In C++, the `std::find` algorithm can be used to locate an element within a vector, returning an iterator to the found element or the end iterator if the element is not present.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a part of the Standard Template Library (STL) and represents a dynamic array that can grow in size as needed. Unlike traditional arrays, vectors provide the advantage of automatic memory management, resizing, and a host of utility functions. This makes them extremely flexible and convenient for dynamic storage of elements.
One key feature of vectors is their dynamic size. As elements are added or removed, vectors automatically manage memory allocation, making it easier for programmers to work with collections of data without having to manually handle memory size.
Basic Vector Operations
Creating Vectors: Vectors can be easily declared and initialized. Here's how:
#include <vector>
std::vector<int> myVector; // An empty vector of integers
std::vector<int> initializedVector = {1, 2, 3}; // A vector initialized with values
Adding Elements: The `push_back()` function allows you to add elements to the end of the vector. For example:
myVector.push_back(4);
myVector.push_back(5);
This will result in `myVector` containing `{4, 5}`.
Accessing Elements: Vector elements can be accessed using the subscript operator. For instance:
int firstElement = myVector[0]; // Accessing the first element
This code snippet retrieves the first element of `myVector`.
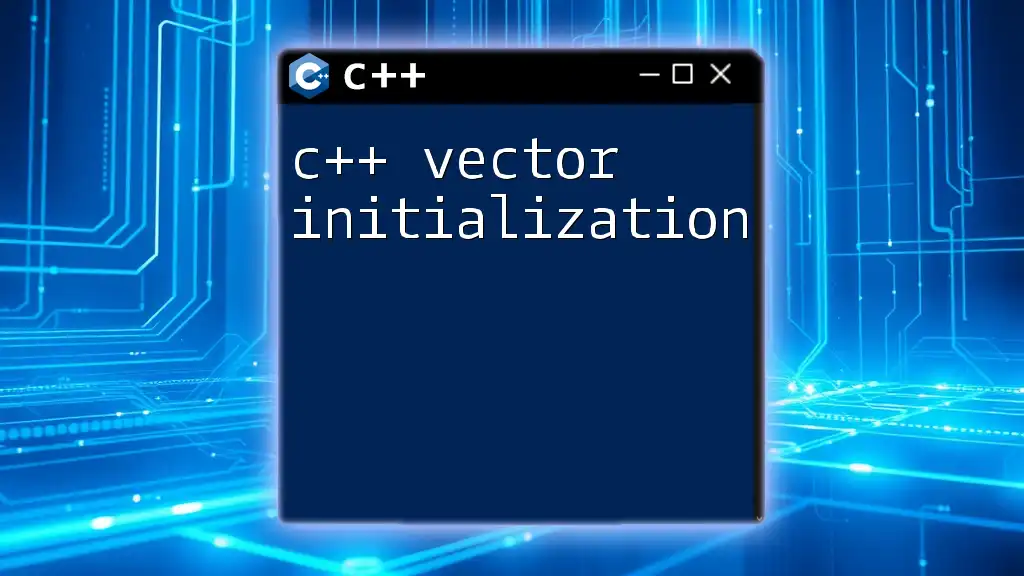
The Find Operation in Vectors
What Does It Mean to Find an Element?
Finding an element within a vector is a fundamental operation that involves searching for a specific value and determining its position. This operation is extremely useful in various programming scenarios, such as searching for a user's input within a list of values or finding specific settings in an array of configurations.
Manual Search Technique
Linear Search Explained
The simplest way to search for an element in a vector is through linear search, where each element is checked one-by-one until the target element is found or all elements are exhausted.
Here’s an implementation of linear search:
#include <vector>
#include <iostream>
int linearSearch(std::vector<int> vec, int target) {
for (int i = 0; i < vec.size(); i++) {
if (vec[i] == target) {
return i; // Return the index of the found element
}
}
return -1; // Return -1 if not found
}
In this example, if the target value is present, the index of the element is returned; otherwise, -1 indicates that the element was not found. This straightforward approach is easy to implement but can be inefficient for large datasets due to its linear time complexity O(n).
