In C++, a vector of pairs allows you to store a dynamic array of pairs, where each pair can hold two related values, typically of different data types.
Here's a code snippet demonstrating how to create and use a vector of pairs:
#include <iostream>
#include <vector>
#include <utility>
int main() {
std::vector<std::pair<int, std::string>> vec;
vec.push_back(std::make_pair(1, "one"));
vec.push_back(std::make_pair(2, "two"));
for (const auto &p : vec) {
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
Understanding the Basics of Pair in C++
What is a Pair in C++?
A pair is a simple data structure provided in the C++ Standard Library through the `<utility>` header. It consists of two values (or elements) stored as a single unit. Each of these elements can be of different data types, enabling you to group two related values without creating a more complex structure.
Code Example
#include <utility>
std::pair<int, std::string> myPair(1, "apple");
In this example, we create a `pair` that holds an integer and a string. The first element can be accessed using `.first`, and the second one with `.second`. Thus, `myPair.first` returns `1`, and `myPair.second` returns `"apple"`.
When to Use Pairs
Pairs are particularly useful when you want to return two related values from a function, or when you're iterating over key-value pairs. Their simplicity and ease of use make them a compelling choice for straightforward data collection in many programs.
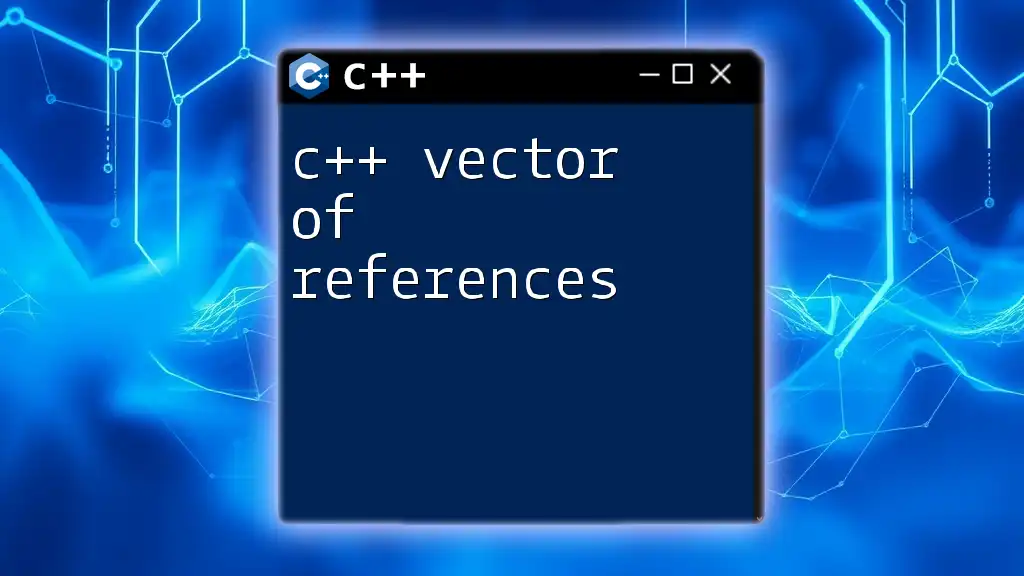
Exploring Vector in C++
What is a Vector in C++?
A vector is a dynamic array that can grow and shrink in size, making it one of the most versatile data structures in C++. Provided by the `<vector>` header, vectors manage memory automatically and allow easy access to a sequence of elements.
Benefits of Using Vectors
Using vectors comes with several notable advantages, such as:
- Dynamic sizing: Unlike traditional arrays, vectors can automatically handle resizing, which alleviates many common memory management issues.
- Ease of use: The C++ Standard Library offers a plethora of functions to manipulate vectors conveniently, such as sorting, searching, and iterating through elements.
