vec.rend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
In this example, the output will be `5 4 3 2 1`, demonstrating how `rbegin()` enables us to access the vector's elements in reverse order. Here, `rend()` serves as the termination point for the loop, indicating the point just before the first element.
Lists
Similarly, `rbegin()` can be applied effectively to lists. This allows processing the items in a list from the end toward the front.
Code Example:
#include <iostream>
#include <list>
int main() {
std::list<int> lst = {10, 20, 30, 40};
for (auto it = lst.rbegin(); it != lst.rend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
The output in this case would be `40 30 20 10`, highlighting the utility of reverse iteration with lists.
Other Containers (e.g., Deques, Strings)
The `rbegin()` function is also compatible with other Standard Library containers such as deques and strings.
Code Example for a String:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
for (auto it = str.rbegin(); it != str.rend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
This code outputs `o l l e H`, showing how `rbegin()` effectively accesses characters in reverse.
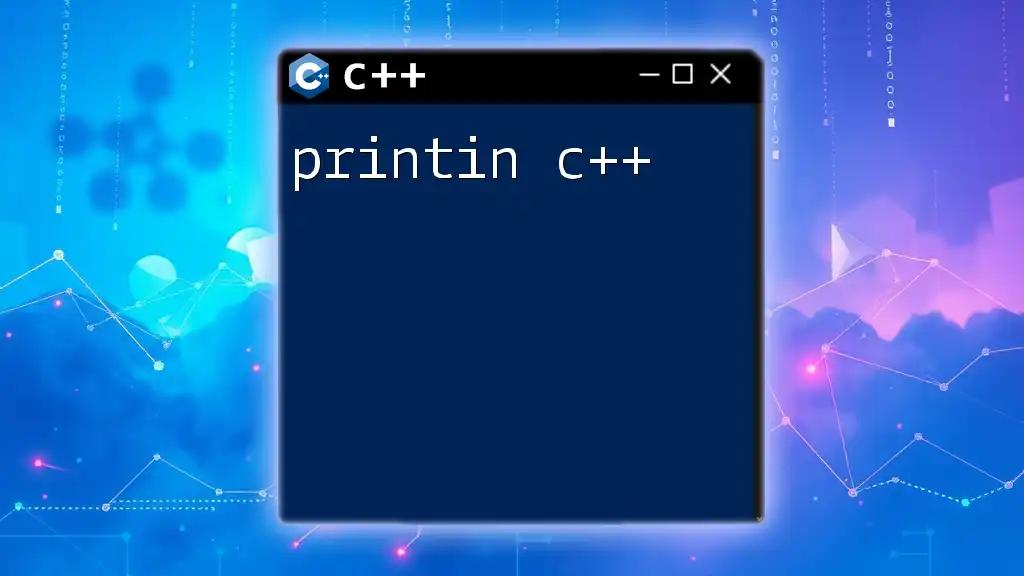
Practical Applications of rbegin
When to Use rbegin
Use `rbegin()` when you need to process data from the end to the beginning. This is particularly useful in algorithms that involve reversing data or when the last elements hold more significance than the first.
Comparison with Standard Iterators
It is essential to distinguish between `begin()` and `rbegin()`. While `begin()` points to the first element, `rbegin()` points to the last. Choosing between them depends on the specific scenario; reverse iterators can lead to a more intuitive and simpler solution when working with certain algorithms.

Common Mistakes When Using rbegin
Misunderstanding the Range
A common pitfall when using `rbegin()` revolves around the range of iteration. Developers often confuse the boundaries provided by the reverse iterators. Always remember that `rend()` signifies the point just before the first element, which can lead to off-by-one errors if not properly accounted for.
Using rbegin with Non-reversible Containers
Another significant mistake is attempting to call `rbegin()` on containers that do not support reverse iteration. Attempting to use reverse iterators inappropriately can lead to compilation errors or runtime exceptions. It is crucial to ensure that the chosen container supports reverse iterators before utilizing `rbegin()`.
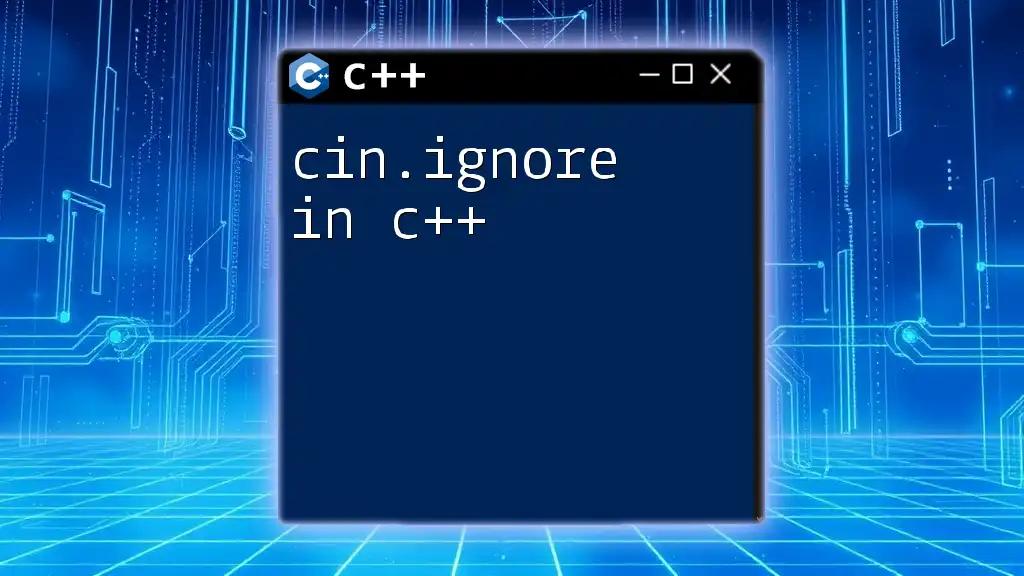
Conclusion
In conclusion, mastering the use of `rbegin` in C++ allows for efficient and intuitive handling of data in reverse order. By practice and familiarity with the C++ Standard Library, you can leverage reverse iterators like `rbegin()` to write cleaner, more efficient code. Now is the time to explore and experiment with `rbegin` in your C++ projects!
