In C++, you can convert a `std::vector` to a `std::tuple` by using the `std::make_tuple` function along with unpacking the vector elements.
Here's a code snippet demonstrating this conversion:
#include <iostream>
#include <vector>
#include <tuple>
int main() {
std::vector<int> vec = {1, 2, 3};
auto myTuple = std::make_tuple(vec[0], vec[1], vec[2]);
std::cout << "Tuple contents: ("
<< std::get<0>(myTuple) << ", "
<< std::get<1>(myTuple) << ", "
<< std::get<2>(myTuple) << ")\n";
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can resize itself automatically when elements are added or removed. Vectors are part of the Standard Template Library (STL) and are defined in the `<vector>` header. They provide several advantages over traditional arrays, including flexibility in size, memory management, and built-in functions for common operations.
Basic Vector Operations
Creating Vectors
You can initialize vectors using the following syntax:
#include <vector>
std::vector<int> myVector; // Empty vector
std::vector<int> initializedVector = {1, 2, 3, 4}; // Initialize with values
Adding Elements
The `push_back()` method allows you to add elements to the end of a vector. For example:
myVector.push_back(5); // Adds 5 to the end of myVector
Accessing Elements
You can access elements in a vector using the bracket notation or `.at()`, which performs bounds-checking:
int firstElement = myVector[0]; // Access first element
int secondElement = myVector.at(1); // Access second element with bounds-checking
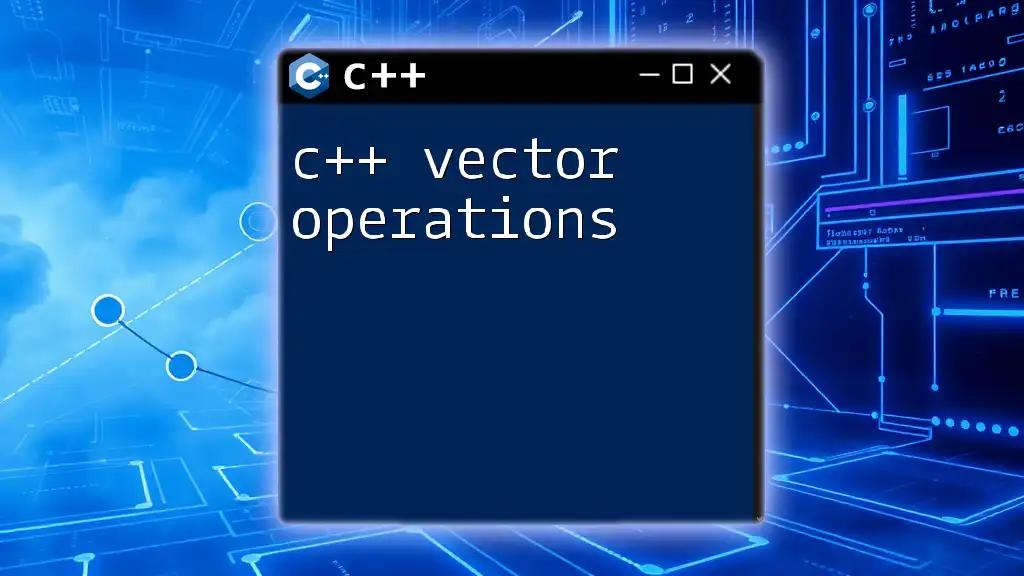
Understanding Tuples in C++
What is a Tuple?
A tuple in C++ is a fixed-size collection that can hold multiple data types. It is defined in the `<tuple>` header and allows you to store heterogeneous data in a single variable. Tuples are particularly useful when you want to return multiple values from a function.
Basic Tuple Operations
Creating Tuples
You can create tuples using `std::make_tuple()`:
#include <tuple>
std::tuple<int, double, const char*> myTuple = std::make_tuple(1, 2.5, "Hello");
Accessing Tuple Elements
To access the individual elements in a tuple, the `std::get<>` function is used. For example:
int a = std::get<0>(myTuple); // Accessing the first element
double b = std::get<1>(myTuple); // Accessing the second element

Why Convert Vector to Tuple?
