In C++, dereferencing an iterator allows you to access the value at the element it points to, typically done using the `*` operator.
Here's an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int>::iterator it = vec.begin();
std::cout << "The first element is: " << *it << std::endl; // Dereference the iterator
return 0;
}
Understanding Iterators in C++
What are Iterators?
In C++, an iterator is an abstract representation of a pointer that allows traversal through the elements of a data structure without exposing the underlying details. Essentially, an iterator acts similarly to a pointer but provides a higher level of abstraction and flexibility. C++ offers several types of iterators:
- Input Iterators: Allow reading data from a container.
- Output Iterators: Allow writing data to a container.
- Forward Iterators: Support moving through a container in one direction.
- Bidirectional Iterators: Allow moving forwards and backwards.
- Random Access Iterators: Permit any access to elements in constant time, similar to pointers.
The Role of Dereferencing in Iterators
Dereferencing is a critical operation when working with iterators. It allows you to access the value that the iterator points to, akin to using a pointer. Understanding how to dereference iterators is essential for effective manipulation of data stored in various STL (Standard Template Library) containers.
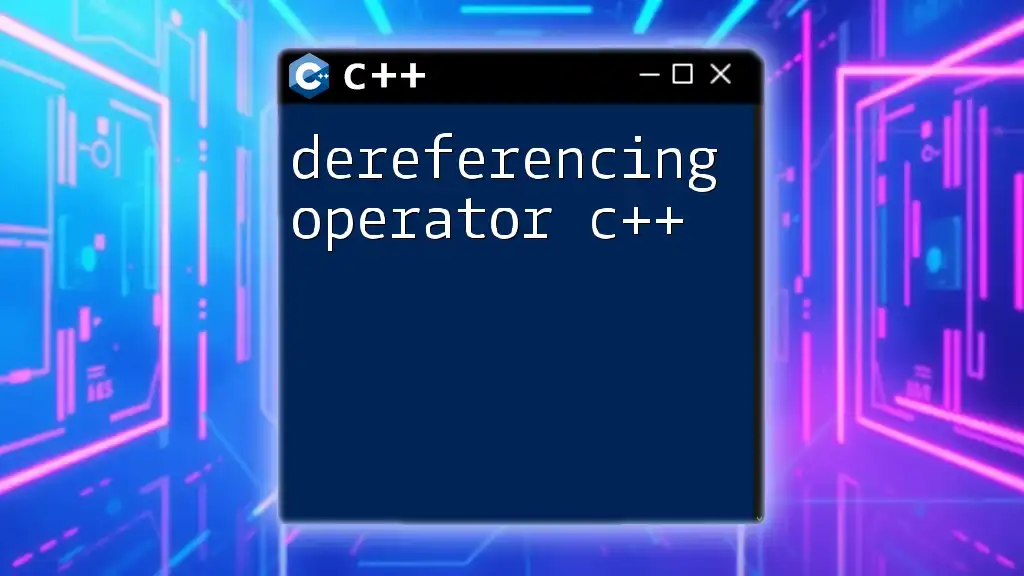
What is Dereferencing?
Definition of Dereferencing
Dereferencing an iterator means accessing the value of the element to which the iterator is currently pointing. This is done using the asterisk (`*`) operator, making it very similar to dereferencing a pointer.
Benefits of Dereferencing an Iterator
Dereferencing iterators provides several important benefits:
- Accessing Elements: Dereferencing allows you to quickly access the value of the current element in a container.
- Modifying Elements: By dereferencing, you can change the value that the iterator points to.
- Simplifying Iteration: Iterators simplify the process of navigating through various data structures, providing a clean and efficient way to iterate over elements.
