In C++, the `<regex>` library provides powerful tools for pattern matching using regular expressions, allowing developers to search, match, and manipulate strings efficiently.
Here’s a simple code snippet demonstrating how to use regex to find a word in a string:
#include <iostream>
#include <regex>
int main() {
std::string text = "Hello, welcome to the world of C++!";
std::regex pattern("C\\+\\+");
if (std::regex_search(text, pattern)) {
std::cout << "Found C++ in the text!" << std::endl;
} else {
std::cout << "C++ not found." << std::endl;
}
return 0;
}
What is Regex?
Regular expressions, often abbreviated as regex, are sequences of characters that form a search pattern. They are immensely useful in programming for tasks such as data validation, searching, and text manipulation. Essentially, regex allows you to define a search criteria that can be used to find particular strings or patterns within larger bodies of text.
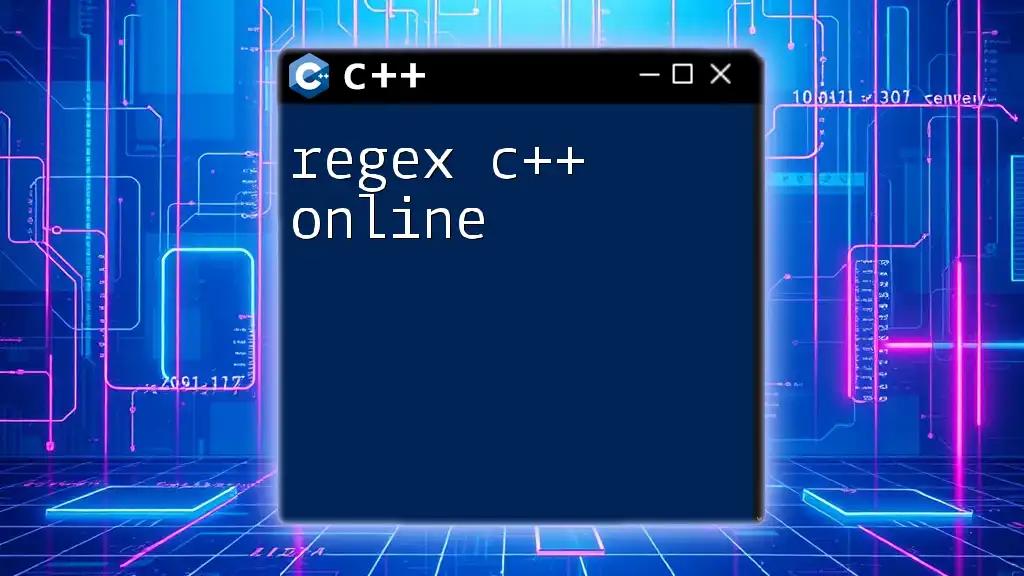
Why Use Regex in C++?
In the realm of C++, using regex can significantly enhance your ability to handle strings efficiently. Here are a few reasons to consider:
- Validation: Quickly check user inputs, like email addresses or phone numbers.
- Search and Replace: Effortlessly find and replace text within strings.
- Complex Pattern Recognition: Identify and extract data that follows specific formats.
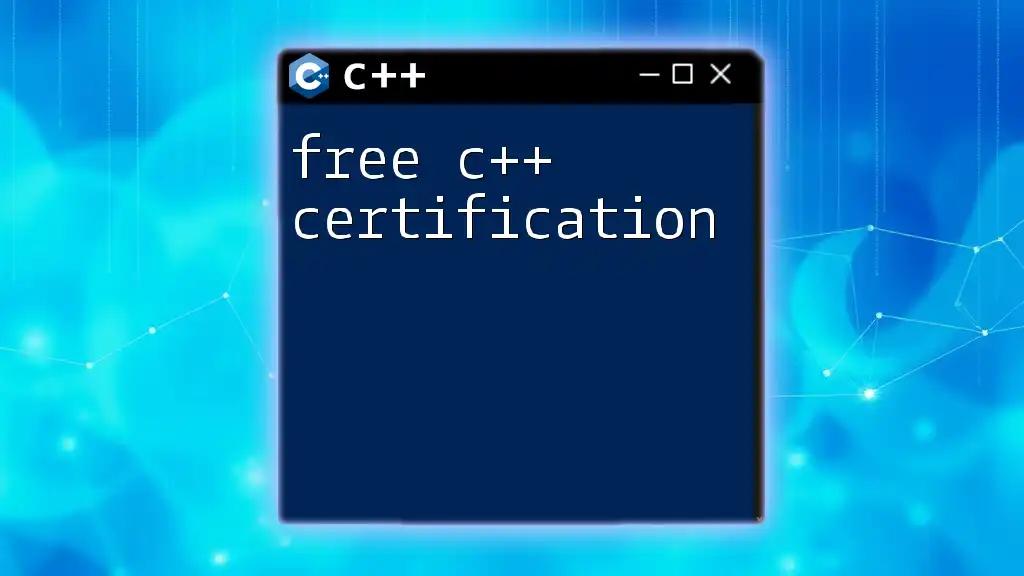
Understanding C++ Regex
What are C++ Regular Expressions?
