The `to_str` function in C++ is not a standard function; however, you can convert various types to strings using the `std::to_string` function.
Here's a code snippet demonstrating how to use `std::to_string`:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << "The string representation of the number is: " << strNumber << std::endl;
return 0;
}
Understanding `to_str` in C++
What is `to_str`?
`to_str` is a utility function that plays a crucial role in modern C++ programming by allowing developers to convert various data types into their string representations. Its primary purpose is to enhance code usability and keep it clean by providing a straightforward interface for string conversion, reducing the clutter that can stem from multiple conversion methods.
Why Use `to_str`?
The beauty of `to_str` lies in its versatility. This function simplifies the conversion process, making it easier for developers to debug and log their applications. By transforming different data types into strings, you can seamlessly integrate output logs or display messages, thereby enhancing code readability.
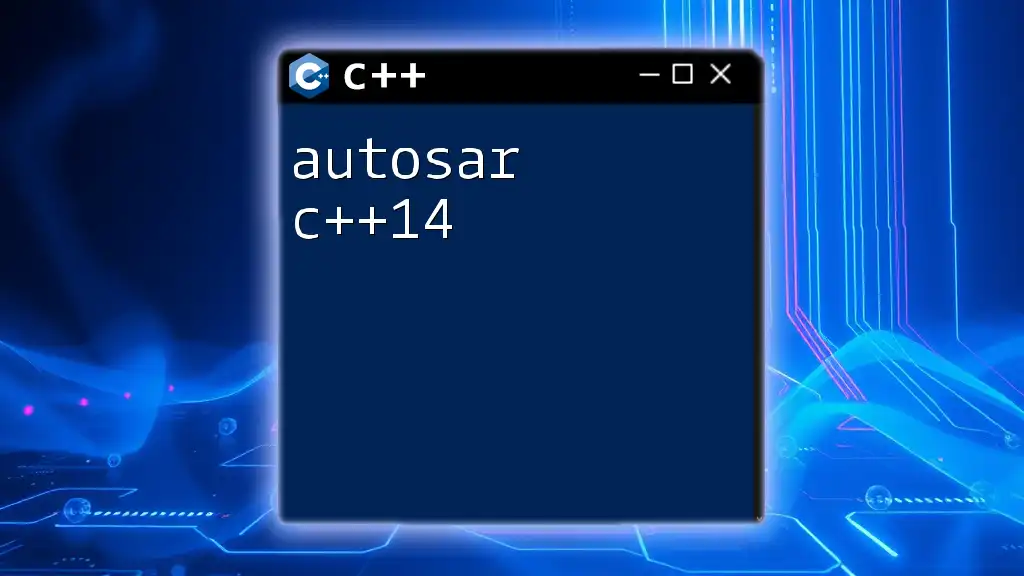
Overview of C++ String Conversions
Common String Conversion Techniques
Before diving into `to_str`, it's essential to be familiar with other string conversion techniques in C++. Among the most common are:
-
Using `std::to_string`: This straightforward function converts fundamental data types like integers and floats into strings. However, it does not work with user-defined types.
-
Using `std::stringstream`: This option offers greater flexibility for custom types and complex conversions. It allows formatted output, making it suitable for various use cases.
-
Using C-style strings: Although less common today, you may encounter `sprintf` or formatted char arrays in legacy code, which can be error-prone and less safe.
Limitations of Standard Methods
While `std::to_string` and `std::stringstream` offer convenient solutions, they come with limitations. For example, `std::to_string` supports only fundamental types, limiting its use when custom types are involved. Additionally, performance matters in environments requiring heavy string manipulations, and the overhead from some of these methods can be significant if used improperly.
