The `toupper` function in C++ is used to convert a given character to its uppercase equivalent, if applicable.
#include <iostream>
#include <cctype> // For toupper function
int main() {
char lower = 'a';
char upper = toupper(lower);
std::cout << upper << std::endl; // Outputs: A
return 0;
}
What is the toupper Function?
The toupper function in C++ is a standard library function designed to convert lowercase alphabetic characters to their uppercase equivalents. This function is particularly useful in scenarios where case sensitivity may impact the logic of your program, such as when comparing strings or processing user input. The toupper function is defined in the `<cctype>` header file, which means you'll need to include this header at the beginning of your program to use it.
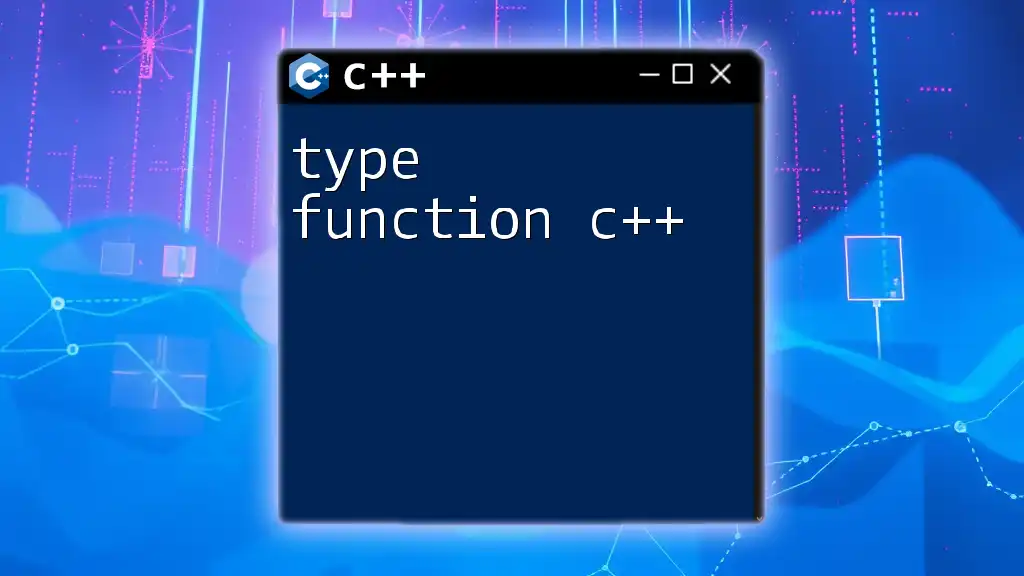
Syntax of the toupper Function
The syntax for the toupper function is straightforward:
int toupper(int ch);
- Parameter: This function takes a single argument, which is typically an integer representing the ASCII value of the character you want to convert.
- Return Type: The function returns an integer, which represents the ASCII value of the converted uppercase character. If the character passed is already uppercase or not an alphabetic character, it returns the character unchanged.
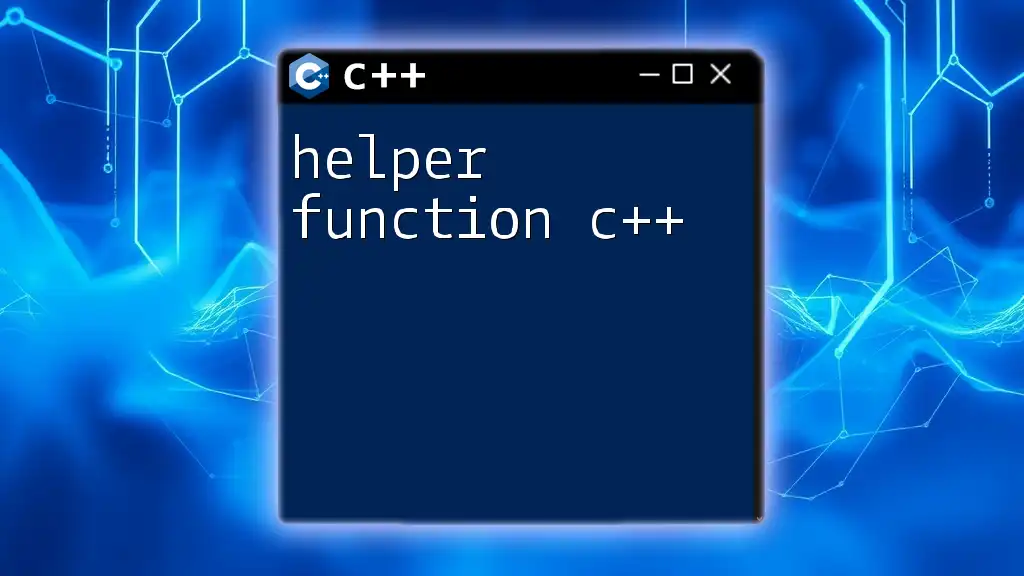
How to Use the toupper Function
Basic Usage
Using the toupper function is simple, even for those new to programming. Here’s a basic example that demonstrates how to convert a single lowercase character to uppercase.