Use Cases for Conversion
Converting a C++ vector to tuple can be advantageous in circumstances where you want to pass a fixed number of values, particularly when you need to group several values related to a specific data point. Tuples are lightweight and do not require memory overhead compared to vectors which are dynamic and more flexible.
Limitations of Vectors and Tuples
While vectors are excellent for dynamic and high-volume data management, they can introduce complexity when you need a fixed structure. Tuples, on the other hand, allow you to group different data types but lack the features for dynamic resizing. Understanding these limitations can help you decide when to convert a vector into a tuple.
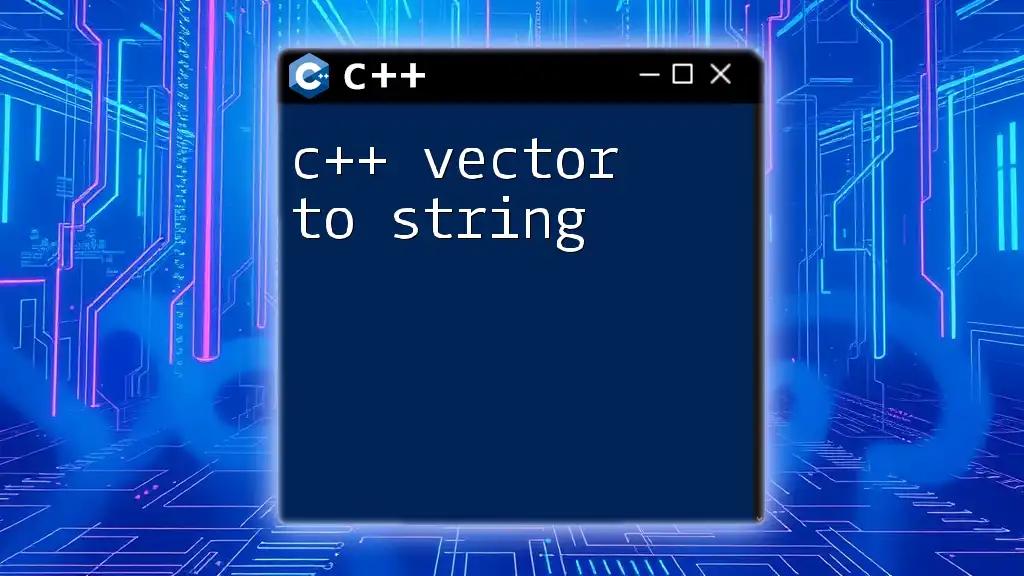
How to Convert a C++ Vector to Tuple
Step-by-Step Guide
To convert a vector to a tuple, follow these steps:
- Ensure your vector has a suitable size: Since tuples are fixed-size, you need to check that your vector contains enough elements.
- Use `std::make_tuple()` to create the tuple: Extract the elements from the vector and pass them to `std::make_tuple()`.
Example Code
Here’s a simple example that demonstrates the conversion process:
#include <iostream>
#include <vector>
#include <tuple>
template<typename T>
std::tuple<T, T> vectorToTuple(const std::vector<T>& vec) {
// Ensure vector has at least two elements
if (vec.size() < 2) throw std::invalid_argument("Vector must have at least two elements");
// Creating a tuple with the first two elements of the vector
return std::make_tuple(vec[0], vec[1]);
}
int main() {
std::vector<int> vec = {1, 2, 3, 4};
auto myTuple = vectorToTuple(vec);
std::cout << "Tuple values: "
<< std::get<0>(myTuple) << ", "
<< std::get<1>(myTuple) << std::endl;
return 0;
}
In this code, the `vectorToTuple` function checks if the vector has at least two elements, then creates a tuple using the first two. This demonstrates a clear and type-safe way to convert a vector into a tuple.