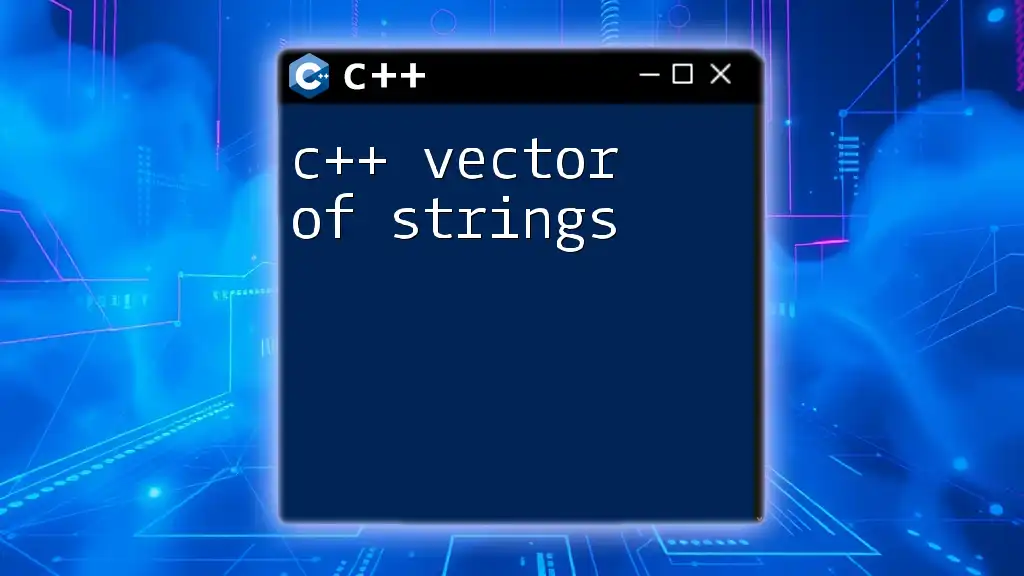
C++ Vector of Pairs
Defining a Vector of Pairs
A C++ vector of pairs combines the flexibility of vectors with the structure of pairs. This allows you to store multiple related pairs of data efficiently.
Code Example
#include <vector>
#include <utility>
std::vector<std::pair<int, std::string>> vecOfPairs;
In this declaration, `vecOfPairs` is a vector that can hold pairs of integers and strings.
Initializing a Vector of Pairs
Vectors of pairs can be initialized in various ways:
-
Method 1: Using `push_back()` You can add pairs to the vector dynamically using the `push_back()` method. Code Example
vecOfPairs.push_back(std::make_pair(1, "Apple")); vecOfPairs.push_back(std::make_pair(2, "Banana"));
-
Method 2: Using an initializer list You can also initialize a vector of pairs at the time of declaration. Code Example
std::vector<std::pair<int, std::string>> vecOfPairs = { {1, "Apple"}, {2, "Banana"} };
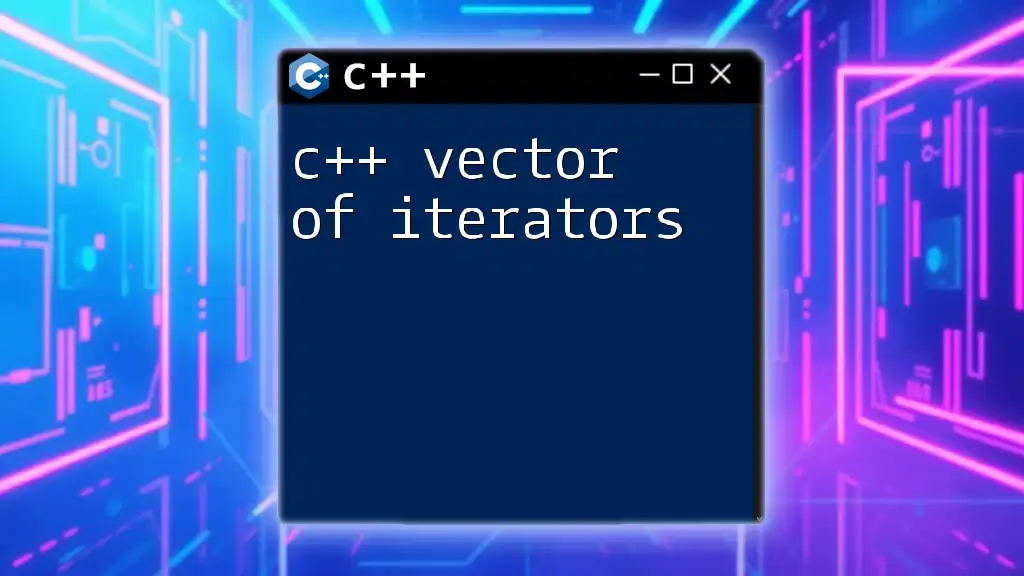
Accessing Elements in a Vector of Pairs
Accessing Individual Elements
To access the elements in a vector of pairs, you can use the index operator. This allows you to retrieve pairs just like you would with any other vector.
