The `type` function in C++ is used to retrieve the type associated with a given expression at compile time, enabling type introspection through the `decltype` keyword.
Here’s a simple example:
#include <iostream>
#include <typeinfo>
int main() {
auto myVar = 10; // An integer variable
std::cout << "The type of myVar is: " << typeid(myVar).name() << std::endl;
return 0;
}
What is the `type` Function?
The `type` function in C++ serves as a powerful tool for type inference, helping developers understand and manipulate data types effectively. It allows you to deduce types at compile time, making your code cleaner and reducing the likelihood of type-related errors. While not a dedicated function in the C++ Standard Library, it refers to the concept of type deduction and commonly involves the use of features like `decltype` and `typeid`.
When leveraging the `type` function, it is crucial to differentiate it from `decltype`, which provides the type of an expression, and `typeid`, which retrieves runtime type information. Understanding how these elements interact will enhance your ability to work with templates and generic programming.
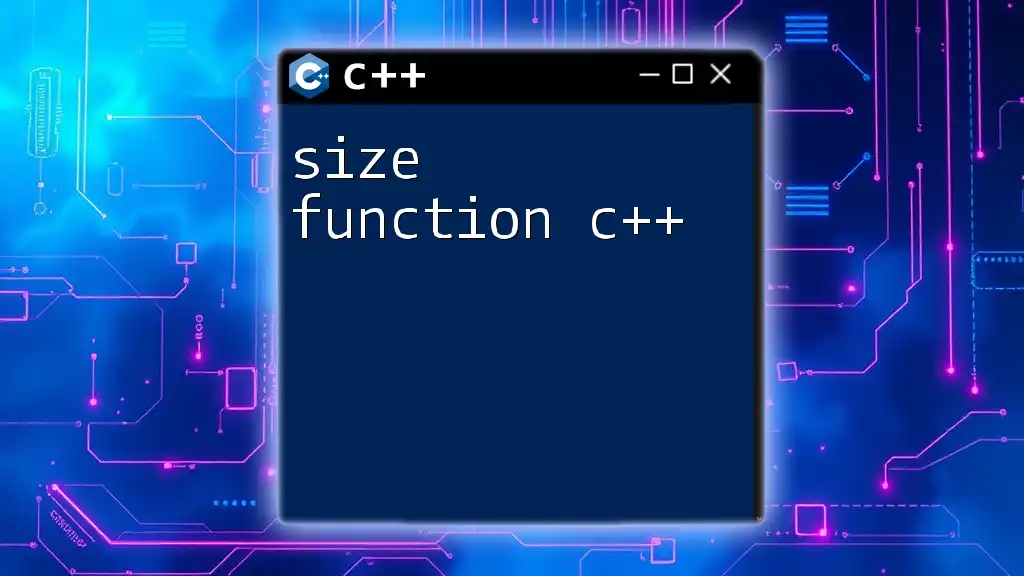
Syntax of the `type` Function
Basic Syntax
The syntax often appears in the context of the language's type deduction features. While there's no explicit `type` function, the way to utilize type inference typically looks like this:
type(expression);
While this is illustrative, what you often use are keywords and structures in C++, such as `auto` and `decltype`.
Parameters
Parameters passed to the `type` function can be any valid expression, such as variables, literals, function calls, or even more complex expressions. Understanding how these parameters translate into types is key for mastering type inference.
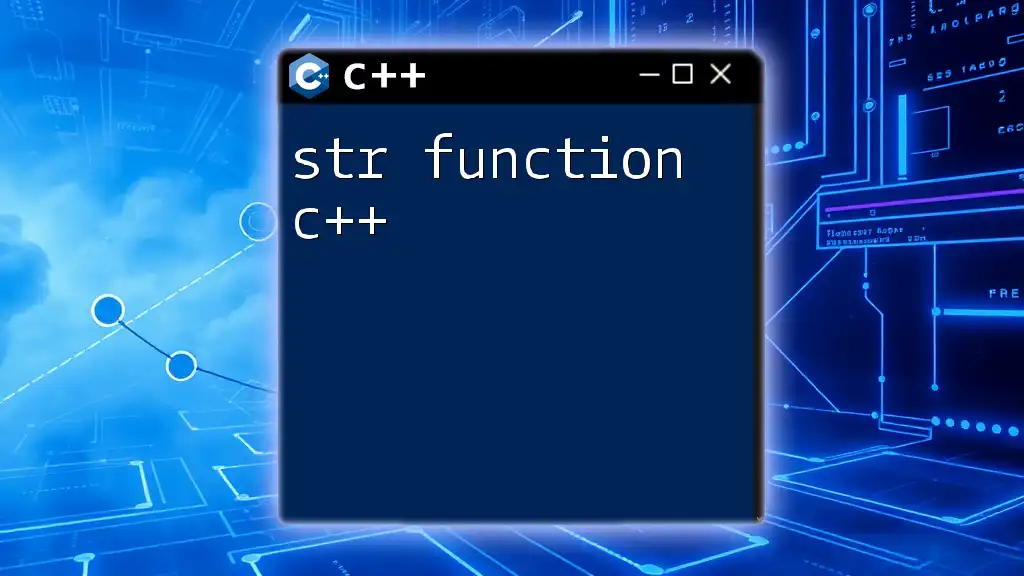
Using `type` in C++: Practical Applications
Type Inference
Type inference in C++ allows the compiler to automatically deduce the type of a variable from its initializer. Using the `auto` keyword is a common practice that encapsulates this concept. For example:
auto myVar = 10; // myVar is deduced as int
In the above case, the compiler automatically determines that `myVar` is of type `int` due to its initializer. This drastically reduces code clutter and enhances readability.
