In C++, the `std::vector` class does not have a `pop_front` method, but you can achieve similar functionality by removing the first element using `erase` in combination with `begin()`.
Here's how you can do it:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin()); // Removes the first element (1)
for (int num : vec) {
std::cout << num << " "; // Output: 2 3 4 5
}
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is part of the Standard Template Library (STL) and represents a dynamic array that can change its size during program execution. Vectors provide a flexible data structure that can grow or shrink as needed, allowing for efficient memory usage. Unlike static arrays, vectors can adjust their capacity automatically based on requirements, making them highly versatile in handling collections of data.
Key Features of Vectors
- Automatic resizing: Vectors can expand or contract automatically as elements are added or removed. This feature eliminates the need for manual memory management.
- Random access: Vectors support direct access to their elements through indexing, enabling quick retrieval and manipulation.
- Compatibility with STL algorithms: Vectors work seamlessly with numerous STL algorithms, providing functional flexibility.
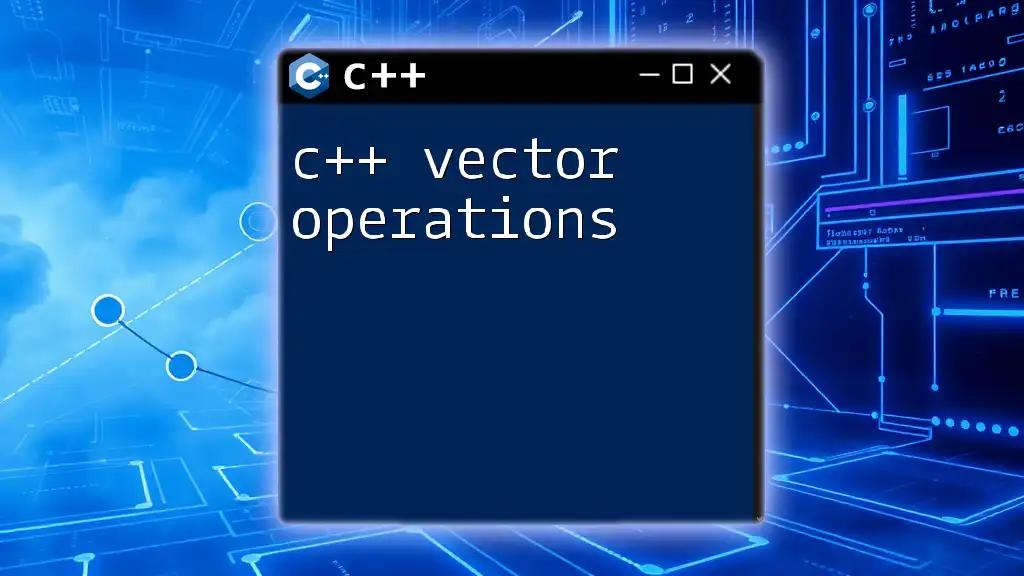
The pop_front Concept Explained
What is pop_front?
The term pop_front refers to the operation of removing the first element from a container, such as a vector or a deque. While many data structures in C++ provide a method for removing elements from the back (like `pop_back()`), C++ vectors do not natively support `pop_front()`. This design choice stems from the underlying mechanics of how vectors operate, since removing the first element necessitates shifting all subsequent elements down to fill the gap.
Why pop_front is not a default function in C++
Implementing `pop_front()` in a vector comes with performance implications. Shifting elements to maintain the contiguous nature of the array results in a time complexity of O(n), where n is the number of elements in the vector. This inefficiency can lead to performance bottlenecks, which is why vector operations are generally optimized for `pop_back()`—an O(1) operation, offering better performance for stack-like behavior.