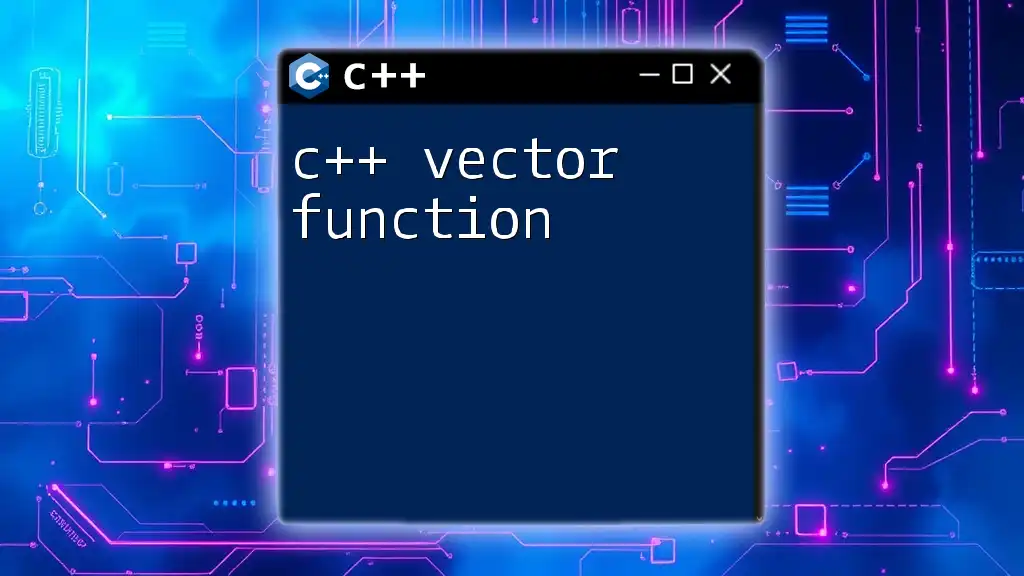
Using STL Algorithms for Finding Elements
Introduction to STL
The Standard Template Library (STL) offers a set of C++ template classes that provide general-purpose classes and functions. One of the major benefits of using STL is its ready-to-use algorithms, like searching, which can save you from writing boilerplate code.
The `std::find` Function
Overview of `std::find`
The `std::find` function allows you to search for a value within a range, such as a vector. It comes from the `<algorithm>` header and is more optimized than manual searching.
How to Use `std::find`
You can use `std::find` as shown in the following code snippet:
#include <vector>
#include <iostream>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found at index: " << std::distance(vec.begin(), it) << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
In this example, `std::find` searches through `vec` for the value `3`. If found, it returns the iterator pointing to that element, and we use `std::distance` to convert the iterator to an index.
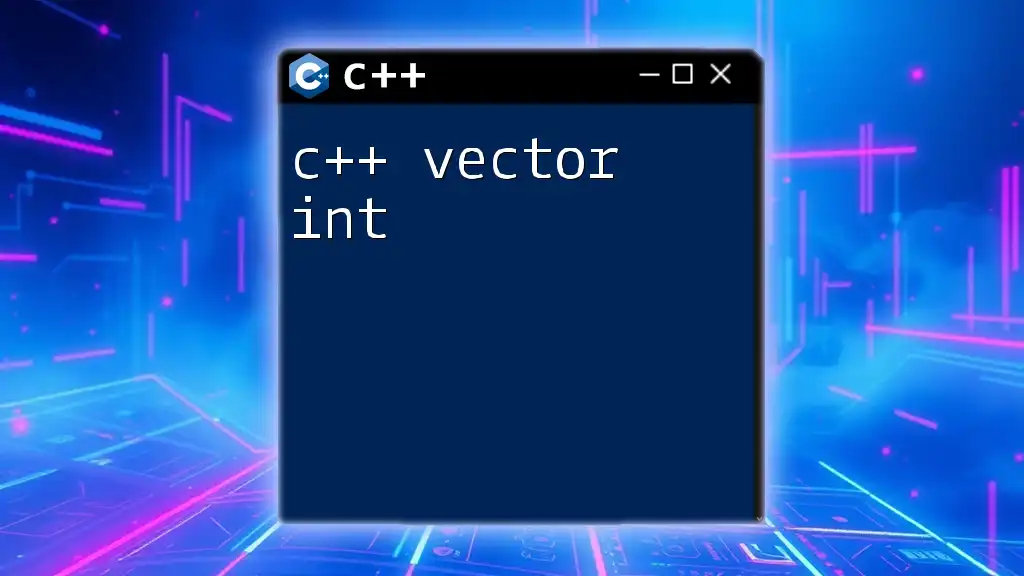
Comparing Manual Search vs STL Search
Performance Considerations
When it comes to performance, linear search has a time complexity of O(n), making it less efficient for larger data sets. In contrast, while `std::find` also performs a linear search internally, its optimized implementation tends to be faster and cleaner.
When deciding whether to use manual searching or STL functions, consider using STL for most cases as it enhances code readability and maintenance.