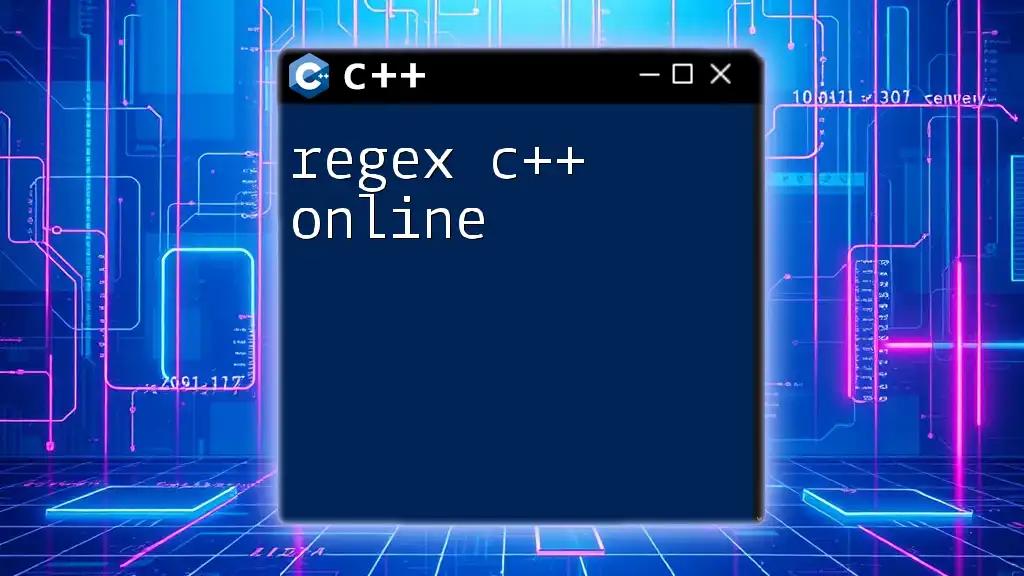
Additional Resources
For more in-depth understanding, you can refer to the official C++ documentation on iterators as well as books and tutorials focused on the C++ Standard Library and iterators.
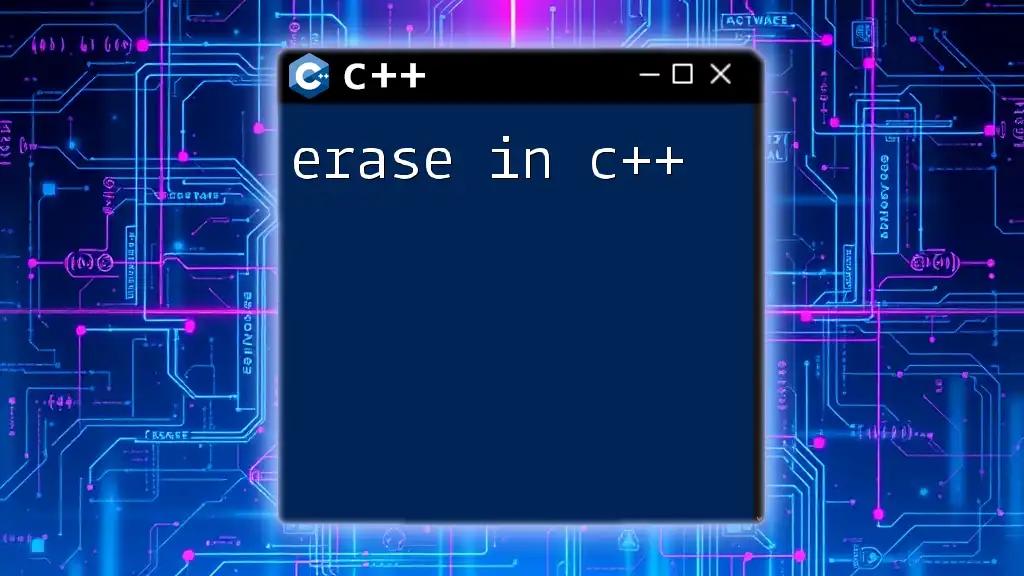
Call to Action
If you found this guide helpful, consider sharing it and following us for more concise and effective C++ tutorials and tips!