C++ operator reference is a concise guide to the various operators in C++, their syntax, and their usage, enabling developers to perform operations with efficiency and precision.
Here's a simple code snippet demonstrating a few fundamental operators in C++:
#include <iostream>
int main() {
int a = 10, b = 20;
// Arithmetic Operators
std::cout << "Addition: " << (a + b) << std::endl; // Addition
std::cout << "Subtraction: " << (a - b) << std::endl; // Subtraction
std::cout << "Multiplication: " << (a * b) << std::endl; // Multiplication
std::cout << "Division: " << (b / a) << std::endl; // Division
// Relational Operators
std::cout << "Equal: " << (a == b) << std::endl; // Equal to
std::cout << "Not Equal: " << (a != b) << std::endl; // Not equal to
return 0;
}
What are Operators in C++?
Operators are special symbols or keywords in C++ that perform operations on one or more operands. They are fundamental to the way calculations and control flows are expressed in programming. By leveraging operators, programmers can handle calculations, comparisons, and logical operations seamlessly within their code. Understanding operators is crucial for writing efficient, clear, and maintainable C++ programs.
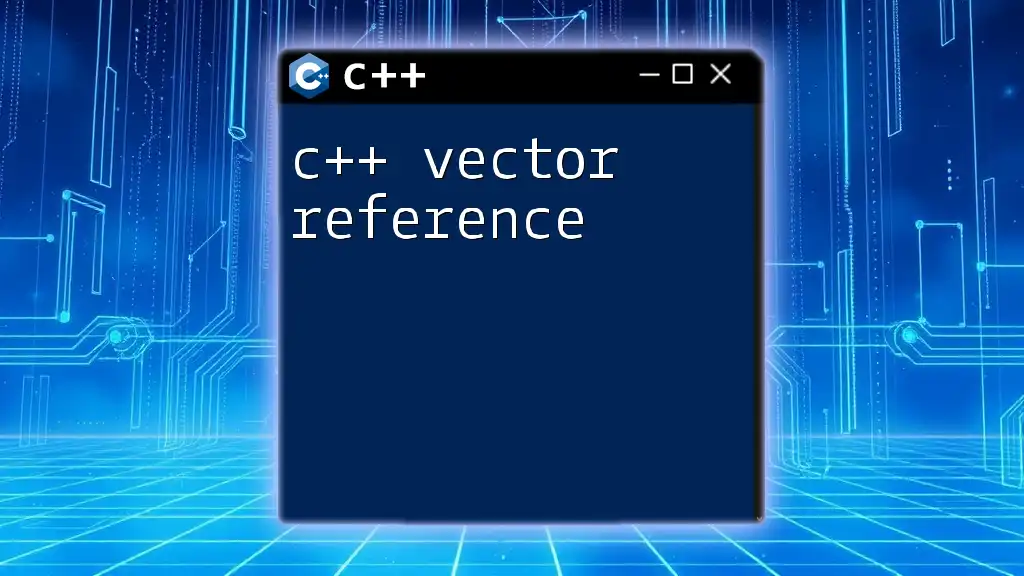
Types of Operators
C++ categorizes its operators into several types, each serving distinct functions:
- Arithmetic Operators for mathematical operations.
- Relational Operators for comparisons between values.
- Logical Operators for combining or negating Boolean expressions.
- Bitwise Operators for operations at the bit level.
- Assignment Operators for assigning values.
- Increment/Decrement Operators for modifying values by one.
- Conditional Operators for ternary evaluations.
- Comma Operators for evaluating expressions in a sequence.
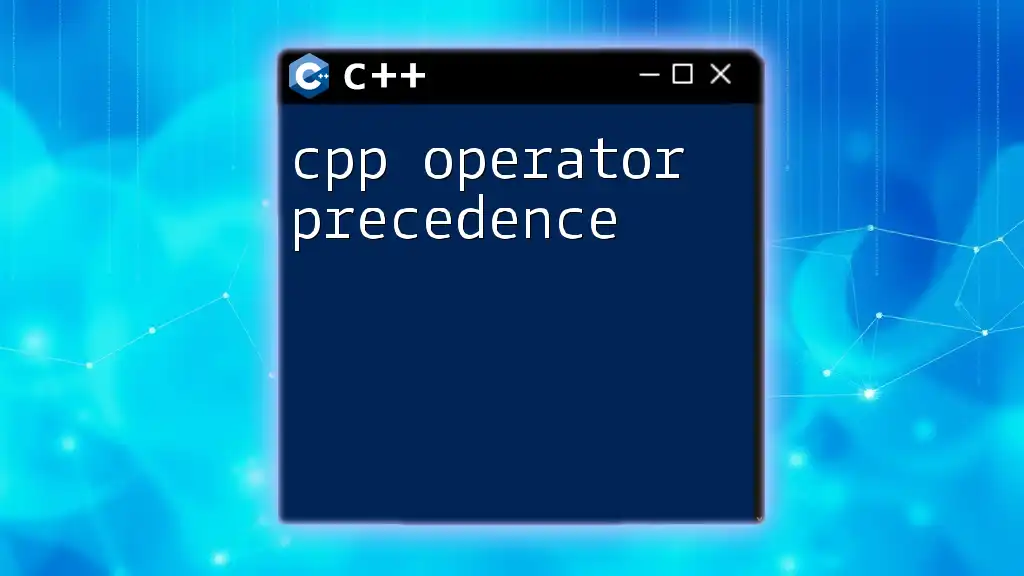
Detailed Breakdown of C++ Operators
Arithmetic Operators
Overview and Purpose
Arithmetic operators are used to perform mathematical calculations. They provide the basic operations one would expect in arithmetic.