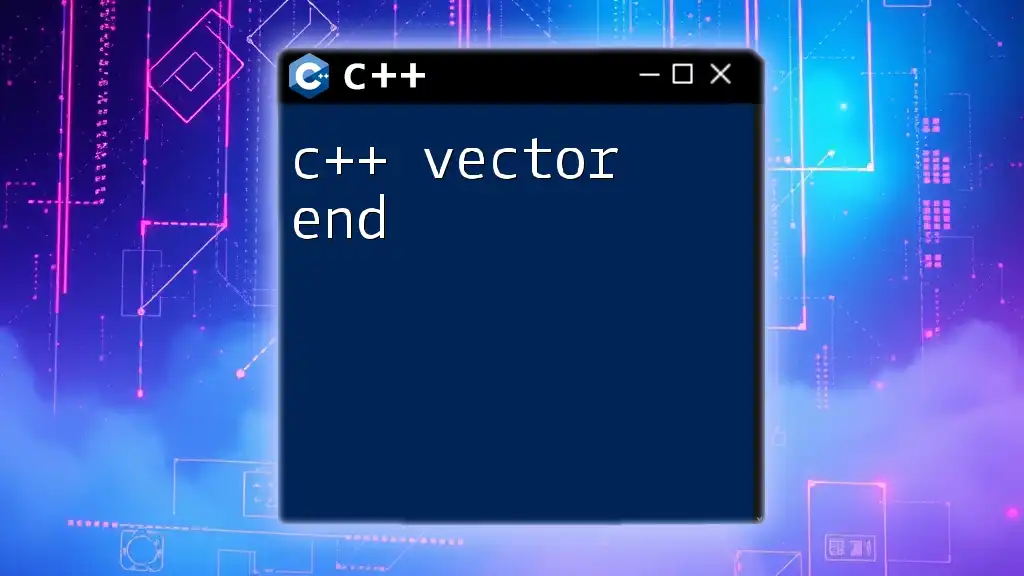
Advanced Searching Techniques
Using Lambdas with `std::find_if`
To enhance search capabilities, you can specify conditions using lambda functions with `std::find_if`. This technique allows you to create custom search criteria based on your needs.
Here's an example:
#include <vector>
#include <iostream>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find_if(vec.begin(), vec.end(), [](int n) { return n > 3; });
if (it != vec.end()) {
std::cout << "First element greater than 3: " << *it << std::endl;
}
return 0;
}
In this code, `std::find_if` searches for the first element greater than `3`. The lambda function `[ ](int n) { return n > 3; }` acts as the condition for the search.
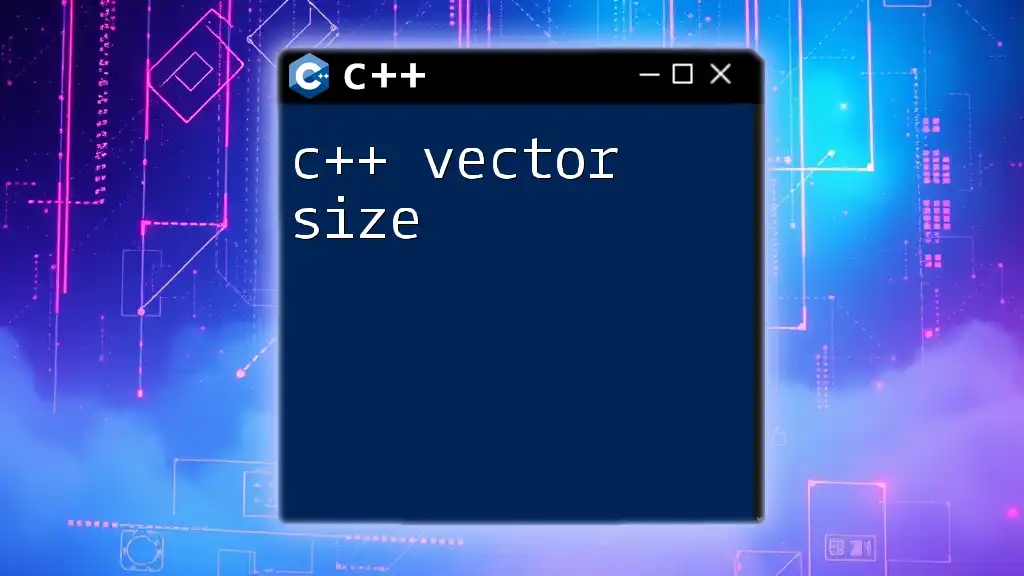
Conclusion
The ability to find elements efficiently in vectors is crucial in C++. Knowing the difference between manual methods and STL algorithms can lead to better coding practices and optimized performance. Whether you choose to rely on manual searching techniques or leverage the power of STL, what's important is understanding the underlying operations and choosing the appropriate method for your specific context.
