In C++, the `for_each` algorithm from the `<algorithm>` header applies a given function to each element in a vector, allowing for concise iteration and manipulation of the elements.
Here's a code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::for_each(vec.begin(), vec.end(), [](int x) {
std::cout << x << " ";
});
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a dynamic array that can grow and shrink in size as elements are added or removed. It is part of the Standard Template Library (STL) and provides a robust means to handle collections of data. Vectors hold elements of the same type, and they offer several advantages over traditional arrays, primarily dynamic memory management and built-in functions.
To create a simple vector, you can use:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers; // Creating an empty vector of integers
return 0;
}
Why Use Vectors?
Vectors are favored in C++ programming due to their flexibility and functionality. Here are some key benefits:
- Dynamic sizing: Unlike arrays, vectors can automatically resize when elements are added or removed, which simplifies memory management.
- Rich functionality: Vectors come with built-in methods such as `push_back()`, `pop_back()`, and sorting algorithms to manage data efficiently.
- Ease of iteration: Vectors are designed to be easily traversed with loops, enhancing readability and maintainability of the code.
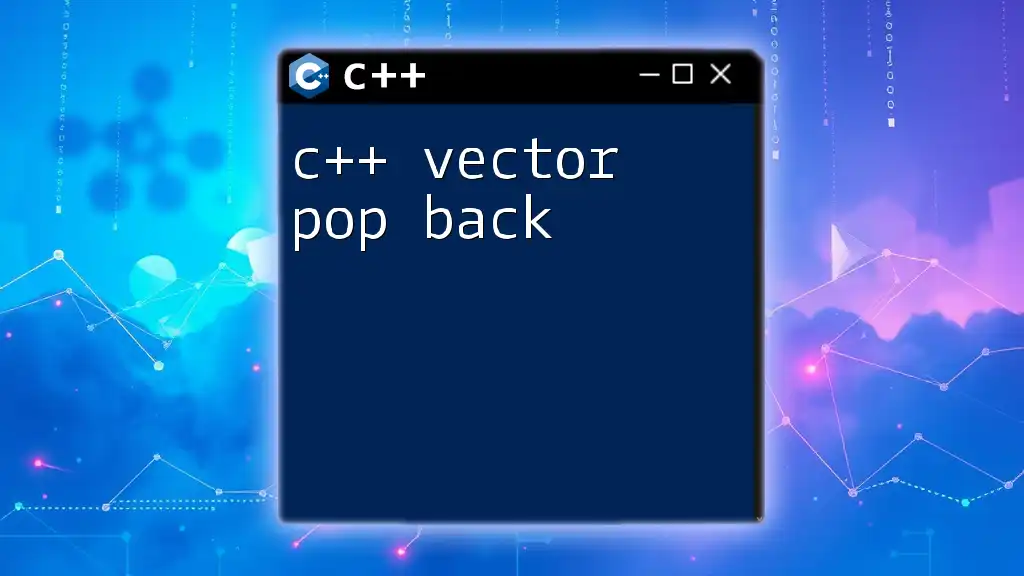
C++ Vector For Each: The Basics
What is the For Each Loop?
The for each loop concept allows you to iterate over a collection of items, providing a cleaner and more concise syntax compared to traditional loops. It abstracts away the underlying indices, making your code more readable and reducing the chance of indexing errors.
Syntax of For Each in C++
The basic structure of a for each loop in C++ looks like this:
for (const auto& element : vector) {
// Use element
}
In this syntax, `auto&` allows for automatic type deducing, while the `const` keyword ensures the original vector elements remain unchanged.
