A dangling pointer in C++ occurs when a pointer continues to reference a memory location after the object it points to has been deleted or deallocated.
Here’s a code snippet illustrating a dangling pointer:
#include <iostream>
void createDanglingPointer() {
int* ptr = new int(42); // Dynamically allocate an int
delete ptr; // Free the allocated memory
// ptr is now a dangling pointer
std::cout << *ptr; // Undefined behavior: accessing a dangling pointer
}
int main() {
createDanglingPointer();
return 0;
}
What is a Dangling Pointer?
A dangling pointer in C++ is a pointer that does not point to a valid object of the appropriate type. The pointer still contains the memory address, but that address no longer holds a valid value. Understanding dangling pointers is crucial for effective memory management and program stability, as they can lead to unexpected behavior or crashes.
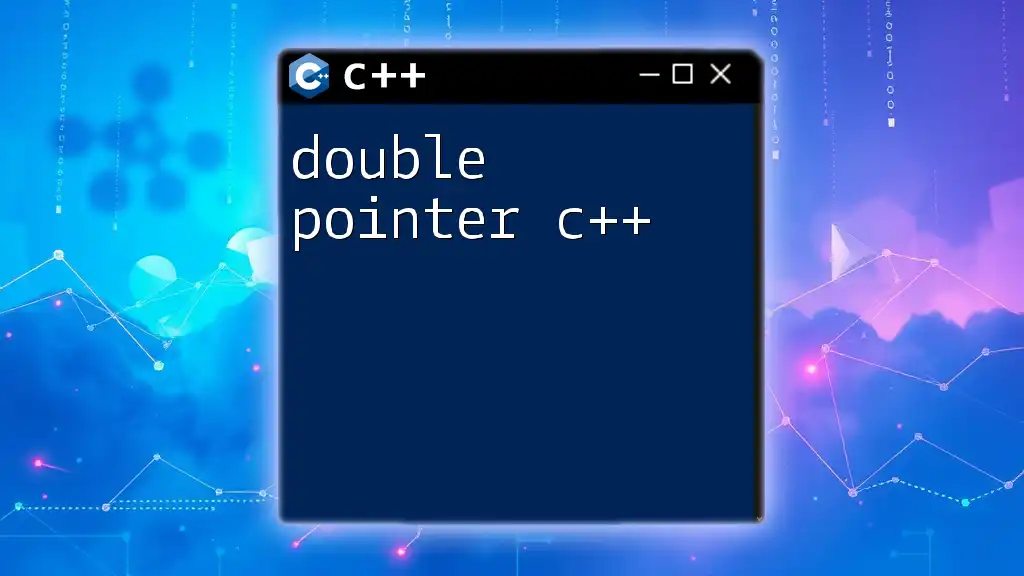
Importance of Understanding Dangling Pointers
The implications of not understanding dangling pointers can be severe, resulting in undefined behavior. A program may seem to work correctly during one run but can crash or produce erroneous results in another due to mere accidental access of a dangling pointer. By recognizing the dangers, developers can write safer, more reliable code.
