Dereferencing pointers in C++ allows you to access the value stored at the memory location the pointer points to, using the dereference operator `*`.
Here’s a code snippet demonstrating this:
#include <iostream>
int main() {
int value = 42;
int* ptr = &value; // Pointer to the variable 'value'
std::cout << "Dereferenced value: " << *ptr << std::endl; // Accessing the value using dereferencing
return 0;
}
Understanding the Concept of Pointers
Pointers in C++ are variables that store the memory address of another variable. They are essential for direct memory access, allowing efficient data manipulation and enabling dynamic memory management. To grasp the use of pointers, it's crucial to understand the relationship between pointers and the concept of dereferencing.
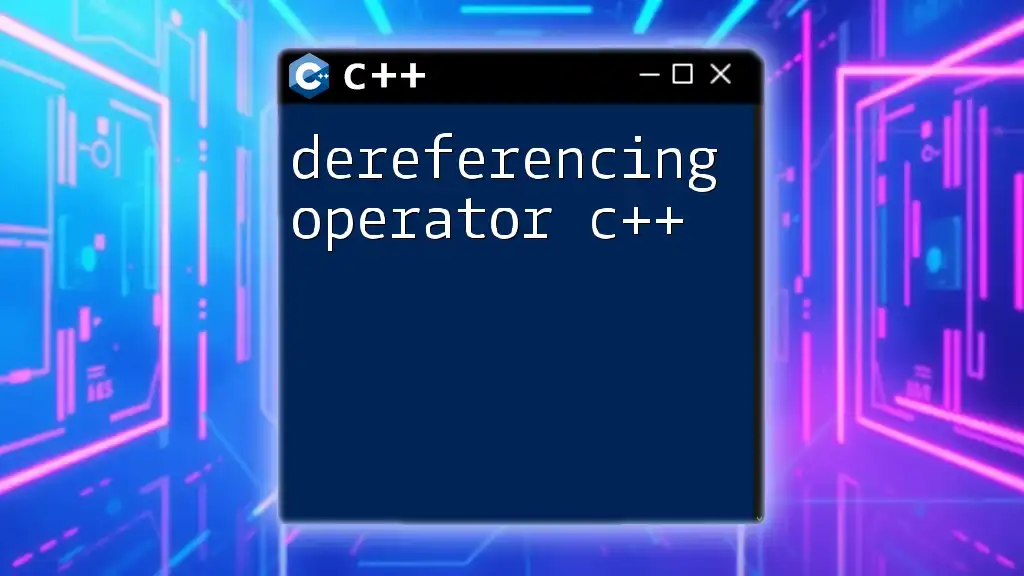
What is Dereferencing?
Dereferencing refers to the process of accessing the value stored at the address a pointer is pointing to. Simply put, when you dereference a pointer, you're getting the value of the variable that is located at the pointer's address. This functionality is central to using pointers effectively in C++.