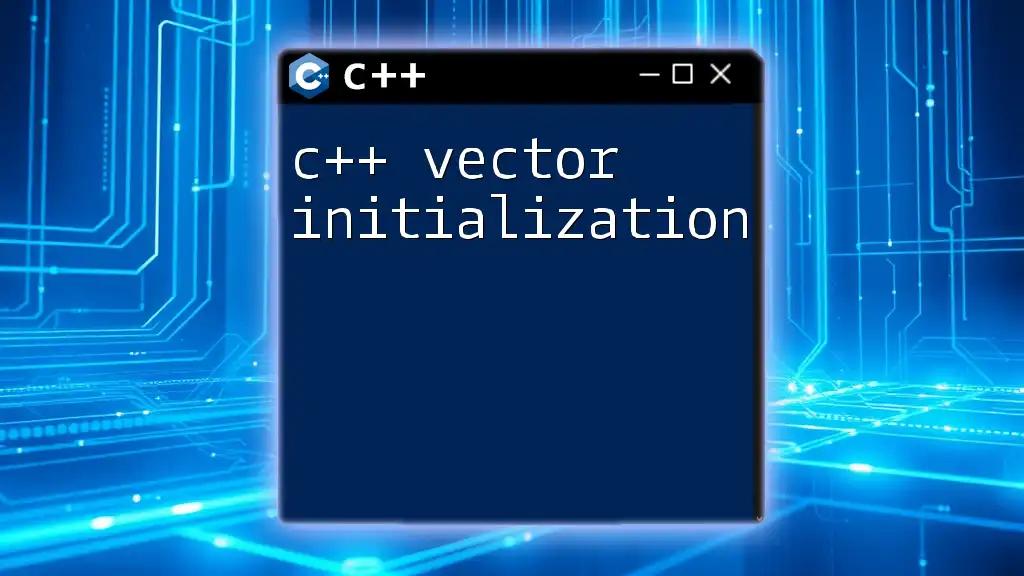
Alternative Methods for Conversion
Using `std::tie`
Another method for converting a vector to a tuple is by using `std::tie`. This is particularly useful when you want to unpack the values directly. Here’s an example:
#include <iostream>
#include <vector>
#include <tuple>
int main() {
std::vector<int> vec = {1, 2, 3};
int a, b;
std::tie(a, b) = std::make_tuple(vec[0], vec[1]);
std::cout << "Values: " << a << ", " << b << std::endl;
return 0;
}
In this example, `std::tie` unpacks values into pre-defined variables, allowing you to manage tuple elements conveniently.
For Loop Method
You can also use a loop to convert vector elements into a tuple when working with larger datasets. This method allows for flexible assignment:
#include <iostream>
#include <vector>
#include <tuple>
template<typename T, std::size_t N>
std::tuple<T, T> vectorToTupleNew(const std::vector<T>& vec) {
if (vec.size() < N) throw std::invalid_argument("Vector does not have enough elements");
std::tuple<T, T> result;
for (size_t i = 0; i < N; ++i) {
result = std::make_tuple(vec[i], vec[i + 1]); // Just taking a pair for simplicity
}
return result;
}
This loop iterates through the vector and creates tuples based on defined logic or sizes.
