The `back_inserter` in C++ is a standard iterator that uses the `push_back` member function to insert elements at the end of a container, such as a vector or a list.
Here's a code snippet showcasing its usage:
#include <iostream>
#include <vector>
#include <algorithm>
#include <iterator>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination;
std::copy(source.begin(), source.end(), std::back_inserter(destination));
for (int num : destination) {
std::cout << num << " ";
}
return 0;
}
What is `back_inserter`?
The `back_inserter` is a powerful utility in C++ that facilitates easy insertion of elements into the back of containers. It essentially transforms the behavior of output operations, allowing developers to dynamically adjust the size of containers like `std::vector`, `std::list`, or other similar data structures.
Benefits of using `back_inserter`
Utilizing `back_inserter` brings several advantages to the table:
-
Dynamic Size Handling: The primary feature of `back_inserter` is its ability to manage the size of a container dynamically. When using `back_inserter`, the container grows automatically to accommodate new elements, eliminating the need for pre-allocation or manual size management.
-
Simplifies Insertion Operations: Instead of writing verbose code to push elements into a container, `back_inserter` allows for a more streamlined and readable code structure, especially when used in conjunction with algorithms from the `<algorithm>` header.
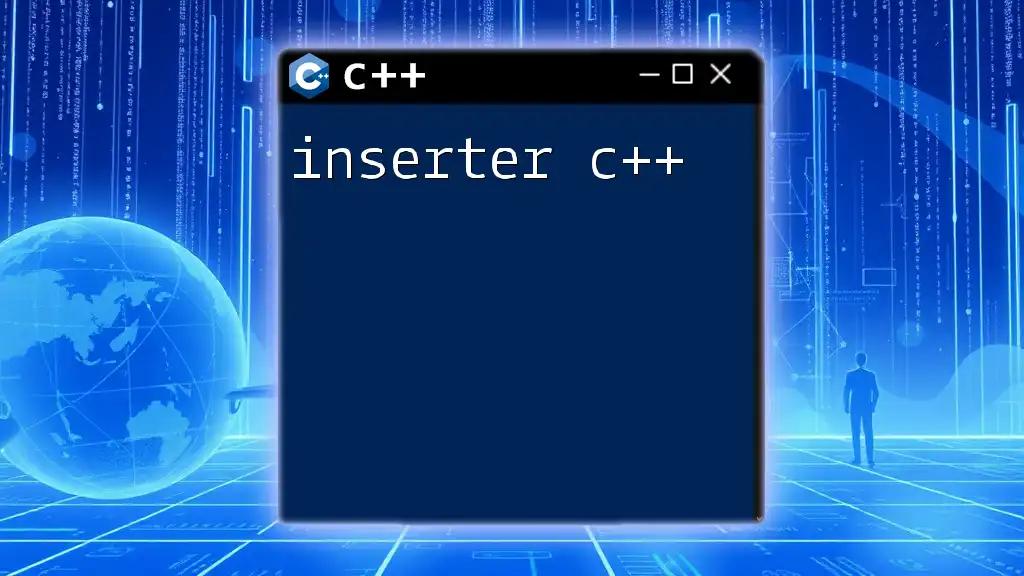
How `back_inserter` Works
Understanding Iterators
Iterators are fundamental components in C++ that provide a way to access elements in a container without exposing the underlying structure. They're often seen as "pointers" to the elements of a container.
- Types of Iterators in C++: C++ provides several types of iterators (input, output, forward, bidirectional, random access), each with varying capabilities. `back_inserter` utilizes output iterators specifically designed for inserting values into containers.
The Role of `back_inserter`
`back_inserter` takes control of where new elements are inserted by wrapping a container and presenting a special type of output iterator. When using this iterator, assignment operations can be performed, which results in the targeted container’s insertion function being invoked, effectively adding elements to the end of the container.

Creating a `back_inserter`
The Basics of `std::back_inserter`
The syntax for `back_inserter` is simple and intuitive. Here is a basic example of its usage:
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> vec;
auto inserter = std::back_inserter(vec);
*inserter = 10; // Inserting into the vector
for (int n : vec) {
std::cout << n << ' ';
}
return 0;
}
Code Explanation
In this example, we begin by including necessary headers, `iostream`, `vector`, and `iterator`. We then define a `std::vector<int>` named `vec`. The `auto inserter = std::back_inserter(vec);` line creates a back inserter associated with `vec`.
The line `*inserter = 10;` effectively pushes the value `10` to the back of `vec`. If you print the vector, it will output `10`, illustrating that the element was successfully inserted.
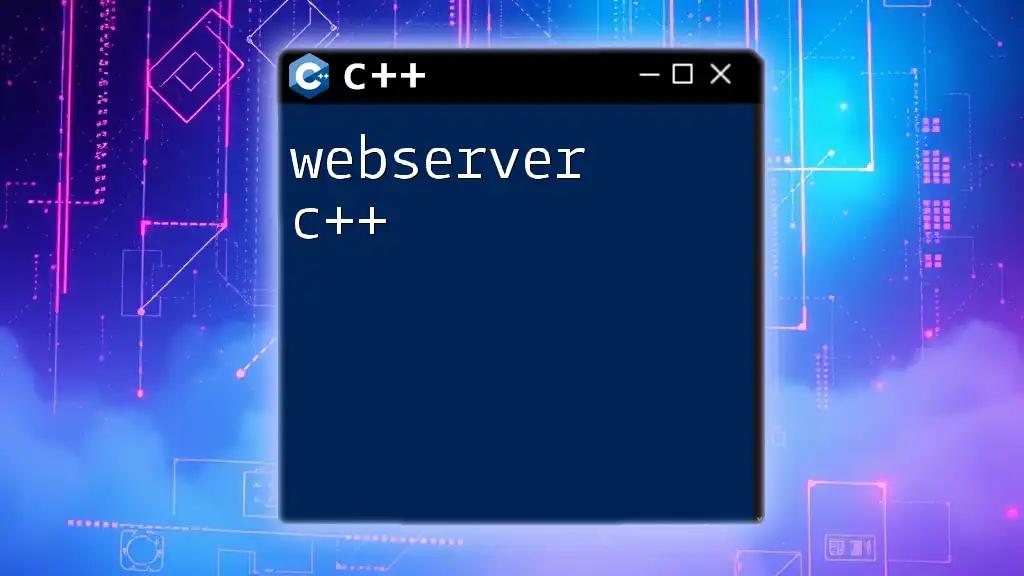
Practical Applications of `back_inserter`
Using `back_inserter` with `std::copy`
`back_inserter` shines when used with algorithms like `std::copy`, which is used to copy elements from one container to another. Here’s a practical example:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> source = {1, 2, 3};
std::vector<int> destination;
std::copy(source.begin(), source.end(), std::back_inserter(destination));
for (int n : destination) {
std::cout << n << ' ';
}
return 0;
}
Example Explanation
The code snippet above initializes a `source` vector containing integers `1, 2, 3`. We create an empty `destination` vector. When we call `std::copy`, it replica every element from `source` into `destination` using `std::back_inserter`. The use of `back_inserter` allows `destination` to automatically resize, so no prior allocation or size management is necessary. The printed output will display `1 2 3`.