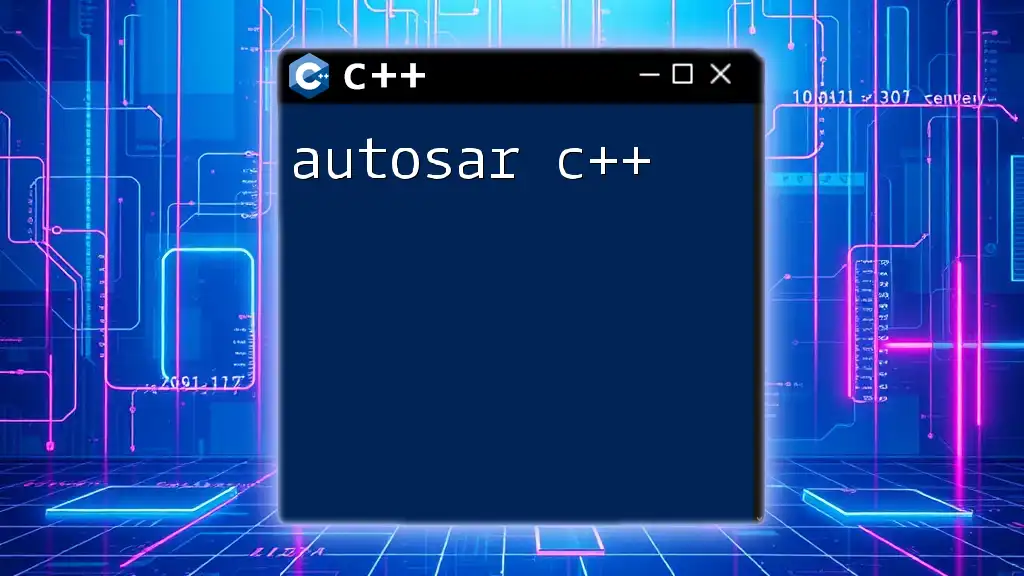
The Benefits of `to_str`
Improved Readability and Maintenance
When you use a utility like `to_str`, your code remains clean and understandable. Consider this example of converting integers and floats:
int num = 42;
std::string strNum = to_str(num);
float pi = 3.14;
std::string strPi = to_str(pi);
By using `to_str`, the intention of the code becomes immediately clear, enabling easier maintenance and faster onboarding of new developers to your team.
Enhanced Functionality
`to_str` supports a variety of data types, including complex user-defined types. Its ability to convert any data type into a string enhances flexibility. Moreover, type safety ensures that developers avoid unnecessary conversions, which can lead to errors down the line.
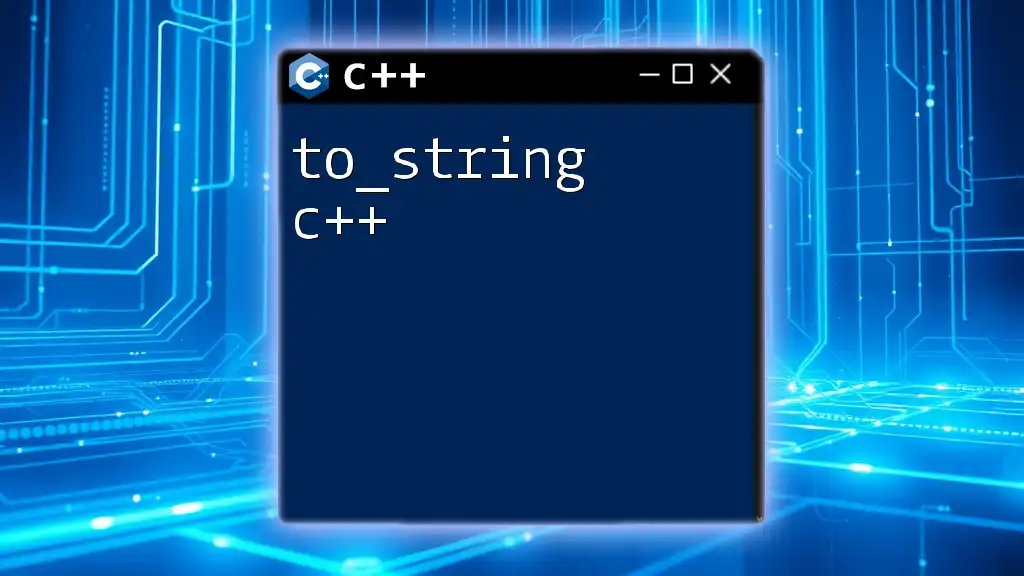
Implementing `to_str` in Your Code
Creating a Simple `to_str` Function
Implementing `to_str` is relatively straightforward. Below is a sample implementation that utilizes `std::ostringstream` for converting data to strings:
#include <sstream>
#include <string>
template<typename T>
std::string to_str(const T& value) {
std::ostringstream oss;
oss << value;
return oss.str();
}
In this function, `std::ostringstream` is used as a stream to facilitate the conversion. It effectively captures the string representation of any provided input type.
Example Use Cases
Using the `to_str` function is simple. Here are a couple of common scenarios:
- Converting Integers:
int num = 42;
std::string strNum = to_str(num);
- Converting Floats:
float pi = 3.14;
std::string strPi = to_str(pi);
Converting Custom Objects
For greater effect, you can also define how `to_str` handles custom objects. This is typically achieved through operator overloading, allowing seamless integration of your classes with the `to_str` function.
