The C++ reference provides an organized compilation of the syntax, libraries, and commands essential for mastering C++ programming, enabling developers to quickly access and utilize crucial information.
Here’s an example of a simple C++ command that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What are C++ Commands?
C++ commands are instructions that tell the compiler what actions to perform in your program. They are essential for controlling the program's flow and operations. Understanding the difference between commands, functions, and statements is crucial for effective programming in C++. Commands can be seen as the building blocks of your code—each serves a unique purpose and helps establish the logic of your program.
C++ Command Syntax
The syntax of C++ commands is paramount. It dictates how your commands are structured and how they communicate with the compiler. A command typically includes keywords and parameters that are defined following specific rules.
For instance, a basic output command would follow this structure:
std::cout << "Hello, World!" << std::endl;
In this example, `std::cout` is the command used to display output, followed by the message enclosed in quotation marks. Syntax errors often occur from missing semicolons or incorrect keywords. Mastering syntax will greatly enhance the efficiency of your programming.
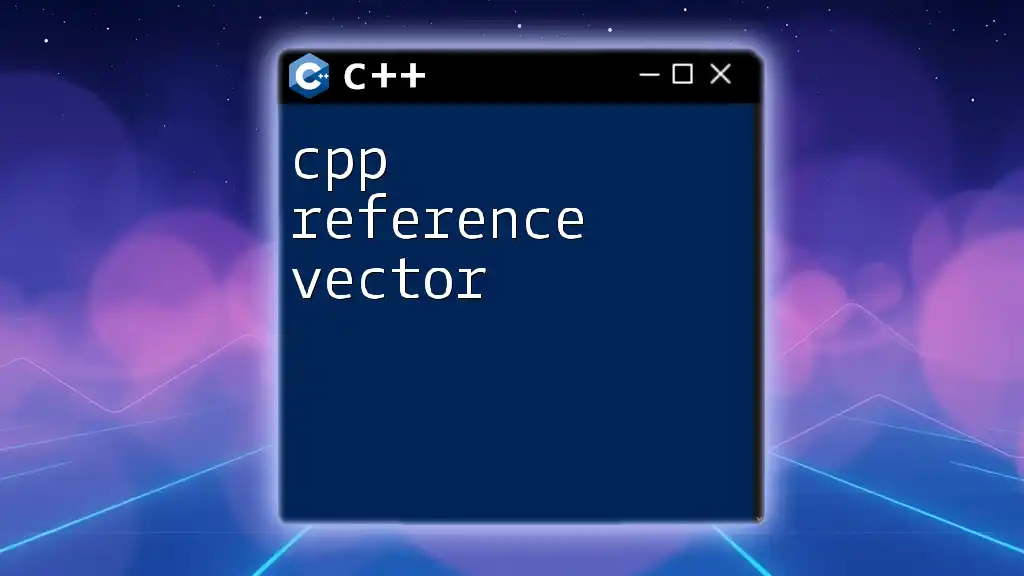
Core C++ Commands
Input and Output Commands
Input and output operations are fundamental in any programming language, and C++ provides built-in commands for these tasks.
-
`cin` Command: This command is used for obtaining user input from the standard input stream. It reads data entered by a user during the program execution.
int age; std::cout << "Enter your age: "; std::cin >> age;
In this snippet, the program prompts the user to enter their age and stores the input in the variable `age`.
-
`cout` Command: This command is used for outputting data to the standard output stream. It displays data or messages to the console.
std::cout << "Your age is: " << age << std::endl;
This line outputs the user's age to the console. Combining `<<` allows for chaining multiple outputs together, making it versatile and powerful for formatting.
Control Flow Commands
Control flow commands are essential for directing the execution of code based on certain conditions.
Conditional Statements
Conditional statements determine which block of code will execute based on a given condition.
-
`if`, `else if`, `else` Commands: These commands are utilized to create branches in the execution of the program.
if (age >= 18) { std::cout << "You are an adult." << std::endl; } else { std::cout << "You are a minor." << std::endl; }
This snippet evaluates the user's age and outputs a message indicating whether they are an adult or a minor. Proper use of conditions helps in making decisions and controlling the flow of the program.
Looping Commands
Loops enable you to run a block of code multiple times, depending on a specific condition.
-
`for`, `while`, `do while` each serve unique looping purposes:
-
For Loop:
for (int i = 0; i < 5; i++) { std::cout << i << std::endl; }
This loop iterates from 0 to 4, outputting each value. It's widely used when the number of iterations is known.