Understanding Return Types
Determining return types is another significant application of the `type` function concept. This often comes into play when working with templates and generic functions. For example:
template<typename T>
auto functionName(T arg) -> decltype(arg) {
return arg; // The return type is automatically deduced
}
In this template, the return type is deduced from the type of `arg`, making the function flexible and reusable across various data types.
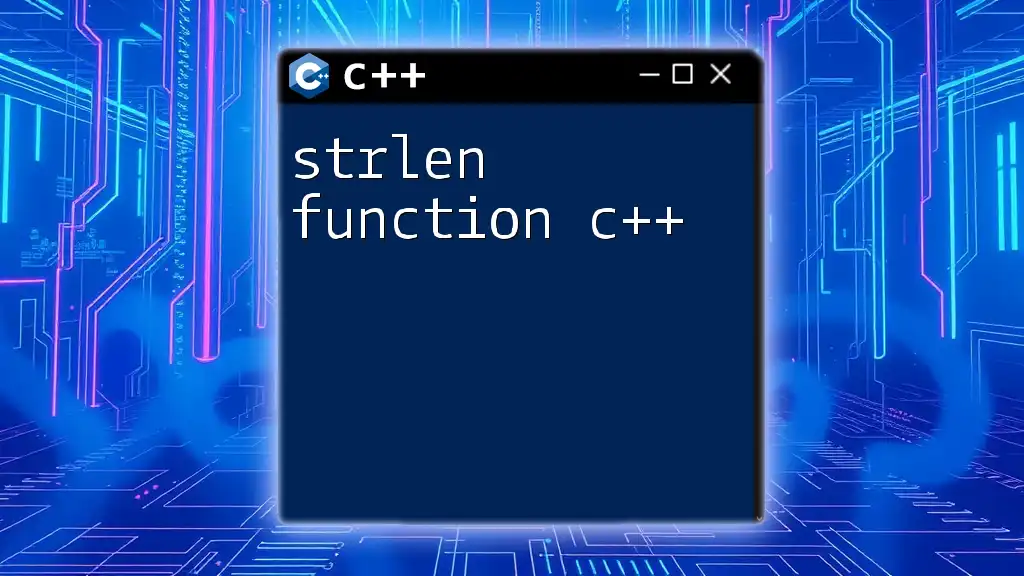
Why Use the `type` Function?
Advantages of `type` Over Manual Definitions
Utilizing the `type` function through type inference offers several advantages:
- Simplification of Code: By eliminating the need for explicit type definitions, your code becomes cleaner and more manageable.
- Reduction of Type-related Errors: The compiler's ability to deduce types helps prevent common pitfalls associated with manual type assignments.
- Encouragement of Code Reuse: Generic programming becomes easier, as you can create more generalized and flexible functions.
Use Cases
Understanding types through the `type` function is particularly beneficial in real-world applications. Consider scenarios such as:
- Generic Programming: Templates in C++ allow you to write code that works with any data type, where understanding inferred types becomes crucial.
- Template Specialization Considerations: When specializing templates for certain types, knowing how types are deduced can help optimize function performance.