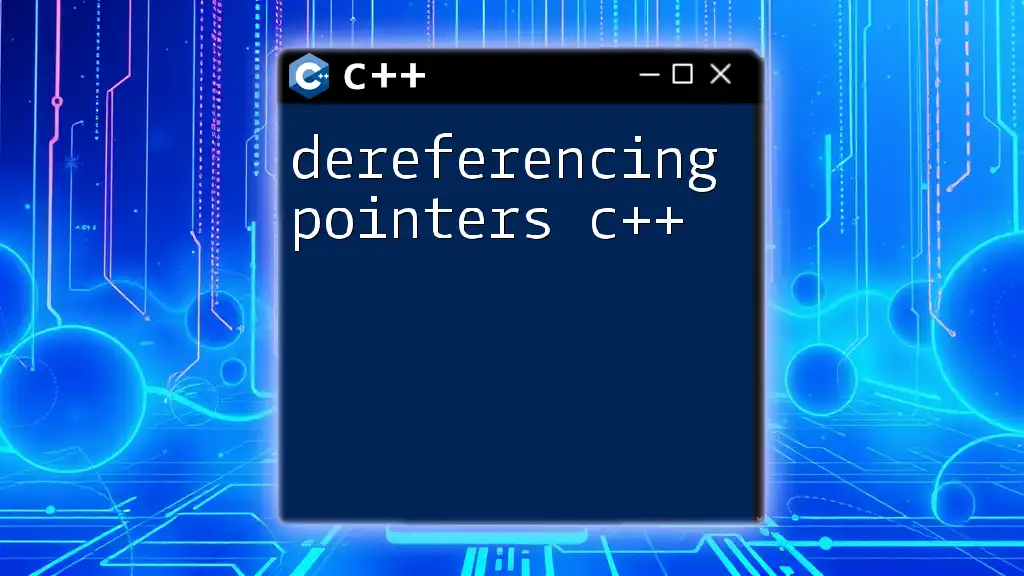
Causes of Dangling Pointers in C++
Deallocation of Memory
One of the most common causes of dangling pointers is the deallocation of memory. When memory that a pointer is referencing is freed, the pointer becomes dangling.
For example:
int* ptr = new int(10);
delete ptr; // ptr becomes a dangling pointer
In this case, `ptr` is still holding the address of the memory that has been deallocated, causing an unpredictable state if accessed thereafter.
Function Exiting
Dangling pointers can also occur when local variables are returned from a function. The memory allocated for local variables is released once the function exits:
int* danglingPtr() {
int localVar = 20;
return &localVar; // returning the address of a local variable
}
Here, `danglingPtr` returns the address of a local variable `localVar`. Once the function exits, this address points to an invalid memory location.
Use of a Pointer After Object Deletion
Deleting an object and then attempting to use the pointer to the deleted object creates yet another scenario for dangling pointers to arise. Consider this:
class Sample {
public:
void display() { std::cout << "Sample" << std::endl; }
};
Sample* obj = new Sample();
delete obj; // obj becomes a dangling pointer
After deleting `obj`, any further access through this pointer leads to undefined behavior.
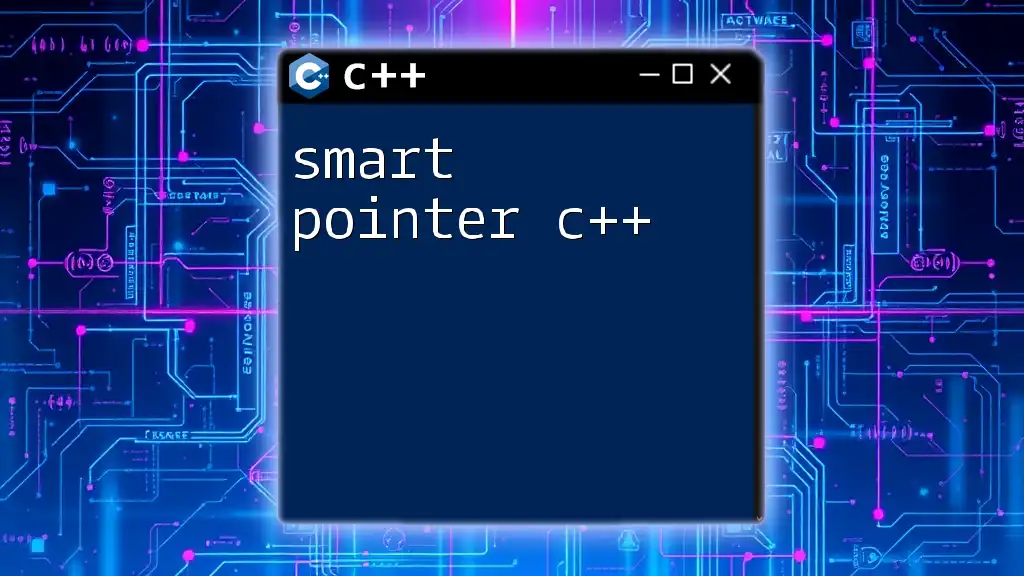
Risks and Consequences of Dangling Pointers
Undefined Behavior
The most alarming risk is undefined behavior. This means that the program may crash, produce incorrect results, or even appear to run successfully at times. Undefined behavior is notoriously difficult to diagnose, making it a major headache for developers.
