C++ vectors can be initialized using an initializer list or the constructor, allowing for dynamic arrays that can resize themselves as needed.
Here’s a code snippet showcasing different ways to initialize a vector:
#include <vector>
#include <iostream>
int main() {
// Initialize a vector with five zeros
std::vector<int> vec1(5);
// Initialize a vector with specific values
std::vector<int> vec2 = {1, 2, 3, 4, 5};
// Initialize a vector from an existing array
int arr[] = {6, 7, 8};
std::vector<int> vec3(arr, arr + sizeof(arr) / sizeof(arr[0]));
// Print the vectors
for (const auto& num : vec1) std::cout << num << " ";
std::cout << std::endl;
for (const auto& num : vec2) std::cout << num << " ";
std::cout << std::endl;
for (const auto& num : vec3) std::cout << num << " ";
return 0;
}
Understanding Vectors
What is a Vector?
A vector in C++ can be thought of as a flexible array that can grow or shrink in size. Unlike traditional arrays, which have a fixed size that must be specified at compile time, vectors can dynamically resize themselves during runtime. This feature makes vectors a preferred choice for handling collections of data where the number of elements may change over time.
Key Features of Vectors
- Dynamic Size: Vectors can easily adjust their size as elements are added or removed, facilitating the management of collections of varying length.
- Automatic Memory Management: C++ handles the memory allocation and deallocation for vectors, minimizing the likelihood of memory leaks or access violations.
- Flexibility with Types: Vectors can store any data type, including user-defined types, allowing for a wide range of use cases.

Basic Initialization of Vectors
Default Initialization
Default initialization creates a vector with no elements. This is beneficial when you plan to add elements to the vector later.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec; // Default initialization
std::cout << "Size: " << vec.size() << std::endl; // Outputs: Size: 0
return 0;
}
In this example, a vector named `vec` is created without specifying any size or initial values, resulting in a vector of size 0.
Initialization with Size
When you want to initialize a vector with a specific number of elements, you can specify the desired size during initialization.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec(5); // Creates a vector of size 5
std::cout << "Size: " << vec.size() << std::endl; // Outputs: Size: 5
return 0;
}
Here, `vec` is initialized to hold five integer elements, all of which will have indeterminate values until explicitly set.
Initialization with Size and Default Value
In situations where you need a vector initialized to a specific size but want all elements to start with a predefined value, you can provide a default value during initialization.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec(5, 10); // Creates a vector of size 5, initialized to 10
std::cout << "First Element: " << vec[0] << std::endl; // Outputs: First Element: 10
return 0;
}
In this scenario, the vector `vec` is initialized with five elements, all set to 10, simplifying initialization when you require uniform initial values.

Advanced Initialization Techniques
Initializing with an Existing Array
If you already have a statically allocated array and wish to convert it into a vector, you can use the beginning and end of the array as arguments to the vector constructor.
#include <iostream>
#include <vector>
int main() {
int arr[] = {1, 2, 3, 4, 5};
std::vector<int> vec(std::begin(arr), std::end(arr)); // Initialize vector from array
for (int elem : vec) {
std::cout << elem << " "; // Outputs: 1 2 3 4 5
}
return 0;
}
The above code snippet shows how to initialize a vector `vec` using the elements from the array `arr`. This method is concise and leverages the power of C++ Standard Library functions.
Using the Initializer List
C++11 introduced the initializer list syntax, which provides a clean and straightforward way to initialize vectors.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5}; // Using initializer list
for (int elem : vec) {
std::cout << elem << " "; // Outputs: 1 2 3 4 5
}
return 0;
}
This technique allows for a simple and readable way to create and initialize a vector in a single statement, improving code clarity.
Copying Another Vector
C++ classes often include a copy constructor to facilitate the creation of new objects based on existing ones. Vectors are no different.
#include <iostream>
#include <vector>
int main() {
std::vector<int> original = {1, 2, 3};
std::vector<int> copy = original; // Copying original vector
for (int elem : copy) {
std::cout << elem << " "; // Outputs: 1 2 3
}
return 0;
}
In this example, the vector `copy` is initialized as a copy of `original`, enabling developers to duplicate vector contents with ease.
