For beginners and seasoned programmers alike, creating impactful C++ projects can greatly enhance your portfolio by showcasing your programming skills and understanding of real-world applications. Here's a simple C++ project example that creates a basic calculator:
#include <iostream>
int main() {
double num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter second number: ";
std::cin >> num2;
std::cout << "Enter operation (+, -, *, /): ";
std::cin >> operation;
switch (operation) {
case '+':
std::cout << "Result: " << num1 + num2 << std::endl;
break;
case '-':
std::cout << "Result: " << num1 - num2 << std::endl;
break;
case '*':
std::cout << "Result: " << num1 * num2 << std::endl;
break;
case '/':
if (num2 != 0)
std::cout << "Result: " << num1 / num2 << std::endl;
else
std::cout << "Error: Division by zero" << std::endl;
break;
default:
std::cout << "Invalid operation" << std::endl;
}
return 0;
}
Why Having a C++ Portfolio Matters
Having a portfolio filled with compelling C++ projects is essential for distinguishing yourself in today's competitive job market. A well-crafted portfolio demonstrates your practical skills, showcasing your ability to translate theoretical knowledge into real-world applications. Employers are not only interested in your academic credentials; they want to see your problem-solving abilities and how you can implement C++ concepts effectively in tangible projects.
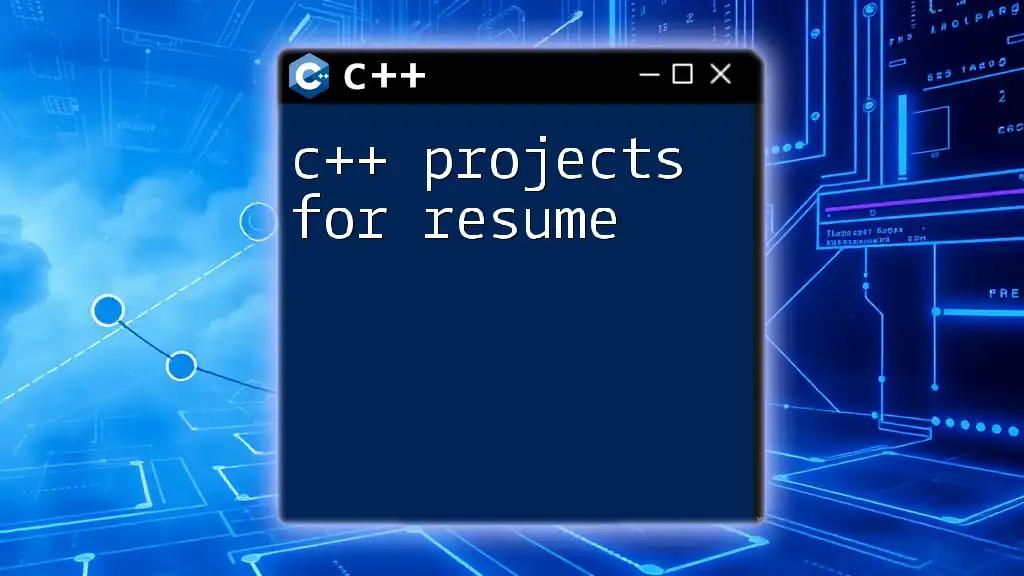
Choosing the Right Projects for Your Portfolio
When selecting C++ projects for portfolio submission, consider several factors:
- Complexity Levels: Begin with simpler projects and gradually move to intermediate and advanced levels. This progression showcases your growth and adaptability over time.
- Personal Interest: Selecting projects that resonate with your interests will keep you motivated and ensure a better final product.
- Usefulness in Job Applications: Aim for projects that illustrate skills relevant to the positions you’re targeting. Research the job descriptions to align your portfolio accordingly.