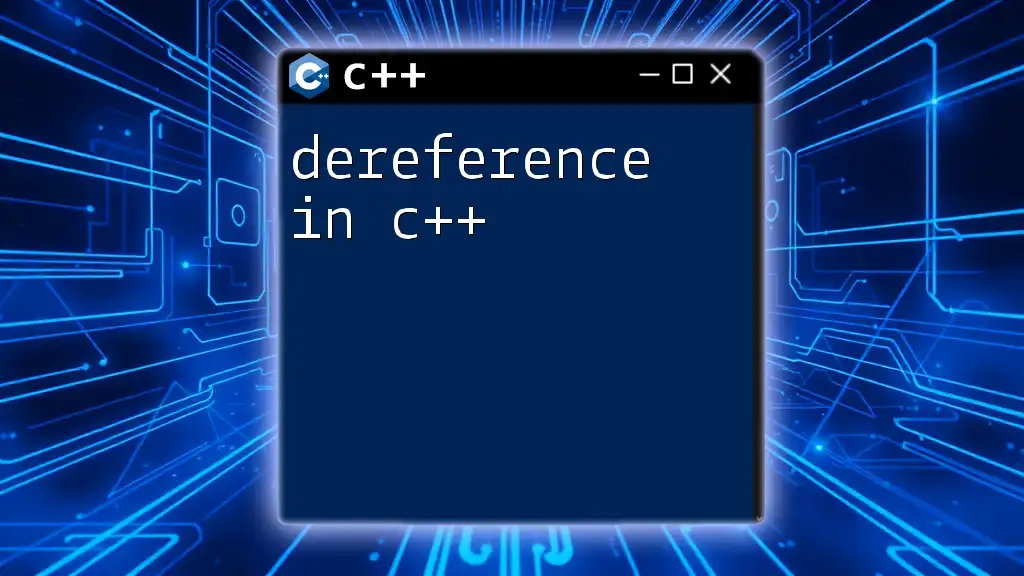
Working with Dereference Iterators
Basic Dereferencing Syntax
The syntax for dereferencing an iterator is straightforward. For example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30};
auto it = vec.begin(); // Iterator to the first element
std::cout << *it; // Dereferencing the iterator
return 0;
}
In this code snippet, `vec.begin()` initializes the iterator to point to the first element of the vector. When we dereference it using `*it`, we obtain the value `10`.
Dereferencing and Multiple Data Types
Dereferencing works seamlessly with various STL containers, such as `std::list` and `std::set`. The way you dereference remains consistent, regardless of the underlying data structure.
Using Iterators with Reference Types
Dereferencing can also involve reference types. When you use iterators with containers, any modifications via dereferencing affect the original elements. Consider the following example:
#include <iostream>
#include <vector>
void incrementElements(std::vector<int>& vec) {
for (auto it = vec.begin(); it != vec.end(); ++it) {
*it += 1; // Dereferencing to increment each element
}
}
int main() {
std::vector<int> vec = {1, 2, 3};
incrementElements(vec);
for (auto val : vec) {
std::cout << val << " ";
}
return 0;
}
In this case, dereferencing `*it` allows us to increment each element in the vector. The output will be `2 3 4`, demonstrating how iterators provide direct access for modifications.
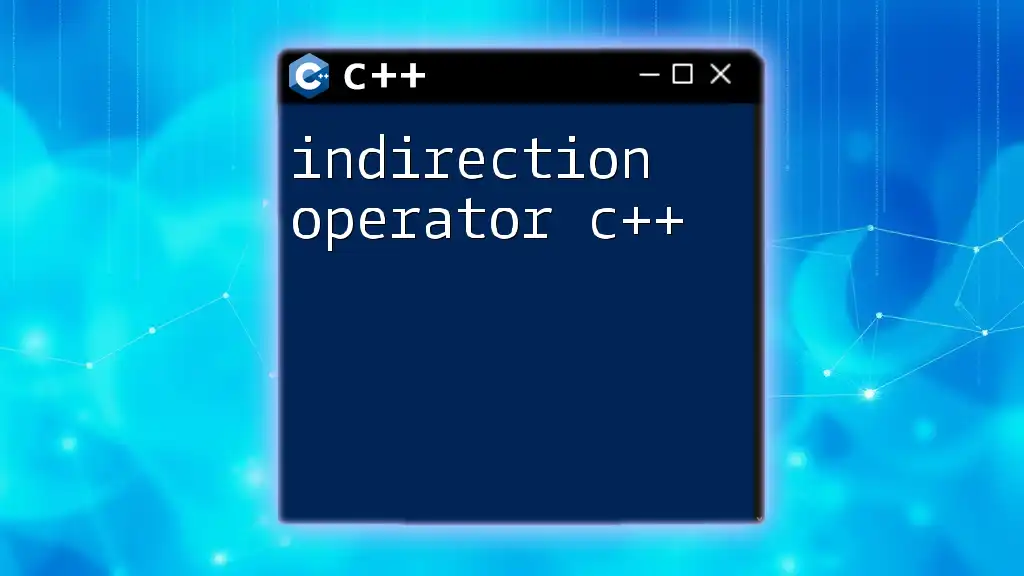
Advanced Dereferencing Techniques
Pointer Arithmetic with Iterators
Dereferencing isn’t just about reading or modifying values; you can also perform pointer arithmetic with iterators. This is particularly useful for navigating through data structures.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
auto it = vec.begin();
++it; // Move to the second element
std::cout << *it; // Output the second element
return 0;
}
In this example, we increment the iterator to point to the second element, which results in the output `2`.