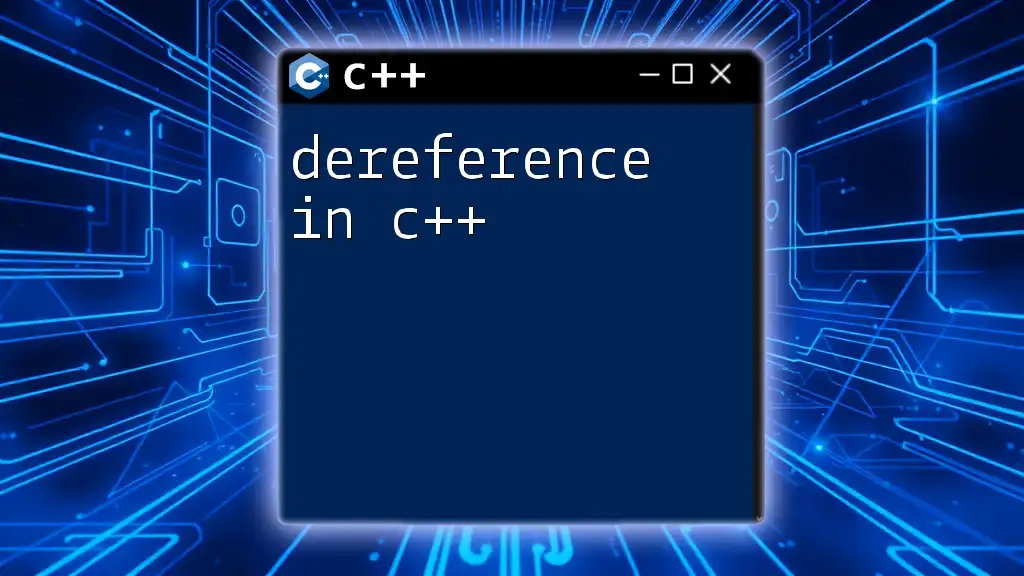
The Basics of Dereferencing in C++
How to Dereference a Pointer in C++
To dereference a pointer, you use the dereference operator, represented by the asterisk (`*`). The syntax is straightforward:
int main() {
int var = 42; // Declare an integer variable
int* ptr = &var; // Create a pointer pointing to the address of var
// Dereferencing the pointer
int value = *ptr; // Accessing the value at the pointer's address
return 0;
}
In this example, `ptr` is a pointer to `var`, and using `*ptr` allows us to access the value of `var`, which is `42`.
Example of Basic Dereferencing
Consider an extended example showing the relationship between a pointer and the variable it references:
#include <iostream>
using namespace std;
int main() {
int number = 10; // Declare an integer variable
int* numPtr = &number; // Pointer initialized to the address of number
cout << "Value of number: " << number << endl; // Outputs 10
cout << "Address of number: " << &number << endl; // Outputs the memory address
cout << "Value at numPtr: " << *numPtr << endl; // Dereferencing to obtain the value (outputs 10)
return 0;
}
Here, `*numPtr` allows us to access and display the content of `number` via the pointer.
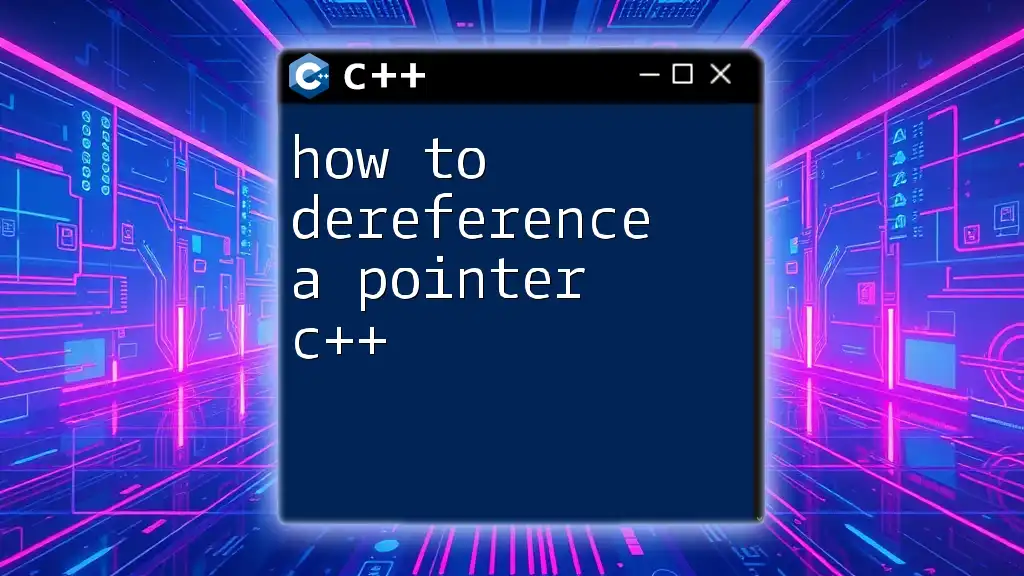
Types of Dereferencing
Dereferencing to Access Values
Dereferencing is primarily used to access the value stored in a variable:
int main() {
int value = 100;
int* ptr = &value;
cout << "Original value: " << *ptr << endl; // Access via pointer (outputs 100)
return 0;
}
Dereferencing to Modify Values
In addition to accessing values, pointers can also modify them. By dereferencing a pointer, you can change the content of the variable it points to:
int main() {
int num = 50;
int* ptr = #
*ptr = 75; // Modify the value at the pointed address
cout << "Modified value: " << num << endl; // Outputs 75
return 0;
}
This code segment illustrates how dereferencing allows you to change the original variable's value through the pointer.