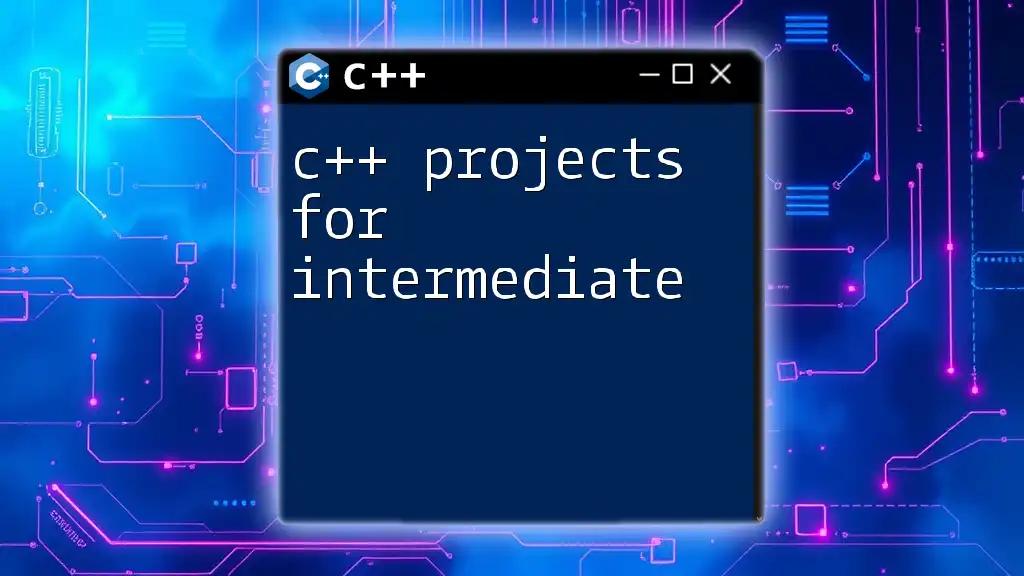
Beginner-Level C++ Projects
Simple Calculator
A simple calculator project is an excellent starting point to demonstrate your fundamental understanding of input/output operations and control structures.
Project Overview: It performs basic arithmetic operations such as addition, subtraction, multiplication, and division, and captures user input for operands and operations.
Code Explanation:
#include <iostream>
using namespace std;
int main() {
float num1, num2;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter second number: ";
cin >> num2;
cout << "Enter operation (+, -, *, /): ";
cin >> operation;
switch (operation) {
case '+':
cout << "Result: " << num1 + num2 << endl;
break;
case '-':
cout << "Result: " << num1 - num2 << endl;
break;
case '*':
cout << "Result: " << num1 * num2 << endl;
break;
case '/':
cout << "Result: " << num1 / num2 << endl;
break;
default:
cout << "Invalid operation!" << endl;
break;
}
return 0;
}
Skills Demonstrated: This project highlights your grasp of basic data types, conditionals, and the use of switch statements in C++, all of which are fundamental for further programming skills.
Tic-Tac-Toe Game
Creating a Tic-Tac-Toe game is another excellent beginner project that introduces the concept of array manipulation and user interaction in a fun way.
Project Overview: It captures user input to place Xs and Os on a board, and determines the game winner.
Key Features: The project emphasizes board representation and user input validation.
Code Snippet:
// Code snippet to represent a Tic-Tac-Toe board
char board[3][3] = {{' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '}};
Skills Demonstrated: This game showcases your ability to work with two-dimensional arrays, loops, and basic game logic.
To-Do List Application
A To-Do List application emphasizes handling data and user interactions, making it perfect for beginners wanting to learn about CRUD operations (Create, Read, Update, Delete).
Project Overview: Users can add tasks, mark them as complete, and delete tasks as needed.
Code Example:
#include <vector>
#include <string>
class TodoItem {
public:
std::string task;
bool completed;
// Add methods here to manage tasks
};
Skills Demonstrated: This project allows you to explore object-oriented programming (OOP) and the management of collections through vectors.
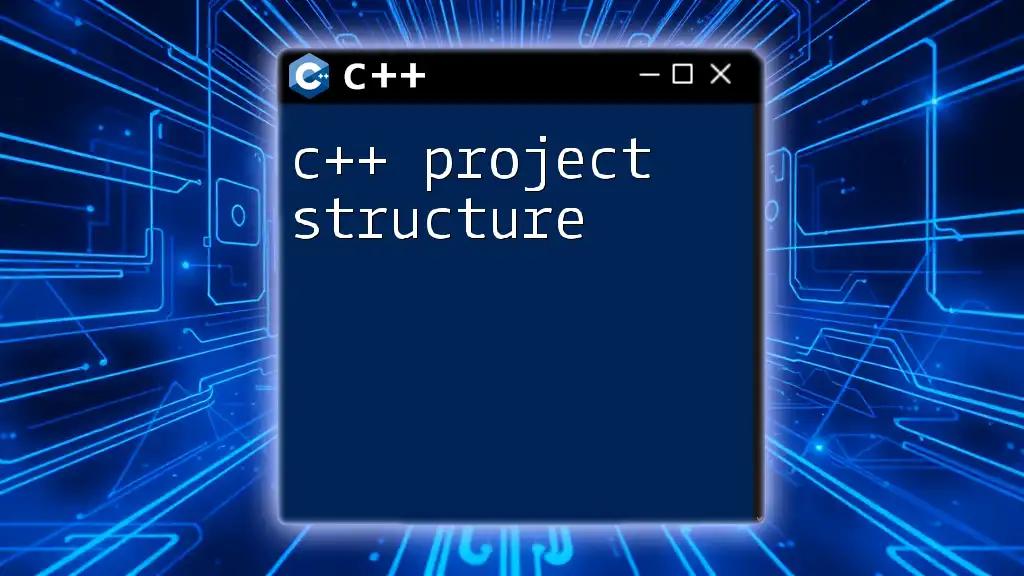
Intermediate-Level C++ Projects
Personal Finance Manager
At the intermediate level, a personal finance manager project provides a deeper exploration of structured data management and user interaction.