Comparing Dereferencing in Custom Containers
When creating custom containers, you may wish to implement custom iterators. This requires implementing the dereference operator in your iterator class. Here’s a brief illustration of a custom iterator:
class CustomIterator {
public:
// Implementation details
int& operator*() {
return data[index]; // Dereferencing operator
}
};
Custom implementations can provide powerful capabilities market-optimized for your specific data structures.
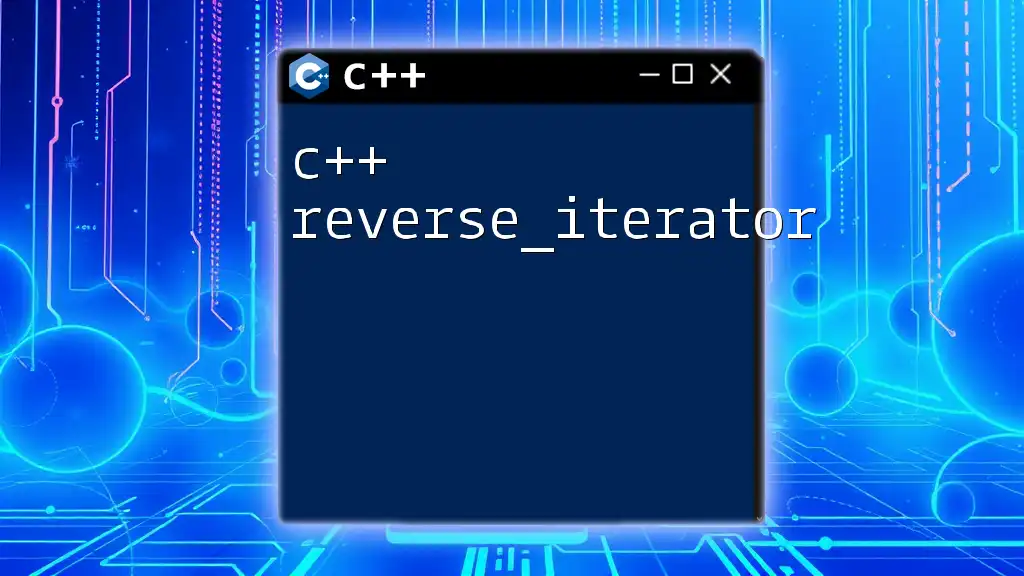
Common Pitfalls When Dereferencing Iterators
Dereferencing Past the End
Dereferencing an invalid iterator can lead to undefined behavior. For instance, if you were to dereference an iterator that points to the end of a container, you'd encounter issues.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3};
auto it = vec.end(); // Iterator to one-past-the-last element
// std::cout << *it; // Dereferencing this leads to undefined behavior
return 0;
}
Always ensure that an iterator is valid before attempting to dereference it to avoid runtime errors.
Invalidating Iterators
Certain operations can invalidate iterators. For example, if you modify a container (like adding or removing elements), iterators to that container may become invalid. It’s crucial to be aware of these scenarios to prevent dereferencing invalidated iterators.