Examples and Code Snippets
int a = 5, b = 3;
int sum = a + b; // Addition
int diff = a - b; // Subtraction
int prod = a * b; // Multiplication
int quot = a / b; // Division
int mod = a % b; // Modulus
In this example, `sum`, `diff`, `prod`, `quot`, and `mod` demonstrate how arithmetic operators can be utilized to perform fundamental calculations.
Relational Operators
Understanding Comparisons
Relational operators are used to compare two values and return a Boolean result (true or false) based on that comparison.
Examples and Code Snippets
bool isEqual = (a == b); // Equals
bool isNotEqual = (a != b); // Not equals
bool isGreater = (a > b); // Greater than
bool isLessOrEqual = (a <= b); // Less than or equal to
These operators are fundamental in decision-making processes within your code, allowing for the creation of conditions for flow control.
Logical Operators
Logical Operations in C++
Logical operators are employed to combine multiple Boolean expressions. The result of these operations is also a Boolean value.
Examples and Code Snippets
bool result = (a > b) && (a != 0); // Logical AND
bool output = (a < b) || (b == 0); // Logical OR
bool negation = !(a == b); // Logical NOT
This ability to control program flow based on multiple conditions makes logical operators essential for complex logic implementation.
Bitwise Operators
Manipulating Bits
These operators work at the binary level, manipulating individual bits of binary numbers. They are particularly effective for low-level programming and performance optimization.
Examples and Code Snippets
int bitwiseAnd = a & b; // Bitwise AND
int bitwiseOr = a | b; // Bitwise OR
int bitwiseXor = a ^ b; // Bitwise XOR
int leftShift = a << 1; // Left shift
int rightShift = a >> 1; // Right shift
Bitwise operations are powerful for applications involving flags, masks, and performance-sensitive routines.
Assignment Operators
Setting Values
Assignment operators are used to assign values to variables. They can also operate in a simplified form for compound assignments.
Examples and Code Snippets
int x = 10;
x += 5; // x = x + 5
x -= 2; // x = x - 2
x *= 3; // x = x * 3
These operators are vital as they simplify coding while maintaining clarity and intent.
Increment/Decrement Operators
Increasing and Decreasing Values
These operators are used for increasing or decreasing the value of a variable by one. They can be employed in two ways: pre-increment and post-increment.
Examples and Code Snippets
int y = 5;
y++; // Post-increment, evaluates to 5, then y becomes 6
++y; // Pre-increment, y becomes 7
Understanding the distinction between pre- and post- forms is important for avoiding subtle bugs in code execution.
Conditional Operators
Using Ternary Operator
The conditional operator, also known as the ternary operator, is a shorthand for if-else statements.
Examples and Code Snippets
int max = (a > b) ? a : b; // Ternary operation: max holds the greater of a or b
This operator is highly useful for compact code, although it should be employed judiciously to avoid decreasing code readability.
Comma Operator
Understanding the Comma Operator
The comma operator allows for multiple expressions to be evaluated in a single statement, with only the last expression's value being returned.
Examples and Code Snippets
int z = (a = 2, b = 3, a + b); // Evaluates and assigns values, z will be 5
Though less common, understanding when and how to use this operator can lead to more succinct code.