Code Example
std::cout << vecOfPairs[0].first << " - " << vecOfPairs[0].second; // Output: 1 - Apple
In this line, we access the first pair in the vector and print its elements.
Iterating through a Vector of Pairs
To perform operations on each element in a vector of pairs, you can iterate through it using loops.
- Using a `for` loop: Code Example
for (size_t i = 0; i < vecOfPairs.size(); ++i) {
std::cout << vecOfPairs[i].first << " - " << vecOfPairs[i].second << std::endl;
}
- Using a range-based `for` loop: Code Example
for (const auto& p : vecOfPairs) {
std::cout << p.first << " - " << p.second << std::endl;
}
Both methods will traverse the vector and print each pair's elements conveniently.
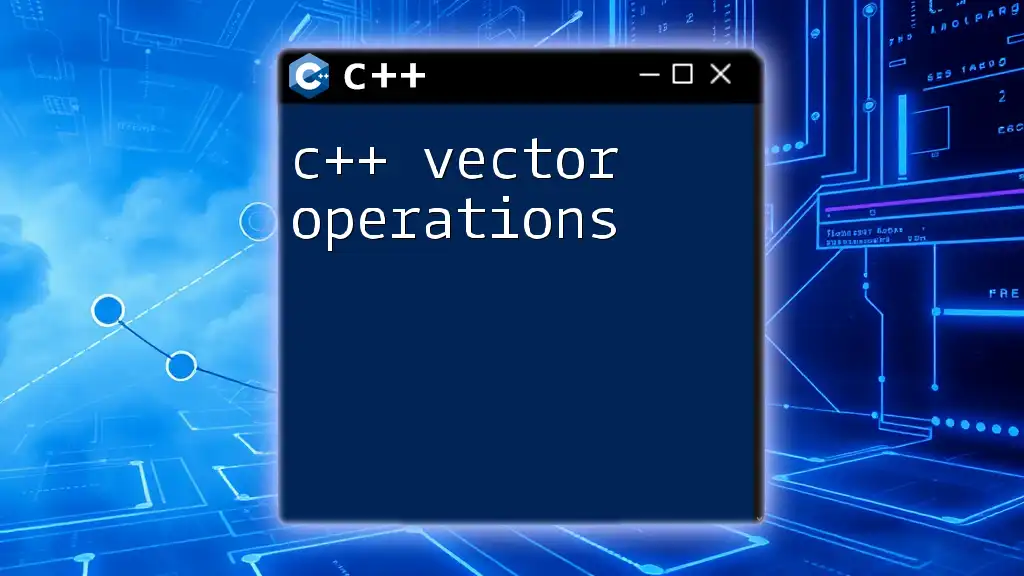
Common Operations with Vector of Pairs
Sorting a Vector of Pairs
You can easily sort a vector of pairs based on either the first or second element.
To sort by the first element, you can simply call `std::sort`.