Performance Implications
Efficiency of `back_inserter`
When it comes to performance, `back_inserter` can significantly enhance code efficiency. It enables developers to use standard algorithms without worrying about the underlying complexity of resizing the containers. This streamlined approach can lead to cleaner and more efficient code.
Memory Considerations
An important aspect to note is how `back_inserter` handles dynamic memory. By abstracting away manual memory management, it reduces the potential for memory-related errors. However, improper use can still lead to performance overhead if not managed correctly. Always ensure that you are working with appropriate types of containers to maintain efficient memory usage.

Common Mistakes and Troubleshooting
Common Issues When Using `back_inserter`
-
Incorrect Iterator Usage: A frequent error occurs when developers attempt to dereference the back inserter without understanding its purpose. Remember, `back_inserter` is an iterator designed for output.
-
Undefined Behavior Scenario: If you try to assign a value when the associated container is in a state that does not allow insertion (like an uninitialized vector), it will lead to undefined behavior.
Debugging Tips
To mitigate issues, incorporate runtime checks and extensive testing. If you encounter bugs related to `back_inserter`, double-check that the iterator is properly initialized, and be aware of the state of the underlying container.
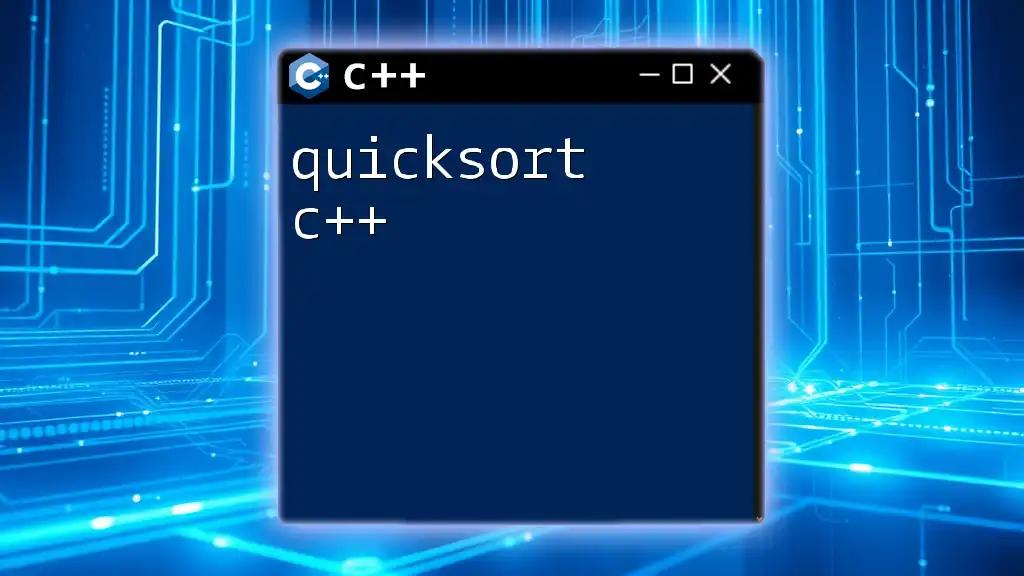
Conclusion
In summary, `back_inserter` is a useful tool that facilitates easier and more efficient manipulation of collections in C++. Its ability to dynamically resize containers and simplify element insertion makes it an invaluable feature in modern C++ programming.
Next Steps for Readers
I encourage readers to implement `back_inserter` in their own projects, enhancing their code’s readability and maintainability. For further learning, consider exploring the C++ standard library documentation and various tutorials available online.

References
- C++ Standard Library Documentation
- Relevant Books and Online Tutorials
- Recommendations for Online C++ Communities for Continued Learning
Call to Action
Now that you know what `back_inserter` in C++ is all about, share your experiences with it. We'd love to hear your tips and tricks! Don’t forget to follow our blog or subscribe to our newsletter for more concise C++ programming insights!