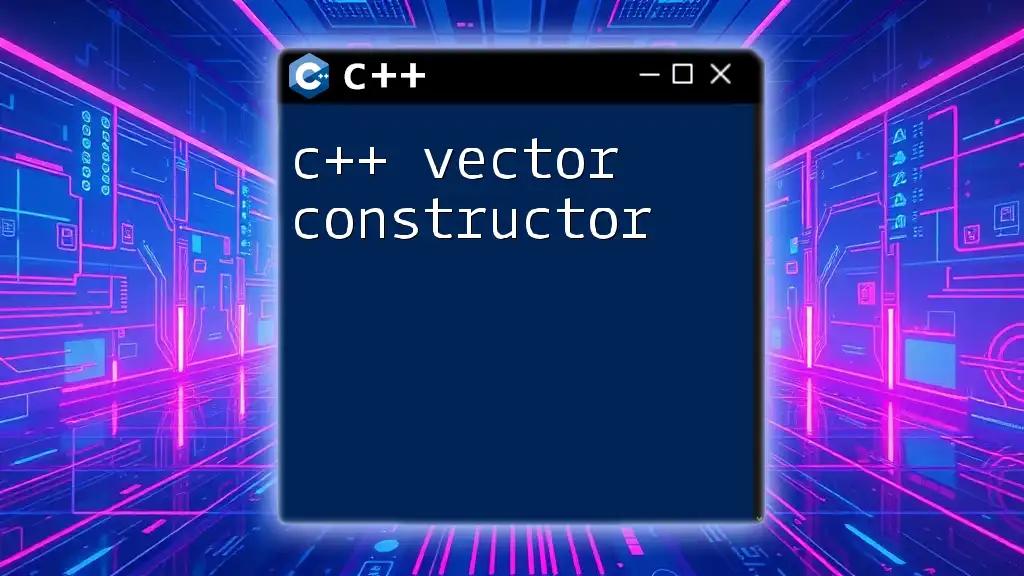
Implementing pop_front in C++
Using Standard Libraries
While there is no native `pop_front()` function in C++, you can implement the functionality using the `erase()` method from the STL. By invoking `erase()` at the beginning of the vector, you can efficiently remove the first element.
Code Snippet: Implementation of pop_front
#include <iostream>
#include <vector>
void pop_front(std::vector<int>& vec) {
if (!vec.empty()) {
vec.erase(vec.begin());
}
}
int main() {
std::vector<int> myVec = {10, 20, 30, 40, 50};
pop_front(myVec);
for (int val : myVec) {
std::cout << val << " ";
}
// Output: 20 30 40 50
return 0;
}
In this code, the function `pop_front()` first checks if the vector is empty to avoid errors. If it's not, it successfully removes the first element by calling `vec.erase(vec.begin())`. The main function showcases its use and prints the updated vector after the first element has been removed.
Performance Analysis
Time Complexity of pop_front
The `pop_front()` operation implemented via `erase()` has a time complexity of O(n) since it requires shifting all remaining elements to fill the gap left by the removed element. This performance consideration is crucial when deciding whether to utilize vectors for applications requiring frequent removals from the front.
Memory Considerations
When elements are erased from a vector, it may not always shrink its capacity. The `erase()` method alters the size of the vector but doesn't necessarily reclaim memory. This distinction is essential in managing both capacity and size, as unoptimized memory handling can lead to inefficient space usage.
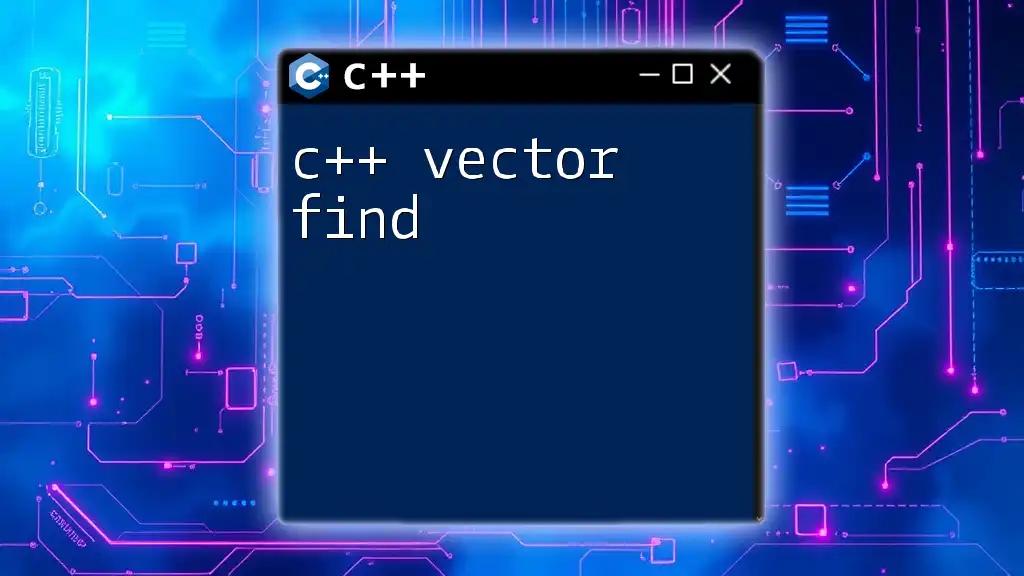
Alternative Solutions to pop_front
Using Deques
If `pop_front()` functionality is frequently needed, consider using a `std::deque` (double-ended queue) instead. Deques are specifically designed to allow fast insertions and deletions at both ends, making `pop_front()` an O(1) operation.
