A tuple in C++ is a fixed-size collection of elements that can hold multiple data types, allowing you to group related values together.
#include <iostream>
#include <tuple>
int main() {
std::tuple<int, std::string, double> myTuple(1, "Hello", 3.14);
std::cout << std::get<0>(myTuple) << ", " << std::get<1>(myTuple) << ", " << std::get<2>(myTuple) << std::endl;
return 0;
}
Understanding Tuples
What is a Tuple?
A tuple is a fixed-size collection of elements, where each element can have a different type. In C++, tuples stand out as a versatile and powerful data structure compared to arrays and structures. Unlike arrays, which require elements to be of the same type, tuples allow mixing various types together, enhancing flexibility when managing data. Furthermore, tuples provide a lightweight alternative to structures for grouping multiple values without the need for defining a full class.
Advantages of Using Tuples
Using tuples in C++ comes with several advantages:
-
Flexible Data Types: Tuples are ideal for scenarios where you wish to store heterogeneous types. Unlike arrays, where all elements need to share a common type, tuples can contain various data types in a single object, which can simplify data manipulation.
-
Immutable Behavior: Once a tuple is created, the size and types of its elements cannot be changed, providing a level of data integrity. This immutability ensures that the tuple retains its values consistently throughout its lifecycle.
-
Ease of Use: Tuples simplify function return types when multiple values need to be returned. Instead of needing to create a struct just to return a few values, a tuple can be returned concisely without added complexity.
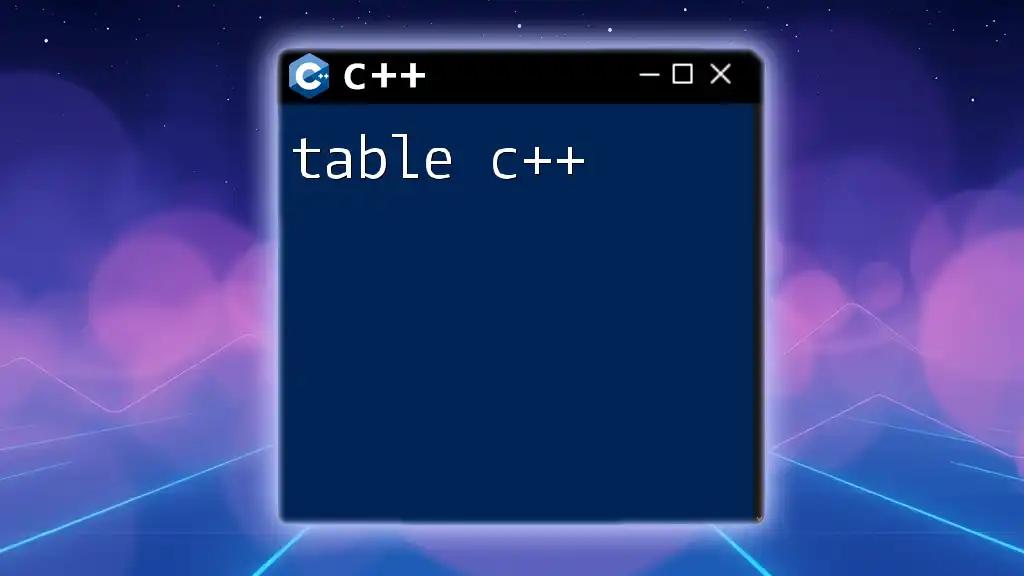
Working with Tuples in C++
Including the Required Header
To make use of tuples in a C++ program, you need to include the `<tuple>` header file:
#include <tuple>
This header provides the necessary functions and types for working with tuples.
Creating a Tuple
Basic Tuple Creation
Creating a tuple is straightforward. Use the `std::tuple` constructor to define a tuple with specific data types:
std::tuple<int, std::string, float> myTuple(1, "example", 3.14);
In this example, `myTuple` consists of an integer, a string, and a float. Each element can be accessed later based on the defined types.
Making Use of `std::make_tuple`
An alternative way to create a tuple is by using `std::make_tuple`, which automatically deduces the types based on the given arguments:
auto anotherTuple = std::make_tuple(2, "sample", 6.28);
This method simplifies type declarations and enhances code readability.
Accessing Tuple Elements
Using `std::get`
To access the elements of a tuple, you can use `std::get`:
std::cout << std::get<1>(myTuple); // Outputs: example
Here, `std::get<1>` retrieves the second element of `myTuple`, which is `"example"`. It's essential to note that the indexing is zero-based.
Tuple Size and Types
You can also determine the size of a tuple using `std::tuple_size`. For example:
constexpr auto size = std::tuple_size<decltype(myTuple)>::value; // size will be 3
This code retrieves the size of `myTuple`, which is 3. Additionally, the `std::tuple_element` template can be used to obtain the type of a specific element in the tuple:
using TupleType = std::tuple_element<0, decltype(myTuple)>::type; // TupleType is int

Manipulating Tuples
Comparing Tuples
Comparing tuples is straightforward in C++. Tuples are compared lexicographically, which means they are compared element by element:
std::tuple<int, float> tuple1(1, 0.1);
std::tuple<int, float> tuple2(1, 0.2);
if (tuple1 < tuple2) {
std::cout << "tuple1 is less than tuple2" << std::endl;
}
In this example, `tuple1` is compared to `tuple2` based on their first and then their second elements.
Swapping Tuples
You can easily swap two tuples using `std::swap`. This function exchanges the values of two tuples:
std::tuple<int, float> t1(10, 20.5);
std::tuple<int, float> t2(30, 40.7);
std::swap(t1, t2);
After executing the `std::swap`, `t1` will hold the values of `t2`, and vice versa.
