In C++, concatenation refers to the process of joining two or more strings together using the `+` operator.
Here’s a simple code snippet demonstrating string concatenation:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2;
std::cout << result << std::endl; // Output: Hello, World!
return 0;
}
Understanding C++ Concatenation
What is Concatenation?
C++ concatenation refers to the operation of joining two or more strings together to form a single string. This fundamental operation is essential in programming, enhancing the way we interact with users and create dynamic content. Concatenation allows developers to build text outputs, messages, and formatted strings efficiently.
Why Use Concatenation in C++?
Using concatenation in C++ plays a vital role in improving readability and facilitating user interaction. When you need to display personalized messages, construct data outputs, or build dynamic strings, concatenation is the key. Common use cases for string manipulation include:
- Crafting user greetings and personalized messages.
- Formatting output results dynamically based on user inputs.
- Constructing file paths and URLs.
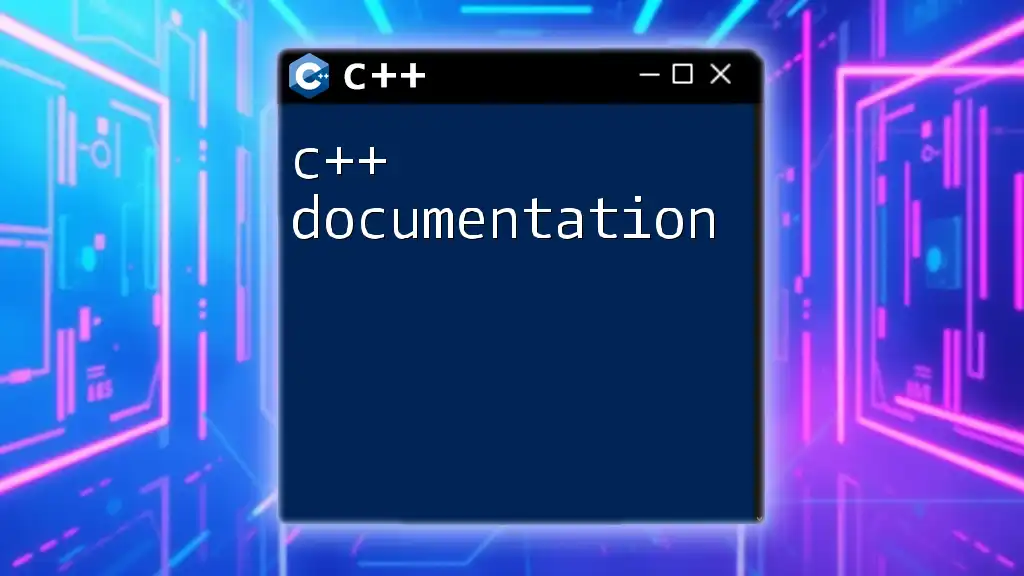
Basic Concepts of String Handling in C++
Overview of C++ Strings
In C++, there are two main types of strings: C-style strings and C++ `std::string`. C-style strings are arrays of characters that terminate with a null character (`'\0'`). However, for simplicity and robust functionality, we primarily rely on `std::string`, which is part of the C++ Standard Library and provides numerous features for string manipulation. Don’t forget to include the header file:
#include <string>
Creating Strings
Creating strings in C++ is straightforward. You can declare and initialize strings as follows:
std::string greeting = "Hello, ";
std::string name = "World!";
Here, `greeting` and `name` are both initialized with literal string values.
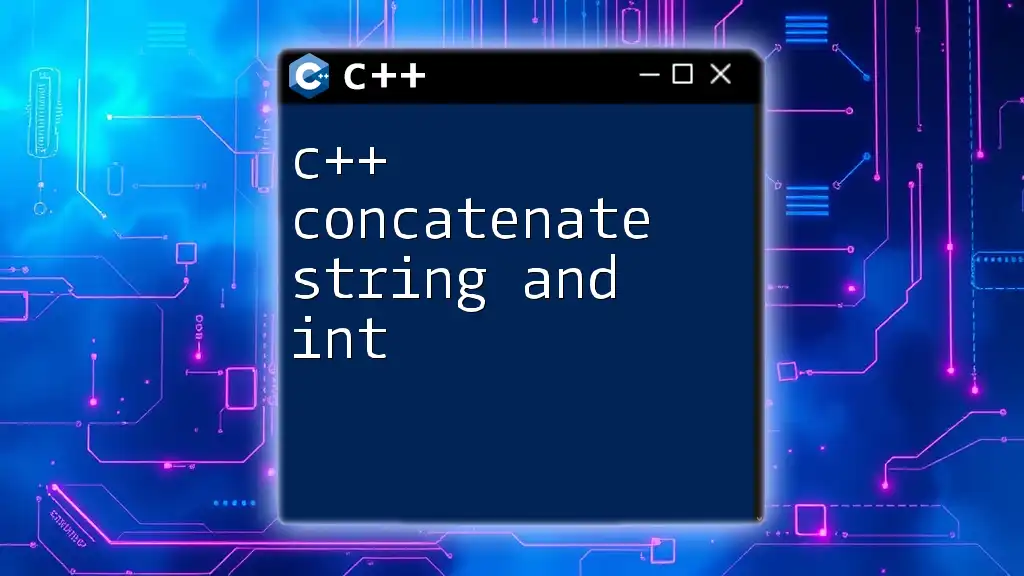
Concatenation in C++
Using the `+` Operator
The most intuitive way to concatenate strings in C++ is by using the `+` operator. This operator allows you to join two strings together easily:
std::string fullGreeting = greeting + name; // Results in "Hello, World!"