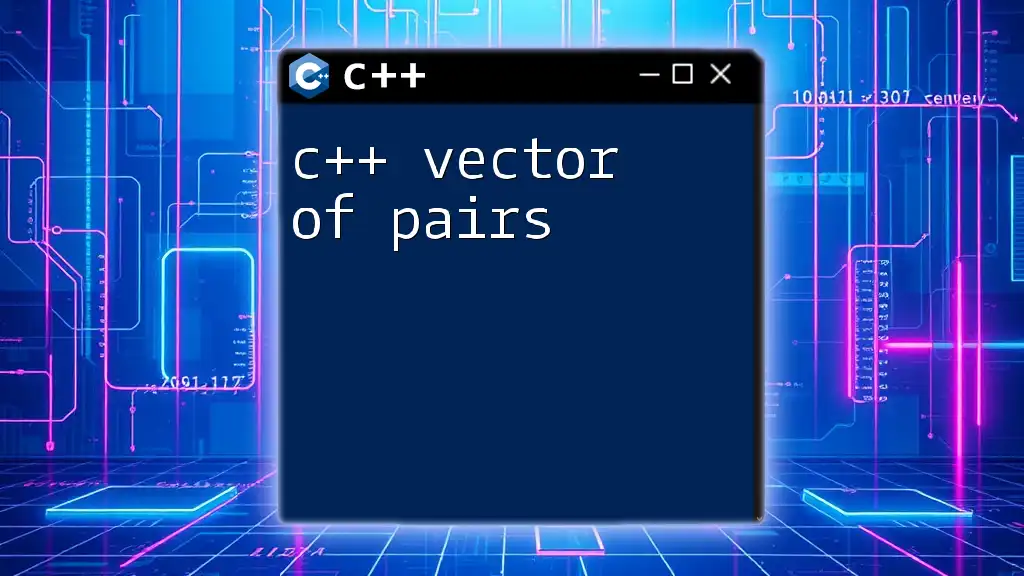
Iterating Through Vectors with For Each
Using Range-Based For Loop
The range-based for loop in C++ is one of the simplest and most efficient ways to iterate through a vector. This loop automatically handles the iteration process without needing to specify an index.
Here's a simple example of iterating through a vector using the range-based for loop:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
This outputs: `1 2 3 4 5`. The advantage of using a range-based for loop is its clarity; it explicitly conveys the intent to traverse every element in the vector.
Using Standard Library Algorithms
Introduction to Standard Library Algorithms
C++'s Standard Library offers numerous built-in algorithms designed to work seamlessly with containers like vectors. These algorithms can simplify data manipulation and reduce the need for boilerplate code.
Using `std::for_each` with C++ Vectors
The `std::for_each` function is a powerful way to apply an operation over all elements in a vector. This is how you can implement it:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int num) {
std::cout << num << " ";
});
return 0;
}
In this example, the lambda function passed to `std::for_each` outputs each number in the vector. This approach is highly flexible and can be adapted to modify elements or perform complex operations.
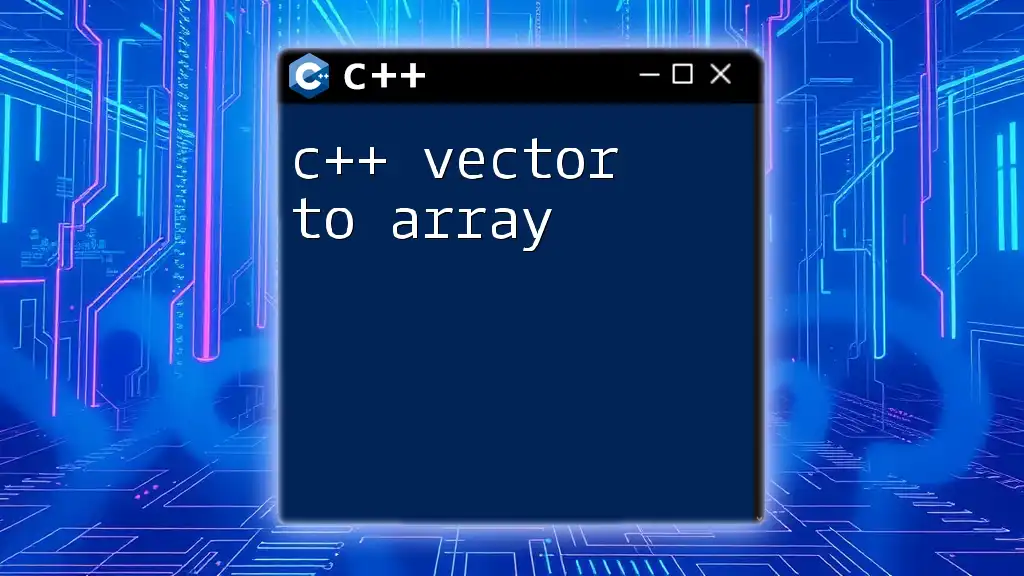
Advanced Usage of For Each with Vectors
Modifying Elements on the Fly
You can also modify elements in a vector during iteration. For instance, if you want to double each value, you can achieve it as follows:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int& num) {
num *= 2; // Modifying the actual element
});
for (const auto& num : numbers) {
std::cout << num << " "; // Prints: 2 4 6 8 10
}
return 0;
}
