Using pointers in C++ allows for efficient memory management and manipulation of data, enabling dynamic allocation and the ability to create complex data structures like linked lists.
Here's a simple code snippet demonstrating pointer usage in C++:
#include <iostream>
int main() {
int num = 42; // Initialize an integer variable
int* ptr = # // Declare a pointer and assign it the address of num
std::cout << "Value of num: " << *ptr << std::endl; // Output the value using pointer dereferencing
return 0;
}
What are Pointers?
Definition of Pointers
A pointer in C++ is a variable that stores the memory address of another variable. Unlike regular variables that hold values directly, pointers give you more control over how and where data is stored in memory. In a language such as C++, which allows low-level memory manipulation, pointers are an essential mechanism to manage data more effectively.
Pointer Syntax
The syntax for declaring a pointer is straightforward. You define a pointer variable with the data type of the variable it will point to, followed by an asterisk (*) symbol.
int* ptr; // Pointer to an integer
To initialize a pointer, you assign it the address of another variable by using the address-of operator (&):
int var = 10;
int* ptr = &var; // ptr now points to var
This initializes the pointer `ptr` to hold the address location of `var`, allowing you to manipulate `var` through its memory location.

Why Use Pointers in C++?
Memory Management
Dynamic Memory Allocation
Pointers are crucial for dynamic memory allocation in C++. When you need memory at runtime, such as creating an array whose size isn't known until execution, pointers enable you to allocate that memory dynamically using the `new` operator. Conversely, the `delete` operator frees that memory when you no longer need it, preventing memory leaks.
int* arr = new int[5]; // Allocating memory for an array of 5 integers
delete[] arr; // Freeing the allocated memory
In this example, `arr` is a pointer to a dynamic array. Using `delete[]` ensures that the memory is released properly once it's no longer needed.
Efficient Use of Memory
Passing large data structures into functions would typically involve copying the entire structure, which can be inefficient and wasteful. Using pointers allows you to pass the address of the data structure instead, conserving memory and improving performance.
For example, consider a function processing a large data structure:
void processLargeData(DataStructure* data) {
// Manipulate data without copying the entire structure
}
By passing a pointer, you avoid the overhead of data duplication and enhance the function's efficiency.
Performance
Reducing Overhead
Pointers can optimize performance by minimizing overhead when managing data. When manipulating large data sets or performing operations that require accessing data structures, pointers prevent unnecessary copying, which can slow down the program.
To illustrate, consider the following example of passing an array to a function through a pointer, which avoids the cost of copying the array itself:
void modifyArray(int* arr, int size) {
for (int i = 0; i < size; i++) {
arr[i] += 2; // Modify the array directly
}
}
By passing the pointer `arr` instead of the entire array, the function operates directly on the original array.
Data Structures
Complex Data Structures
Pointers are invaluable when implementing complex data structures like linked lists, trees, and graphs. These structures frequently rely on pointers to connect elements because they cannot always be fixed in size or order.
For instance, a simple implementation of a linked list uses pointers to connect nodes:
struct Node {
int data;
Node* next; // Pointer to the next node
};
Each node holds data and a pointer to the next node, allowing for a flexible, dynamic structure that can grow and shrink as needed.
Function Arguments
Pass by Reference vs. Pass by Value
In C++, function parameters can be passed by value or by reference. When you pass by value, a copy of the variable is made, which can lead to performance issues with large data structures. Passing by reference using pointers avoids this overhead.
Here's how you can modify a variable by using pointers:
void increment(int* ptr) {
(*ptr)++; // Increments the value at the address ptr points to.
}
When the function `increment` is called with a pointer to an integer, it directly modifies the integer value in memory rather than working on a copy.
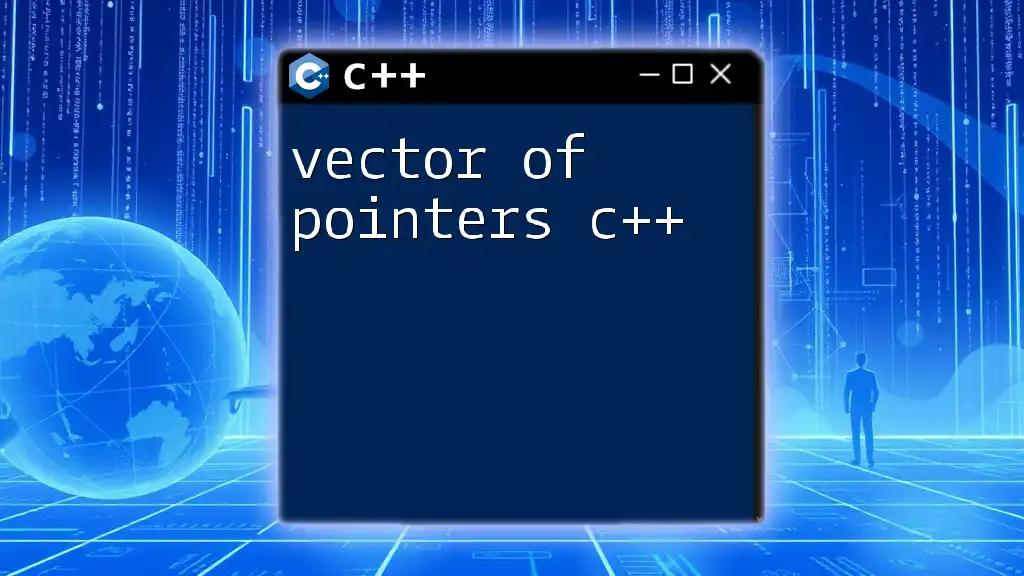
Common Misconceptions about Pointers
Pointers are Complicated
Many new programmers view pointers as intimidating and overly complex. While they do have a steeper learning curve, understanding pointers is crucial for effective C++ programming. With practice, the concepts become clearer, and their utility more apparent.
Pointers and Memory Safety
One common issue with pointers is dangling pointers, which occur when a pointer still references memory that has been deallocated. This can lead to undefined behavior if you try to access such memory. Following best practices, like initializing pointers and setting them to `nullptr` after deletion, can prevent these problems.

Best Practices for Using Pointers
Always Initialize Pointers
Initializing pointers upon declaration is a good habit that minimizes risks of undefined behavior caused by unintentionally accessing uninitialized memory. For instance:
int* ptr = nullptr; // Safe initialization
Setting pointers to `nullptr` helps in preventing dangling pointer issues.
Use Smart Pointers
C++11 introduced smart pointers like `std::unique_ptr` and `std::shared_ptr`, which help manage memory automatically, relieving developers from manual memory handling. These smart pointers ensure that memory is efficiently and safely managed, reducing the risk of leaks.
For example, a simple use case of `std::unique_ptr`:
#include <memory>
std::unique_ptr<int> ptr(new int(5)); // Automatically managed memory
Using smart pointers enhances code safety and can simplify ownership semantics.

Conclusion
In summary, why use pointers in C++? They are essential for dynamic memory management, improving performance, and implementing complex data structures. By mastering pointers and understanding their benefits, you can write more efficient, optimized code.
Hands-on practice is crucial for solidifying your understanding of pointers and developing confidence in your programming skills. Explore various scenarios, play around with code, and soon you will appreciate the power and flexibility pointers offer in C++.