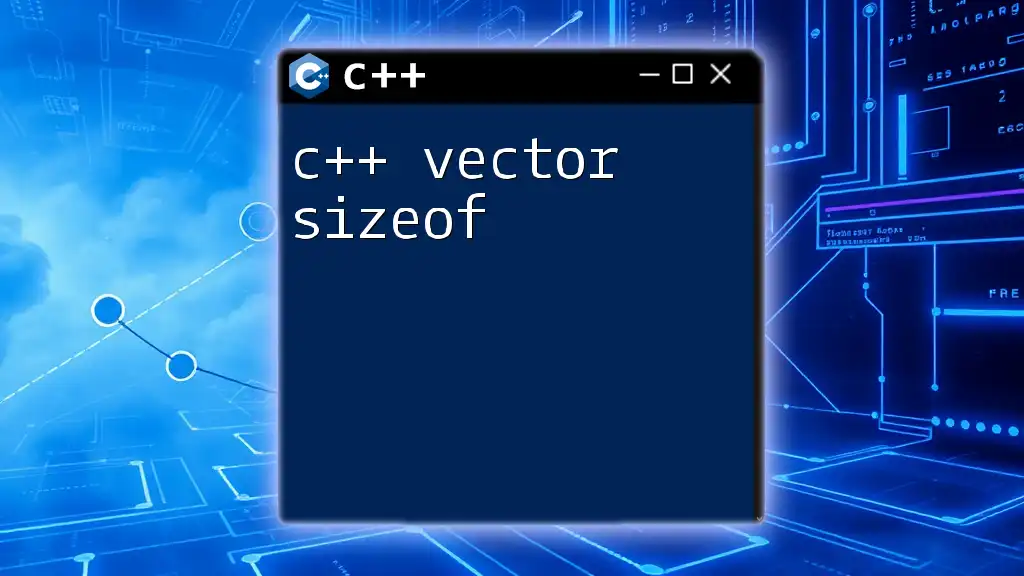
Additional Resources
For further exploration of C++ vectors and advanced searching techniques, consider seeking out comprehensive tutorials and resources specific to C++. Books, coding challenges, and online courses can deepen your knowledge and enhance your programming skills in this powerful language.
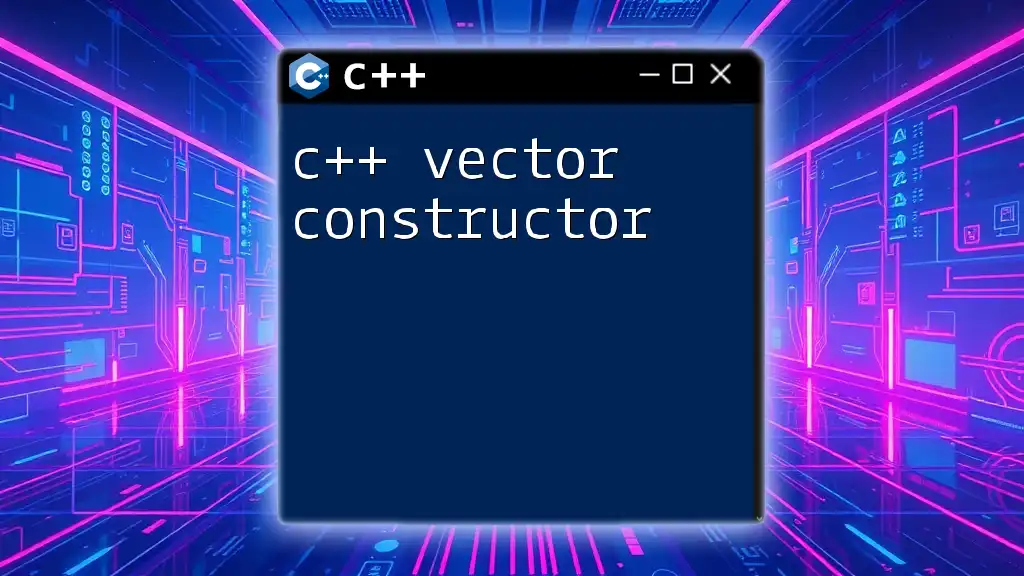
Call to Action
Have you implemented searching in C++ vectors? Share your experiences and challenges in the comments below. Consider signing up for our newsletter to stay updated with the latest tips and tutorials on mastering C++ commands!