Code Example
#include <algorithm> // include for std::sort
std::sort(vecOfPairs.begin(), vecOfPairs.end());
If you want to sort by the second element, you may need to define a custom comparator.
Code Example
std::sort(vecOfPairs.begin(), vecOfPairs.end(),
[](const std::pair<int, std::string>& a, const std::pair<int, std::string>& b) {
return a.second < b.second; // Sort by second element
});
Finding Elements in a Vector of Pairs
To find an element in a vector of pairs, you can use the `std::find_if` function, which enables custom conditions.
Code Example
auto it = std::find_if(vecOfPairs.begin(), vecOfPairs.end(), [](const std::pair<int, std::string>& p) {
return p.second == "Banana";
});
In this example, we search for the pair where the second element equals "Banana".
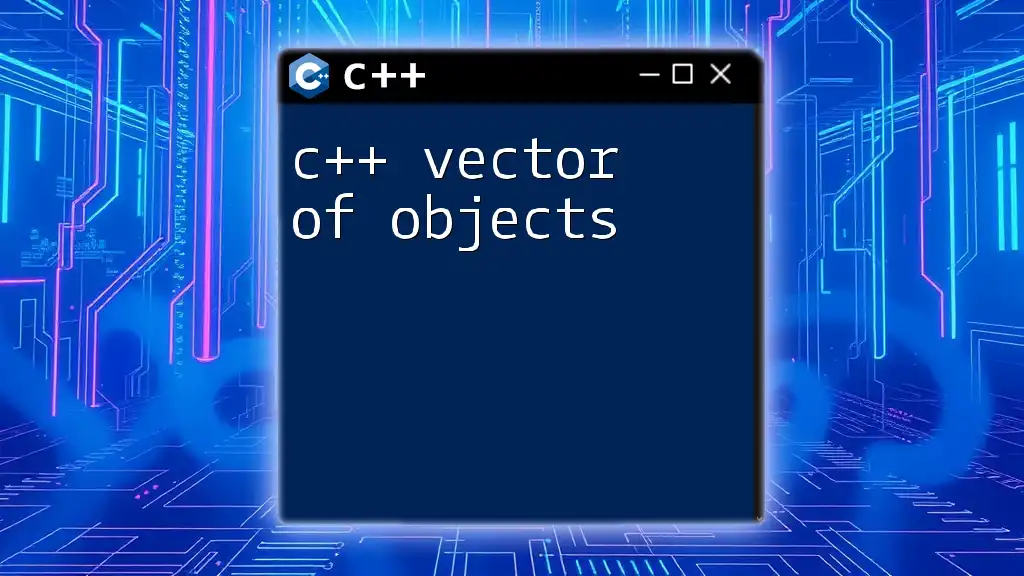
Practical Applications of Vector of Pairs
Use Case 1: Storing Key-Value Pairs
A C++ vector of pairs can act as a straightforward substitute for associative containers like maps. This can be particularly beneficial when the data is relatively small in size or you want to maintain order.
For instance, consider a simple phonebook with names and associated phone numbers stored as pairs.
Use Case 2: Managing Data Points
Vectors of pairs can also represent data points in various applications, such as graphing or mapping.
For example, in a 2D space, you can represent coordinates as pairs within a vector, making it very handy in graphical programs or data analysis scenarios.