Project Overview: The application helps users track income and expenses, offering insights into their financial health.
Data Structures and Algorithms Used: You can utilize linked lists or vectors to store transactions effectively.
Example Code Snippet:
struct Transaction {
std::string description;
float amount;
std::tm date; // Using standard library for date handling
};
Skills Demonstrated: This project highlights your understanding of data types, structures, and basic financial algorithms.
Maze Solver
This project introduces algorithms and pathfinding, presenting significant coding challenges and logical thinking.
Project Overview: A maze solver uses algorithms to find a path through a grid from start to finish.
Algorithms to Demonstrate: Implementing Depth-First Search (DFS) or the A* algorithm showcases advanced problem-solving abilities.
Code Snippet:
bool solveMaze(int maze[N][N], int path[N][N], int x, int y);
Skills Demonstrated: This project highlights the ability to implement recursive algorithms and manage complex logic through condition checking.
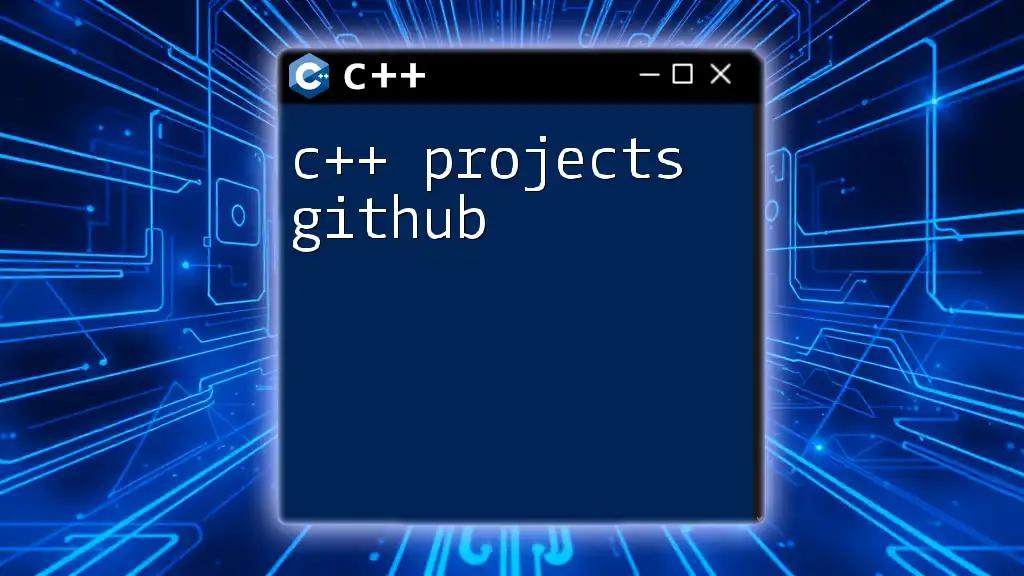
Advanced C++ Projects
Game Development with C++
Game development is a hands-on way to learn complex programming paradigms and software architecture.
Project Overview: Create a 2D game such as Space Invaders, where you will design game mechanics, graphics rendering, and user controls.
Libraries to Use: Libraries like SDL or SFML make it accessible to create rich graphical experiences.
Challenges Addressed: This project encapsulates advanced concepts such as graphics rendering, collision detection, and effective game loops.
Chat Application
Building a chat application helps you understand networking and multi-threading in C++.
Project Overview: The app enables real-time messaging between users, focusing on server-client architecture.
Technologies Required: Familiarity with sockets and multi-threading is essential.
Code Example:
void * handleClient(void * arg);
Skills Demonstrated: This project showcases concurrency management, data synchronization, and the understanding of network protocols.