Code Snippet: Using Deque
#include <iostream>
#include <deque>
void pop_front(std::deque<int>& dq) {
if (!dq.empty()) {
dq.pop_front();
}
}
int main() {
std::deque<int> myDeque = {10, 20, 30, 40, 50};
pop_front(myDeque);
for (int val : myDeque) {
std::cout << val << " ";
}
// Output: 20 30 40 50
return 0;
}
In this deque implementation, the `pop_front()` function uses the built-in `pop_front()` method, which efficiently removes the first element without the need for element shifting.
Best Practices for Managing C++ Vectors
Maintaining Vector Integrity
When working with vectors, always ensure proper initialization with the correct size and type. Additionally, be cautious of iterator invalidation scenarios, especially after operations that modify the vector.
Performance Tips
To enhance performance, consider using `reserve()` to preallocate memory when you know the vector will store a specific number of elements. This practice can improve insertion speeds by reducing the need for repeated reallocations as elements are added.
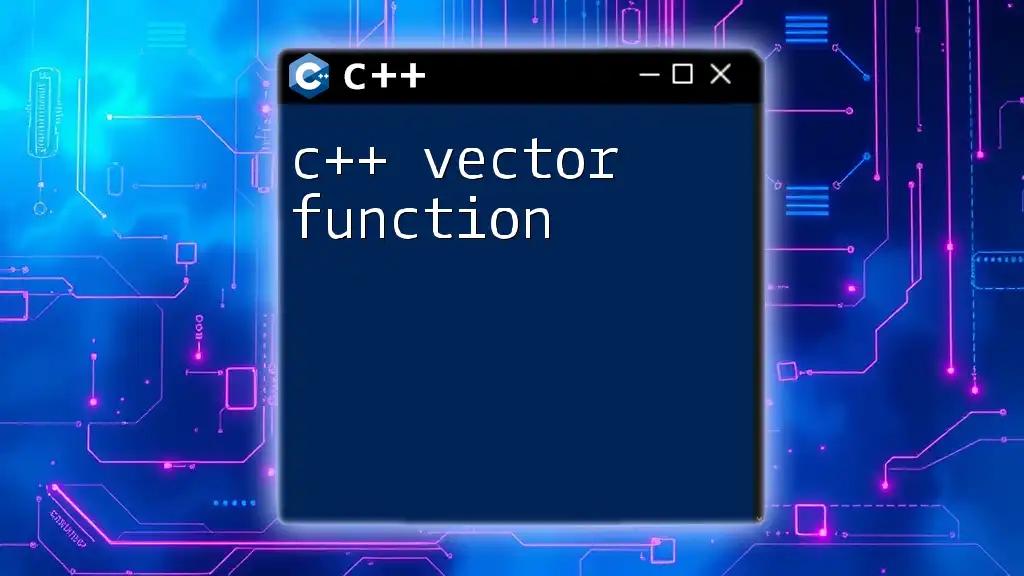
Conclusion
In summary, understanding the C++ vector pop_front concept is essential when manipulating collections in C++. While vectors do not natively support `pop_front()`, you can implement this functionality through the `erase()` method. However, for frequent operations that require removing elements from the front, `std::deque` offers a more efficient alternative. With proper techniques and cautious memory management, you can leverage the strengths of these data structures for optimal performance in your C++ applications.

Additional Resources
For further learning, consider exploring the official C++ documentation on vectors and deques. Practicing with coding exercises on platforms like LeetCode or HackerRank can also enhance your understanding of these concepts.