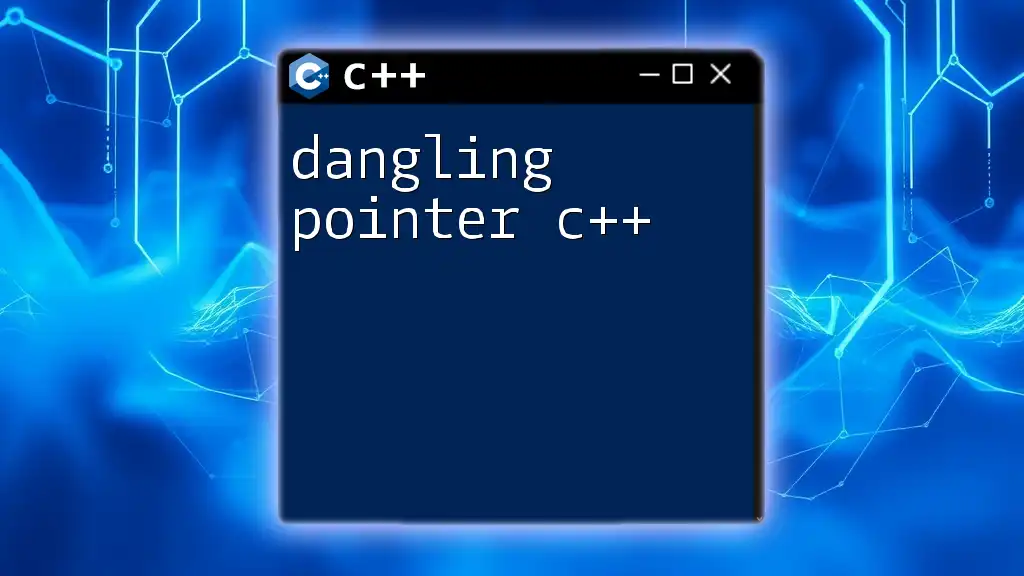
Understanding Pointer Dereference in Depth
Pointer Dereference Operator (`*`)
The dereference operator (`*`) is fundamental in pointer arithmetic and manipulation. It provides a means to access or change the data a pointer references directly. The difference between a pointer and its dereferenced value is critical to understand:
- Pointer: A variable holding a memory address.
- Dereferenced Value: The actual data at the memory address stored in the pointer.
Common Mistakes in Dereferencing
Dereferencing a null pointer can lead to undefined behavior and crashes. Always check for null before dereferencing:
int* nullPtr = nullptr;
if (nullPtr != nullptr) {
cout << *nullPtr << endl; // Safe dereference
} else {
cout << "Pointer is null, cannot dereference!" << endl; // Prevent crashing
}
Wild pointers — pointers that have not been initialized — can also cause issues. Always ensure that pointers are initialized properly.
Lifetime and Scope of Pointers
Dangling pointers occur when a pointer points to a memory location that has been deallocated. Avoiding this issue involves being conscious of the lifetime of variables:
int* danglingPtr;
{
int temp = 5;
danglingPtr = &temp;
} // temp goes out of scope here
// DanglingPtr is now a dangling pointer
cout << *danglingPtr; // Undefined behavior
Carefully managing pointer scope is crucial to prevent dereferencing errors.
