In C++, an iterator is an object that allows you to traverse through the elements of a container, such as an array or a vector, in a sequential manner.
Here's a simple example of using an iterator with a `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (std::vector<int>::iterator it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
What is an Iterator?
A C++ iterator is a specialized object that enables traversal of elements in data structures such as arrays, lists, and vectors without exposing their internal structure. Iterators provide a consistent interface for navigating through various containers, essentially acting as pointers that point to the next element in a sequence.

Importance of Iterators
Using iterators has several advantages over traditional indexing when accessing elements in a container:
- Abstraction: Iterators abstract the process of element access, allowing you to treat different containers uniformly.
- Flexibility: They can traverse not only containers like arrays and vectors but also more complex structures like trees and graphs.
- Consistency: Iterators provide a way to perform operations on each element without the need for specific container knowledge, promoting code reusability and maintenance.
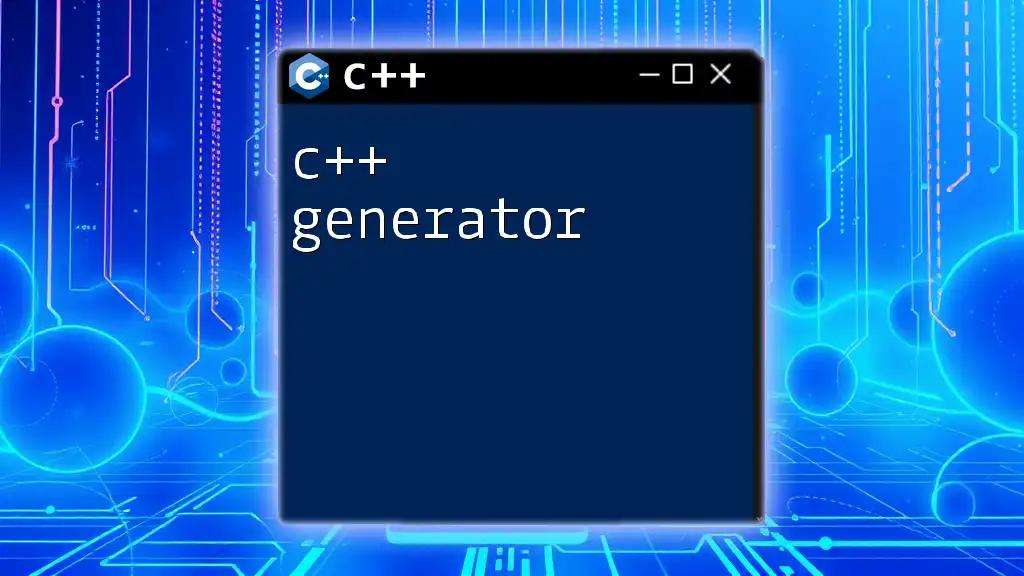
Types of Iterators in C++
Input Iterators
Input iterators allow read-only access to a sequence. They can be incremented to point to the next element but cannot be decremented or modified.
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int>::iterator it;
for (it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
In this example, iterators are used to loop through each element in a vector, demonstrating how simple it is to access container elements using iterators. Use input iterators in scenarios where you only need to read data from a collection.
Output Iterators
Output iterators are designed for write-only access, allowing you to write data to a sequence but not to read it.
Example Code Snippet:
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::ostream_iterator<int> output(std::cout, " ");
std::copy(vec.begin(), vec.end(), output);
return 0;
}
Here, output iterators send the elements of a vector to standard output using `std::copy`. Output iterators are essential in cases where data needs to be written to an external output like a file or console.
Forward Iterators
Forward iterators enhance input iterators, allowing both reading and writing access while permitting increment operations only in the forward direction.
Example Code Snippet:
#include <iostream>
#include <list>
int main() {
std::list<int> my_list = {1, 2, 3};
auto it = my_list.begin();
while (it != my_list.end()) {
std::cout << *it << " ";
++it;
}
return 0;
}
Forward iterators are particularly useful in scenarios where you want to traverse through a container once while allowing modifications, emphasizing their capability to easily access and manipulate elements.
Bidirectional Iterators
Bidirectional iterators extend the functionality of forward iterators by allowing movement in both directions—forward and backward.
Example Code Snippet:
#include <iostream>
#include <set>
int main() {
std::set<int> myset = {1, 2, 3, 4, 5};
std::set<int>::iterator it = myset.end();
while (it != myset.begin()) {
--it;
std::cout << *it << " ";
}
return 0;
}
This example demonstrates iterating backward through a set. Bidirectional iterators are well-suited for data structures where reverse traversal is needed, offering more flexibility in data handling.
Random Access Iterators
Random access iterators are the most powerful, allowing traversal with constant-time access to any element while supporting increment, decrement, and arithmetic operations.
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
for (size_t i = 0; i < vec.size(); ++i) {
std::cout << vec[i] << " ";
}
return 0;
}
Random access iterators, as shown, allow not only looping through a container but also jumping to any specific index. They are particularly advantageous when you need to perform operations that require frequent access to non-consecutive elements or when executing algorithms like sorting.

Using Iterators Effectively
Common Operations
Increment and Decrement
Iterators support increment (`++it`) and decrement (`--it`) operations to navigate through sequences. The ability to increment and decrement is crucial for implementing loops and algorithms efficiently.
Dereferencing
Dereferencing an iterator using the `*` operator provides access to the element it points to. For example, `*it` retrieves the value at the current iterator position, allowing for both read and write operations depending on the type of iterator.
Iterators and Standard Algorithms
C++ Standard Template Library (STL) algorithms seamlessly integrate with iterators, allowing you to easily perform operations on container elements. For instance, you can use `std::sort`, `std::copy`, and many other algorithms with iterators to manipulate collections without writing custom loops, thus promoting cleaner and more maintainable code.
Custom Iterators
Creating a custom iterator is an advanced topic but is beneficial for specialized data structures. A custom iterator class can define how to traverse a specific type of container.
Example Code Snippet:
template <typename T>
class SimpleIterator {
T* ptr;
public:
SimpleIterator(T* p) : ptr(p) {}
T& operator*() { return *ptr; }
SimpleIterator<T>& operator++() { ++ptr; return *this; }
bool operator!=(const SimpleIterator& other) { return ptr != other.ptr; }
};
Custom iterators allow for greater control over the traversal logic and behavior, especially when dealing with non-standard data structures, providing enhanced flexibility to developers.

Conclusion
In summary, C++ iterators provide a powerful mechanism for accessing and manipulating elements in diverse container types, promoting code reuse and maintainability. Understanding the various types of iterators—input, output, forward, bidirectional, and random access—allows developers to select the most appropriate iterator for specific tasks.
As you delve deeper into using C++ iterators, consider experimenting with custom iterators and employing them alongside STL algorithms to leverage the full potential of the language. For further learning, check out C++ documentation, online tutorials, and recommended textbooks tailored to mastering this essential C++ feature.