The `+` operator creates a new string that contains the contents of both `greeting` and `name`, effectively combining them into a single string.
Using the `+=` Operator
The `+=` operator is another convenient way to concatenate strings. It appends the right-hand operand to the left-hand string, allowing you to add to an existing string:
greeting += name; // greeting now contains "Hello, World!"
This operator modifies the original string and provides an efficient way to build strings incrementally.
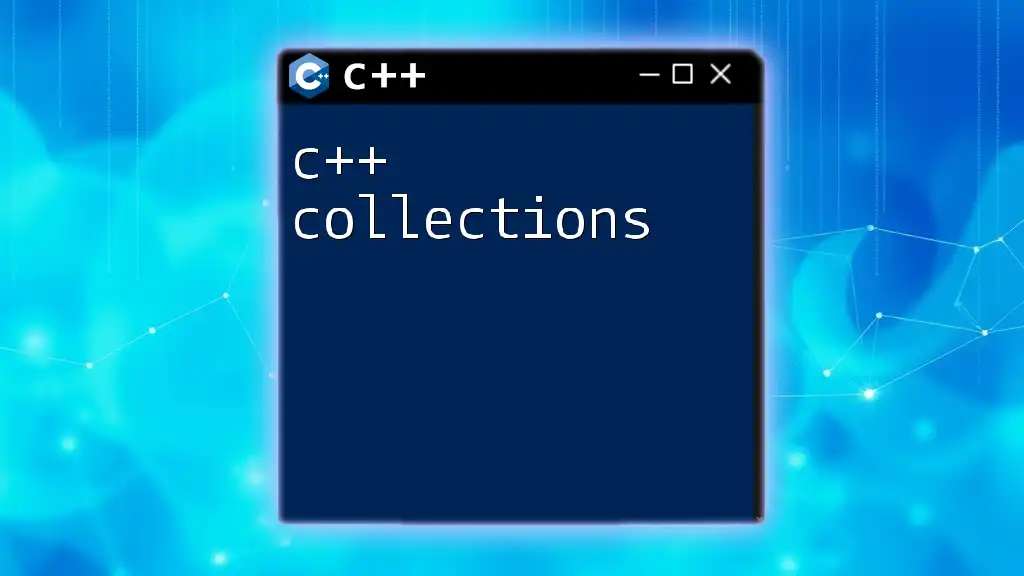
Advanced String Concatenation Techniques
Using `std::string::append()`
For even more flexibility, C++ provides the `std::string::append()` method. This method allows you to append additional characters or another string to an existing string:
std::string farewell = "Goodbye, ";
farewell.append("everyone!"); // farewell now contains "Goodbye, everyone!"
Using `append()` can sometimes be clearer in intent, especially when manipulating multiple segments of strings.
Concatenating Multiple Strings
When you need to join several strings together, looping through collections can be efficient. Here’s an example using a loop:
std::string sentence;
for (const auto& word : {"C++", "is", "fun!"}) {
sentence += word + " ";
}
This approach demonstrates that you can build strings dynamically through iteration, allowing for more complex outputs.