C++ regular expressions are built on the Standard Template Library (STL) and follow the syntax defined by the C++11 standard. Regular expressions in C++ can be powerful tools for performing text processing, allowing you to condense complex searches into simple expressions.
Key Components of C++ Regex
To fully understand C++ regex, you should familiarize yourself with its key components:
- Patterns: A sequence of characters defining your search criteria.
- Metacharacters: Special characters that have specific meanings in regex. They include:
- `.` (dot) for any character
- `^` (caret) for start of a line
- `$` (dollar sign) for end of a line

Setting Up C++ Regex
To start using regex in your C++ program, you'll need to include the necessary library:
#include <regex>
This header file contains the definitions and functions associated with regex operations in C++. By including it, you enable the use of `std::regex`, `std::smatch`, and other related types.
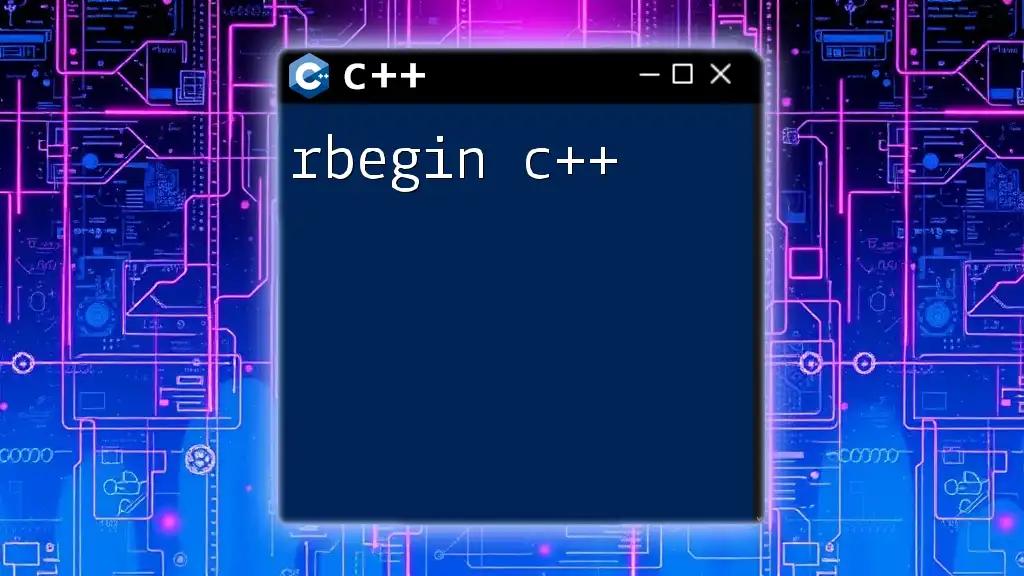
Using C++ Regex
Creating a Regex Object
To create a regex object, utilize the `std::regex` class. Here's a simple code snippet to illustrate defining a regex pattern:
std::regex email_pattern(R"((\w+)(\.\w+)*@(\w+)(\.\w+)+)");
In this example, we define a regex pattern to match typical email addresses. Using raw string literals (with `R"(...)"`) helps avoid the need for escape sequences.
Matching Strings with Regex
Once you have your regex object, you can use it to match patterns against strings.
- std::regex_match() is used to check if an entire string matches the pattern:
std::string email = "user@example.com";
if (std::regex_match(email, email_pattern)) {
std::cout << "Valid email!" << std::endl;
} else {
std::cout << "Invalid email." << std::endl;
}
In this example, we verify if the string `email` follows the defined `email_pattern`.

C++ Regex Functions
Commonly Used Functions
When working with regex in C++, there are several fundamental functions that you're likely to use frequently:
- std::regex_search(): This function checks for a match within a string but does not require the whole string to conform to the pattern.
- std::regex_replace(): This is used to replace substrings matching the regex with a specified replacement string.
Here’s a practical code example of each function:
Matching Example with `regex_search`
std::string text = "Contact us at support@company.com";
if (std::regex_search(text, email_pattern)) {
std::cout << "Found an email address!" << std::endl;
}
This example looks for an email address within the string `text` using `std::regex_search()`.