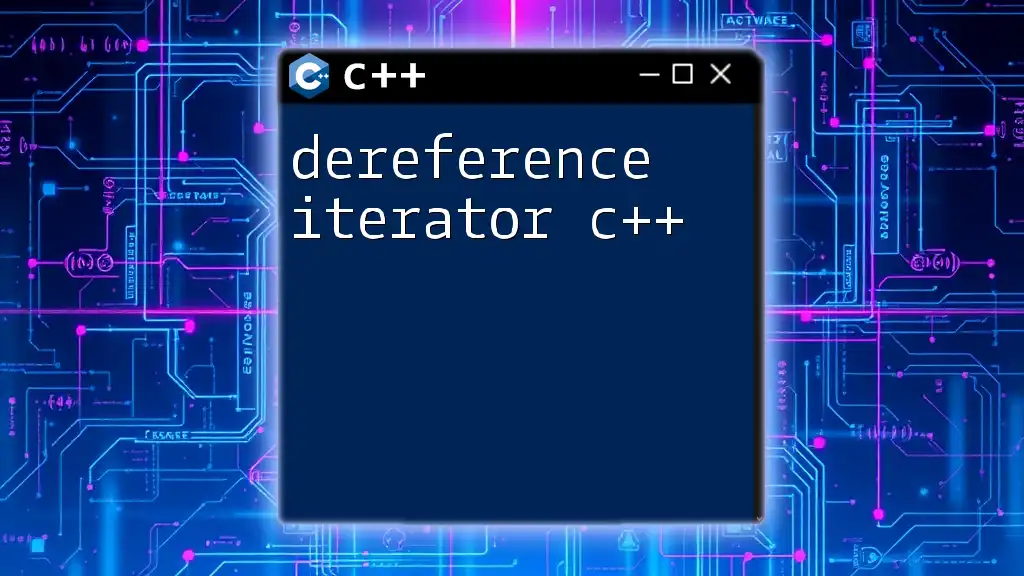
Advanced Dereferencing Techniques
Multi-level Pointers
Multi-level pointers allow for more complex data structures. A double pointer (`int**`) can store the address of a pointer.
int main() {
int value = 30;
int* ptr = &value; // Pointer to value
int** ptr2 = &ptr; // Double pointer to ptr
cout << "Value via double pointer: " << **ptr2 << endl; // Outputs 30
return 0;
}
This concept is useful for dynamic data structures like linked lists or trees.
Dynamic Memory Allocation and Dereferencing
Using dynamic memory management, you can allocate and deallocate memory at runtime. With `new` and `delete`, dereferencing becomes essential for managing heap-allocated memory:
int main() {
int* dynamicInt = new int; // Allocate memory for an integer
*dynamicInt = 100; // Dereference to assign value
cout << "Value: " << *dynamicInt << endl; // Outputs 100
delete dynamicInt; // Free allocated memory
return 0;
}
This example illustrates how to properly manage memory using pointers, highlighting the importance of dereferencing in dynamic contexts.
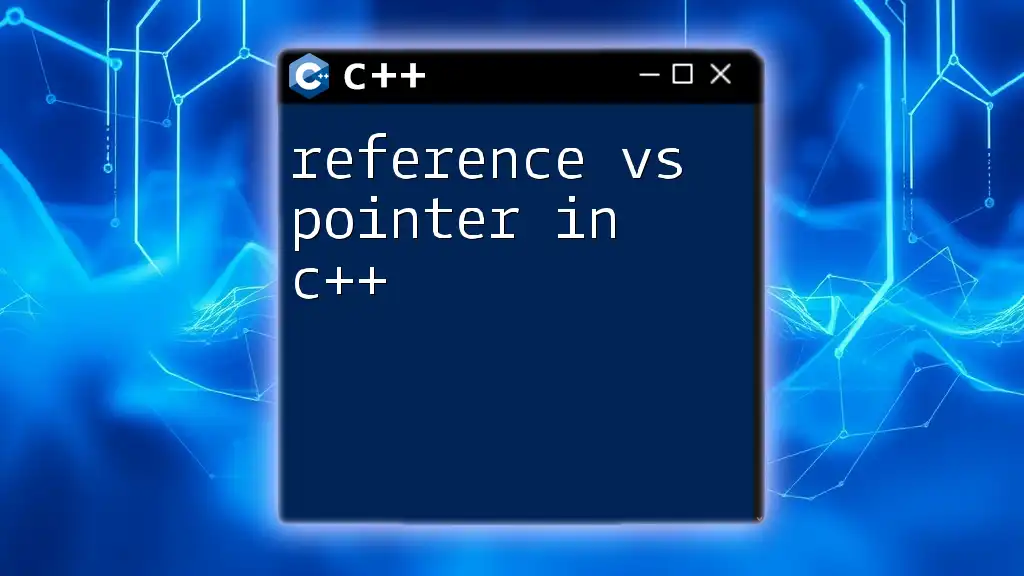
Best Practices for Dereferencing Pointers
Safe Dereferencing Strategies
Implement safety checks and error-handling measures to avoid issues related to pointer dereferencing:
- Always check for null pointers before dereferencing.