Combining `for_each` with Other Algorithms
`std::for_each` can be combined with other STL algorithms, such as `std::sort`, to produce powerful, compact code. Here’s an example of sorting a vector and then printing its contents:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 1, 4, 2};
std::sort(numbers.begin(), numbers.end()); // Sort first
std::for_each(numbers.begin(), numbers.end(), [](int num) {
std::cout << num << " "; // Print sorted numbers
});
return 0;
}
Comparing Traditional Loops with For Each
When considering traditional loops versus for each, it’s crucial to weigh the advantages and disadvantages. Traditional loops provide full control over the iteration process, allowing for complex operations requiring indices. However, they can lead to error-prone code if not managed carefully.
On the other hand, for each loops enhance readability and minimize complexity. They conceal the underlying mechanics, making code less verbose and easier to understand at a glance.
Consider the following example comparing both methods:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Traditional Loop
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << numbers[i] << " ";
}
std::cout << "\n"; // New line
// For Each Loop
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
Both code segments yield the same result, but the for each version is noticeably more concise and easier to read.
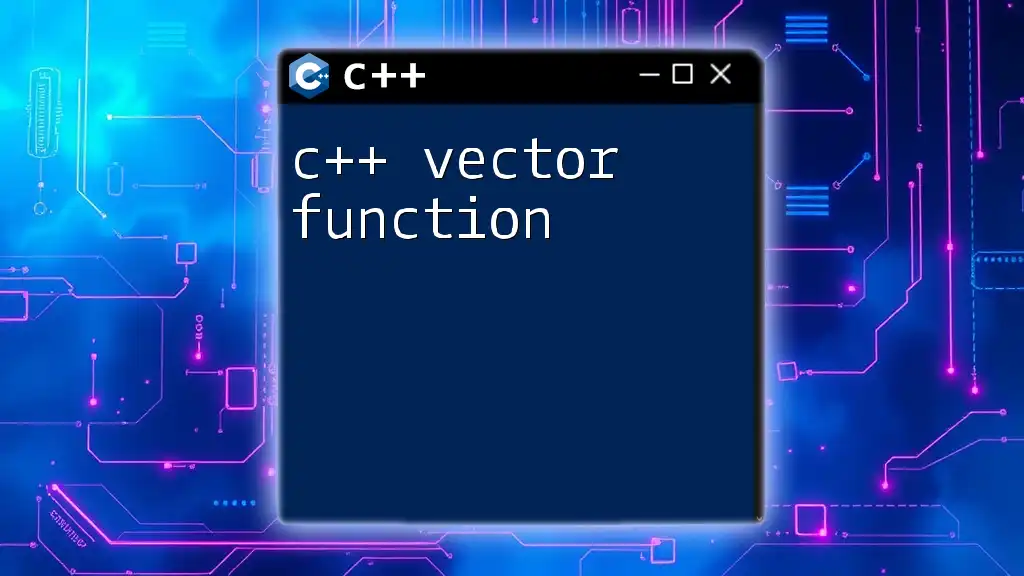
Common Pitfalls and Best Practices
Common Mistakes with For Each
While using the for each loop, programmers might inadvertently try to modify the vector in the context of a loop other than `std::for_each`. This can lead to errors or undefined behavior. It is also critical to ensure that the vector remains unchanged while iterating through it, especially when using const qualifiers.