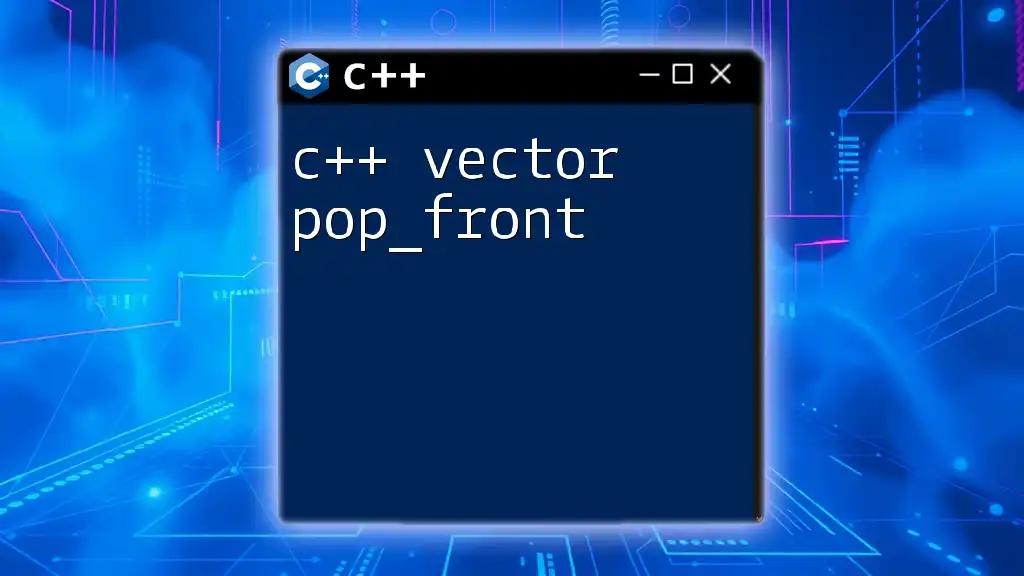
Highlighting Your Projects in Your Portfolio
Ultimately, the presentation of your projects is as critical as the projects themselves.
Presentation Techniques: Clear documentation is vital for demonstrating your thought process and understanding of the code. Use comments liberally within your code to clarify complex logic, and generate README files that explain the project purpose, setup steps, and usage instructions.
Using GitHub for Showcase: An effective way to showcase your projects is through a well-structured GitHub repository. Follow best practices with headings, descriptions, and appropriate file structures.

Conclusion
In conclusion, building a portfolio of C++ projects is a crucial step in your programming journey. Starting from simple projects and gradually advancing to more complex ones not only enhances your coding skills but also prepares you for job applications in software development. Remember to document your work, highlight your skills, and take pride in your progress as you create an impressive portfolio.
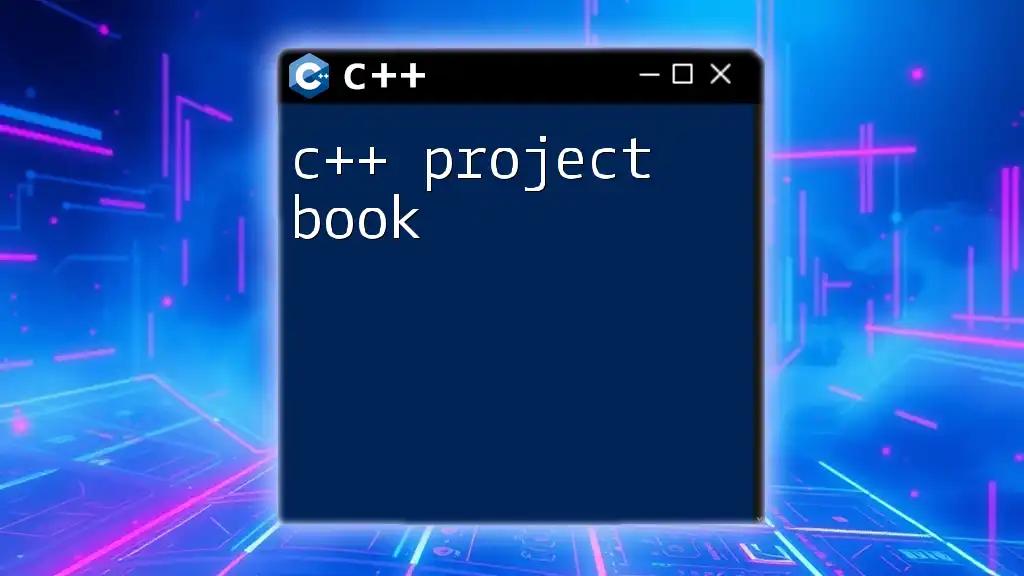
Additional Resources
To further enrich your understanding and expertise in C++, consider checking out recommended books such as "C++ Primer" and "Effective C++." Online courses on various educational platforms can also supplement your learning and provide more structured guidance as you build your projects. Happy coding!