Security Vulnerabilities
Dangling pointers can introduce security vulnerabilities. Malicious users might exploit these pointers to manipulate memory, leading to data corruption or unauthorized access. Hence, vulnerable code can be a significant risk in software applications, especially in environments that handle sensitive data.
Debugging Challenges
Debugging code that involves dangling pointers can be an arduous task. Issues arising from these could manifest far from the point of dereferencing the dangling pointer, complicating the debugging process because the source of error can appear random.
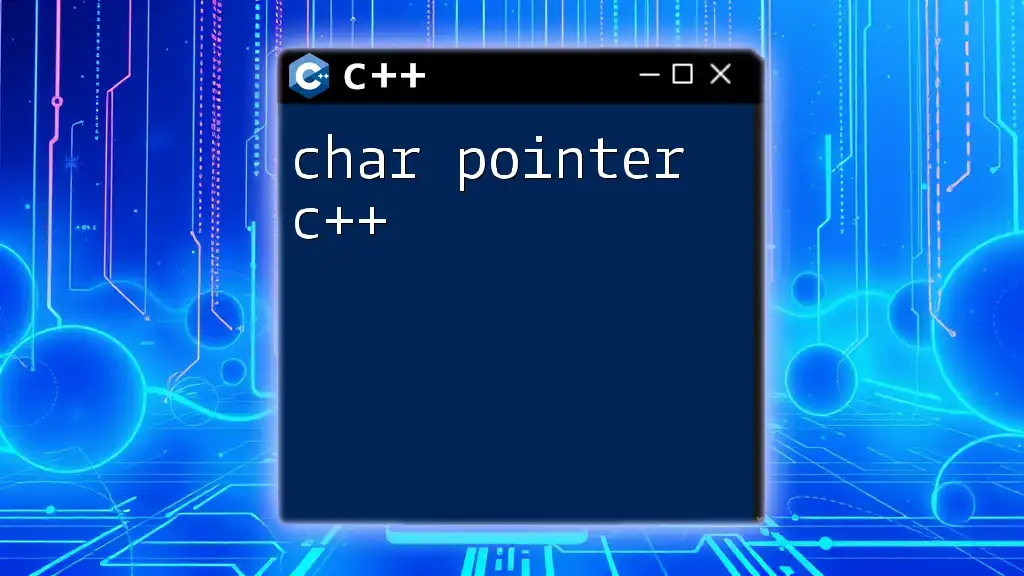
Identifying Dangling Pointers
Using Debugging Tools
To identify dangling pointers, developers can utilize debugging tools such as Valgrind and AddressSanitizer. These tools help detect memory mismanagement, including memory leaks, invalid accesses, and dangling pointers. Basic commands, like using Valgrind:
valgrind --leak-check=full ./your_program
This command will show detailed information about memory allocation and errors.
Code Review Practices
Another method to identify dangling pointers is through rigorous code review practices. Reviewers should focus on pointer usage within functions, the lifecycle of variables, and areas where memory allocations and deallocations occur. Such practices often catch potential issues before they make it to production.