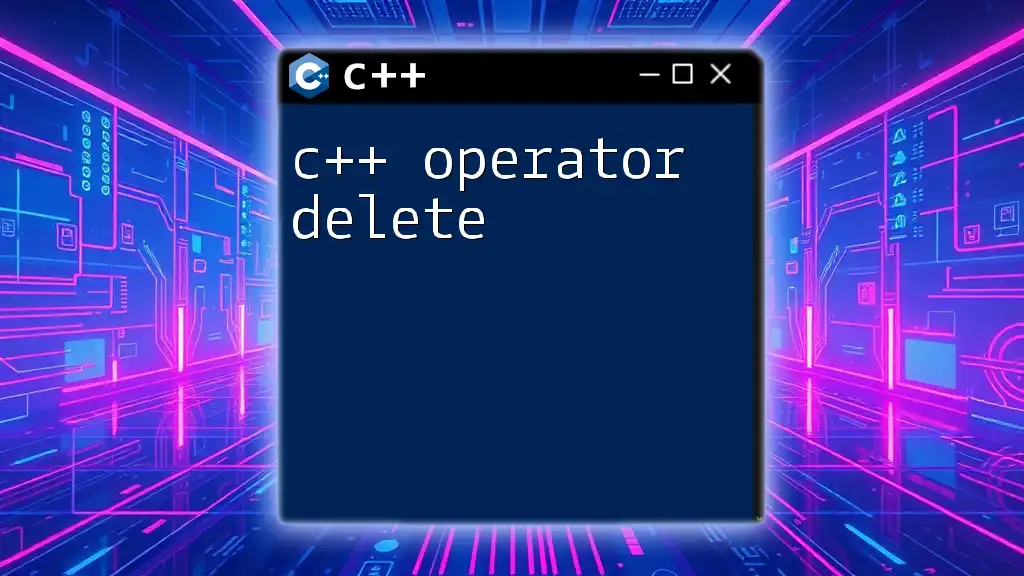
Operator Overloading in C++
What is Operator Overloading?
Operator overloading allows C++ operators to be redefined and used on user-defined types (classes). This expands the expressiveness and functionality of the code, making it easier to work with custom data types as if they are built-in types.
Examples of Operator Overloading
Overloading Operators
class Complex {
public:
float real, imag;
Complex(float r = 0, float i = 0) : real(r), imag(i) {}
// Overloading the + operator
Complex operator + (const Complex& obj) {
return Complex(real + obj.real, imag + obj.imag);
}
// Overloading the << operator for easy printing
friend std::ostream& operator<<(std::ostream& out, const Complex& c) {
out << c.real << " + " << c.imag << "i";
return out;
}
};
This example demonstrates how to overload the `+` operator for complex numbers, enhancing the readability and functionality of complex arithmetic operations.
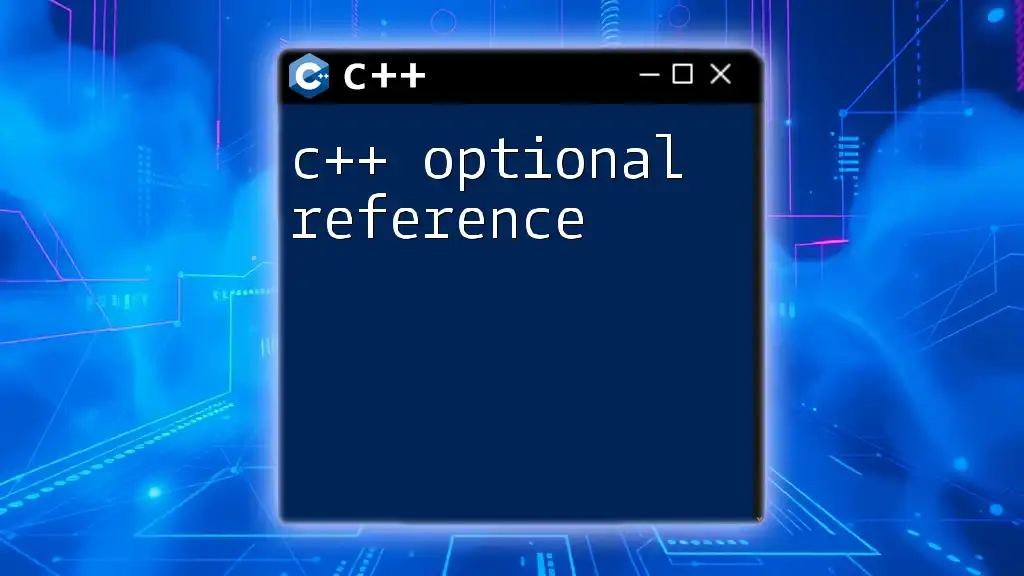
Best Practices
Writing Clear and Concise Operator Code
When using operators, clarity should be prioritized. It's beneficial to:
- Use operator overloading judiciously to avoid confusion.
- Stick to well-known operator functionalities to prevent misunderstandings.
Common Pitfalls to Avoid
One common mistake includes using overloaded operators in unexpected ways, which can lead to code misinterpretation. Always ensure overloaded operators behave in ways that are intuitive to their original purpose.
