Exploring sample C++ projects can help beginners understand fundamental concepts and improve their coding skills through practical application. Here's a simple C++ program that calculates and displays the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int num;
cout << "Enter a positive integer: ";
cin >> num;
cout << "Factorial of " << num << " = " << factorial(num) << endl;
return 0;
}
Why Choose C++ for Projects?
C++ is a powerful programming language that combines both high performance and flexibility. Its capacity to manage low-level memory while offering high-level object-oriented features makes it an ideal choice for various applications, from systems programming to game development. C++ has a rich library ecosystem and a strong community, which supports developers in building robust applications.
One of the main advantages is the language’s versatility. Understanding C++ opens doors to fields such as embedded systems, financial software, game engines, and even operating system development. Engaging with sample C++ projects equips learners with practical skills and reinforces theoretical knowledge, enabling them to tackle real-world challenges efficiently.
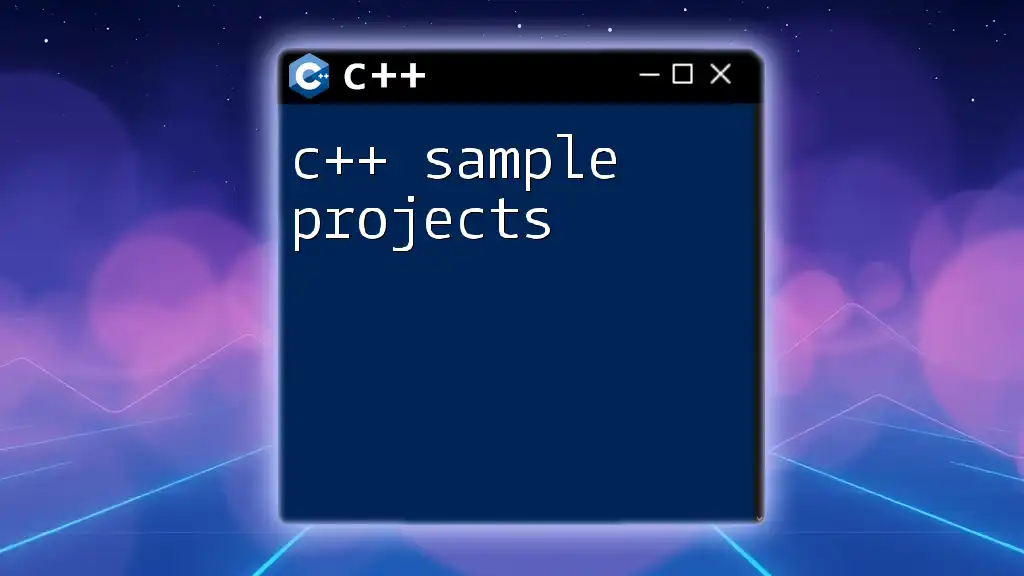
Types of Sample C++ Projects
Beginner Projects
Basic Calculator
Creating a simple command-line calculator is an excellent way to start in C++. This project allows you to grasp essential programming concepts such as input and output, control structures, and simple arithmetic operations.
Code Snippet:
#include <iostream>
int main() {
char op;
double num1, num2;
std::cout << "Enter operator (+, -, *, /): ";
std::cin >> op;
std::cout << "Enter two operands: ";
std::cin >> num1 >> num2;
switch(op) {
case '+': std::cout << num1 + num2; break;
case '-': std::cout << num1 - num2; break;
case '*': std::cout << num1 * num2; break;
case '/': std::cout << num1 / num2; break;
default: std::cout << "Error! operator is not correct"; break;
}
return 0;
}
Learning Points: This project teaches you about basic input/output operations, control structures like switch statements, and handling user inputs.
Tic-Tac-Toe Game
Building a two-player tic-tac-toe game introduces you to array manipulation, loops, and functions, enhancing your understanding of how to handle user interfaces in a console application.
Code Snippet:
#include <iostream>
using namespace std;
char board[3][3] = { {'_', '_', '_'},
{'_', '_', '_'},
{'_', '_', '_'} };
void printBoard() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
// Additional game functions go here
int main() {
printBoard();
// Game loop and user input would be implemented here
return 0;
}
Learning Points: This project solidifies knowledge about arrays and functions, while also providing opportunities to work with loops to create game logic.
Intermediate Projects
Library Management System
As you progress, managing a library system can be a rewarding project. It encompasses various functionalities, including allowing users to add, remove, and search for books and members. This project teaches you to design classes and manage data efficiently.
- Key Features:
- Book Management: Add, remove, and search titles.
- Member Management: Keep records of members and their transactions.
- Transaction Management: Record book checkouts and returns.
Learning Points: This project dives into class design, file handling for data persistence, and overall system architecture.
Basic Chat Application
Creating a command-line chat application introduces fundamental networking concepts. You will learn about sockets, client-server architecture, and how data is transferred over a network.
Learning Points: This project helps in understanding network programming and multi-threading, essential skills for real-world applications.
Advanced Projects
Game Development with SDL
Using the Simple DirectMedia Layer (SDL) library to develop a simple game provides an excellent opportunity to explore graphics rendering and user input handling.