- Use smart pointers (like `std::unique_ptr` and `std::shared_ptr`) to manage memory automatically and reduce risks of memory leaks.
Performance Considerations
Dereferencing pointers can have implications on performance. While pointer dereferencing is generally fast, excessive dereferencing (especially in loops) can add overhead. It's crucial to weigh the performance options when designing algorithms.
Understanding the difference between stack and heap allocation will also aid in optimizing dereferencing use cases, as accessing stack variables is generally quicker than accessing heap-allocated memory.
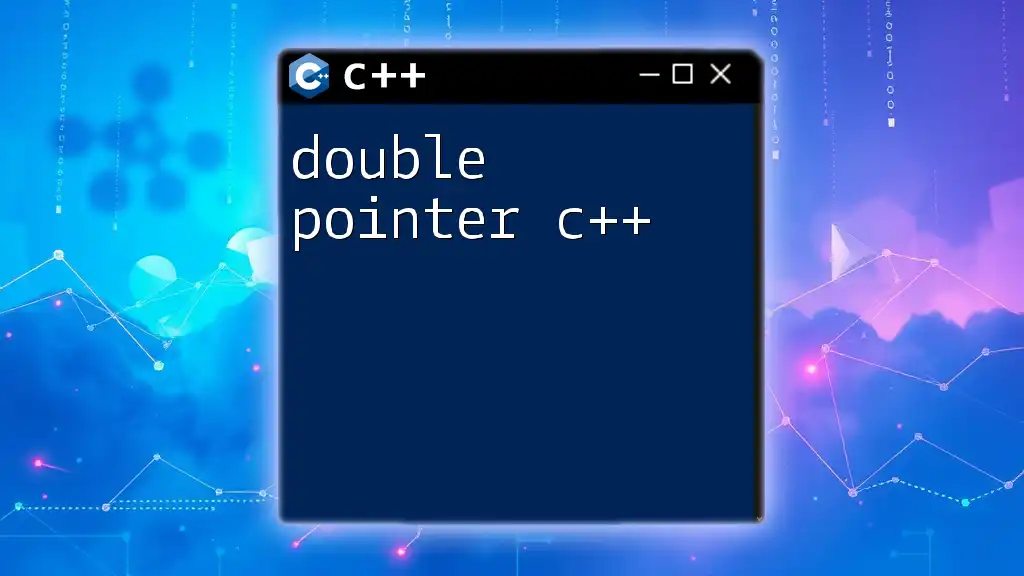
Debugging Dereferencing Issues
Common Errors and Solutions
Dereferencing problems, like segmentation faults, are prevalent in C++ logic. Using tools like gdb or Valgrind can help identify memory issues quickly.
gdb ./your_program
run
# When a crash occurs:
bt # Backtrace to examine stack actions
Real-Life Debugging Example
Consider a scenario where dereferencing a pointer caused a crash. Using debugging tools, you can analyze memory and pinpoint the errant code.
int main() {
int* errorPtr = nullptr;
cout << *errorPtr; // This will cause a crash
}
Using `gdb`, you can trace this back to the null dereference and implement checks to mitigate such errors in future developments.