#include <iostream>
#include <cctype>
int main() {
char lowercase = 'a';
char uppercase = toupper(lowercase);
std::cout << uppercase; // Output: A
return 0;
}
In this example, the character 'a' is passed to the toupper function, which converts it to 'A'.
Processing Strings
When working with strings, you may want to convert all characters within a string to uppercase. This can be achieved using a loop, iterating through each character of the string and applying the toupper function.
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string str = "hello world";
for (char& c : str) {
c = toupper(c);
}
std::cout << str; // Output: HELLO WORLD
return 0;
}
In this example, each character in the string "hello world" is converted to uppercase, resulting in "HELLO WORLD".
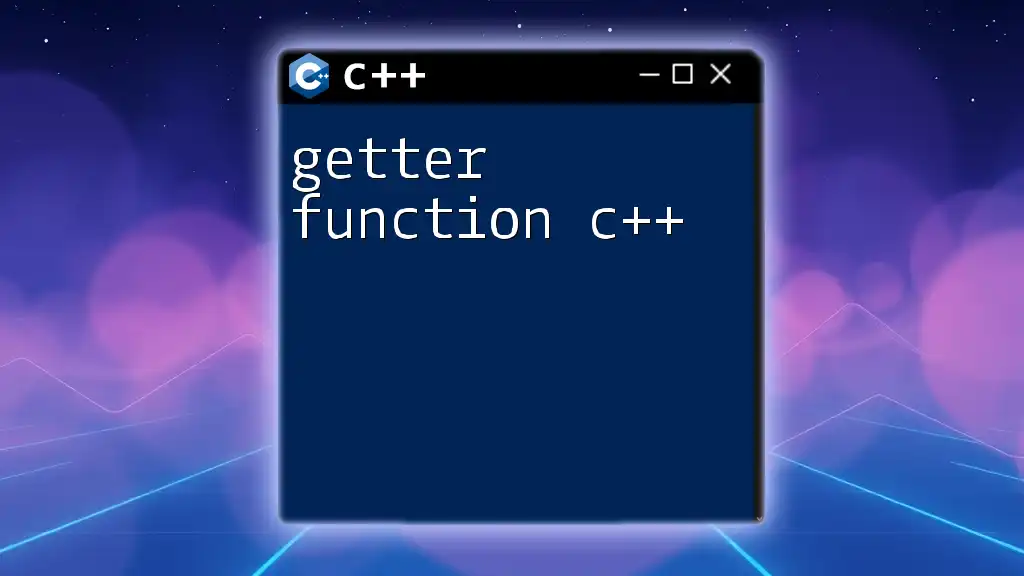
Behavior of the toupper Function
Character Conversion Rules
The toupper function has specific rules regarding the conversion of characters:
- Alphabetic Characters: The function converts lowercase letters ('a' to 'z') to their uppercase counterparts ('A' to 'Z').
- Preservation of Uppercase Characters: If the character passed is already uppercase (e.g., 'A'), or if it's a non-alphabetical character (e.g., '1', '#', or ' '), the toupper function returns the character unchanged.
Here's an example demonstrating this behavior:
char lower = 'b';
char upper = toupper(lower); // Output: B
char unchanged = '2';
std::cout << toupper(unchanged); // Output: 2
Examples of Multiple Use Cases
The toupper function is versatile and can be applied in various contexts. Below are a few scenarios:
- Single Character: Converting individual characters is direct and straightforward.
