A functor in C++ is an object that can be treated as if it were a function, allowing you to define custom behavior by overloading the `operator()`.
Here’s a simple example of a functor in action:
#include <iostream>
class Adder {
public:
Adder(int x) : value(x) {}
int operator()(int y) const {
return value + y;
}
private:
int value;
};
int main() {
Adder addFive(5);
std::cout << addFive(3); // Outputs: 8
return 0;
}
What is a Functor?
In C++, a functor is defined as an object that can be called as if it were a function. This is achieved by overloading the `operator()`. Unlike regular functions or function pointers, functors can maintain state and encapsulate both data and functionality.
Why Use Functors?
One of the primary advantages of using functors is their ability to carry their state, making them quite powerful for operations that require context. They can be passed around and used just like functions, but with the added ability to store data, leading to greater flexibility in programming.
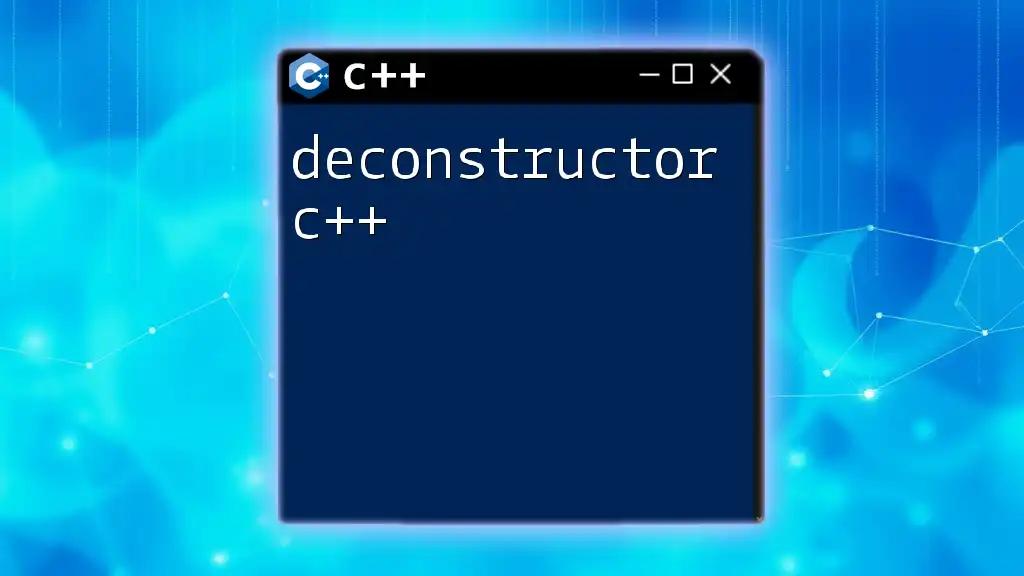
Understanding the Basics
Functor Syntax in C++
The syntax for creating a functor involves defining a class or struct whose `operator()` is overloaded. The signature of this operator can vary based on the required functionality.
Here’s a simple example of a functor:
struct Add {
int operator()(int a, int b) const {
return a + b;
}
};
In this example, the `Add` struct acts as a functor. The `operator()` takes two integers, adds them, and returns their sum. The `const` ensures that the functor does not modify its state when called.
Creating Your First Functor
To demonstrate how to use the `Add` functor, consider the following code snippet, which utilizes the functor to perform addition:
Add add;
int result = add(3, 4); // Calls operator()
Here, we create an instance of `Add`, then call it like a function, passing `3` and `4`. The value of `result` becomes `7`.
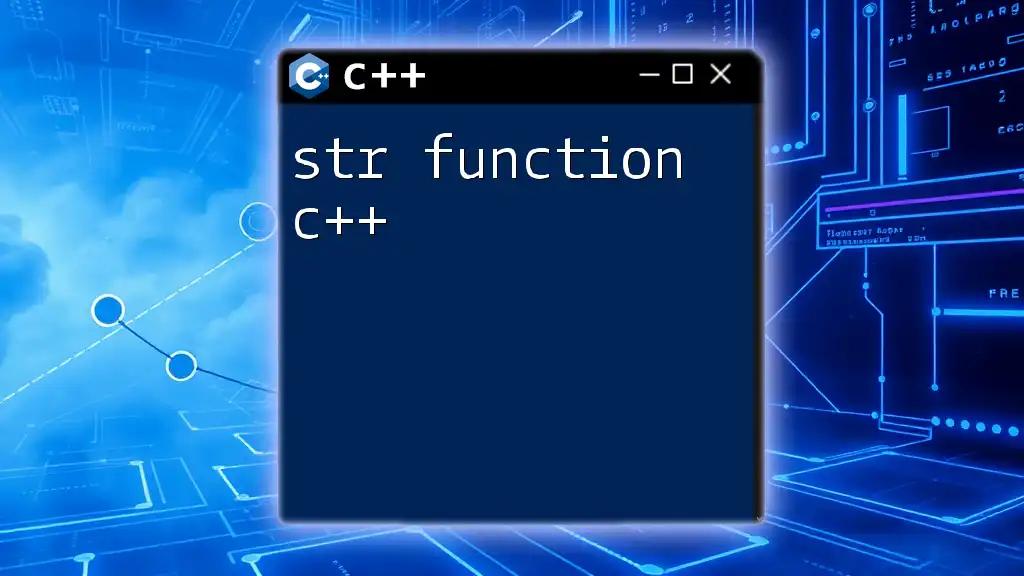
Functors in Action
Using Functors with STL Algorithms
The Standard Template Library (STL) in C++ extensively uses functors, particularly with algorithms like `std::sort`. By defining a functor, you can specify custom sorting behavior.