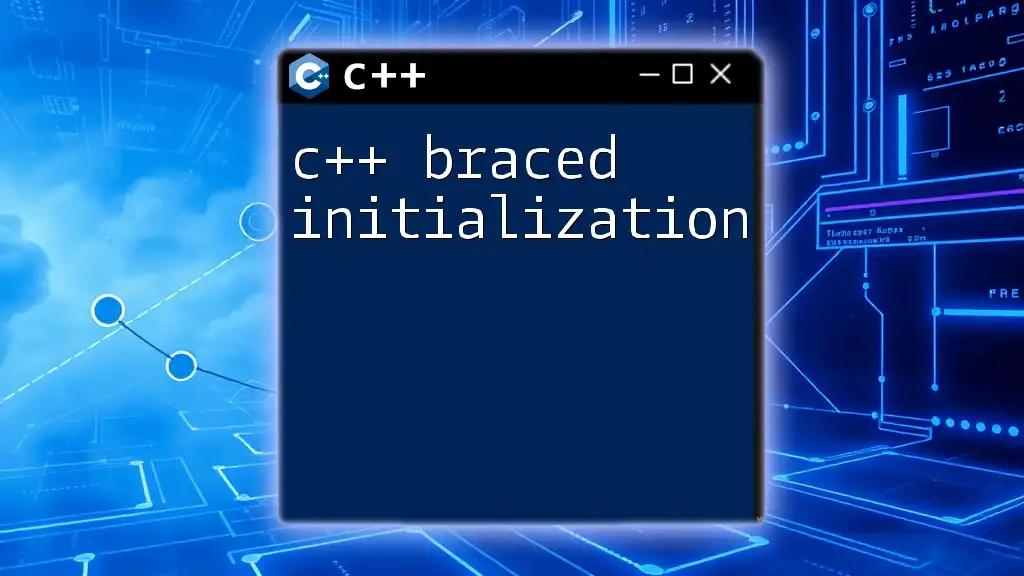
Performance Considerations
Memory Allocation
Vectors utilize dynamic memory allocation, which means they can grow and shrink as necessary. However, resizing a vector requires the allocation of new memory, copying existing elements, and deallocating the old memory, which can have a negative impact on performance. Knowing how to use the capacity and reserve functions correctly can help manage this overhead.
Initialization and Performance
Choosing the right initialization technique can impact performance, especially with large datasets. For substantial collections, methods such as reserve() can allocate enough memory in advance to prevent multiple reallocations.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.reserve(100); // Reserve space for 100 elements
// Now you can efficiently add elements without frequent reallocations
return 0;
}
This ensures optimal performance as the vector grows, primarily reducing resizing costs during element insertion.
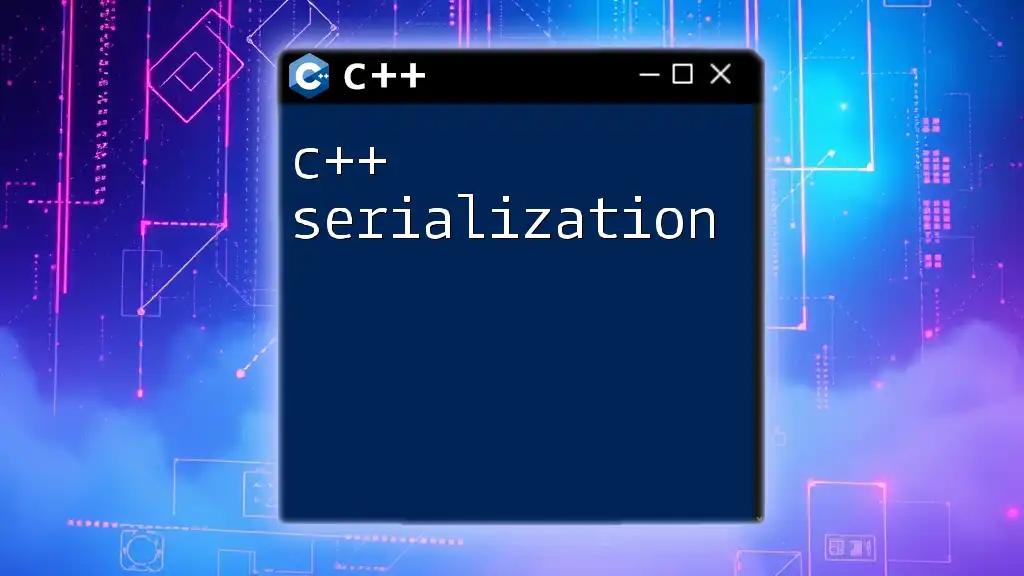
Common Mistakes and Best Practices
Forgetting to Include Necessary Headers
Always remember to include the appropriate headers. For vector usage, the necessary inclusion is:
#include <vector>
This is crucial for your program to compile successfully.