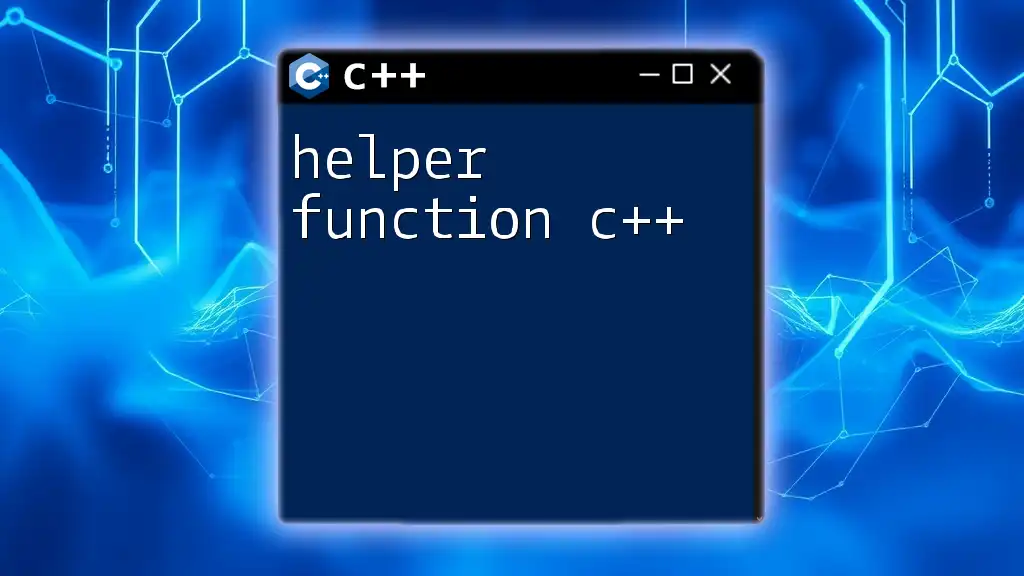
Common Pitfalls and Misunderstandings
Misinterpreting Type Information
When using the `type` function, developers might misconstrue deduced types. An example is confusing function pointer types with their target types. For instance:
int function(int) { return 0; }
auto funcPtr = function; // funcPtr is interpreted as int
Here's where extra care is necessary. Avoid making assumptions about deduced types, as they can lead to bugs that are difficult to trace.
Performance Considerations
While type inference generally improves code readability and maintenance, be mindful of its potential performance implications, especially in cases involving complex types or excessively deep templates. Sometimes denoting exact types can lead to more straightforward, performant code.
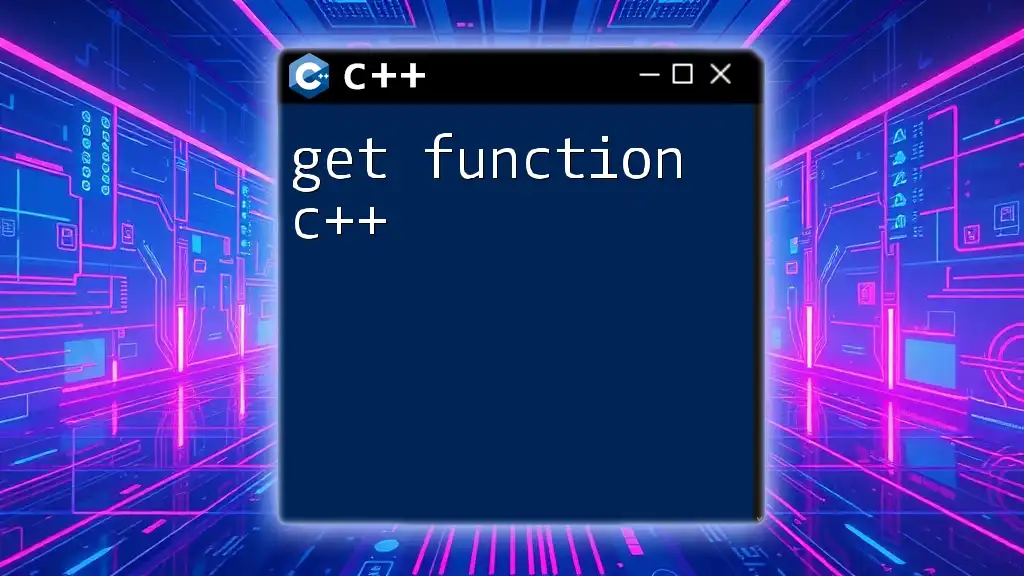
Code Snippets to Illustrate the `type` Function
Practical Examples
Example 1: Using `type` in templates
Understanding how to use the `type` function can simplify complex template definitions:
template<typename T>
void printType(T arg) {
std::cout << "Type: " << typeid(arg).name() << std::endl;
}
This template function prints the type of the passed argument, showcasing the use of `typeid` to retrieve type information.
Example 2: Working with pointers and references
Understanding how `type` relates to pointers can enhance your coding practices:
int x = 10;
int* ptr = &x;
auto typePtr = typeid(ptr).name(); // Retrieves the type of the pointer
In this case, `typePtr` will yield the name of the pointer type.