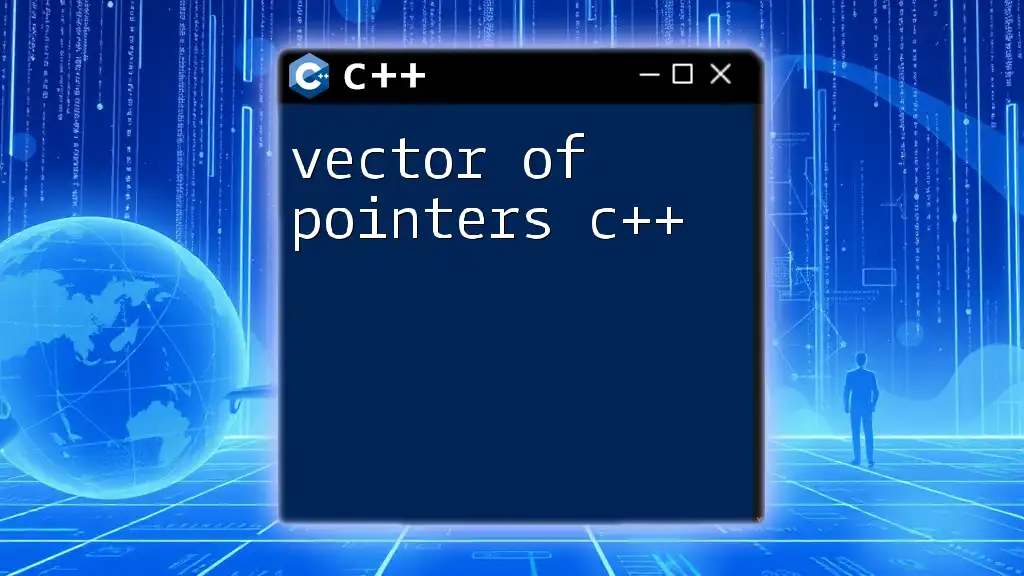
Conclusion
Understanding dereferencing pointers in C++ is fundamental in effectively leveraging the power of pointers. From accessing values to manipulating data, mastering these concepts allows developers to optimize memory use and enhance program efficiency. Practice implementing dereferencing in your coding projects to solidify these concepts and improve your programming skills.
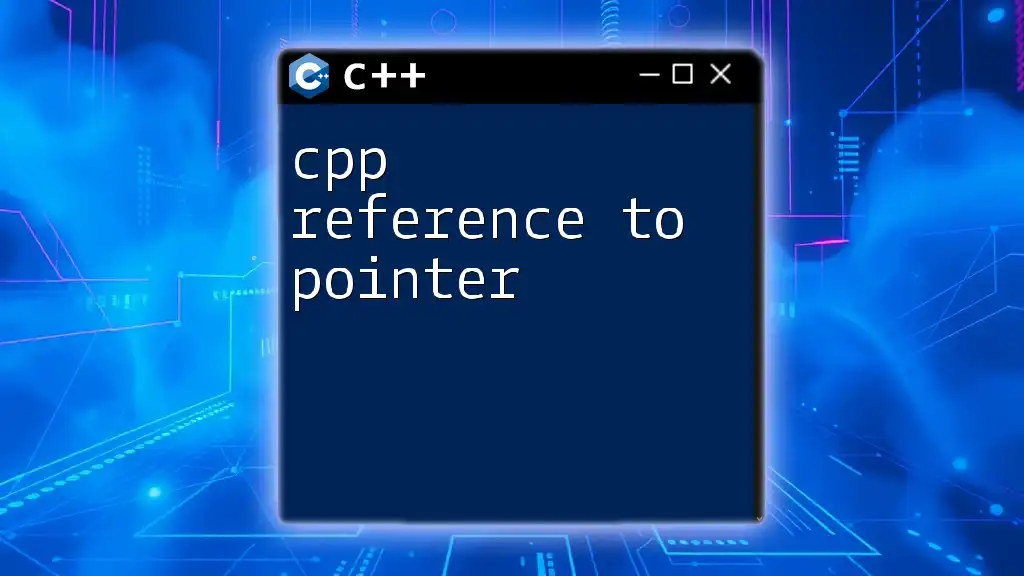
Additional Resources
For those seeking to dive deeper, consider exploring resources like online tutorials, books on memory management in C++, or courses that focus on pointers and memory handling. Engage in coding communities or forums to ask questions and share your experiences, as collaboration is a key part of the learning process.