C++ functional programming allows you to use functions as first-class citizens, enabling techniques like higher-order functions, lambda expressions, and function objects to create more modular and reusable code.
#include <iostream>
#include <algorithm>
#include <vector>
#include <functional>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using std::for_each to apply a lambda function
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n * n << " "; // Print the square of each number
});
return 0;
}
Introduction to C++ Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. It emphasizes declarative programming rather than imperative programming, where the focus is on what to solve rather than how to solve it.
Unlike procedural programming, which organizes code into functions and procedures, functional programming focuses on using functions as the primary building blocks. This can lead to cleaner and more maintainable code, as well as easier debugging and testing.
Why Use C++ Functional Programming?
Using C++ functional programming offers numerous benefits. It can lead to improved code readability, since functional-style code often expresses its intent more clearly. Additionally, because functional programming emphasizes immutability and pure functions (functions with no side effects), it can help prevent bugs that stem from variable state changes.
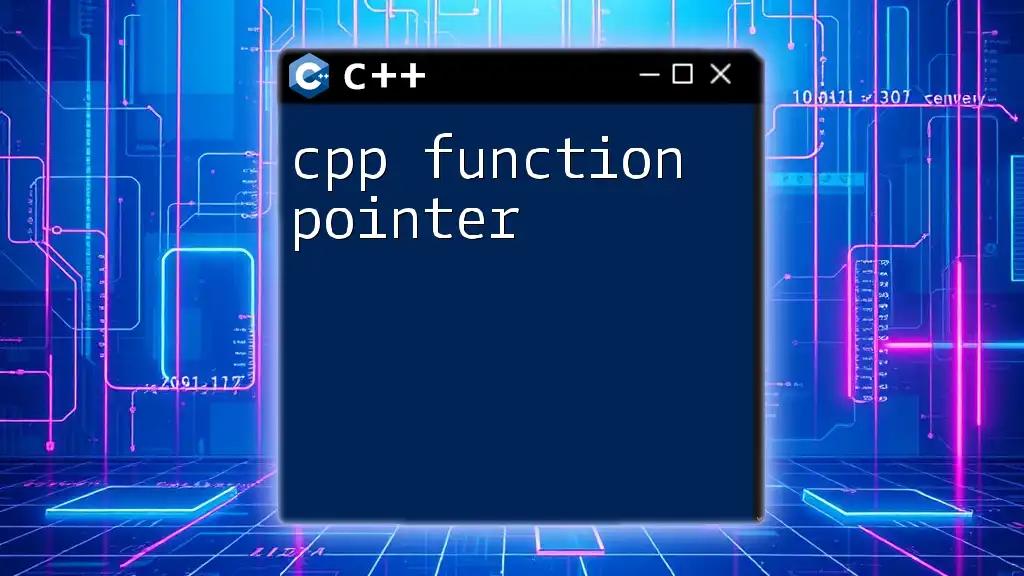
Understanding C++ Standard Library Functional
Overview of `<functional>` Header
The `<functional>` header in C++ is a powerful feature that enables functional programming techniques by providing various function objects, binders, and utilities that support higher-order functions. This header is part of the C++ Standard Library, ensuring compatibility across different C++ compilers.
Key Components of `<functional>`
Function Objects
Function objects, or functors, are objects that can be called as if they were a function. They are a core part of C++ functional programming as they allow for the encapsulation of a function's behavior. A functor can hold state and thus can behave like a function while also having the ability to maintain its state.
For example:
struct Multiply {
int operator()(int a, int b) const {
return a * b;
}
};
Multiply multiply;
int result = multiply(4, 5); // result is 20
Standard Function Wrapper (`std::function`)
`std::function` is a class template that acts as a general-purpose polymorphic function wrapper. It can store any callable target—lambda expressions, bind expressions, or function pointers—allowing for greater flexibility in function handling.
Here’s a simple example demonstrating `std::function`:
#include <functional>
#include <iostream>
void add(int x, int y) {
std::cout << "Sum: " << x + y << std::endl;
}
int main() {
std::function<void(int, int)> func = add;
func(10, 20);
return 0;
}
Bind Functionality (`std::bind`)
The `std::bind` function is instrumental in creating function objects with some of the parameters pre-set. This allows for greater flexibility in passing functions to algorithms.
