The `peek` function in C++ is used to access the next character in the input stream without extracting it, allowing you to analyze the value without modifying the stream's state.
Here’s a simple example:
#include <iostream>
#include <sstream>
int main() {
std::istringstream input("Hello, world!");
char nextChar = input.peek(); // Peek at the next character
std::cout << "The next character is: " << nextChar << std::endl; // Output: H
return 0;
}
Understanding Peek Concept in C++
What Does Peek Do?
The `peek` function in C++ is a powerful utility that allows programmers to examine the next character in an input stream without removing it from the stream. This operation is essential when you want to check what the next input will be without actually reading it. The peek function returns the character as an integer but does not alter the position of the stream pointer, making it distinct from other input operations such as `get()`, which reads the character and moves the pointer.
The Importance of Peek in Input Streams
Using the `peek` function is crucial for controlling the flow of text or binary data read from streams. When processing user input or reading from files, knowing what the next character is can help avoid unnecessary errors or allow for conditional logic to be implemented. For instance, if you first check whether the next character is a specific letter that requires a different handling procedure, you can optimize the data processing and enhance user experience.
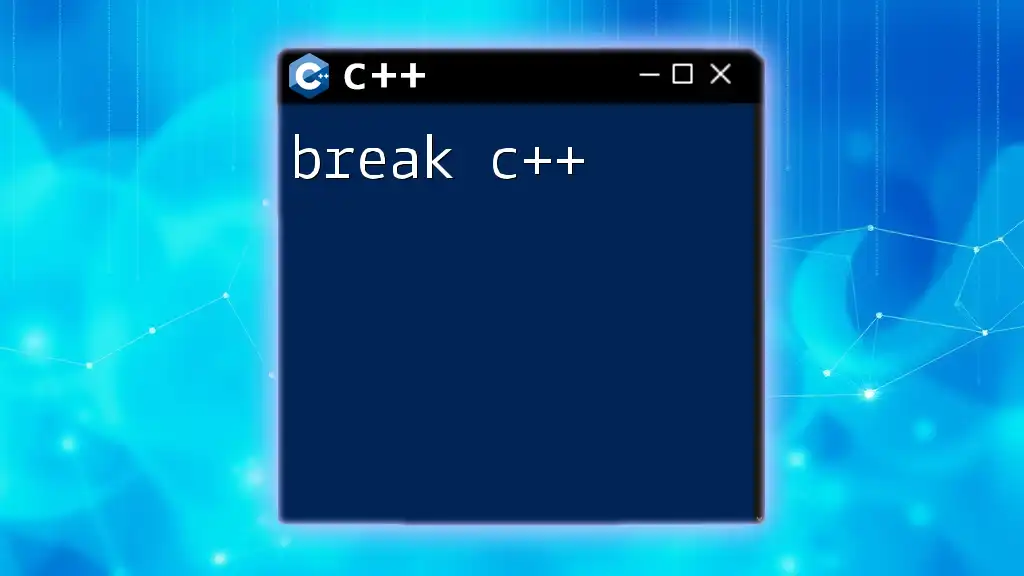
How to Use Peek in C++
Syntax of Peek in C++
The basic syntax of the `peek` function is straightforward:
int peekChar = inputStream.peek();
Here, `inputStream` can be any input stream object like `ifstream`, `cin`, or `stringstream`, and `peekChar` will store the integer value representing the next character that can be read.
InputStream Peek Functionality
Using the Peek Function with Ifstream
The `ifstream` class allows you to read bytes or characters from a file. Using the `peek` function here can help check if there is data available to read without actually consuming it.
Basic Example
#include <iostream>
#include <fstream>
int main() {
std::ifstream inputFile("example.txt");
char ch;
if (inputFile.peek() != EOF) {
inputFile.get(ch);
std::cout << "Read character: " << ch << std::endl;
}
inputFile.close();
return 0;
}
In this example, before attempting to read a character from `inputFile`, we first check if we have reached the end of the file using `peek()`. Only if there is data (not EOF) do we actually call `get(ch)` to read the character, ultimately helping us avoid unnecessary errors.
InputStream Peek with String Streams
The `stringstream` class is another useful stream that allows us to manipulate strings as input/output sources. Using `peek` on a string stream provides a similar functionality.
Example Using StringStream
#include <sstream>
#include <iostream>
int main() {
std::stringstream ss("Hello, world!");
char ch = ss.peek();
std::cout << "Next character to be read: " << ch << std::endl;
return 0;
}
In this example, by calling `peek()`, we can see what the next character will be in the string stream without actually removing it from the stream, allowing further flexibility in handling the data.