Advanced Examples
Example 3: Using `type` with lambda functions
As lambdas can have complex and deduced types, the ability to retrieve those types is notably useful:
auto lambda = [](int a) { return a * 2; };
auto lambdaType = typeid(lambda).name(); // Retrieve the type of the lambda function
This snippet illustrates how you can capture and utilize the type information of lambda expressions, which can otherwise be challenging to manage.
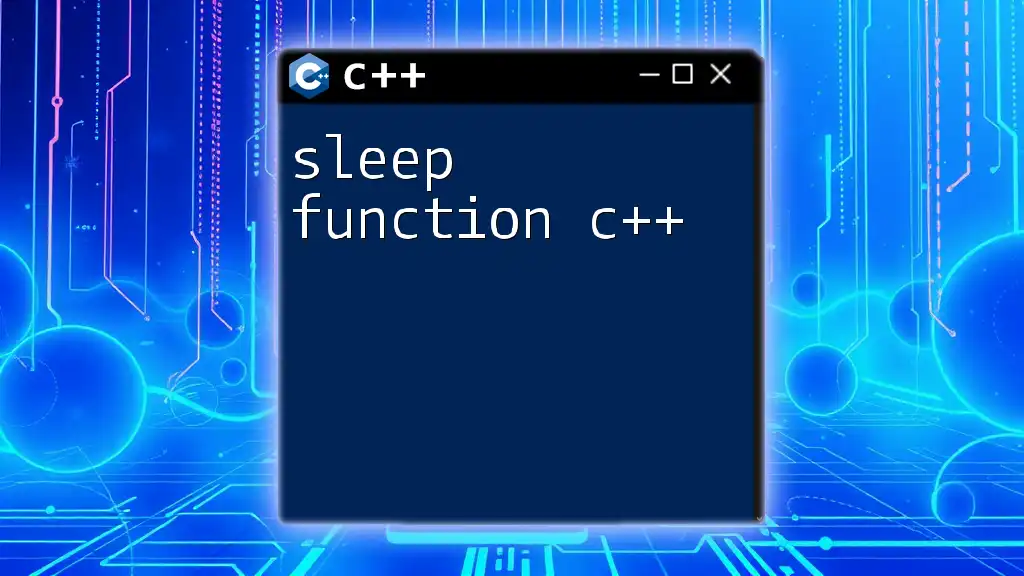
Conclusion
Mastering the `type` function in C++ is a vital skill for any developer. From leveraging type inference to enhancing the readability and maintainability of your code, understanding how to utilize types efficiently will empower you to write better, more robust C++ programs. The journey doesn't end here; continuous practice and exploration of C++'s features will expand your proficiency in this versatile language.
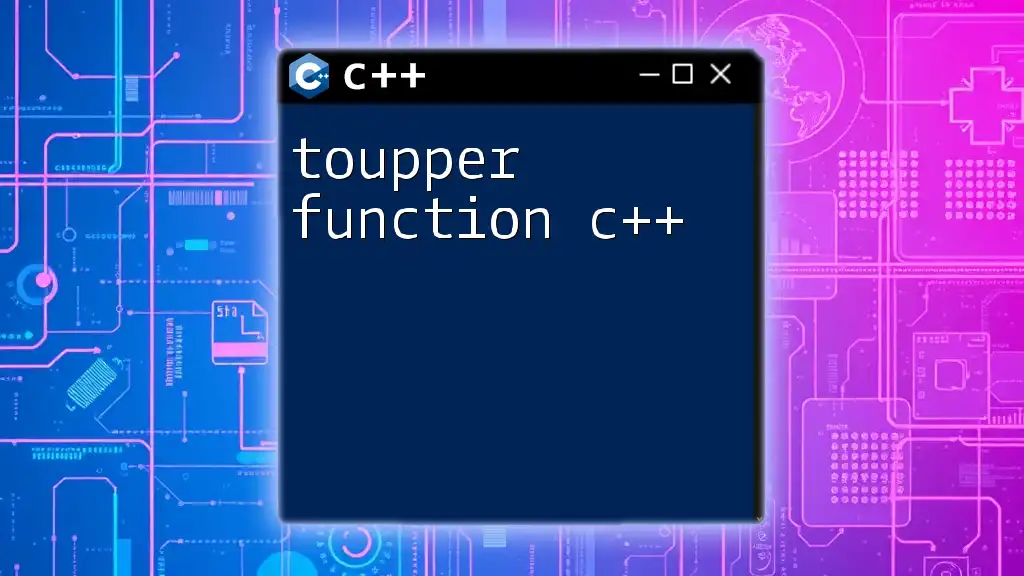
Additional Resources
To further enhance your understanding of the `type function C++, consider exploring C++ documentation, engaging with community forums, and pursuing recommended books or online courses to complement your learning. Embrace the challenges and keep coding!