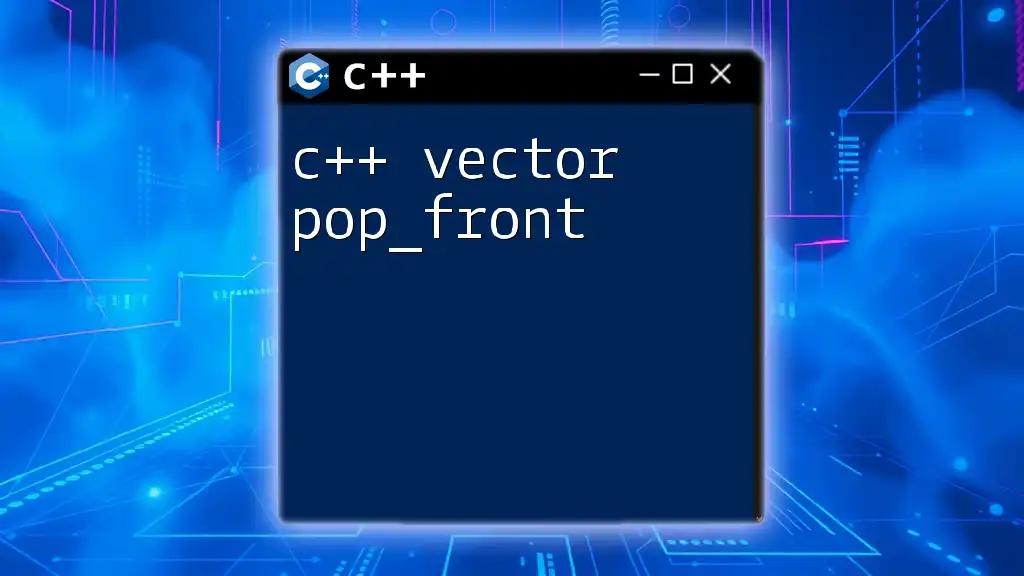
Best Practices
When to Use Which Data Structure
Choosing between vectors and tuples depends on your specific use case. Use vectors when you require dynamic sizing and operations such as appending or deleting elements. On the other hand, use tuples when you need a fixed-size grouping of values, particularly when returning multiple values from functions.
Performance Considerations
When converting between a vector and a tuple, it's crucial to acknowledge potential performance implications. For instance, accessing data in vectors incurs a slight overhead compared to tuples due to dynamic resizing. Always assess your requirements for performance against flexibility. If efficiency is critical, keep your data structure choices aligned with your performance goals.
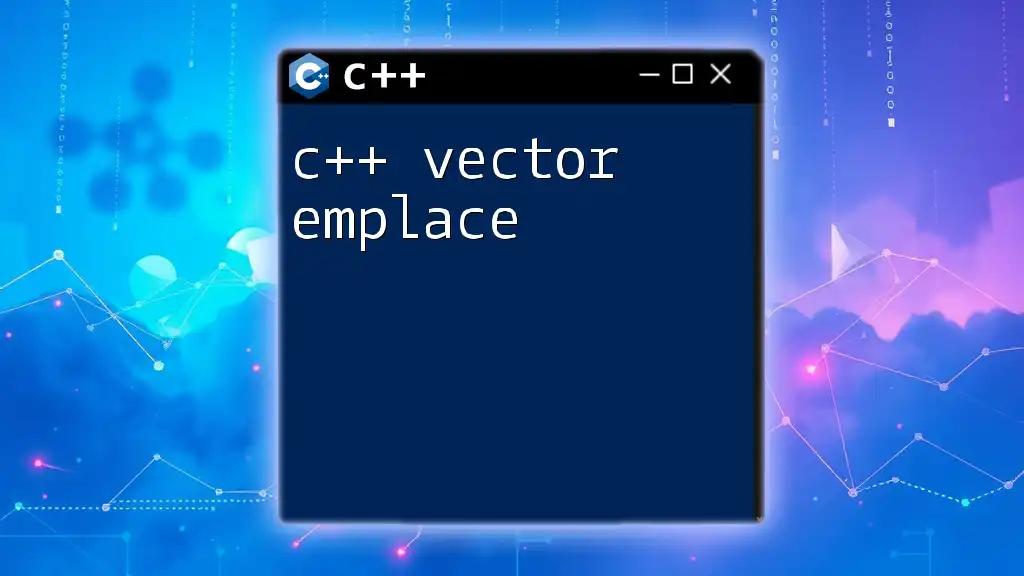
Conclusion
In conclusion, understanding how to convert a C++ vector to tuple expands your ability to manipulate data structures effectively. Knowing when to use each type can enhance your application's performance and readability. As you continue to explore C++, consider experimenting with various data structures to see how they fit your programming needs.

FAQs
Common Questions About C++ Vectors and Tuples
As you delve deeper into C++ programming, you may encounter common questions regarding the conversion process, best practices, and potential pitfalls. Remember to always validate your data before conversions, and continue seeking knowledge through practice and exploration.
Additional Resources
For those interested in further developing their C++ skills, online courses, books, and official documentation can provide additional insight and techniques for mastering C++. Happy coding!