Consider the following example illustrating how to sort a vector of integers in ascending order using a functor:
struct Ascending {
bool operator()(int a, int b) const {
return a < b;
}
};
std::vector<int> numbers = {5, 1, 4, 2, 3};
std::sort(numbers.begin(), numbers.end(), Ascending());
In this code, the `Ascending` functor defines the sorting criteria. The `std::sort` function calls `operator()`, allowing it to compare elements during sorting.
Functors vs. Lambda Functions
When to Use Functors Over Lambdas
While both functors and lambda functions can achieve similar tasks, there are scenarios where one might be preferrable. Functors are beneficial when you require reusable logic across multiple invocations, while lambdas excel in situations requiring short, concise syntax without the need for separate structure definitions.
Example of Both Approaches
Here's a side-by-side comparison showcasing the use of a functor versus a lambda function for sorting:
// Functor
struct Descending {
bool operator()(int a, int b) const {
return a > b;
}
};
// Lambda
auto descendingLambda = [](int a, int b) { return a > b; };
std::sort(numbers.begin(), numbers.end(), Descending());
std::sort(numbers.begin(), numbers.end(), descendingLambda);
In this case, both approaches effectively sort the vector in descending order. However, the functor `Descending` can be declared and reused, whereas `descendingLambda` is an anonymous function that lives only in the scope where it is defined.
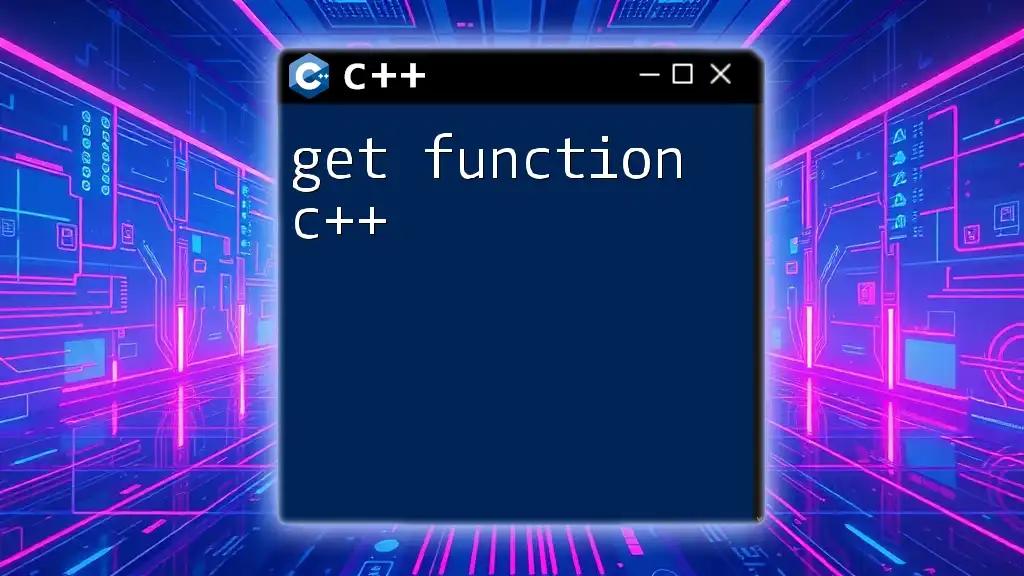
Advanced Functor Techniques
Stateful Functors
A stateful functor is a functor that maintains their state between calls. This characteristic makes them powerful for operations where context matters.
Here’s an example of a stateful functor:
struct Multiplier {
int factor;
Multiplier(int f) : factor(f) {}
int operator()(int value) const {
return value * factor;
}
};
In this example, the `Multiplier` functor takes a `factor` during its construction and uses it in the `operator()`. This allows it to perform multiplication by a specific factor, maintaining its state throughout its usage.
Functors and Templates
Templates can enhance the flexibility of functors. By making a functor a template, you can create a generalized version that works with different data types.
template <typename T>
struct Subtractor {
T operator()(T a, T b) const {
return a - b;
}
};
In this code, the `Subtractor` functor can work with any data type that supports subtraction, allowing for greater reusability and flexibility.