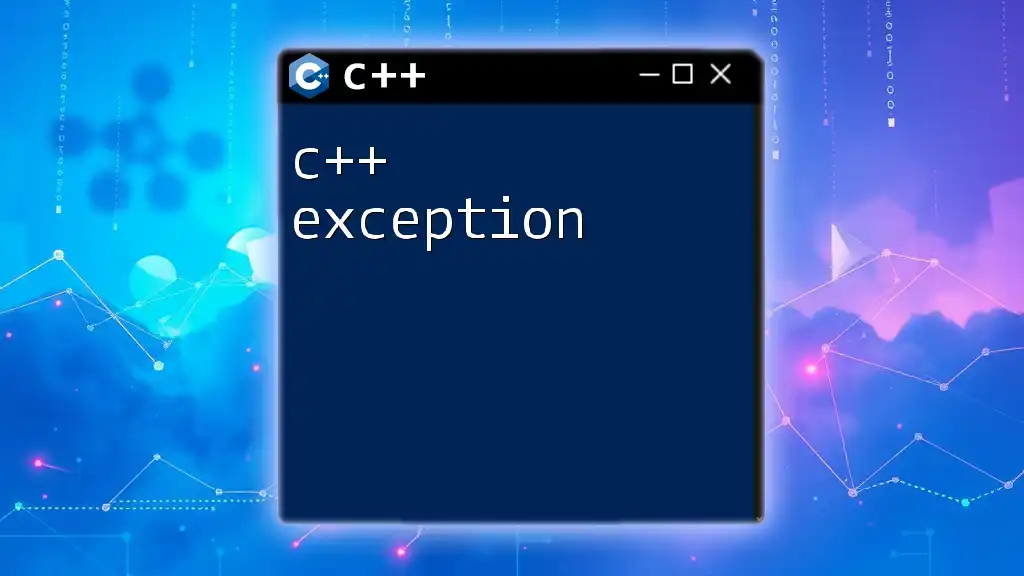
Best Practices for String Concatenation
Performance Considerations
Concatenation can have performance implications. The `+` operator creates a new string every time it is used, leading to potential inefficiencies in scenarios where many concatenations occur. To mitigate this, consider using the `std::string::reserve()` method before concatenating if you know the final size of your string. This way, you can reduce the frequency of memory allocations:
std::string message;
message.reserve(100); // Reserve space for 100 characters
Avoiding Common Pitfalls
Be cautious when mixing C-style strings with C++ strings. Mixing both types without proper conversion can lead to undefined behavior. Stick to `std::string` for a more robust string management solution in C++.
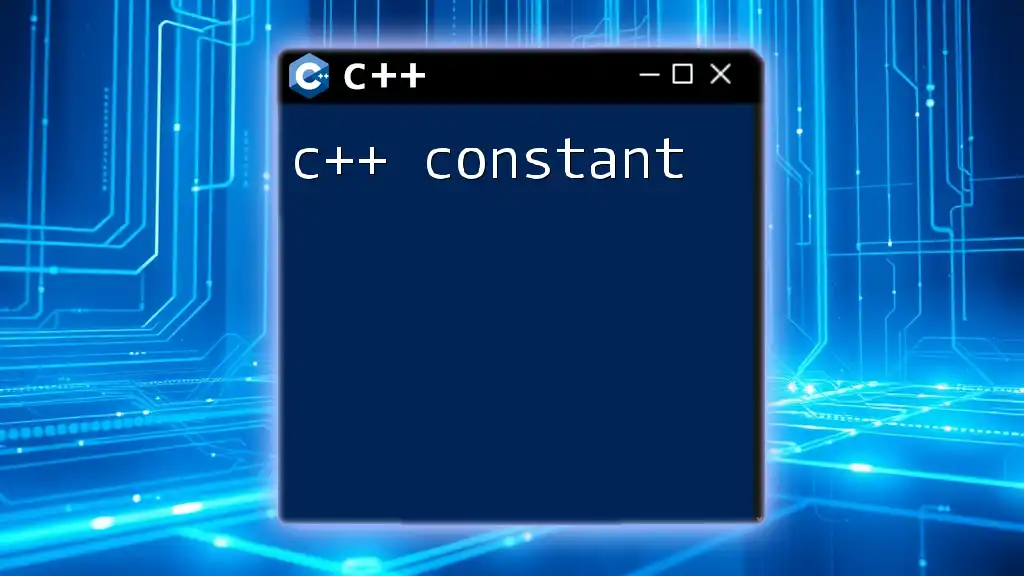
Real-World Applications of Concatenation in C++
User Input and Response Generation
Concatenation shines in user interaction scenarios, where building personalized messages is often required. For instance, you can create a custom greeting based on user input:
std::string userName;
std::cout << "Enter your name: ";
std::cin >> userName;
std::string customGreeting = "Welcome, " + userName + "!";
This small snippet demonstrates how effortlessly you can create a dynamic message tailored to the user.
Formatting Strings for Output
String concatenation is also useful for formatting output in applications, such as a simple calculator. Here’s how you can generate a formatted result using string concatenation:
int a = 5, b = 10;
std::cout << "The sum of " + std::to_string(a) + " and " + std::to_string(b) + " is " + std::to_string(a + b);
This example converts integers to strings before concatenation, allowing you to build a formatted output string seamlessly.