Example Concepts:
- Initializing SDL and creating a game window
- Rendering graphics and handling keyboard inputs
Learning Points: Engaging with game development enhances your skills in event-driven programming, graphics rendering, and multimedia handling, making it a fun yet challenging project.
File Compression Tool
Developing a file compression tool using algorithms like Huffman coding is a complex but highly rewarding project. This task involves in-depth knowledge of data structures and algorithms.
Learning Points: This project will deepen your understanding of data structures, algorithm implementation, and file I/O operations, as you work through compressing and decompressing files.
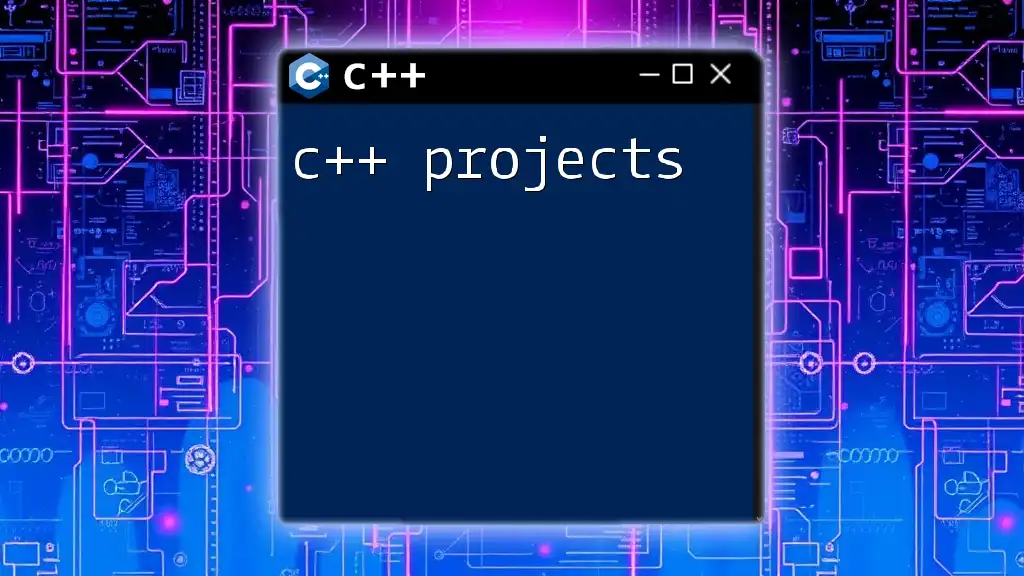
Tips for Completing C++ Projects
Planning and Design
Before diving into coding, planning and design are crucial. Sketch a rough design of your program and break it down into smaller, manageable components. This approach ensures you have a roadmap to follow, reducing the likelihood of getting lost in complexities.
Debugging Techniques
Debugging is an essential part of the development cycle. Utilizing methods like print statements can help identify where things may have gone wrong. Also, familiarize yourself with debugging tools available for C++, such as GDB (GNU Debugger), to streamline the process.
Best Practices in C++
Following coding best practices is key to writing clean and maintainable code. Here are a few tips:
- Code organization: Separate declarations in header files and definitions in source files.
- Naming conventions: Use descriptive names for variables and functions to improve code readability.
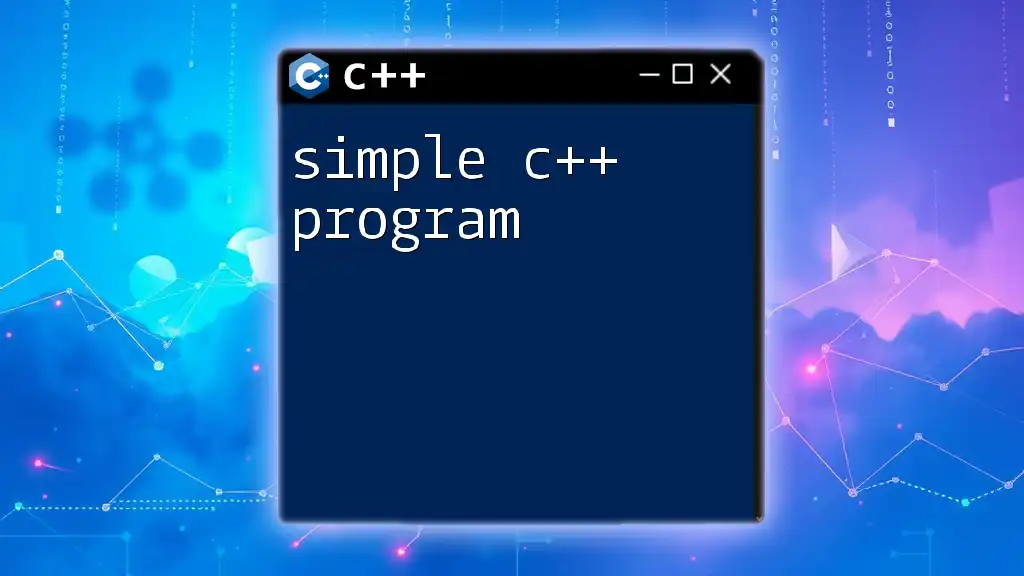
Resources for Further Learning
If you are interested in continuing your education in C++, here are some resources:
- Books like "C++ Primer" by Lippman et al. and "Effective C++" by Scott Meyers.