Here’s how it works:
#include <iostream>
#include <functional>
void show(int a, int b) {
std::cout << "a: " << a << ", b: " << b << std::endl;
}
int main() {
auto boundShow = std::bind(show, 10, std::placeholders::_1);
boundShow(20);
return 0;
}
In this example, `boundShow` is effectively a new function that only requires the second parameter when called.
The Role of Lambdas in C++ Functional
What are Lambda Expressions?
Lambda expressions are a convenient way to create unnamed function objects directly in the code. They allow developers to write inline functions without needing to define a separate function each time. The syntax of a lambda is straightforward:
[capture](parameters) -> return_type { body }
Lambdas are particularly useful in algorithms that require function pointers, making them invaluable for functional programming.
Using Lambdas with STL Algorithms
Lambdas integrate seamlessly with the Standard Template Library (STL), allowing for more readable and concise code. Here is an example of using a lambda with the `std::for_each` algorithm:
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
std::for_each(nums.begin(), nums.end(), [](int n) {
std::cout << n * 2 << " ";
});
return 0;
}
In this case, the lambda takes each element from the `nums` vector, doubles it, and prints it.
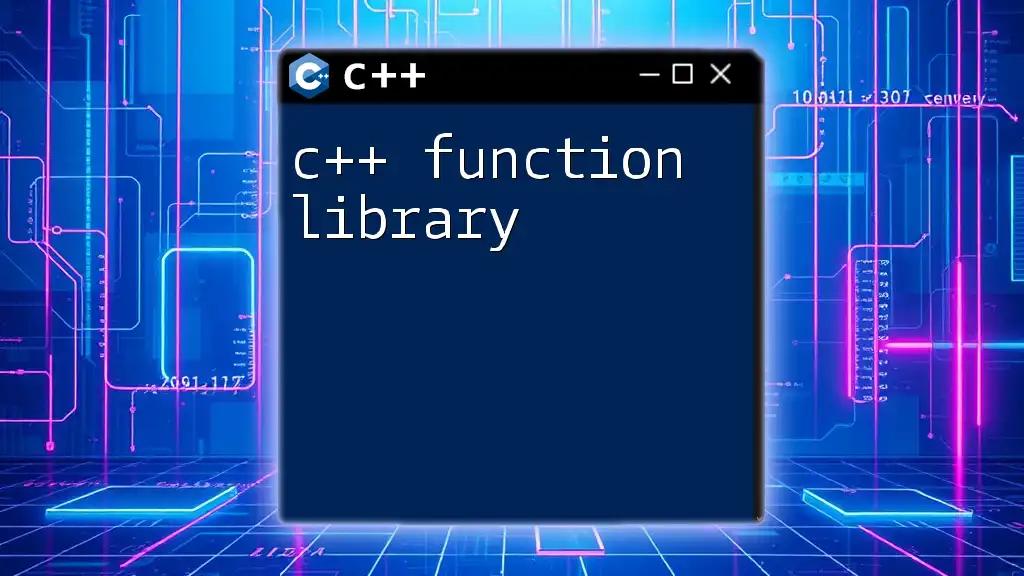
Functional Programming Features in C++
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return them as results. They allow for more abstract and reusable code. This is a defining feature of functional programming.
For example, consider a function that applies a transformation to every element in a vector:
#include <iostream>
#include <vector>
#include <algorithm>
#include <functional>
std::vector<int> applyFunction(const std::vector<int>& vec, std::function<int(int)> func) {
std::vector<int> result;
for (int n : vec) {
result.push_back(func(n));
}
return result;
}
int main() {
std::vector<int> vec = {1, 2, 3, 4};
std::vector<int> doubled = applyFunction(vec, [](int n) { return n * 2; });
for (int n : doubled) {
std::cout << n << " "; // Prints: 2 4 6 8
}
return 0;
}
The `applyFunction` takes a vector and a function as parameters, demonstrating the power of higher-order functions.
Immutability in C++
Functional programming often emphasizes immutability, meaning the data remains unchanged after creation. This principle reduces errors related to shared state between functions.