Incorrectly Accessing Vector Elements
When working with vectors, be cautious of out-of-bounds access. Using an index that exceeds the current size of the vector can lead to undefined behavior. Therefore, it's a good practice to always ensure that a vector is not empty before accessing its elements.
Recommended Practices
- Maintain consistency in your vector initialization style to enhance readability.
- Use reserve() to preallocate space for larger vectors to improve performance during insertion.

Conclusion
In conclusion, mastering C++ vector initialization is key to harnessing the full power of vectors in your C++ programming journey. Various initialization techniques, from basic to advanced, serve different use cases. Understanding these methods allows you to create efficient, dynamic, and robust applications. As you continue to explore C++, experimenting with vector initialization techniques will deepen your understanding and enhance your coding proficiency.
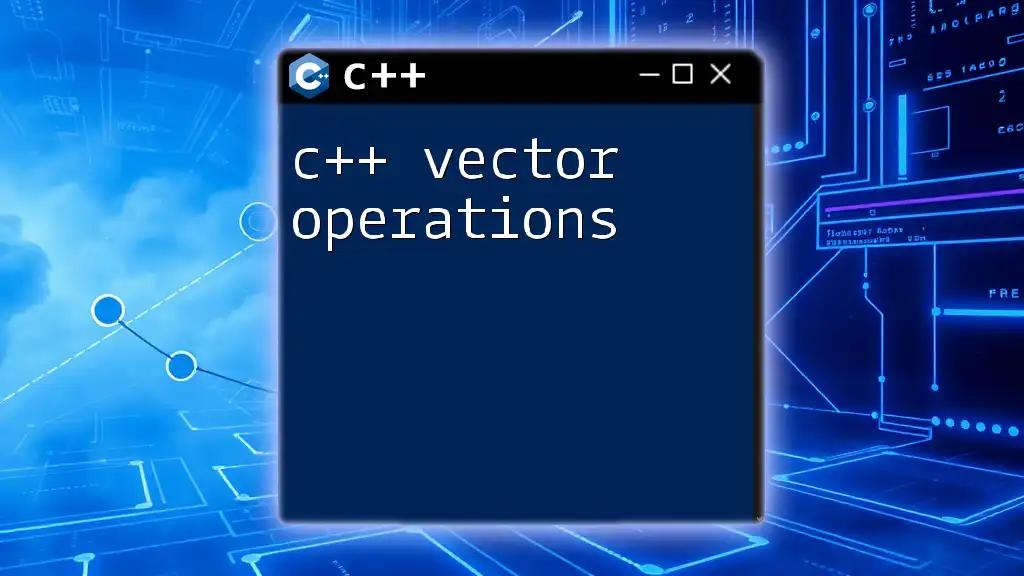
Resources for Further Learning
For further exploration, consider diving into recommended books, online courses, and official C++ documentation to expand your knowledge on C++ vector initialization and related topics. By deepening your understanding, you will become a more proficient C++ programmer, capable of tackling diverse challenges with confidence and skill.