Best Practices for Using For Each in C++
When using the for each construct in C++, adhere to these best practices:
- Use const references whenever possible to avoid unnecessary copies and maintain performance.
- Keep lambda functions concise. While C++ allows complex lambda expressions, clarity should be prioritized to maintain the code's readability.
- Limit vector modifications during iteration unless using methods designed for that, such as `std::for_each` with mutable references.
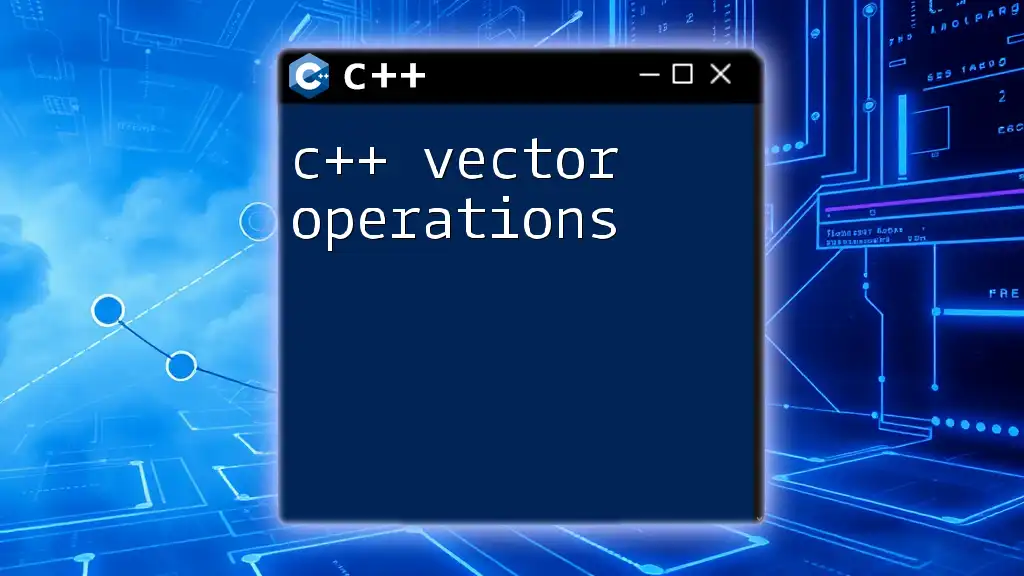
Conclusion
In conclusion, mastering the C++ vector for each technique is an invaluable skill for any C++ programmer. This method enhances code readability, simplifies iteration over data collections, and provides robust features for data manipulation. By practicing these techniques, programmers can improve their efficiency and effectiveness in writing clean, maintainable C++ code.