-
While Loop:
int j = 0; while (j < 5) { std::cout << j << std::endl; j++; }
In this case, the loop continues until `j` is no longer less than 5, demonstrating a condition-controlled execution.
-
Do While Loop:
int k = 0; do { std::cout << k << std::endl; k++; } while (k < 5);
The `do while` loop ensures that the block of code runs at least once before checking the condition.
-
Declaring and Defining Variables
Understanding command syntax also encompasses variable declaration and definition:
-
Variable Commands: Variable declaration specifies the type and allocates storage, while definition can assign a value.
int myNumber = 10; // Declaration and definition
Here, `myNumber` is both declared as an integer and initialized with the value 10. Proper variable usage enhances data handling and manipulation throughout your code.
Function Commands
Functions are crucial for creating modular and reusable code.
-
Defining Functions:
int add(int a, int b) { return a + b; }
In this function, `add` takes two integers as parameters and returns their sum. This encapsulation allows for better organization and reusability of code within larger programs.
-
Calling Functions:
std::cout << "Sum: " << add(5, 3) << std::endl; // Outputs: Sum: 8
This invocation of the `add` function outputs the result directly, showcasing how functions can streamline code execution.
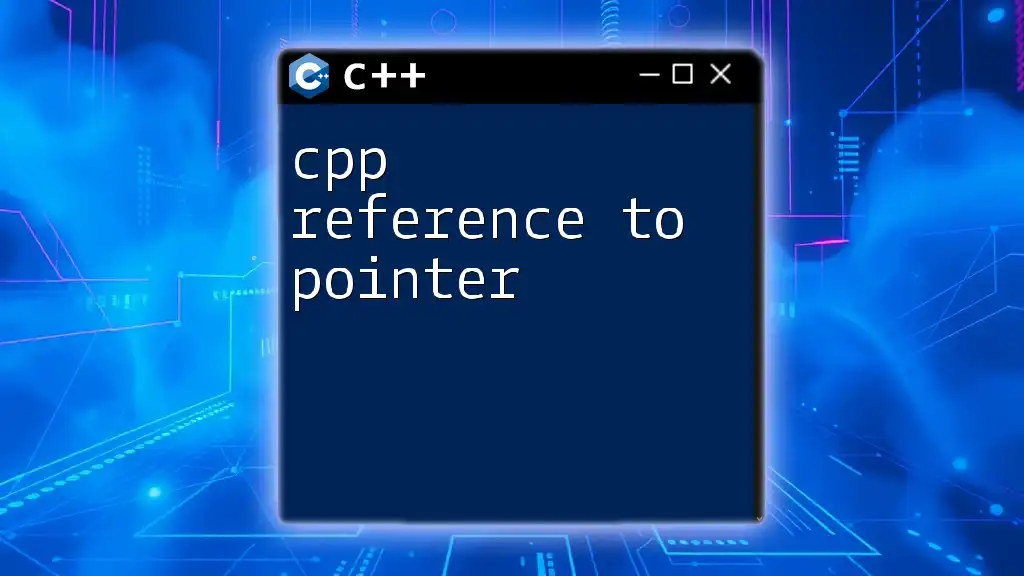
Advanced C++ Commands
Object-Oriented Commands
C++ supports object-oriented programming, which enhances the structuring of complex programs through the use of objects and classes.
Class Commands
-
Defining Classes:
class Car { public: void start() { std::cout << "Car is starting." << std::endl; } };
In this example, the `Car` class includes a method `start`. Classes encapsulate data and functionality, allowing for better organization and representation of real-world entities.
Inheritance Commands
Inheritance facilitates the creation of new classes based on existing ones, promoting code reuse.
class ElectricCar : public Car {
void charge() {
std::cout << "Electric car is charging." << std::endl;
}
};
This snippet demonstrates how `ElectricCar` inherits from `Car`, adding additional functionality to the base class. Inheritance is fundamental to object-oriented programming, allowing developers to build on existing code.
Exception Handling Commands
Robust programming requires handling errors gracefully.
-
Try-Catch Commands:
try { // Code that might throw an exception } catch (const std::exception& e) { std::cout << "Exception: " << e.what() << std::endl; }
This method allows you to handle runtime errors without crashing your program. By using try-catch, you can manage exceptions and ensure smooth execution flow, which is essential for user experience and program reliability.