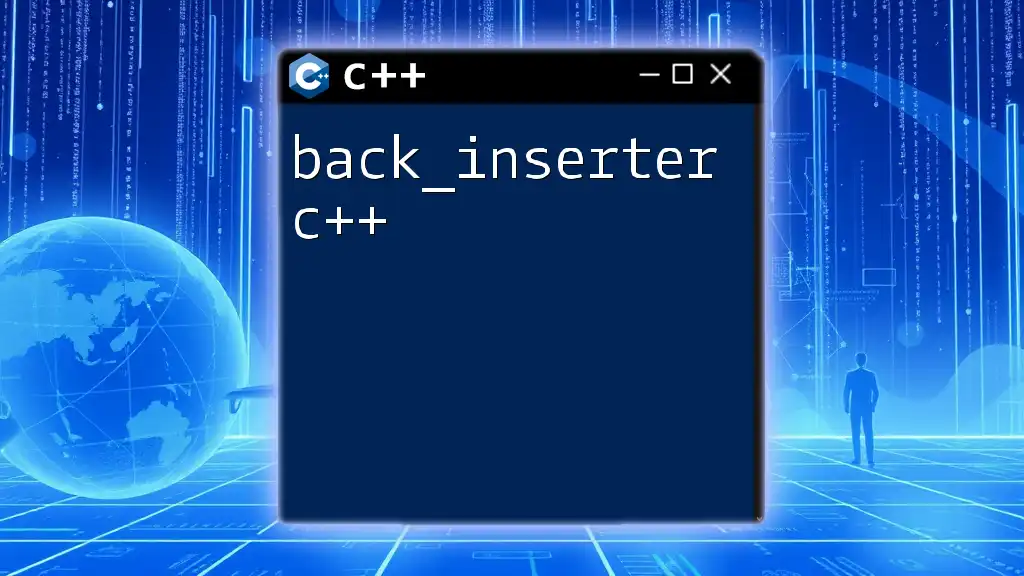
Preventing Dangling Pointers
Best Practices in C++ Programming
One of the most effective ways to prevent dangling pointers is by using smart pointers provided by C++11 and later. Smart pointers like `std::unique_ptr` and `std::shared_ptr` handle memory automatically, ensuring it is reclaimed when it goes out of scope.
std::unique_ptr<int> smartPtr(new int(10)); // Automatically freed
By employing smart pointers, developers can avoid manual memory management pitfalls.
Setting Pointers to Null
Another essential preventive measure is to reset pointers to null after they have been deleted. This practice ensures that the pointer does not accidentally reference invalid memory.
delete ptr;
ptr = nullptr; // Now, ptr is no longer dangling
Doing this allows for a safer dereference operation later on, as dereferencing a null pointer can be more easily caught than one that points to invalid memory.
Using RAII (Resource Acquisition Is Initialization)
RAII is a powerful programming idiom in C++ where resource allocation is tied to the lifespan of objects. In this model, resources are acquired during initialization and released upon object destruction. By applying RAII principles, developers ensure that their resources are managed appropriately, which indirectly mitigates the risk of dangling pointers.
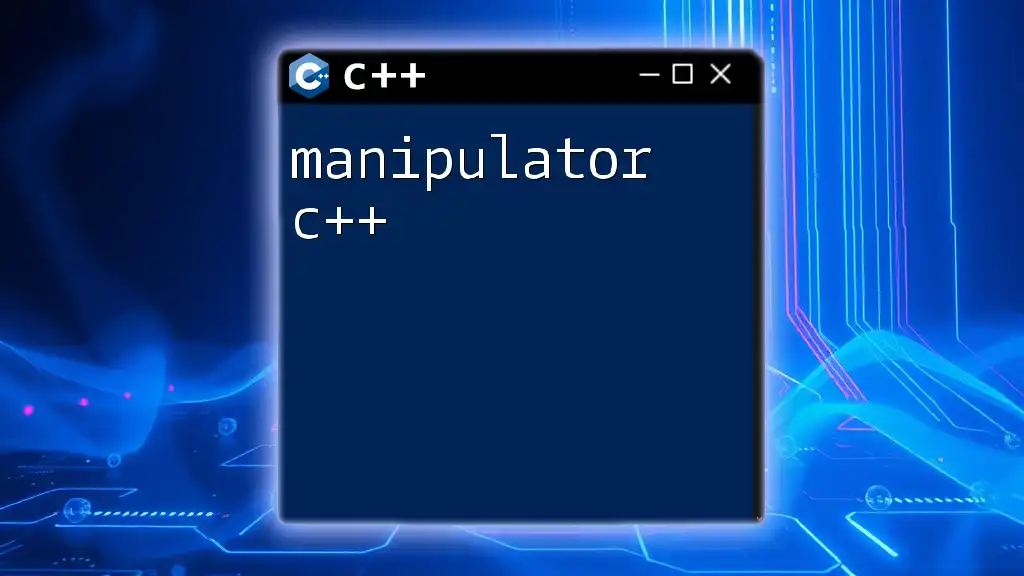
Examples of Dangling Pointers and Prevention Techniques
Code Snippet: Common Mistakes
Consider a function that creates a dangling pointer:
void danglingPointerExample() {
int* ptr = new int(5);
delete ptr;
std::cout << *ptr; // Unsafe access to dangling pointer
}
In this example, accessing `*ptr` after it has been deleted results in undefined behavior.