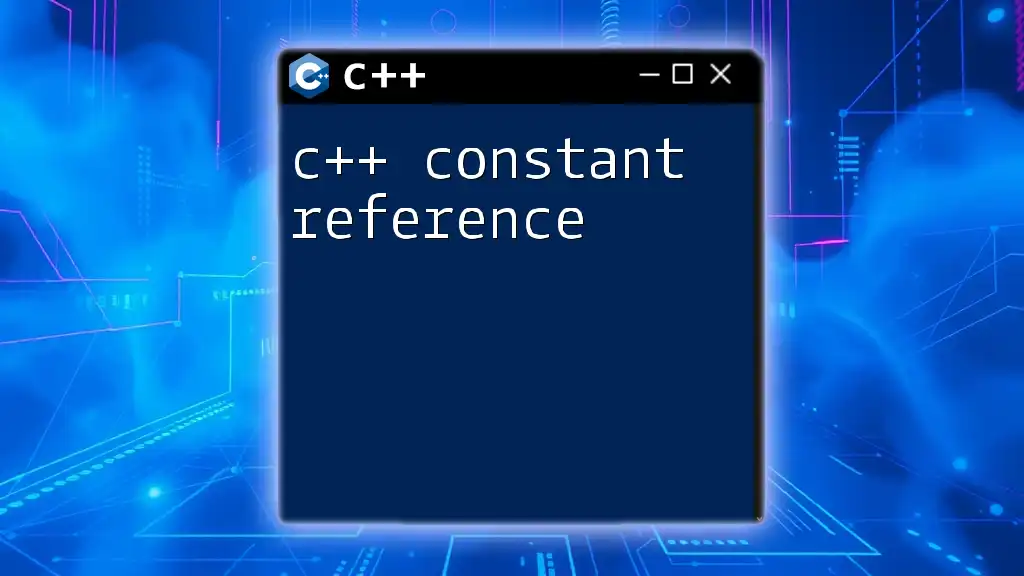
Conclusion
Mastering operators is essential for efficient programming in C++. By understanding how each operator functions and when to use them, programmers can write cleaner, more effective code. Practice and experimentation with different operator types will strengthen your skills and enhance your ability to solve problems using C++.
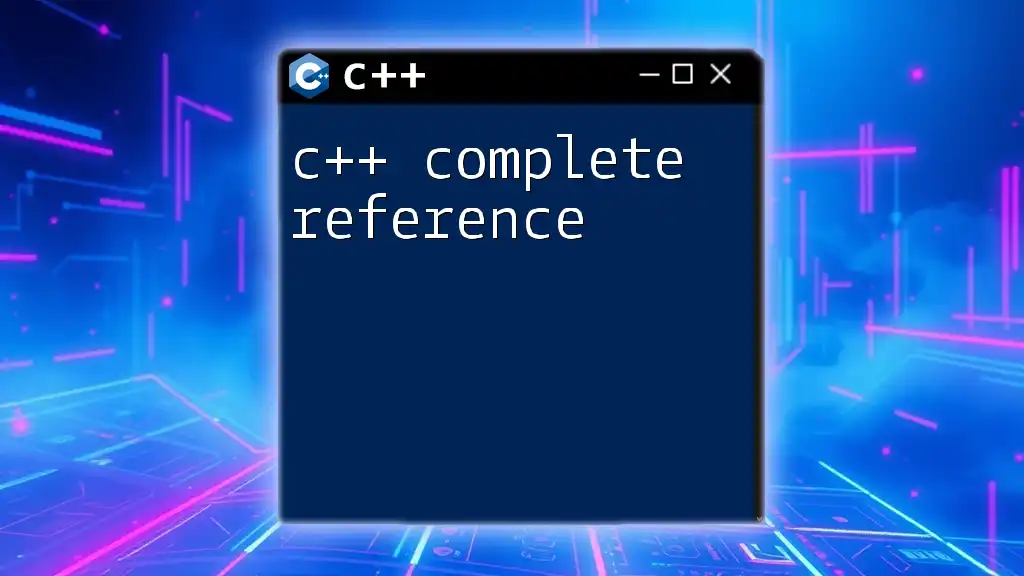
Additional Resources
Recommended Books and Online Courses
Investigate resources like "The C++ Programming Language" by Bjarne Stroustrup and consider online platforms such as Codecademy, Udemy, or Coursera for courses specifically covering C++ and its operators.
Community Forums and Platforms
Join programming communities like Stack Overflow or the C++ section on Reddit for discussions, problem-solving, and support from fellow developers as you continue to refine your understanding of C++ operators.