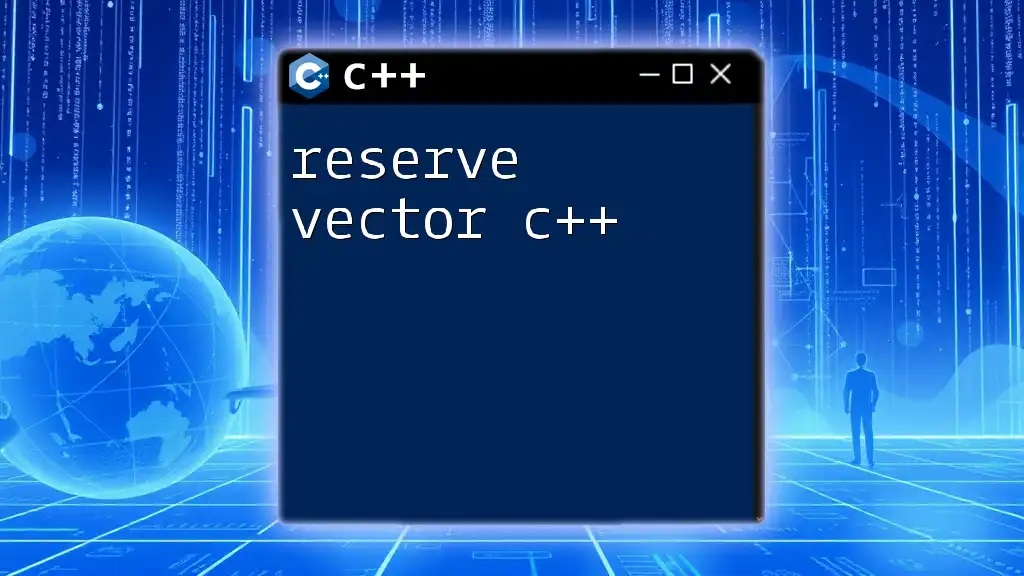
Dereferencing with Smart Pointers and Iterators
Using Smart Pointers with Iterators
Combining smart pointers with iterators enriches your C++ programming experience. Smart pointers manage memory automatically, and dereferencing occurs just as with regular pointers.
#include <iostream>
#include <vector>
#include <memory>
int main() {
std::vector<std::unique_ptr<int>> vec;
vec.push_back(std::make_unique<int>(10));
auto it = vec.begin();
std::cout << **it; // Dereferencing smart pointer iterator
return 0;
}
In this code, we first create a unique pointer that points to an integer. When dereferencing `it`, we access the value `10`, showcasing how dereferencing works with smart pointers.
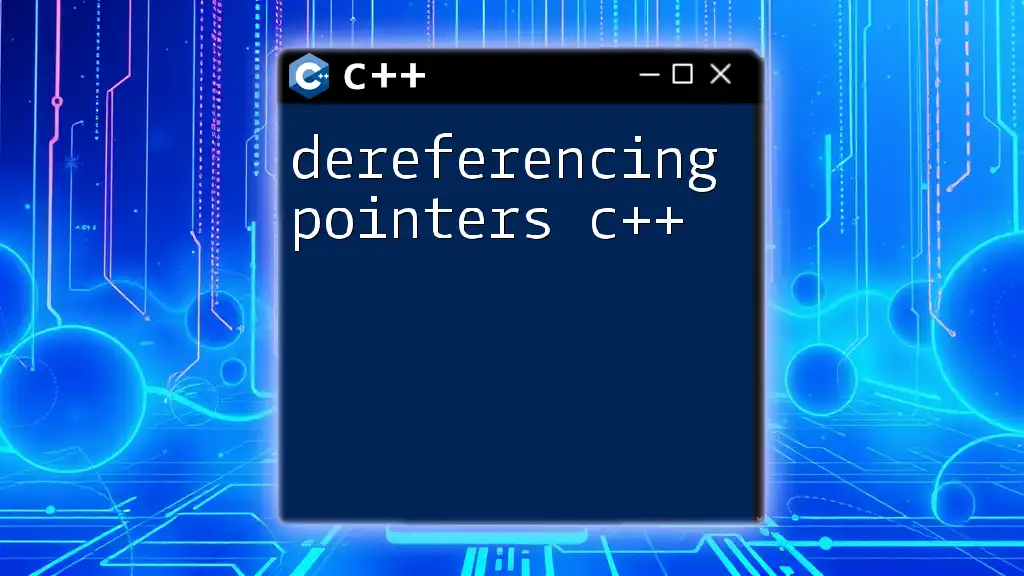
Conclusion
Understanding how to dereference iterators in C++ is an essential skill for effective programming. It enables you to navigate and manipulate data structures in a clean and efficient manner. From basic usage to advanced techniques, this guide equips you with the knowledge needed to leverage iterators effectively. Practice using iterators in your projects to strengthen your grasp of this critical component of C++.