- Special Characters: Any non-alphabetic character remains unaffected by the function. For example:
char specialChar = '#';
std::cout << toupper(specialChar); // Output: #
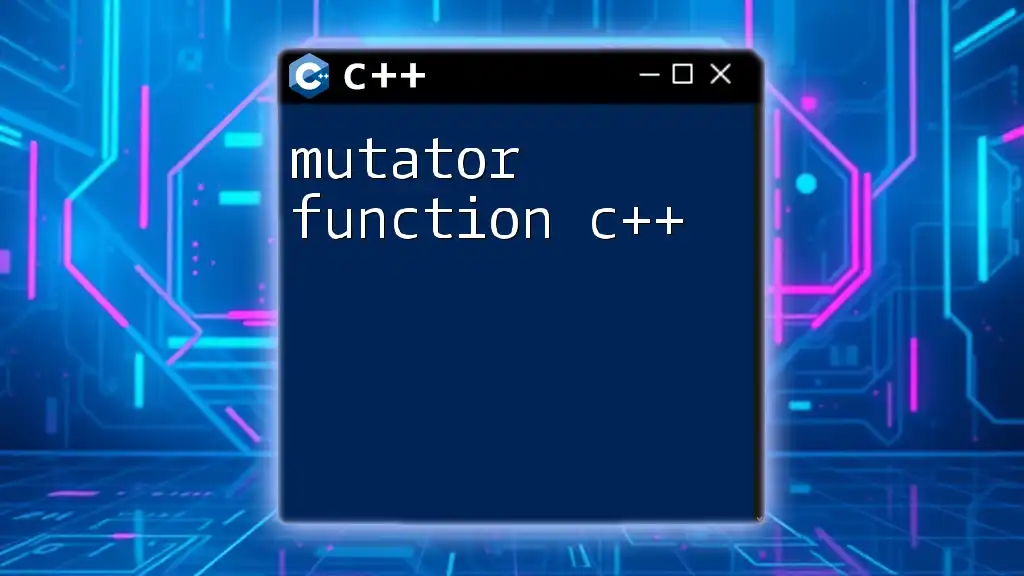
Common Pitfalls and Best Practices
Invalid Input Handling
When using the toupper function, it's essential to be aware of potential invalid inputs. While the function is designed to handle characters within the ASCII range, it's prudent to ensure that the inputs are indeed valid characters before conversion. A good practice is to include checks to filter out invalid types before attempting conversion.
Performance Considerations
While the toupper function is efficient for small datasets, performance can become a concern when manipulating larger strings or data collections. To optimize performance, consider minimizing repeated calls to toupper in loops, possibly utilizing lookup tables or caching results when processing large datasets.
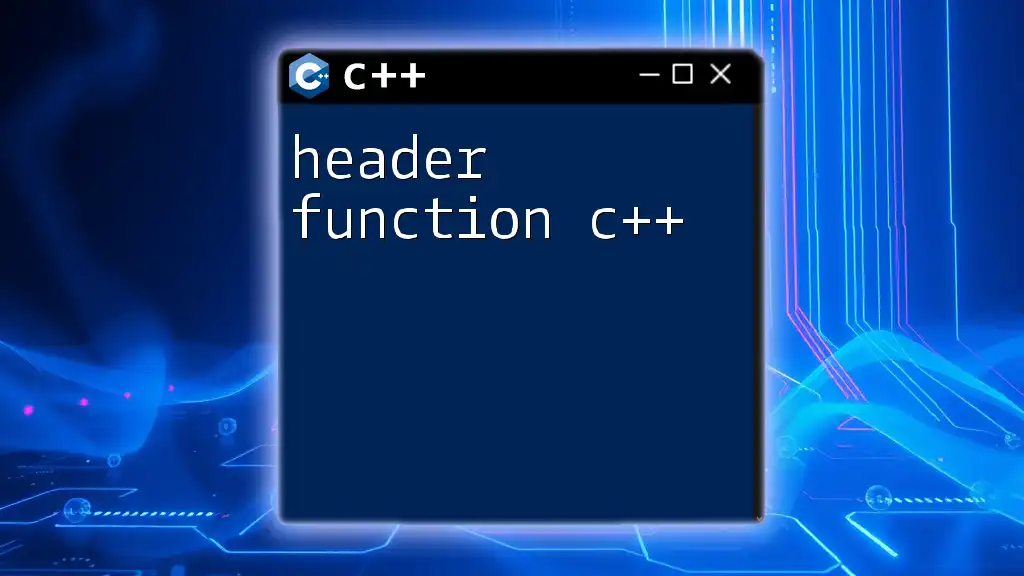
Alternatives to toupper in C++
Using Other Libraries
If you need more flexibility or additional functionality, consider using C++ libraries like `<algorithm>` or `<locale>`. For instance, you can leverage `std::transform` to convert entire strings to uppercase in a more idiomatic way:
#include <algorithm>
#include <iostream>
#include <string>
int main() {
std::string s = "hello world";
std::transform(s.begin(), s.end(), s.begin(), ::toupper);
std::cout << s; // Output: HELLO WORLD
return 0;
}
This approach is often more elegant and can lead to cleaner code, especially when performing multiple transformations.
Manual Conversion
In some cases, you might want to create a custom function to handle more complex cases or to apply additional logic while converting characters to uppercase. This custom implementation allows for detailed control over character processing.
For example:
char myToUpper(char c) {
if (c >= 'a' && c <= 'z') {
return c - 'a' + 'A';
}
return c; // Return unchanged
}
This custom function explicitly checks if a character is lowercase and converts it accordingly.