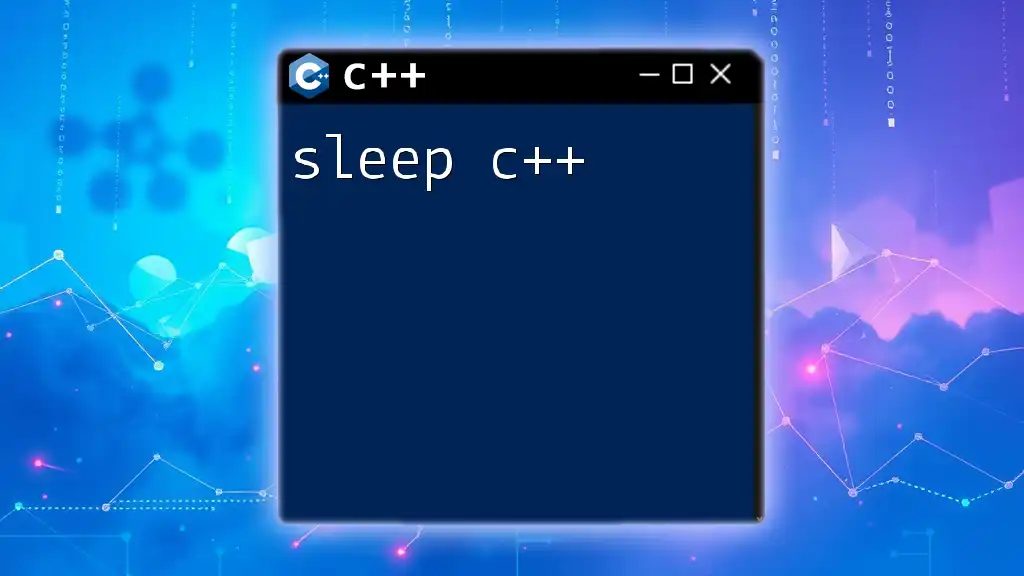
Practical Applications of Peek in C++
Use Case: Buffering Characters
Buffering is a technique used to hold data temporarily while it is being transferred from one place to another. Using `peek` allows you to analyze the next character before deciding how to process it. For example, when reading from a file and expecting different data formats, `peek` can guide your conditional statements regarding how to read the next piece of data (as numeric or string).
Enhanced Input Processing
When interacting with user input via the console, employing Peek can help enhance the overall flow of data processing.
Example: Conditional Reading
std::cout << "Enter a string: ";
char ch = std::cin.peek();
if (ch == 'A') {
std::cout << "You entered a string starting with 'A'." << std::endl;
}
In this scenario, we can preemptively check the first character of the user input before choosing to process it further, creating a more dynamic and responsive input experience.
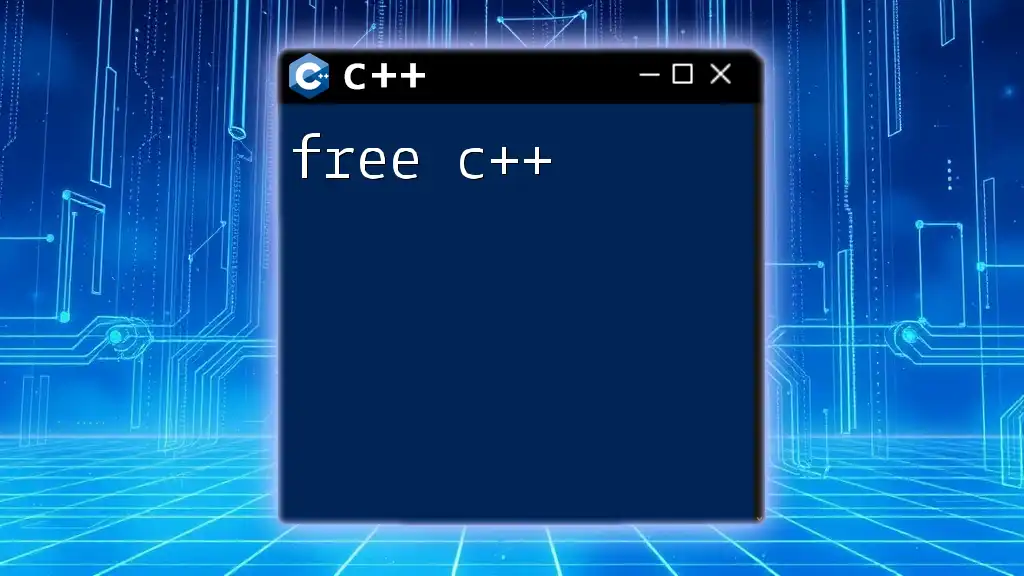
Limitations and Considerations
When Not to Use Peek
While `peek` is useful, it's not suitable for scenarios where immediate consumption of input data is required. Relying too much on `peek` may lead to complex or convoluted code where simpler read methods might suffice.
Performance Considerations
Using `peek`, while generally low-cost, can add overhead if used excessively in performance-critical code. If you find yourself using it too often, consider more efficient methodologies for input processing.