Code Snippet: Correcting the Mistakes
Here’s how we can correct the mistake by adopting better practices:
void safeExample() {
int* ptr = new int(5);
delete ptr;
ptr = nullptr; // Safe access, ptr is not dangling
}
By setting `ptr` to `nullptr`, any attempt to dereference it afterward will safely lead to a predictable behavior rather than allowing a dangling pointer access.
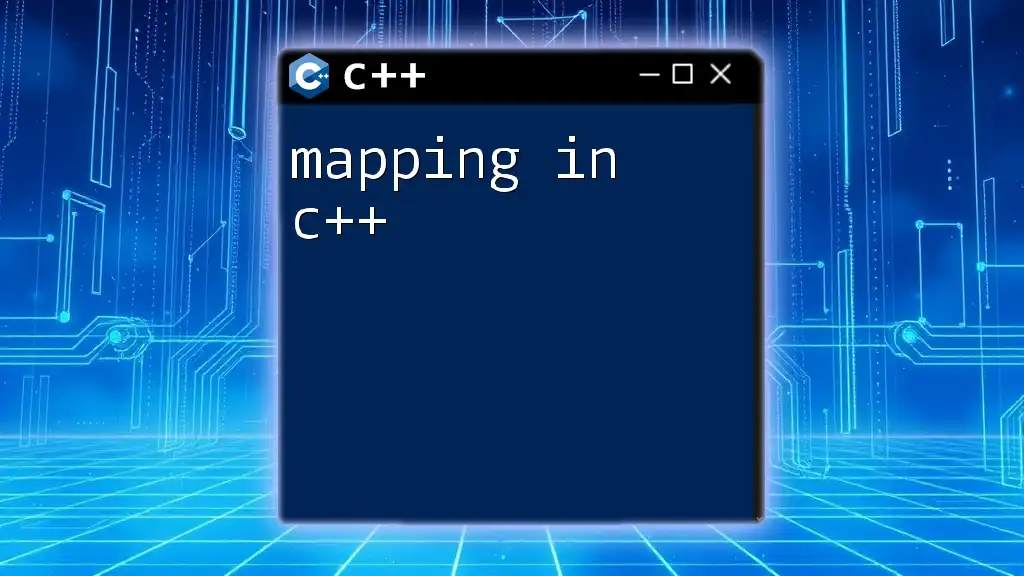
Conclusion
In summary, understanding dangling pointers in C++ is crucial for ensuring robust memory management and enhancing program reliability. By recognizing the common causes, realizing the associated risks, and employing effective preventive techniques, developers can minimize the likelihood of encountering dangling pointers and the issues they cause. Best practices such as utilizing smart pointers, resetting pointers to null, and applying RAII can significantly improve code safety and maintainability. Engaging with these practices can lead to better-designed programs, mitigating risks associated with dangling pointers and other memory-related challenges.