While C++ does not enforce immutability, best practices suggest using `const` and references to ensure that data cannot be modified unintentionally. Here's a practical example showcasing immutability principles:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
const std::vector<int> nums = {1, 2, 3, 4};
auto result = std::transform(nums.begin(), nums.end(), std::back_inserter(std::vector<int>()), [](int n) { return n * 2; });
// The original nums vector remains unchanged.
return 0;
}
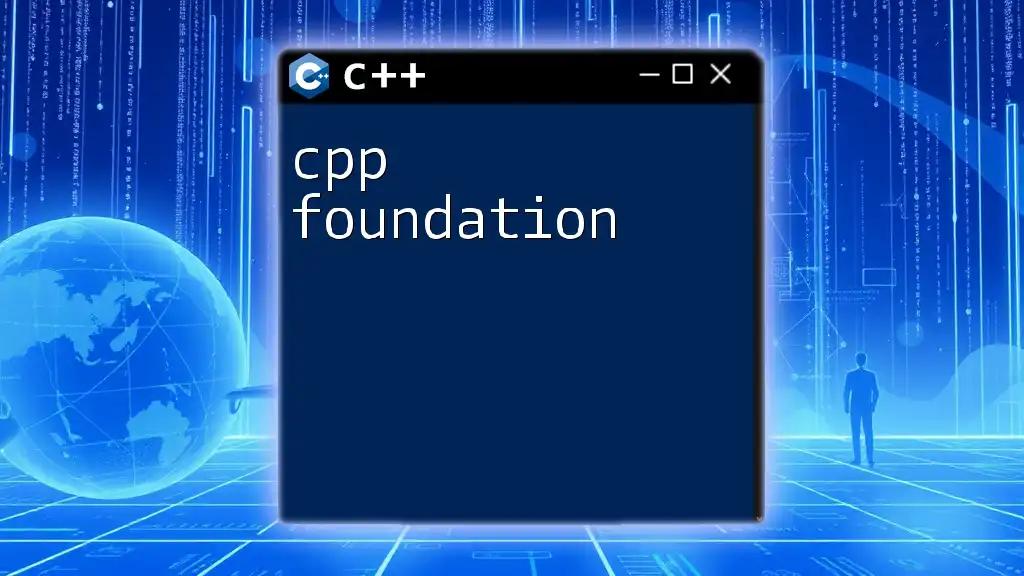
Common Use Cases of C++ Functional Programming
Callback Functions
Callback functions are functions passed as arguments to other functions and are executed at a specified time. They are commonly used in asynchronous programming and event-handling scenarios.
Here is an example that illustrates a simple use of callback functions:
#include <iostream>
#include <vector>
#include <algorithm>
void processNumbers(const std::vector<int>& nums, std::function<void(int)> func) {
for (int n : nums) {
func(n);
}
}
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
processNumbers(numbers, [](int n) {
std::cout << n * n << " "; // Prints: 1 4 9 16 25
});
return 0;
}
Using Functional Programming with STL Algorithms
The C++ STL is rich with algorithms that naturally lend themselves to functional programming. By combining STL algorithms with functional programming techniques, developers can write expressive code that is both efficient and readable.
Here’s an example using the `std::transform` algorithm:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> input = {1, 2, 3, 4};
std::vector<int> output;
std::transform(input.begin(), input.end(), std::back_inserter(output), [](int n) {
return n * 3;
});
for (const auto& n : output) {
std::cout << n << " "; // Prints: 3 6 9 12
}
return 0;
}
In this case, `std::transform` is used to create a new vector based on transformations applied to each element from the original vector.