Replacing Text with `regex_replace`
std::string new_text = std::regex_replace(text, email_pattern, "REDACTED");
std::cout << new_text << std::endl;
In this snippet, any found email address is replaced with the word "REDACTED".
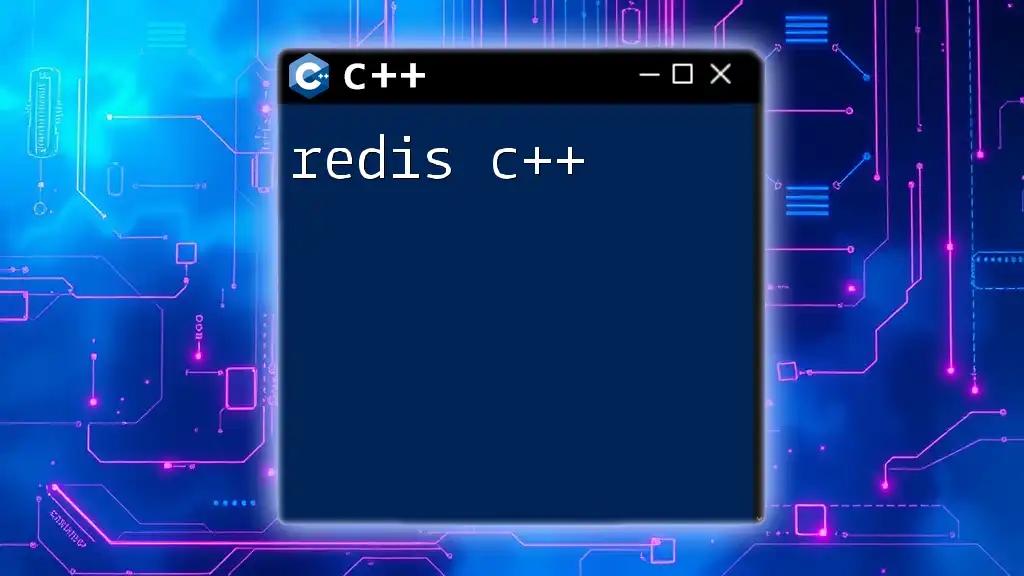
C++ Regular Expression Patterns
Character Classes
Character classes are essential in defining more precise criteria. They allow you to specify a set of characters your regex will match, such as:
std::regex digit_pattern(R"([0-9]+)"); // Matches one or more digits
In this example, `digit_pattern` will match any sequence of numeric characters.
Quantifiers
Quantifiers define how many times a character or group must appear for a match to occur. Common quantifiers include:
- `*`: Matches 0 or more times
- `+`: Matches 1 or more times
- `?`: Matches 0 or 1 time
- `{n,m}`: Matches between `n` and `m` times
Example:
std::regex alpha_pattern(R"([a-zA-Z]{3,5})"); // Matches between 3 to 5 alphabet characters
Anchors and Boundaries
Anchors allow you to specify the position of matches. The caret (`^`) indicates the start of a string, while the dollar sign (`$`) denotes the end.
Here's a basic example:
std::regex starts_with_hello(R"(^Hello)"); // Matches strings that start with "Hello"
Practical C++ Regex Examples
Email Validation Example
Here's a more elaborate example demonstrating how to use regex for email validation:
std::string test_email = "user_name123@gmail.com";
std::regex email_regex(R"((\w+)(\.\w+)*@(\w+)(\.\w+)+)");
if (std::regex_match(test_email, email_regex)) {
std::cout << "Valid email address." << std::endl;
} else {
std::cout << "Invalid email address." << std::endl;
}
This example checks if `test_email` is a valid email address based on the provided regex pattern.