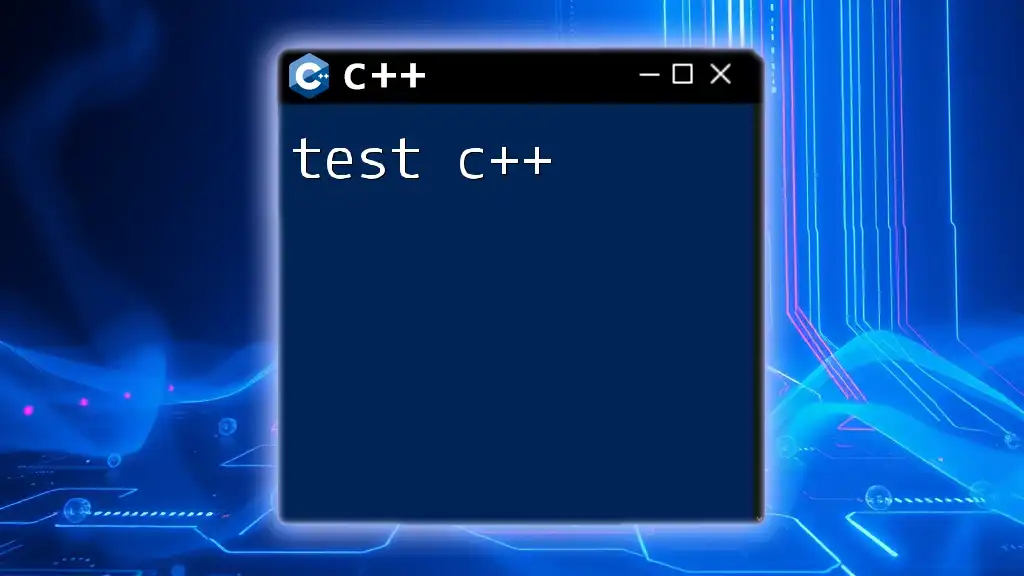
Best Practices for Using `to_str`
Type Safety Considerations
Type safety remains critical when converting data types to strings. Avoid implicit conversions that could lead to unexpected results. Designing interfaces that enforce the expected types improves the reliability of your applications.
Performance Tips
Not all scenarios call for `to_str`. For fundamental conversions, `std::to_string` may be more efficient. Always evaluate the best choice based on performance requirements and code complexity. Optimizing memory management also contributes to higher performance.
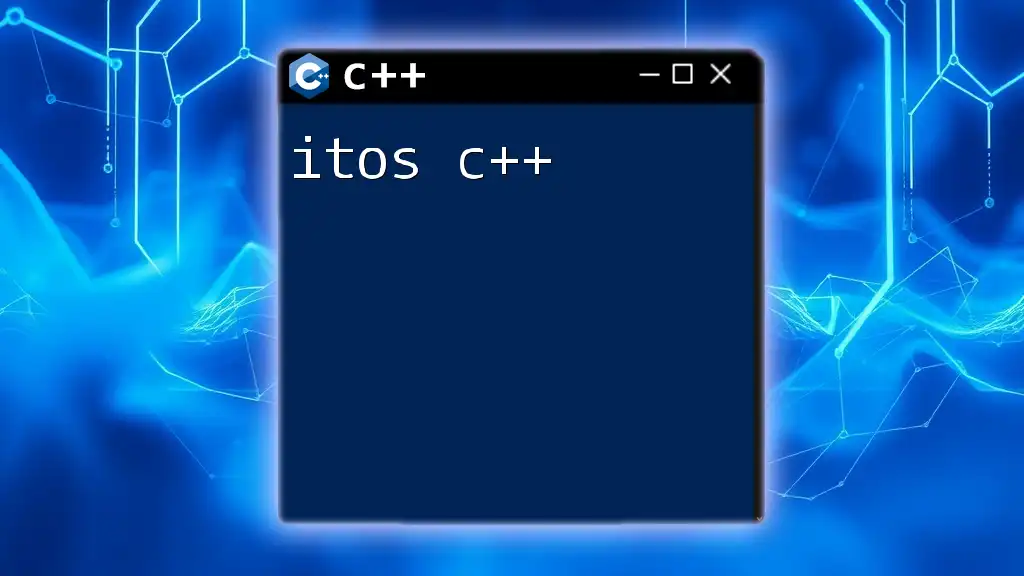
Advanced Usage of `to_str`
Handling Special Cases
It’s essential to handle special cases effectively. Customize the `to_str` function to deal with nullptrs or other edge conditions gracefully. Here’s an example:
template <>
std::string to_str(const char* value) {
return value ? std::string(value) : "null";
}
This specialization ensures that string representations of C-strings account for null pointers, returning a clear "null" message when appropriate.
Extending `to_str` for Custom Types
Your implementation can be enhanced through template specialization. If you have custom types, you can implement specific conversions directly within the relevant class. Consider a simple class example:
class Point {
public:
int x, y;
// Conversion logic
std::string to_str() const {
return "(" + std::to_string(x) + ", " + std::to_string(y) + ")";
}
};
This allows you to maintain type safety while providing a clear conversion path when using your `to_str` function.