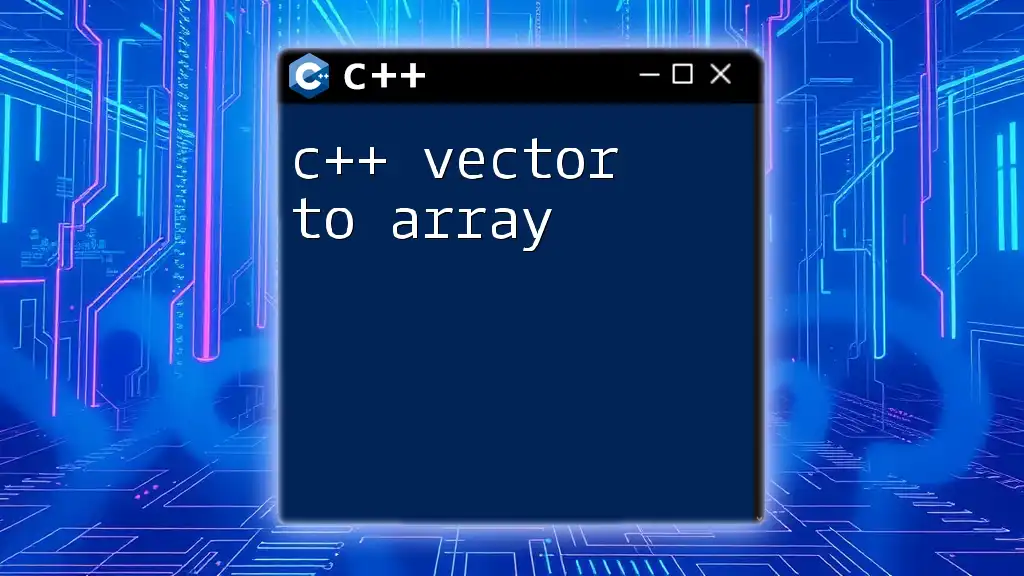
Conclusion
In summary, a C++ vector of pairs offers a powerful combination of flexibility and structure, allowing programmers to manage related data efficiently. The simplicity and utility of pairs make them an excellent choice for various programming tasks. Whether you are developing applications that require grouping of related values or dealing with key-value pairs, mastering the use of vectors of pairs will enhance your coding toolkit. So, dive in and practice with these concepts to fully harness their capabilities in your C++ programming journey.
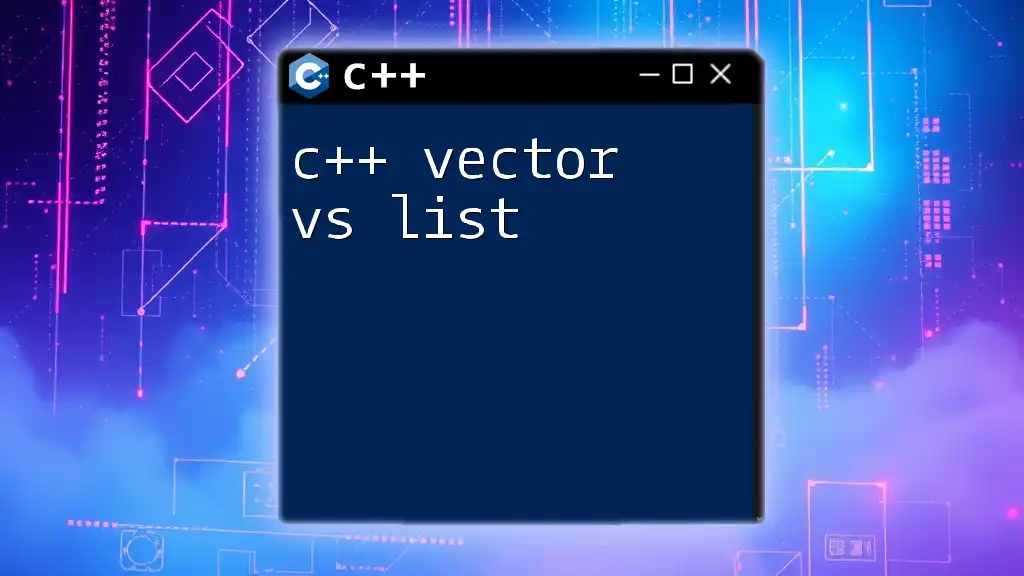
Additional Resources
To deepen your understanding of C++ vectors and pairs, consider exploring further through recommended books, online tutorials, and the official C++ documentation. Happy coding!