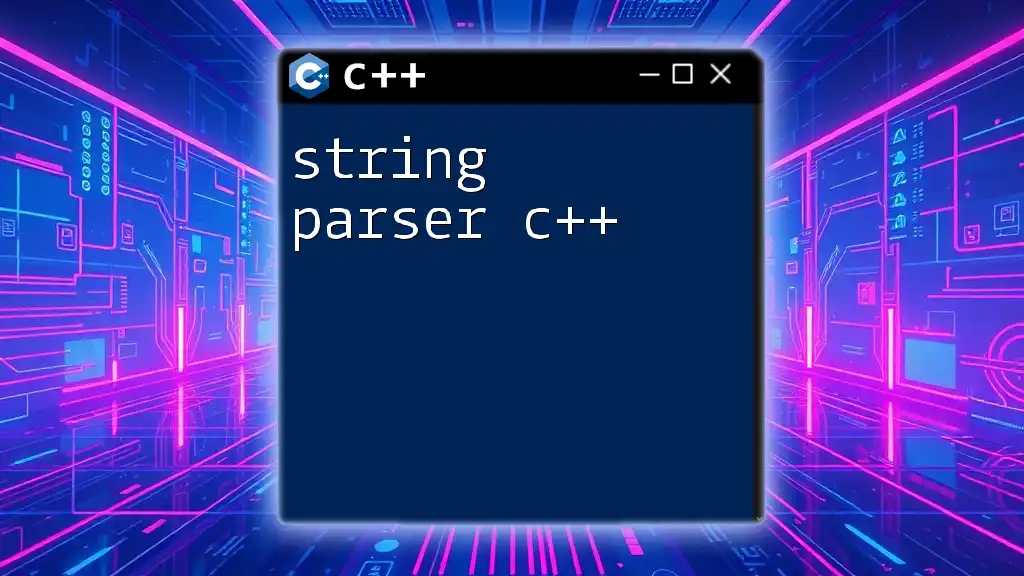
Additional Resources
To further enhance your understanding of dangling pointers and related memory management concepts, consider diving into recommended books and articles that provide deeper insights into C++ programming. Online platforms also offer courses catering to memory management and best practices for effective coding, enabling you to master this critical aspect of C++.
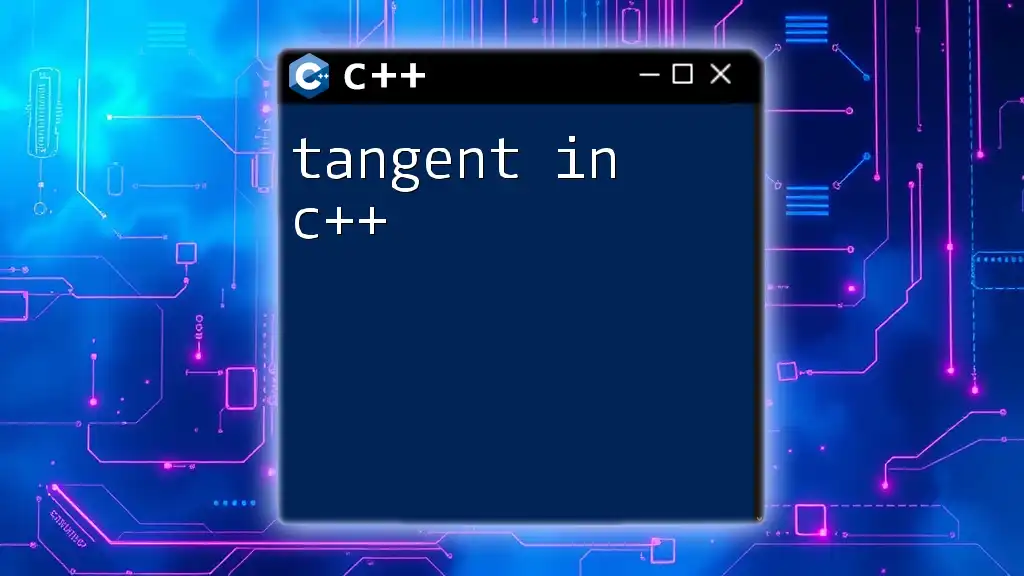
Final Thoughts
Feel free to share your own experiences with dangling pointers in C++, as well as strategies you have found effective in preventing issues related to pointer misuse. Engaging with the community can lead to shared learning and improved coding practices.