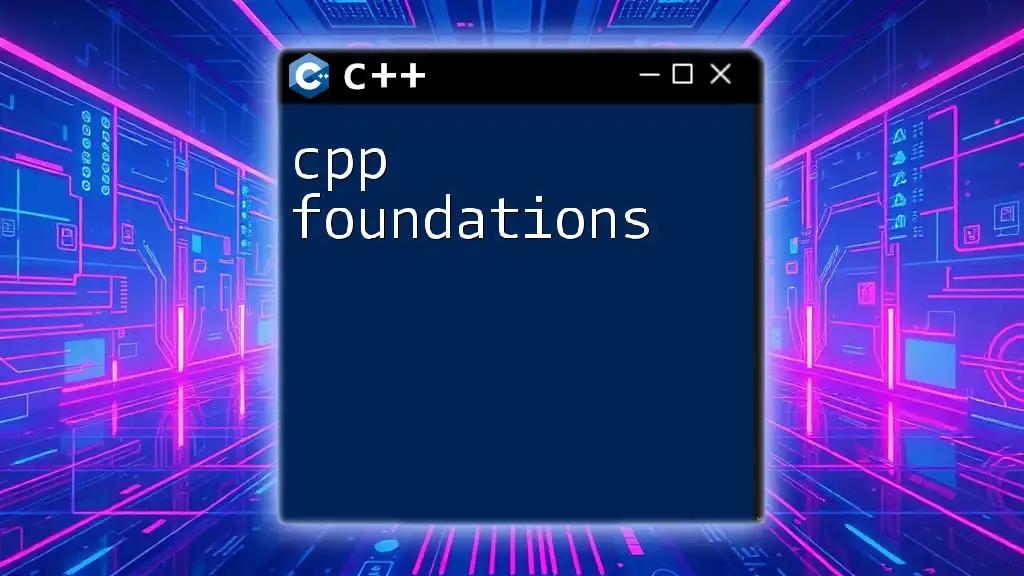
Conclusion
In conclusion, the cpp functional programming paradigm allows for the development of more modular, readable, and maintainable code. Leveraging the capabilities of the C++ Standard Library's `<functional>` header, function objects, lamdbas, and higher-order functions can significantly enhance your programming experience.
Functional programming is not only about the techniques you use; it's a mindset that embraces immutability, pure functions, and a declarative approach to problems. As C++ continues to evolve, its support for functional programming opens the doors to more expressive and powerful coding practices. Embracing this paradigm can lead to cleaner and more efficient code in your C++ projects.
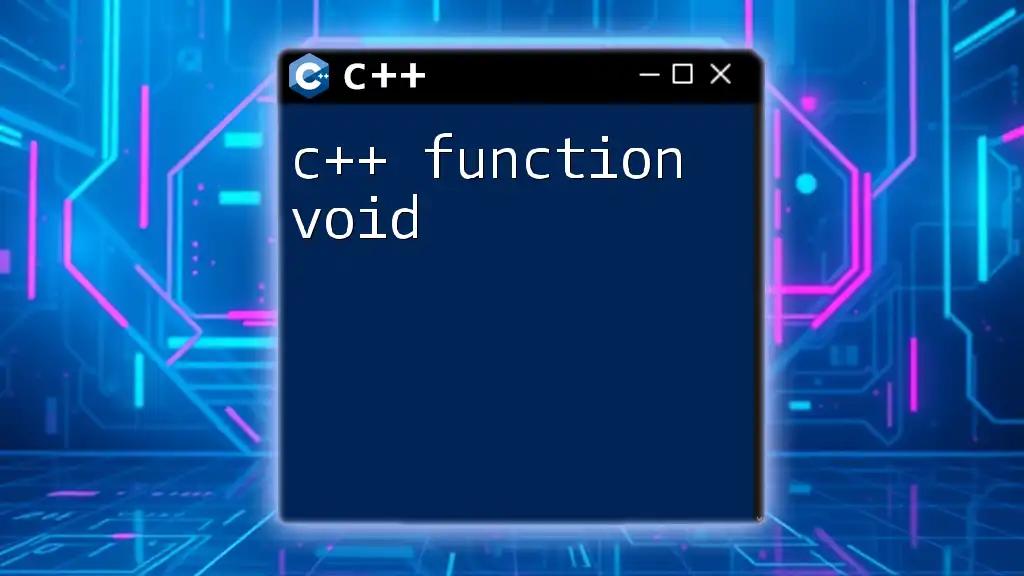
Additional Resources
For those eager to dive deeper into cpp functional programming, consider exploring a variety of books, online tutorials, and official documentation. These resources can provide valuable insights and a broader understanding of functional programming techniques within C++.