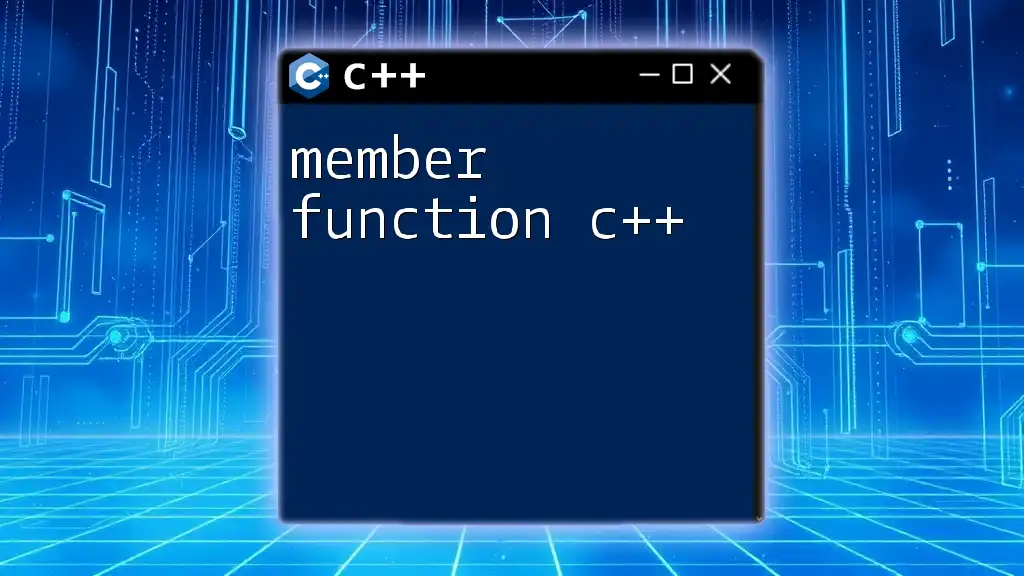
Conclusion
In this comprehensive guide on the toupper function c++, we explored the function's purpose, usage syntax, behaviors, best practices, and alternatives. Mastering the toupper function is essential for effective character manipulation in C++. By understanding its functionality and applying the concepts discussed, you'll enhance your programming skills and manage character cases efficiently. Now, I encourage you to practice using the toupper function in your projects, and continue exploring additional character manipulation functions to further sharpen your C++ programming expertise.
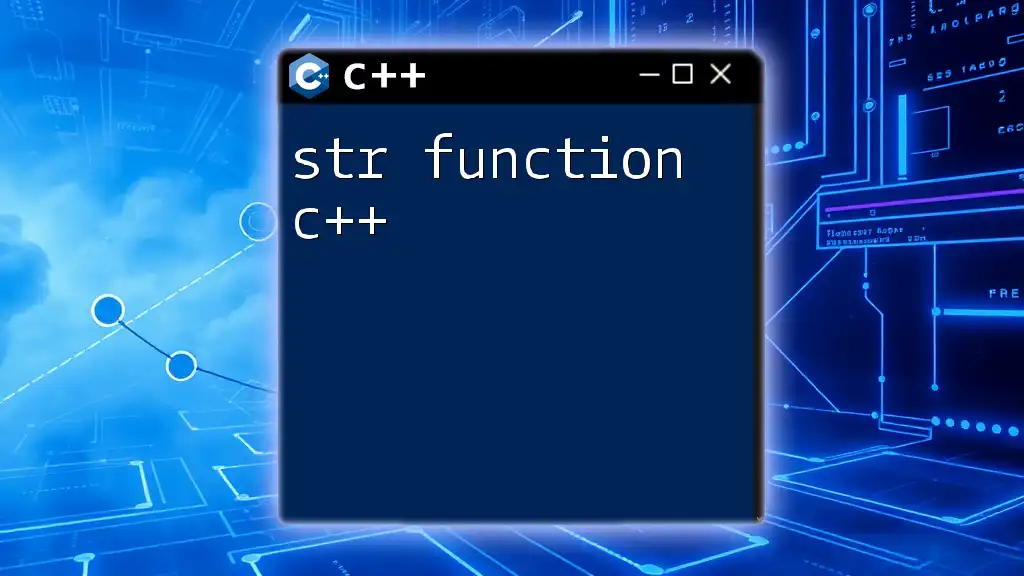
Additional Resources
For further learning, consider exploring the official C++ documentation for toupper alongside recommended programming books and online courses that delve deeper into mastering C++. Engaging with forums and communities can also provide invaluable insights and assistance as you progress on your learning journey.