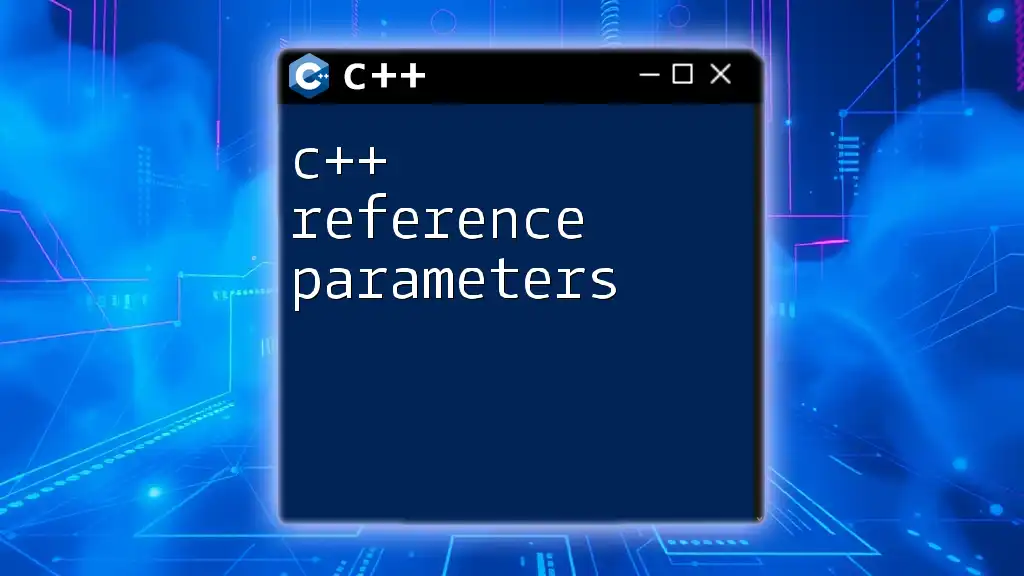
Resources for C++ Command Reference
Documentation
For comprehensive learning, always refer to reliable documentation. Websites like the official C++ documentation provide invaluable insights. Staying updated with the latest C++ standards is important as the language continues to evolve.
Community and Forums
Engaging with the programming community can enhance your learning experience. Online forums and platforms like Stack Overflow offer opportunities to ask questions, share knowledge, and collaborate with other programmers. This interaction fosters growth and understanding.
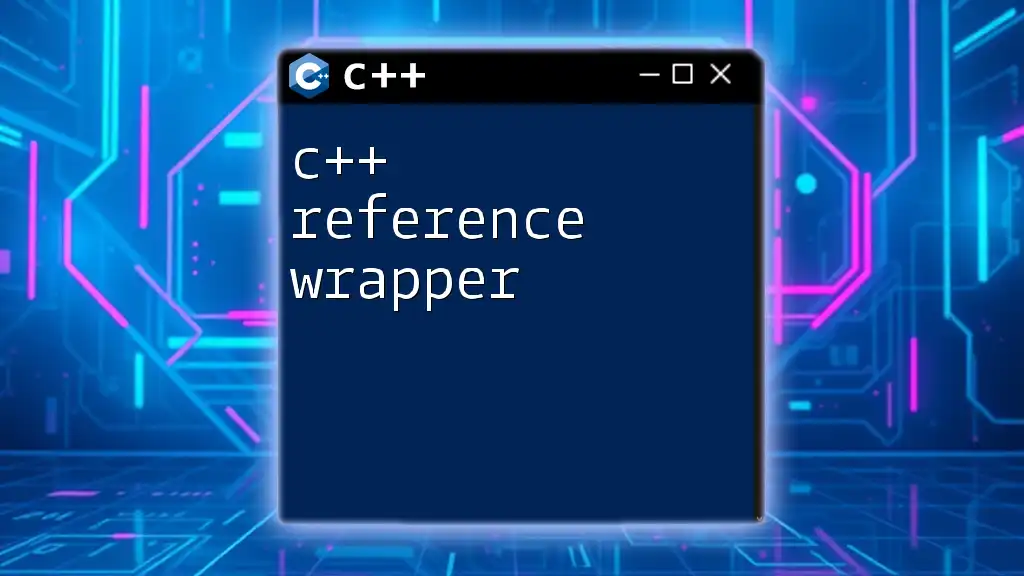
Conclusion
In mastering C++ commands, you lay the foundation for effective programming. Each command serves a critical role in defining the actions and logic of your programs. By applying what you've learned, experimenting with code, and engaging with the community, you can further enhance your C++ skills. Embrace the journey of discovery as you delve deeper into the world of programming!