Phone Number Formatting
You can also validate phone numbers. Here's an example for various phone formats:
std::string phone = "(555) 123-4567";
std::regex phone_regex(R"(\(\d{3}\) \d{3}-\d{4})");
if (std::regex_match(phone, phone_regex)) {
std::cout << "Valid phone number format." << std::endl;
} else {
std::cout << "Invalid phone number format." << std::endl;
}

Advanced C++ Regex Techniques
Lookaheads and Lookbehinds
Lookaheads and lookbehinds allow you to assert conditions about what precedes or follows a match without including them in the match itself. For example:
std::regex lookahead_regex(R"(\d(?=\s))"); // Matches a digit followed by a space
Using Flags with C++ Regex
C++ regex also supports flags that modify the matching behavior. For example, you can enable case-insensitive matching:
std::regex case_insensitive_regex(R"(hello)", std::regex_constants::icase);
if (std::regex_match("HELLO", case_insensitive_regex)) {
std::cout << "Match found in a case-insensitive manner!" << std::endl;
}
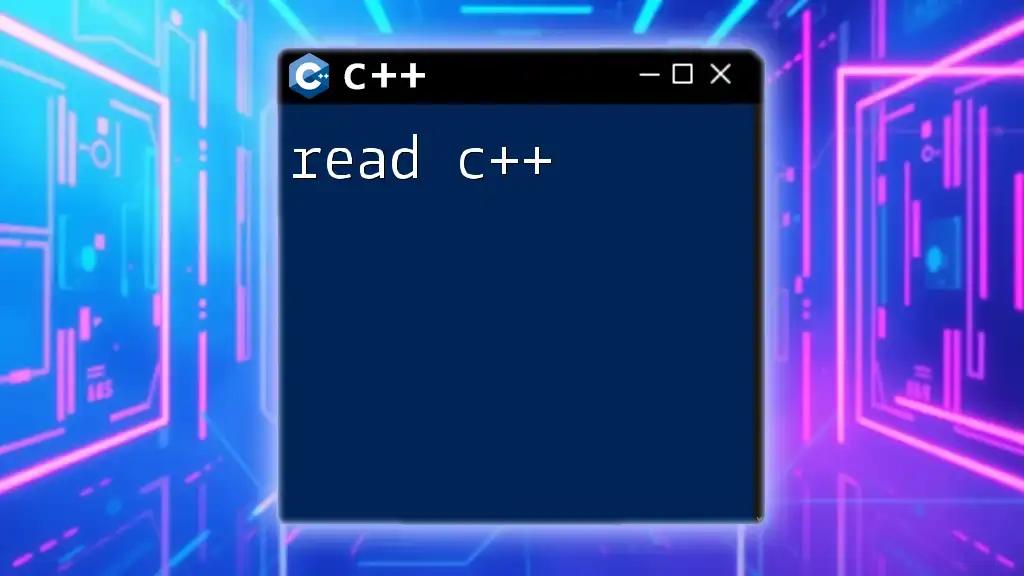
Debugging C++ Regex
Common Issues and Solutions
While regex can be powerful, it can also lead to common pitfalls such as mismatches or unexpected results. Always ensure that your patterns are tightly defined to prevent false positives.
Performance Considerations
Regular expressions can affect performance, especially with complex patterns. When crafting regex, aim for simplicity, and avoid unnecessary backtracking.

Conclusion
In this comprehensive guide, we explored the various facets of using regex in C++. Understanding regex can significantly enhance your ability to manipulate and validate data efficiently. By practicing with the examples provided, you'll become proficient in crafting regex patterns that suit your needs.
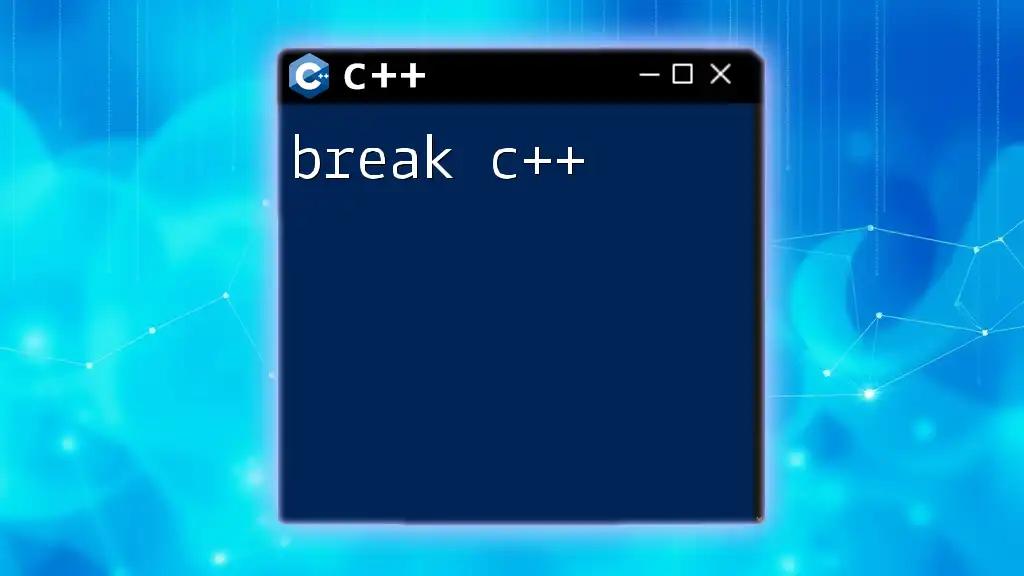
Additional Resources
For those looking to dive deeper into regex and its application in C++, consider exploring specialized books and online tools such as regex testers and validators, which can assist you in developing and debugging your patterns. Keep experimenting and enhancing your skills with regex to unlock its full potential in your C++ programming endeavors!