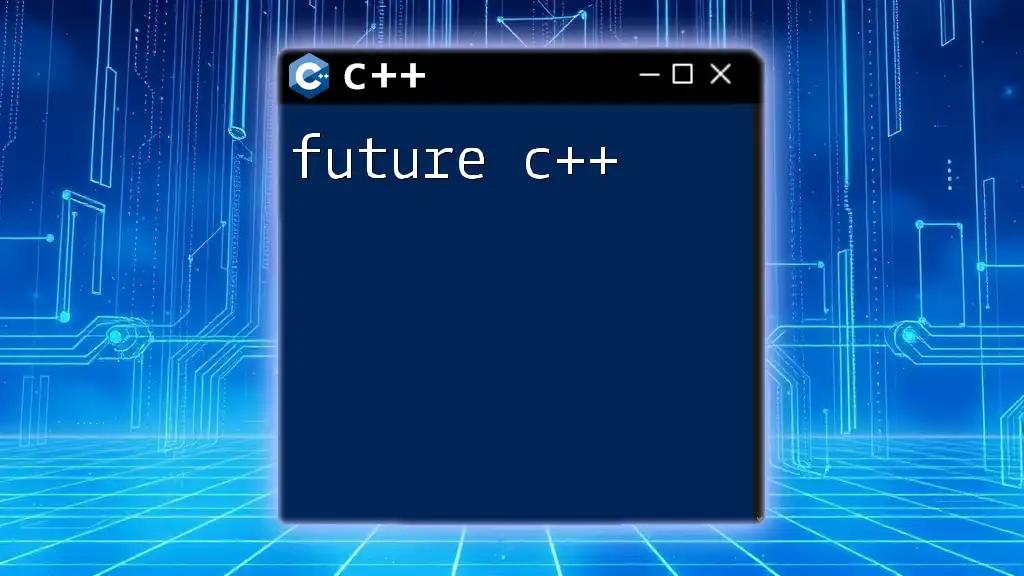
Advanced Tuple Operations
Tuple Iteration
C++17 introduced structured bindings, which allow for easy iteration over tuples. This new syntax provides clarity:
auto [a, b, c] = myTuple;
In this example, `a`, `b`, and `c` will assume the values from `myTuple`, making code easier to read.
Nested Tuples
Tuples can also contain other tuples (nested tuples), which can be useful for complex data organization:
std::tuple<std::tuple<int, int>, float> nestedTuple(std::make_tuple(1, 2), 3.0);
You can access the innermost elements using nested `std::get`:
std::cout << std::get<0>(std::get<0>(nestedTuple)); // Outputs: 1
Tuple and Functions
Returning Multiple Values from Functions
Returning multiple values from functions can be achieved by utilizing tuples:
std::tuple<int, float> returnMultiple() {
return std::make_tuple(5, 2.5);
}
When you call `returnMultiple`, you can capture the returned tuple to access both values directly.

Best Practices and Common Pitfalls
Best Practices for Using Tuples
When leveraging tuples, keep in mind:
- Use tuples when you need to group together related but different types of data. They excel when you want to return multiple heterogeneous values efficiently.
- Avoid excessive complexity by keeping tuples simple and understandable. Overusing tuples for intricate data structures can lead to confusion.
Common Pitfalls
There are some pitfalls to watch out for:
-
Confusing tuple elements without context: Remember that tuples do not provide names for their components. When using tuples, ensure that their context is clear.
-
Overusing tuples for complex data management: While tuples offer flexibility, they should not replace proper data structures such as classes or structs when additional functionality is necessary.
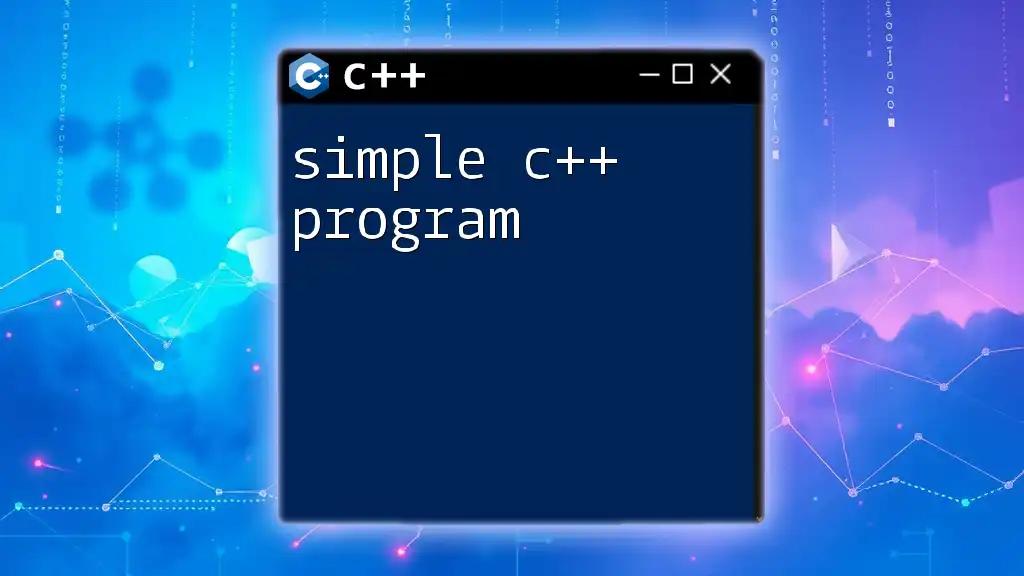
Conclusion
Tuples are a valuable addition to the C++ programmer's toolkit, providing an elegant solution for managing multiple data types collectively. By understanding how to create, manipulate, and utilize tuples, you can streamline your code and enhance efficiency in many programming scenarios. Delve deeper into this topic, and you'll discover countless opportunities to optimize your development processes!