- Websites and online courses, including platforms like Coursera, Udacity, or Codecademy, that offer structured learning paths.
- Explore open-source C++ projects on platforms like GitHub for practical insights and inspiration.
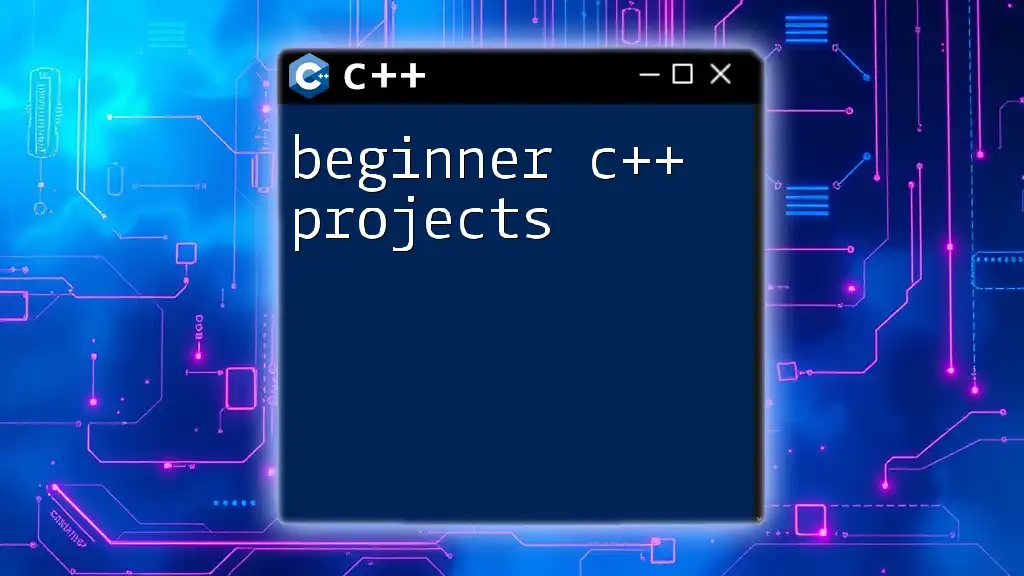
Conclusion
Completing sample C++ projects allows learners to apply theoretical knowledge and build practical skills. Starting with simpler projects and gradually taking on more complex challenges enables a deeper understanding of programming concepts. Embark on one of the projects mentioned, and let your learning journey be enriched by both success and the challenges you overcome. Don’t forget to share your experiences and seek feedback in the community; it’s all part of the growth process.
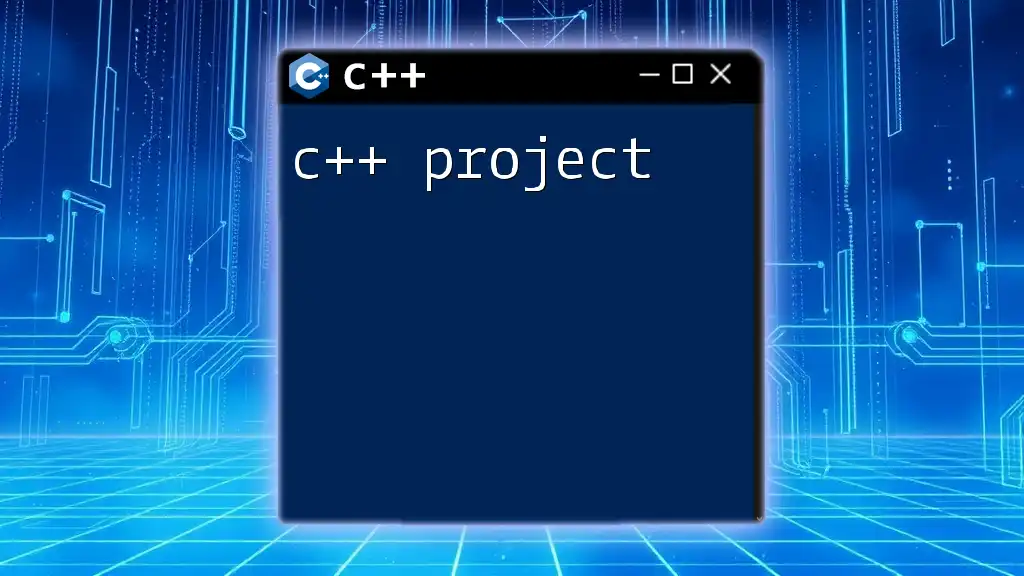
FAQs
What is the best project to start with as a beginner? Starting with a basic calculator is highly recommended as it lays the groundwork for understanding core concepts.
How can I progress from beginner to advanced projects? Incrementally tackle more challenging projects like Tic-Tac-Toe and subsequently move on to intermediate projects like the Library Management System.
Where can I find more sample C++ projects? You can explore resources such as GitHub, coding challenge sites, and C++ programming communities for additional project ideas and inspiration.