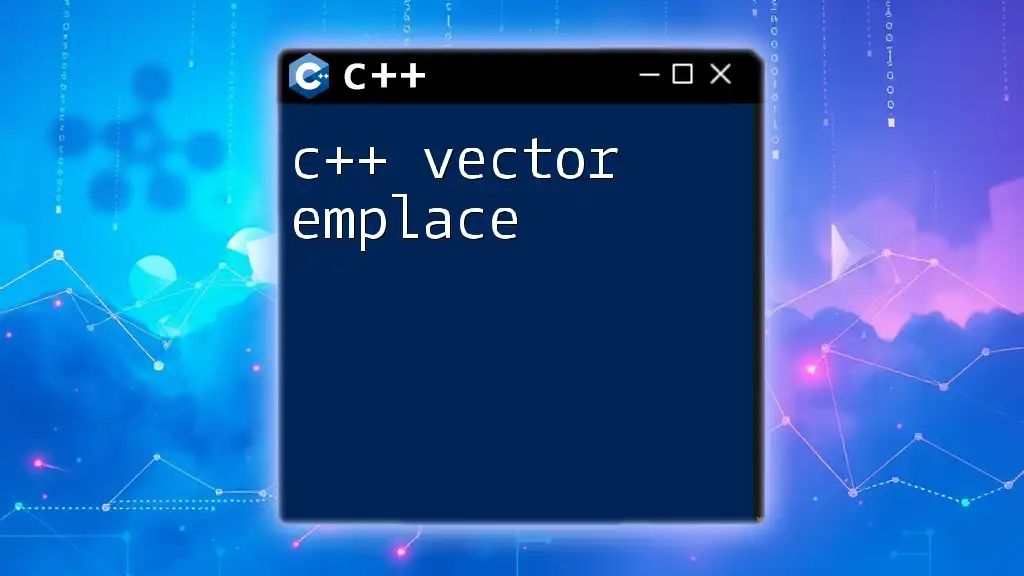
Additional Resources
For further learning on C++ vectors and iteration techniques, consider exploring the following resources:
- Books on C++ STL and vector implementations
- Online courses that focus on advanced C++ programming
- Official C++ documentation that details the Standard Template Library and built-in algorithms
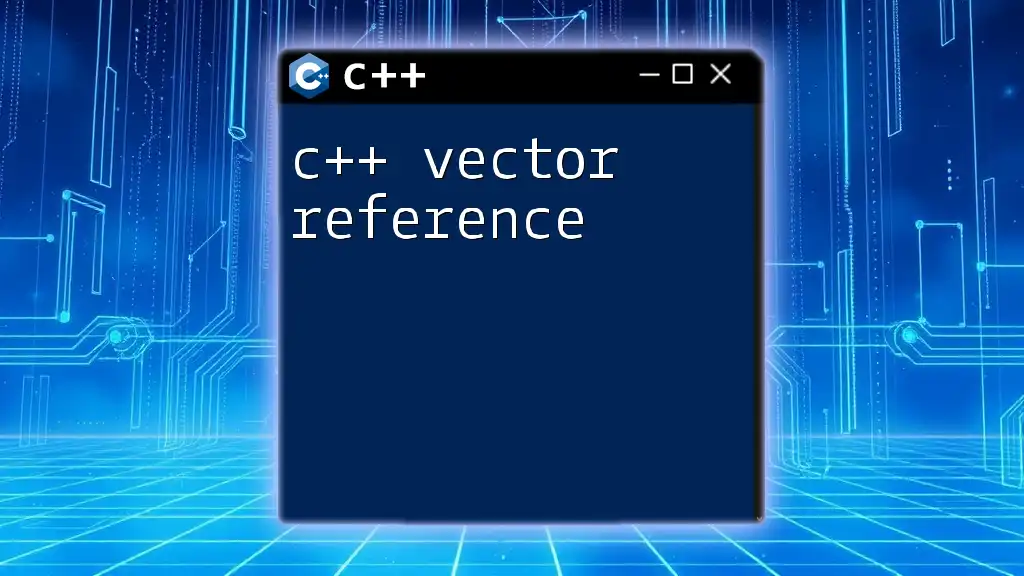
FAQs
What is the difference between a vector and an array in C++? Vectors can dynamically resize and have built-in methods for management, while arrays have a fixed size determined at compile time.
When should I use a range-based for loop instead of `std::for_each`? Use a range-based for loop for simpler iteration tasks where mutation isn't required. Opt for `std::for_each` when applying complex operations or transformations across your vector elements.