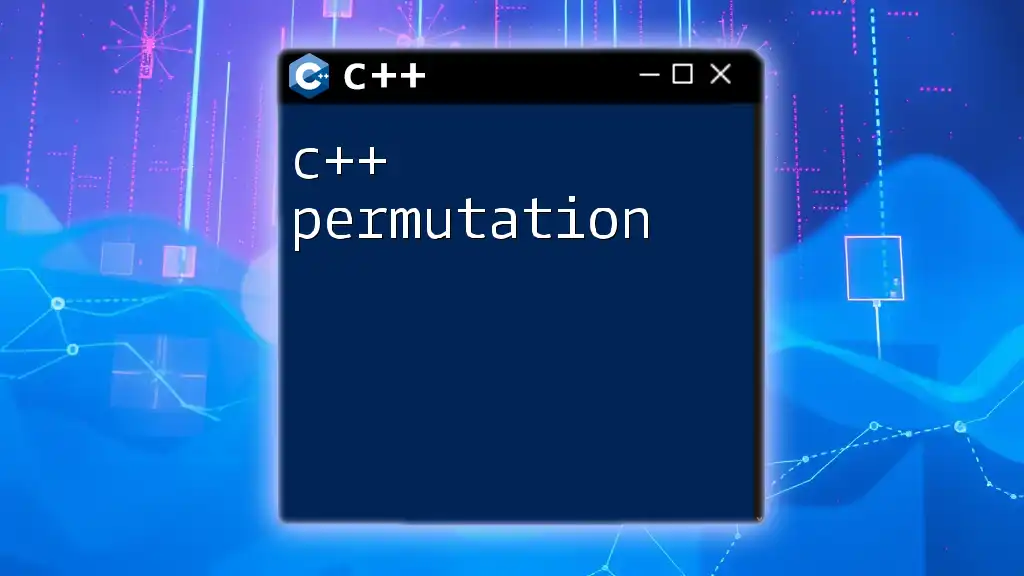
Conclusion
In summary, C++ concatenation is a powerful tool for string manipulation, making it a fundamental skill for any programmer. From basic concatenation using operators to more advanced techniques like `append()`, understanding and applying these methods will enhance your programming capabilities.
I encourage you to experiment with these string concatenation methods in your projects and challenge yourself with exercises that require string manipulation. The more you practice, the more proficient you’ll become in using C++ concatenation effectively.
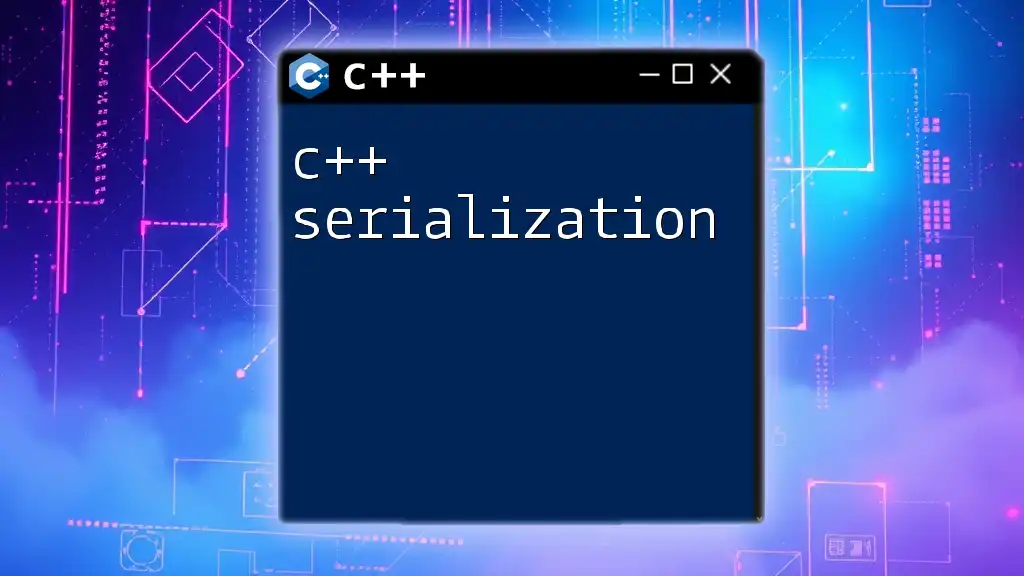
Additional Resources
For further reading, explore the C++ Standard Library documentation on strings and additional tutorials focused on string manipulation. Familiarize yourself with tools like online compilers and IDEs where you can practice your C++ skills interactively.