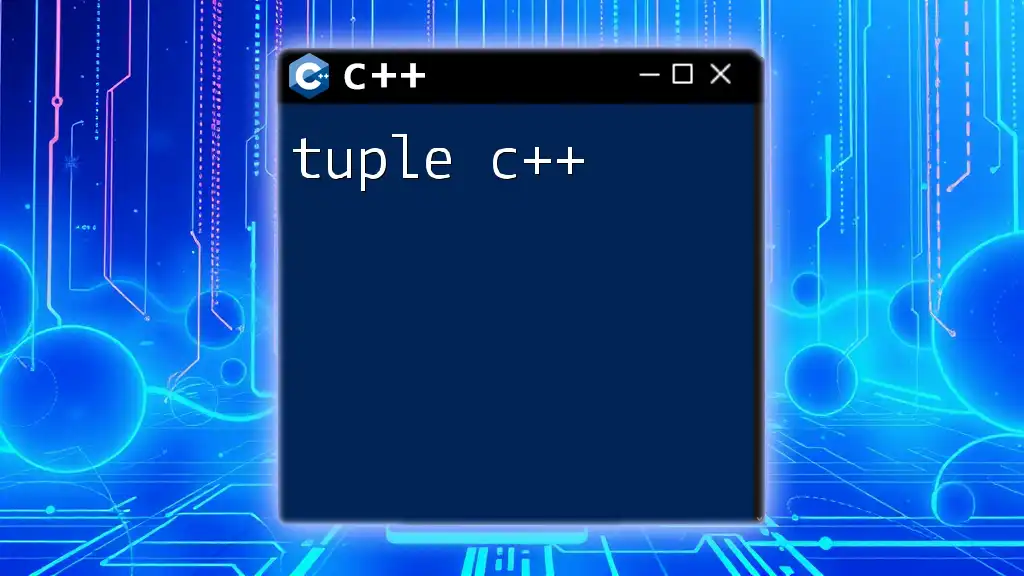
Best Practices for Using Peek in C++
Code Readability and Commenting
When employing `peek` in your code, always prioritize clarity. Write clear comments explaining why you use `peek`, particularly in complex scenarios. This practice makes your code more maintainable and understandable for other developers.
Error Handling with Peek
Always account for potential errors when dealing with streams. Although `peek` helps you look ahead, it’s vital to check if the stream is in a valid state before making assumptions.
Example: Managing Errors
if (inputFile.peek() == EOF) {
std::cerr << "Error: End of file reached without reading!" << std::endl;
}
Here, checking if the `peek` returns `EOF` helps prevent unexpected behavior due to accessing data that's no longer available.
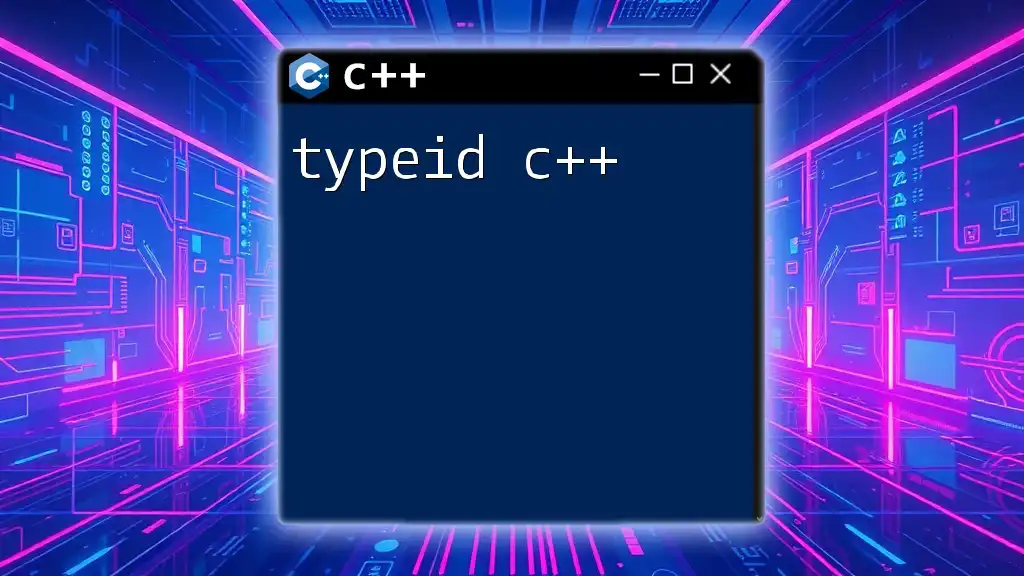
Conclusion
The `peek` function in C++ is an invaluable tool that provides programmers with the ability to preview the next character in an input stream without altering the stream state. Its importance in input processing cannot be overstated, as it allows for more nuanced control over how data is read and processed.
By mastering the `peek` command, along with understanding its best practices and common limitations, developers can create efficient, clear, and stable code that enhances overall application performance. Regularly experimenting with examples and scenarios will deepen your understanding, helping you become more proficient in using `peek` effectively in your C++ programming endeavors.