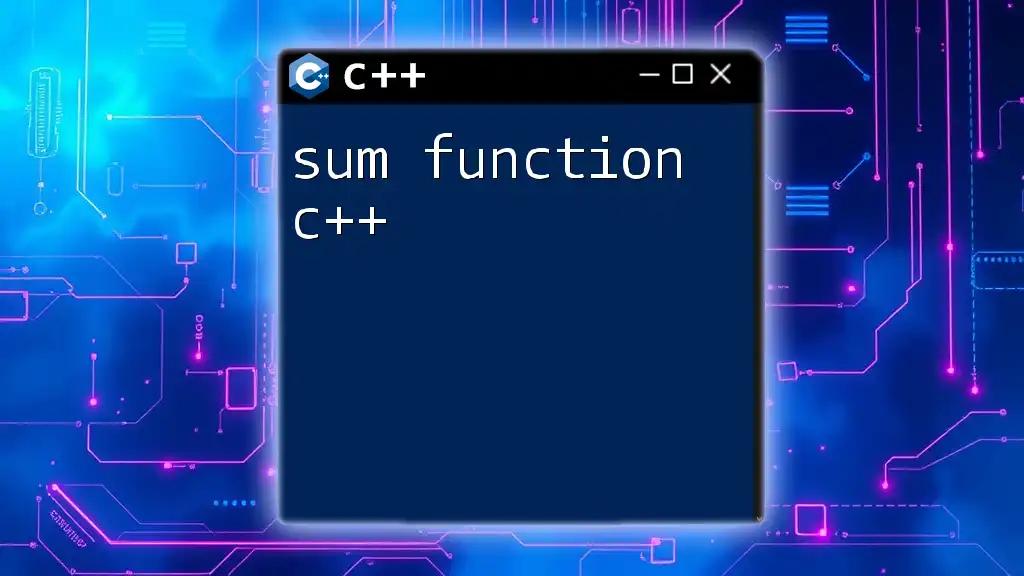
Functors in Real-World Applications
Use Cases for Functors
Functors find applications in various programming paradigms. One common use case is within the STL, particularly for algorithms like searching, sorting, and transformation. They allow developers to define custom behavior while leveraging the efficiency and power of STL algorithms.
Functor Example: Custom Comparators
Creating custom comparators is an excellent practical application of functors. For instance, consider a scenario where you need to sort a list of `Person` objects based on their ages. Here’s how you would implement that using a functor:
struct Person {
std::string name;
int age;
};
struct CompareByAge {
bool operator()(const Person &a, const Person &b) const {
return a.age < b.age;
}
};
In this example, the `CompareByAge` functor allows you to sort a vector of `Person` objects based on their ages. This enhances readability and encapsulates the sorting logic within a structured type.
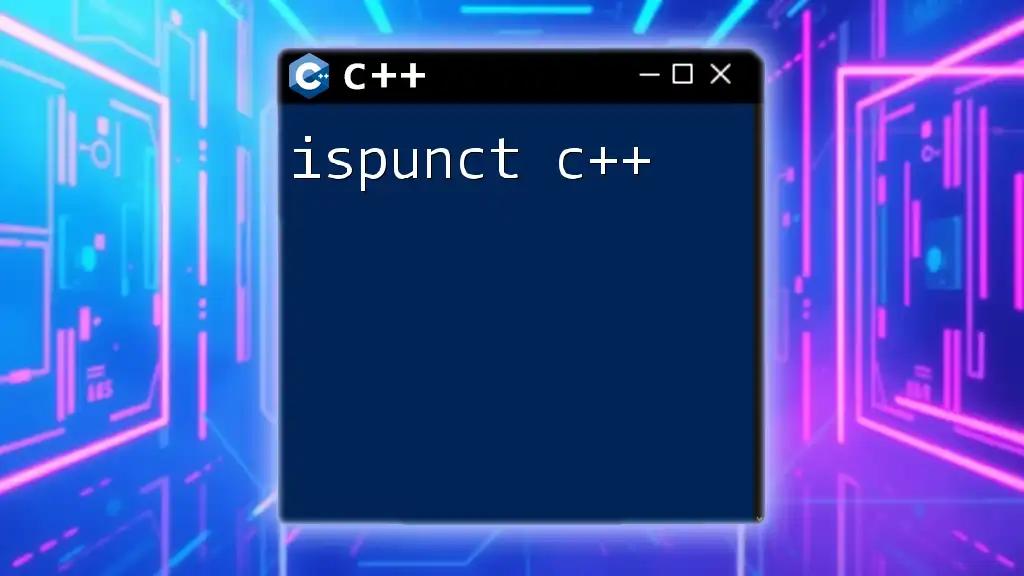
Conclusion
In summary, functors in C++ are a powerful programming construct that combines the functionality of functions with the flexibility of objects. They allow for encapsulation, state management, and integration with the STL, providing developers with a rich toolset for building efficient and expressive algorithms. By experimenting with functors, you’ll deepen your understanding of C++ and enhance your coding practices. Engage with these concepts, explore their usages, and see how functors can elevate your programming projects!