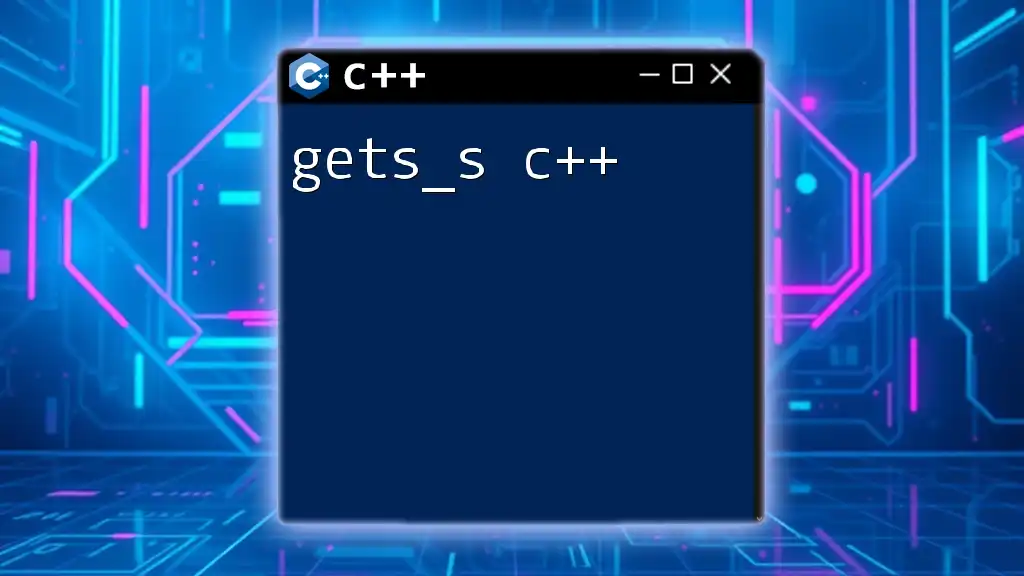
Conclusion
Recap of Key Points
In summary, the `to_str` utility function in C++ serves as an essential tool for converting various data types into strings effectively. It simplifies coding, enhances readability, and promotes best practices regarding type safety and performance. By utilizing `to_str`, developers can ensure clearer, more maintainable codebases.
Future of String Conversions in C++
As C++ continues to evolve with new features and standards, string conversions will likely become even more powerful and flexible. Experimentation with your `to_str` implementation can lead to deeper insights and improvements, encouraging you to explore robust string manipulation techniques.
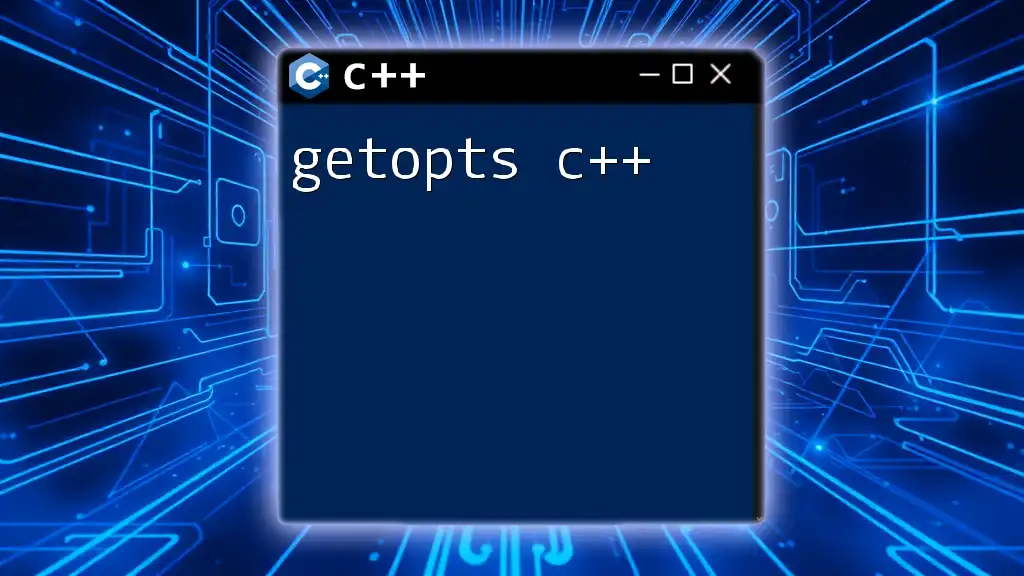
Additional Resources
Recommended Readings
- Official C++ Documentation
- Blogs and Tutorials on Effective C++ String Handling
Further Learning Opportunities
Engage in online courses and coding challenges that focus on string manipulations and conversions, allowing you to solidify your understanding of these vital programming concepts.